- 사각형: RectangleShape
- 원: CircleShape
- 모서리가 둥근 사각형: RoundedCornerShape(size)
- 팔각형(모서리를 자른 사각형): CutCornerShape(size)
@Composable
fun ShapeContainer() {
var polySides by remember { mutableStateOf(3) }
Column(
modifier = Modifier
.background(Color.White)
.fillMaxSize(),
verticalArrangement = Arrangement.SpaceEvenly,
horizontalAlignment = Alignment.CenterHorizontally
) {
DummyBox(modifier = Modifier.clip(RectangleShape))
DummyBox(modifier = Modifier.clip(CircleShape))
DummyBox(modifier = Modifier.clip(RoundedCornerShape(10.dp)))
DummyBox(modifier = Modifier.clip(CutCornerShape(10.dp)))
}
}
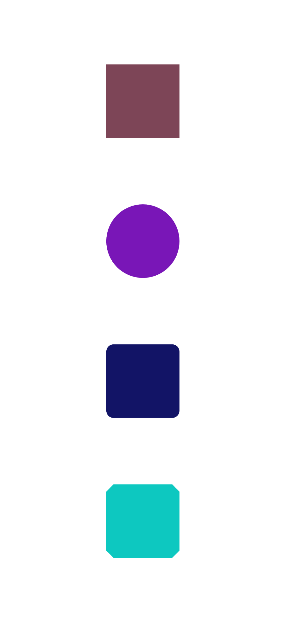
- Shape를 직접 설정도 가능
- Path에서 moveTo와 lineTo로 Box 형태에서 자름
class TriangleShape(): Shape {
override fun createOutline(
size: Size,
layoutDirection: LayoutDirection,
density: Density
): Outline {
val path = Path().apply {
moveTo(size.width / 2f, 0f)
lineTo(size.width, size.height)
lineTo(0f, size.height)
close()
}
return Outline.Generic(path = path)
}
}
변의 수와 크기를 지정하여 도형을 생성하는 방법
class PolyShape(private val sides: Int, private val radius: Float): Shape {
override fun createOutline(
size: Size,
layoutDirection: LayoutDirection,
density: Density
): Outline {
return Outline.Generic(path = Path().apply { this.polygon(sides, radius, size.center) })
}
}
fun Path.polygon(sides: Int, radius: Float, center: Offset) {
val angle = 2.0 * Math.PI / sides
moveTo(
x = center.x + (radius * cos(0.0)).toFloat(),
y = center.y + (radius * sin(0.0)).toFloat()
)
for (i in 1 until sides) {
lineTo(
x = center.x + (radius * cos(angle * i)).toFloat(),
y = center.y + (radius * sin(angle * i)).toFloat()
)
}
close()
}
DummyBox(modifier = Modifier.clip(PolyShape(polySides, 100f)))
Text(text = "polySize: $polySides")
Row(
horizontalArrangement = Arrangement.SpaceEvenly,
modifier = Modifier.fillMaxWidth()
) {
Button(onClick = {
polySides++
}) {
Text(text = "polySize + 1")
}
Button(onClick = {
polySides = 3
}) {
Text(text = "polySize = 3")
}
}
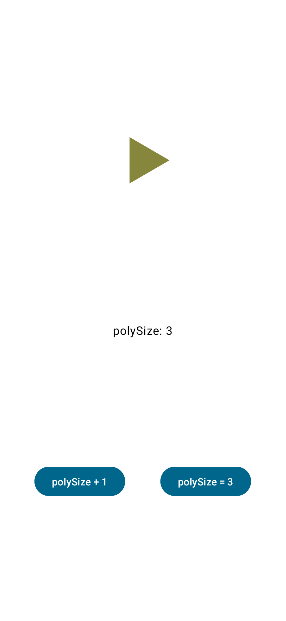