.env
JWT_SECRET_KEY=secret
JWT_EXPIRES=5m
BCRYPT_SALT_ROUNDS=12
SERVER_PORT=8000
config.js
import dotenv from "dotenv";
dotenv.config();
export const config = {
jwtSecretKey: process.env.JWT_SECRET_KEY,
jwtExpires: process.env.JWT_EXPIRES,
bcryptSaltRounds: parseInt(process.env.BCRYPT_SALT_ROUNDS),
port: parseInt(process.env.SERVER_PORT),
};
database.js
import { Sequelize } from "sequelize";
const sequelize = new Sequelize("twitter", "root", "0000", {
host: "127.0.0.1",
dialect: "mysql",
});
export default sequelize;
app.js
import express from "express";
import helmet from "helmet";
import morgan from "morgan";
import cors from "cors";
import tweetController from "./tweet/tweet.controller.js";
import authController from "./auth/auth.controller.js";
import { config } from "../config.js";
import sequelize from "../database.js";
const app = express();
app.use(express.json());
app.use(morgan("dev"));
app.use(helmet());
app.use(cors());
app.use("/tweet", tweetController);
app.use("/auth", authController);
app.use((req, res, next) => {
res.sendStatus(404);
});
app.use((err, req, res, next) => {
res.sendStatus(500);
});
sequelize.sync().then(() => {
app.listen(config.port, () => {
console.log("Server On...");
});
});
user.repository.js
import { DataTypes } from "sequelize";
import sequelize from "../../database.js";
export const User = sequelize.define("user", {
id: {
type: DataTypes.BIGINT,
