Making mysite file
- On terminal,
$ django-admin startproject mysite
will make a file called 'mysite' on selected directory.
- Under mysite file, these python files exist.
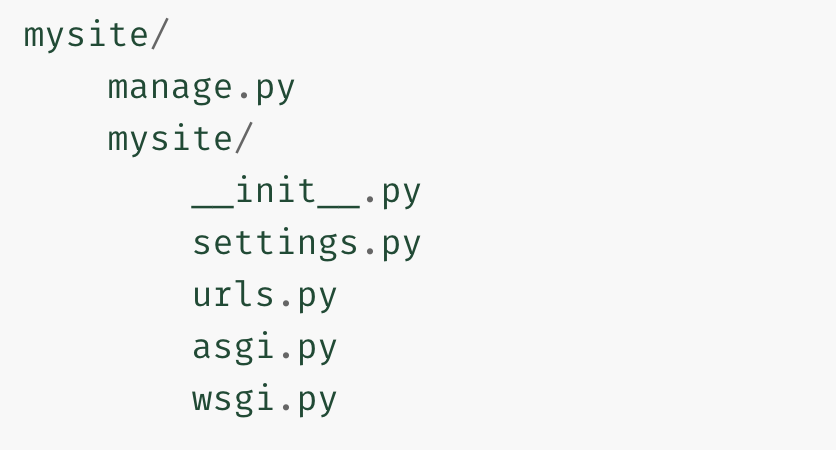
What do these individual files do?
manage.py
- Django 프로젝트와 다양한 방법으로 상호작용 하는 커맨드라인의 유틸리티 입니다.
__init__.py
- Python으로 하여금 이 디렉토리를 패키지처럼 다루라고 알려주는 용도의 단순한 빈 파일입니다.
settings.py
- 현재 Django 프로젝트의 환경 및 구성을 저장합니다.
urls.py
- 현재 Django project 의 URL 선언을 저장합니다. Django 로 작성된 사이트의 "목차" 라고 할 수 있습니다.
Running the server
$ python manage.py runserver
will start the server.
Making a website called polls
$ python manage.py startapp polls
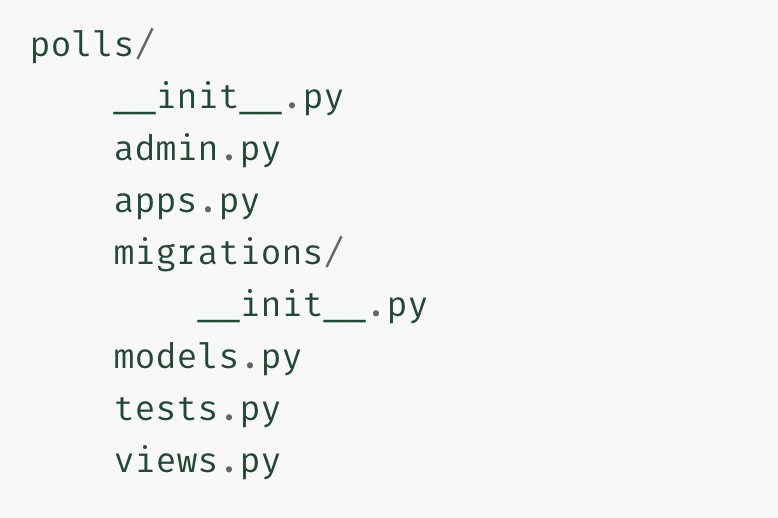
1. polls/view.py
- A view function is a Python function that takes a Web Request and returns a Web resopnse.
from django.http import HttpResponse
def index(request):
return HttpResponse("Hello, world. You're at the polls index.")
- In order to call view, we need an url that connects to the view. So, we need to created urls.py to create URLconf. (A URLconf is a mapping between URLs and the view functions that should be called for those URLs.)
2. polls/urls.py
from django.urls import path
from . import views
urlpatterns = [
path('', views.index, name='index'),
]
3. mysite/urls.py
fron django.contrib import admin
from django.urls import include, path
urlpatterns = [
path('polls/', include('polls.urls')),
path('admin/', admin.site.urls),
]
- Here, include() function lets you have access to other URLconf.
- When Django meets include(), it cuts the part of URL up to that point, and return the rest to URLconf.
- For example,
path('ads.txt', views.ads)
means when the web client asks for 'ads.txt', 'ads' from home/views.py is called.
- When you use different URL patters, you must use include(), with the exception of
admin.site.urls