autoIncrement: true,
allowNull: false,
primaryKey: true,
},
name: {
type: DataTypes.STRING(100),
allowNull: false,
},
username: {
type: DataTypes.STRING(100),
allowNull: false,
},
password: {
type: DataTypes.STRING(100),
allowNull: false,
},
email: {
type: DataTypes.STRING(100),
allowNull: false,
},
});
export const findByUsername = async (username) => {
return User.findOne({
where: {
username,
},
}).then((data) => data.dataValues);
};
export const findById = async (id) => {
return User.findByPk(id);
};
export const createUser = async (user) => {
return User.create(user).then((data) => data.dataValues.id);
};
import sequelize from "../../database.js";
import * as userRepository from "../user/user.repository.js";
import { DataTypes } from "sequelize";
export const Tweet = sequelize.define("tweet", {
id: {
type: DataTypes.BIGINT,
autoIncrement: true,
allowNull: false,
primaryKey: true,
},
text: {
type: DataTypes.TEXT,
allowNull: false,
},
});
Tweet.belongsTo(userRepository.User);
export const getAllTweets = async () => {
return Tweet.findAll({
attributes: ["id", "text", "createdAt"],
include: {
model: userRepository.User,
attributes: ["id", "username"],
},
order: [["createdAt", "DESC"]],
});
};
export const getAllTweetsByUsername = async (username) => {
return Tweet.findAll({
attributes: ["id", "text", "createdAt"],
include: {
model: userRepository.User,
attributes: ["id", "username"],
where: {
username,
},
},
order: [["createdAt", "DESC"]],
});
};
export const getTweetById = async (id) => {
return Tweet.findOne({
attributes: ["id", "text", "createdAt"],
include: {
model: userRepository.User,
attributes: ["id", "username"],
},
where: {
id,
},
}).then((data) => data.dataValues);
};
export const createTweet = async (text, userId) => {
return Tweet.create({ text, userId }).then((data) => data.dataValues);
};
export const updateTweet = async (id, text) => {
Tweet.update(
{
text,
},
{
where: {
id,
},
}
);
};
export const deleteTweet = async (id) => {
Tweet.destroy({
where: {
id,
},
});
};
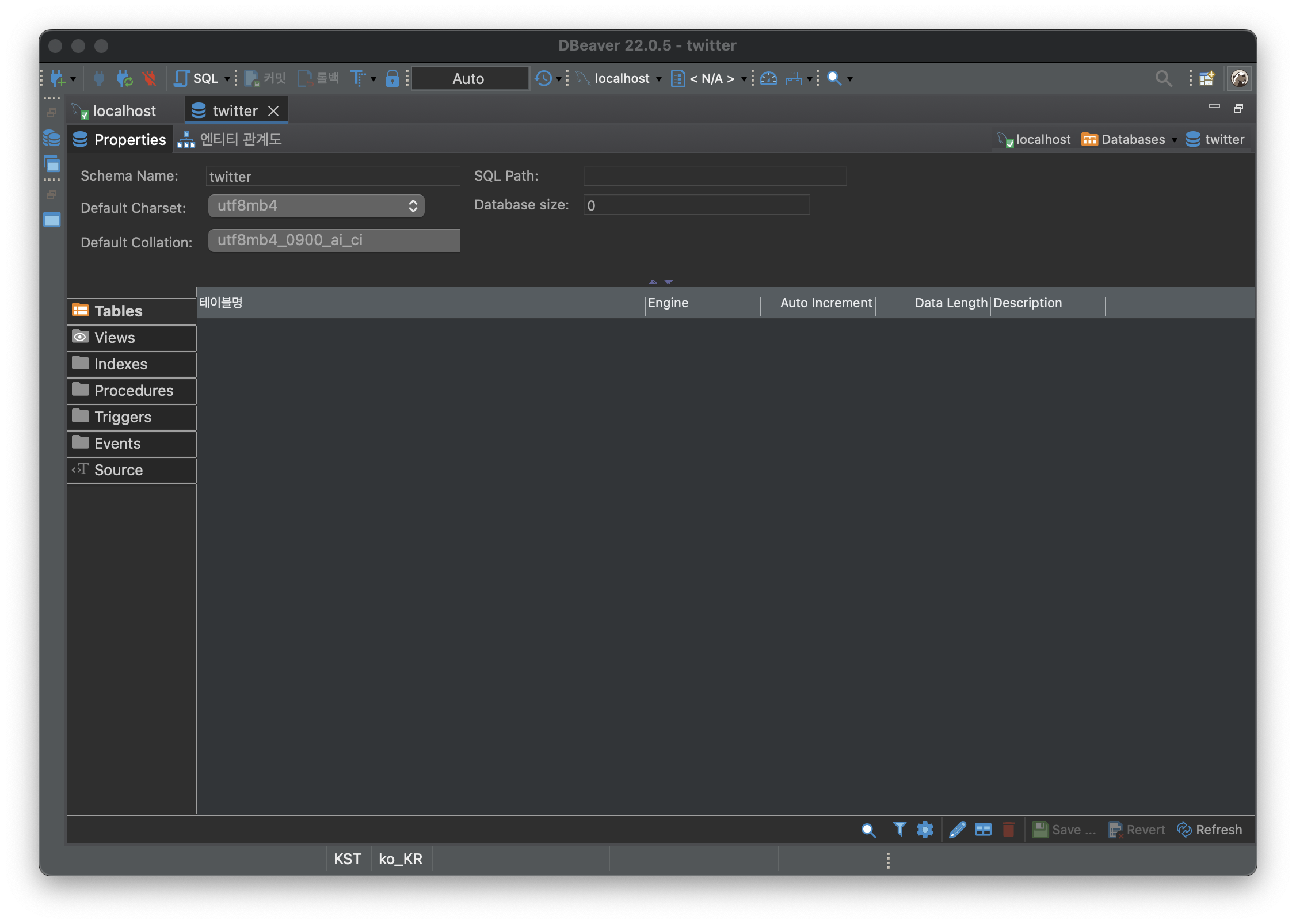
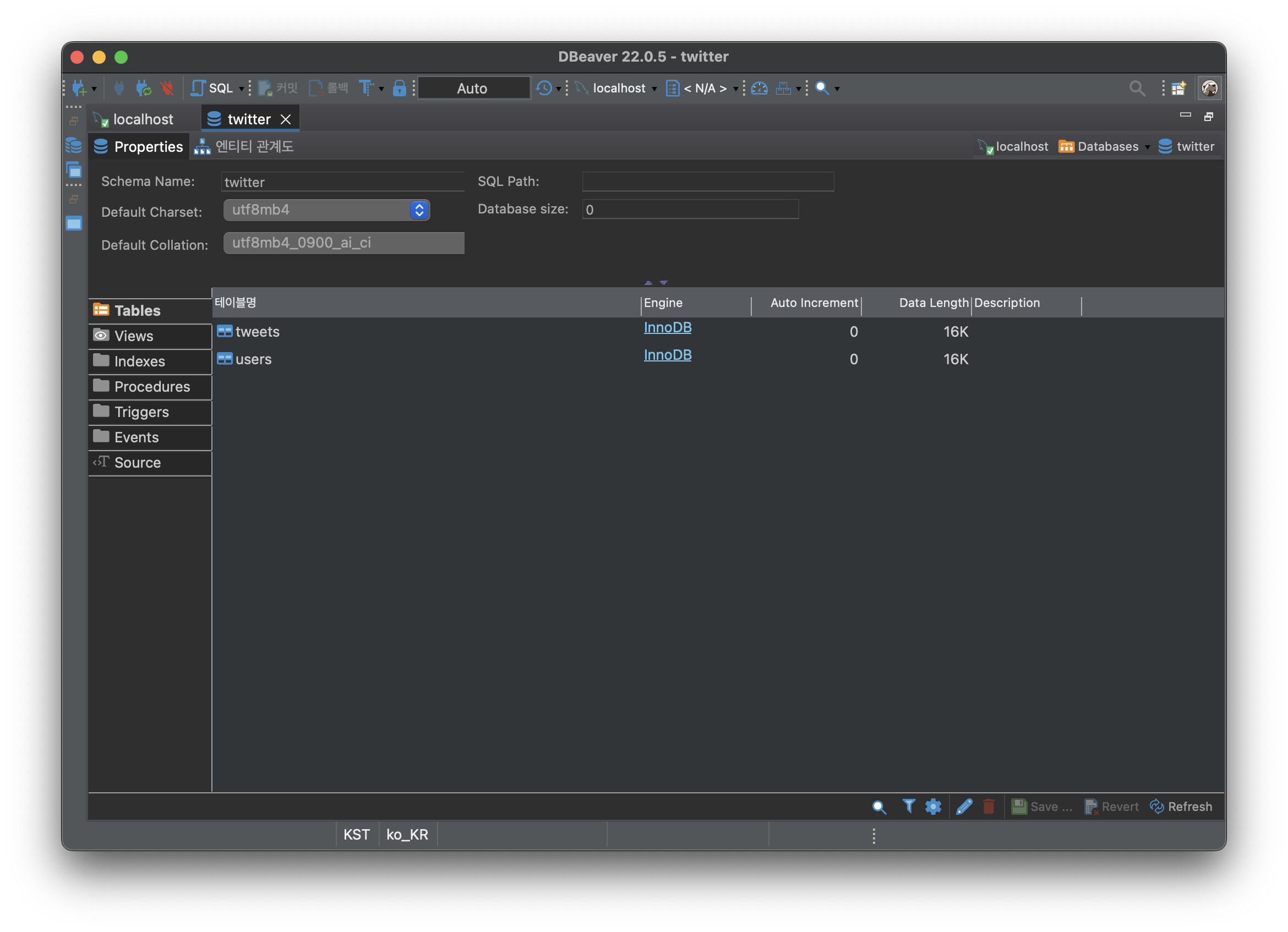
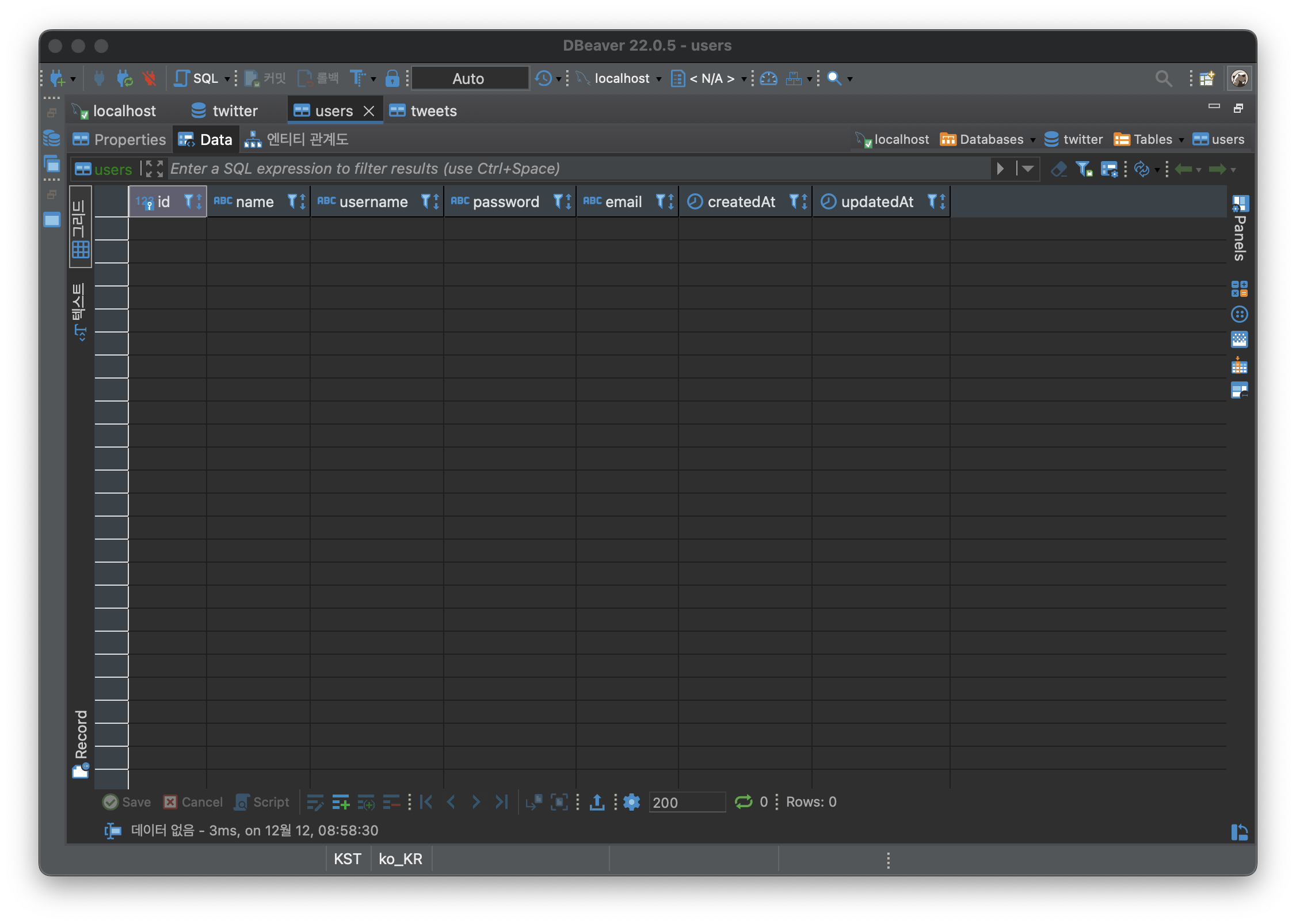
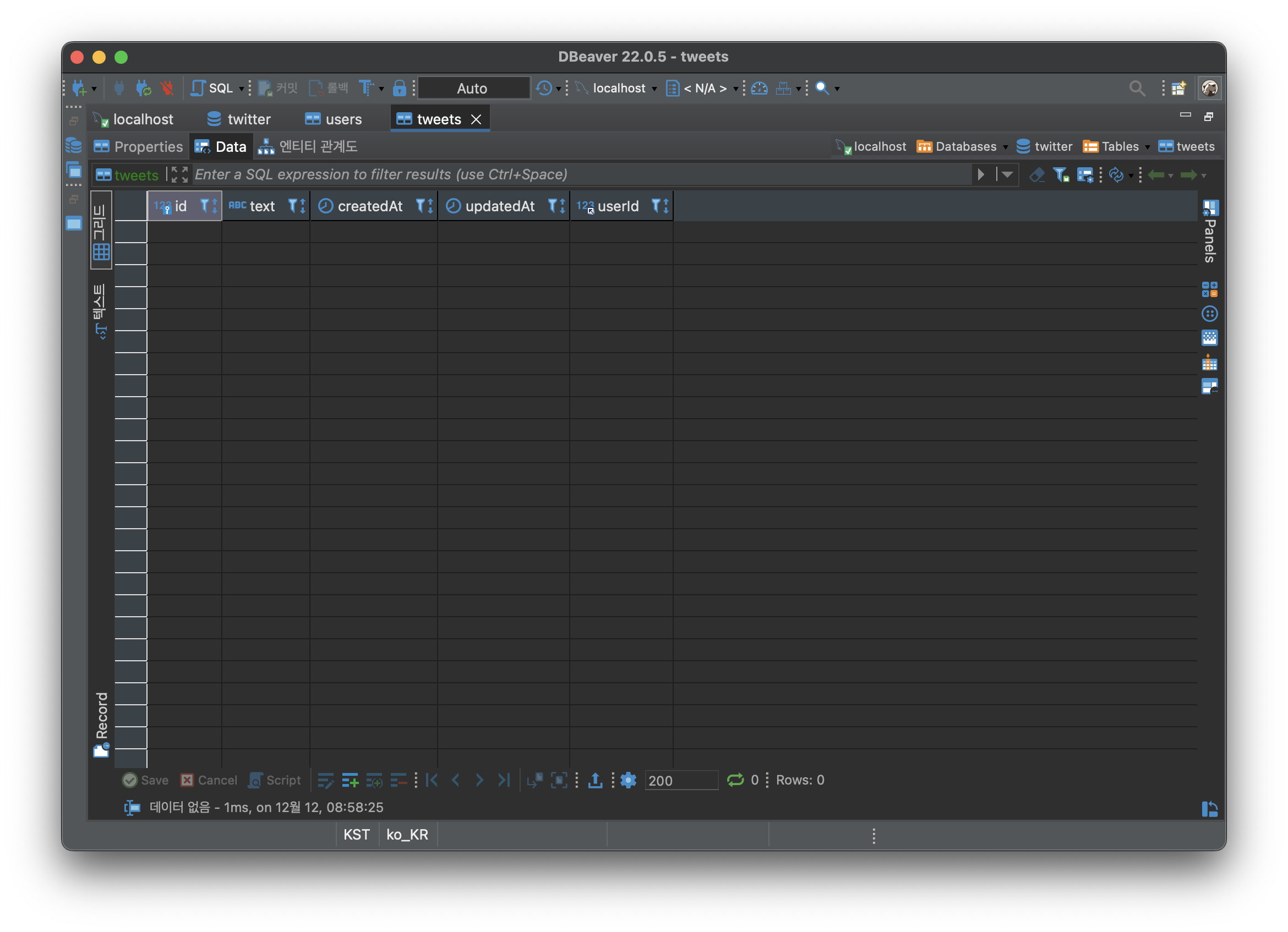
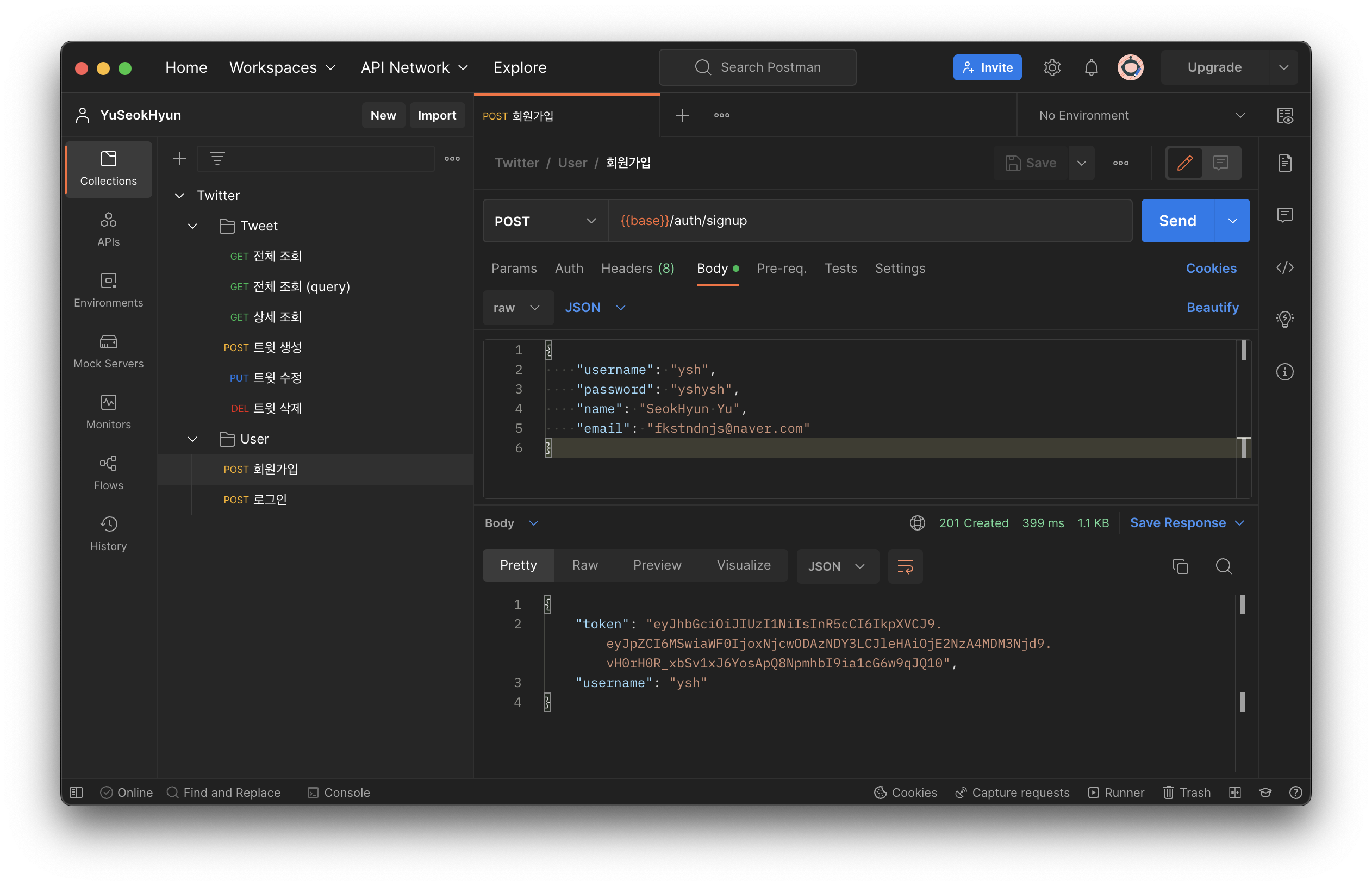
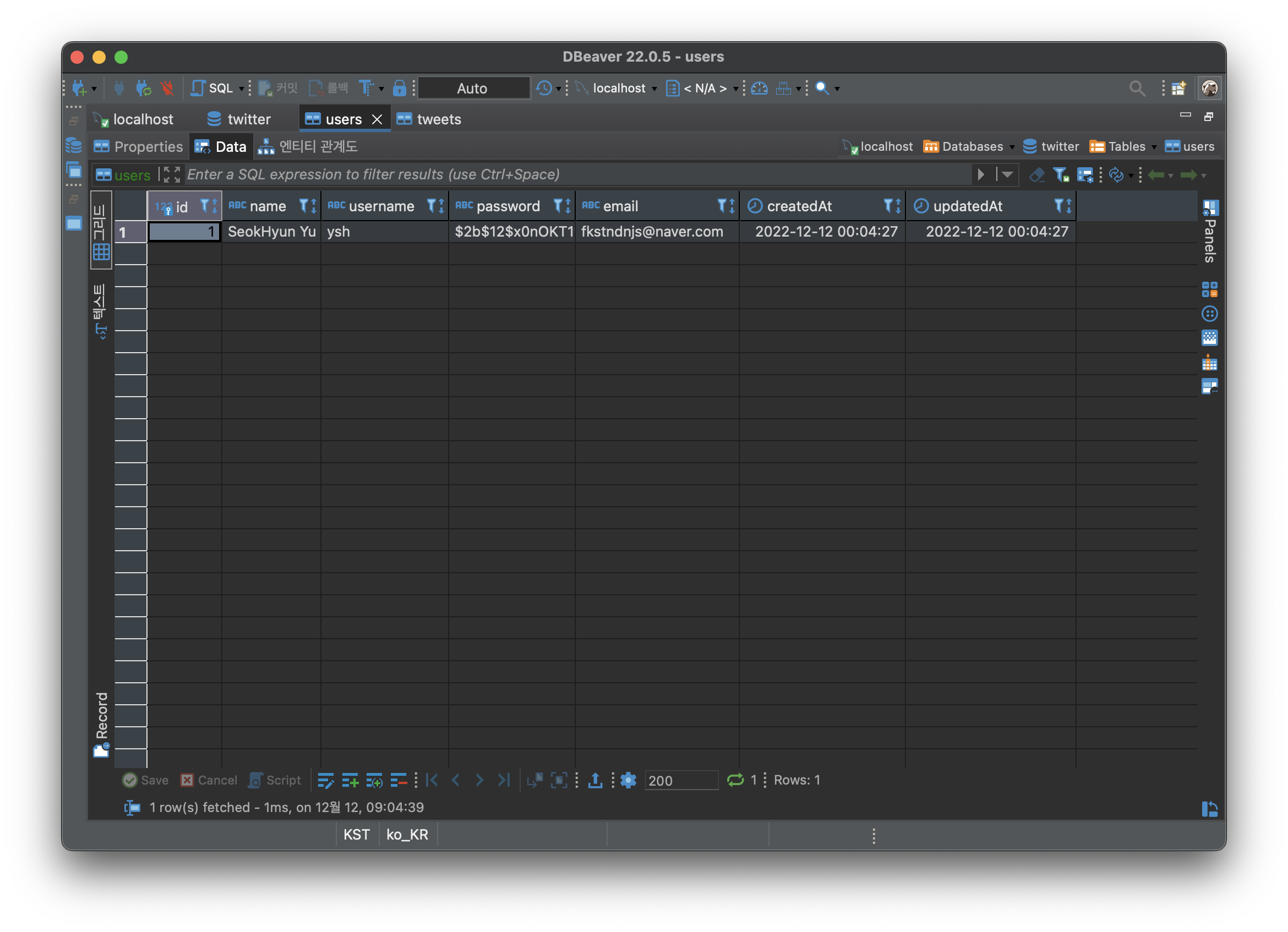
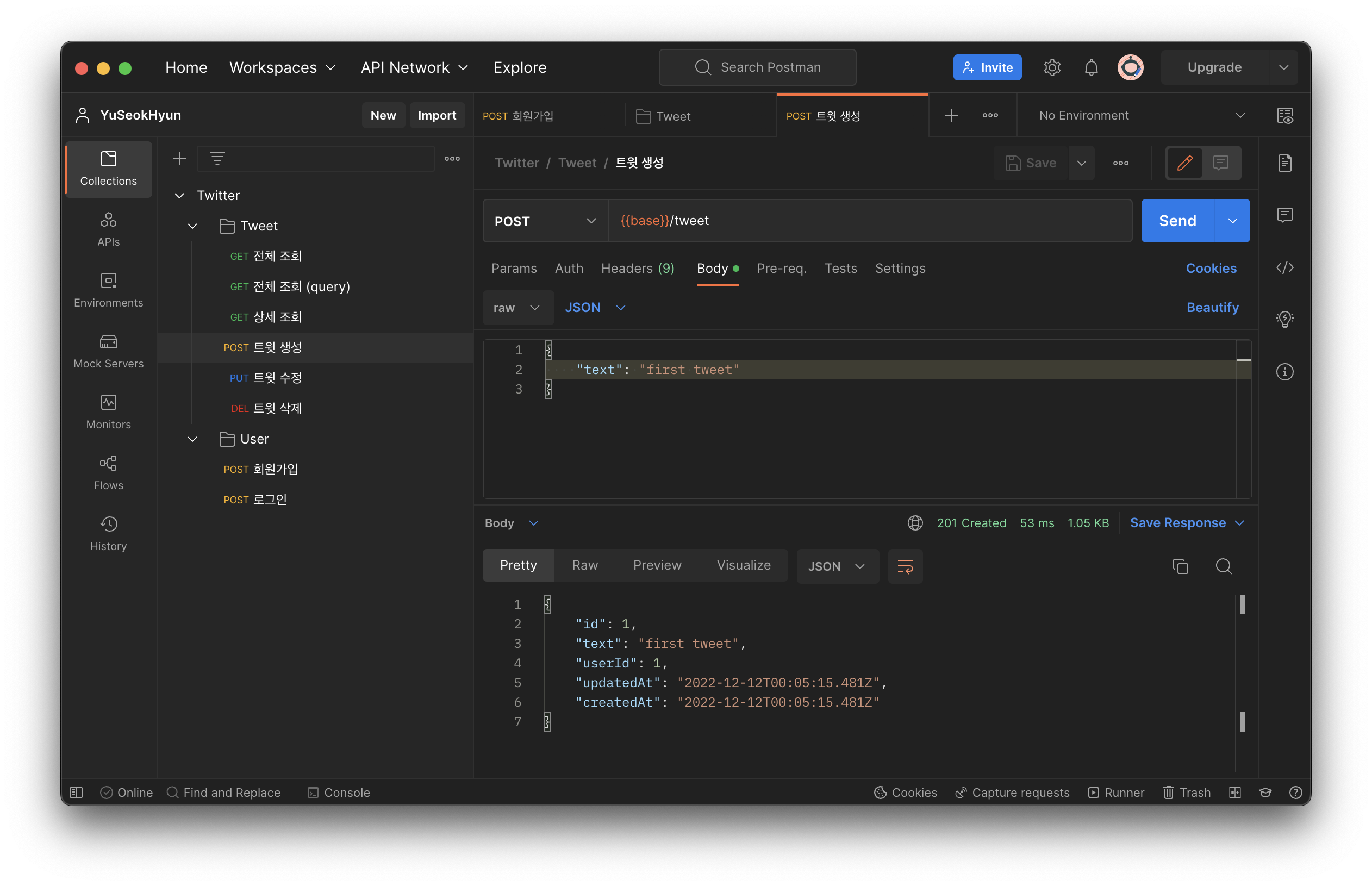
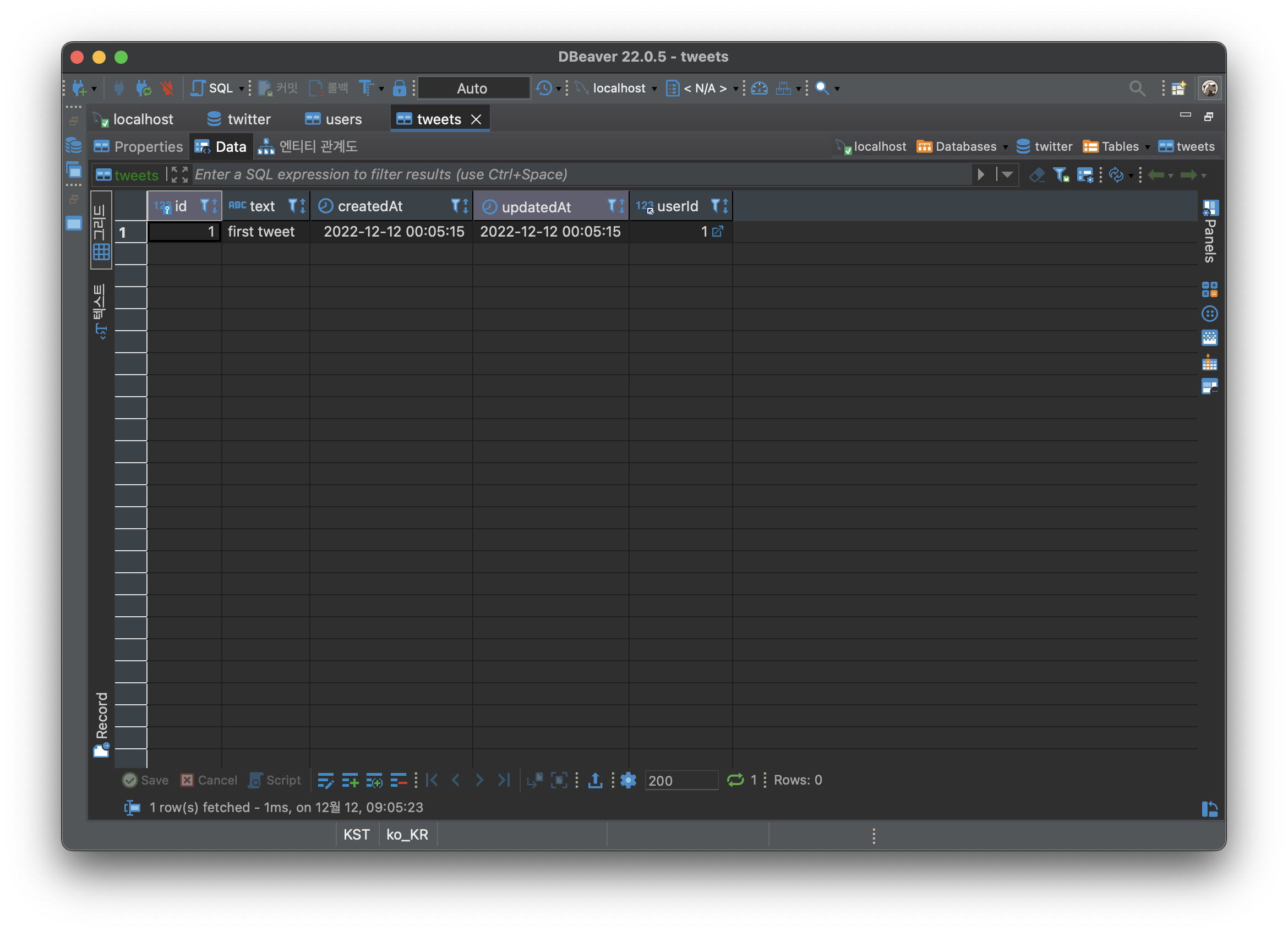
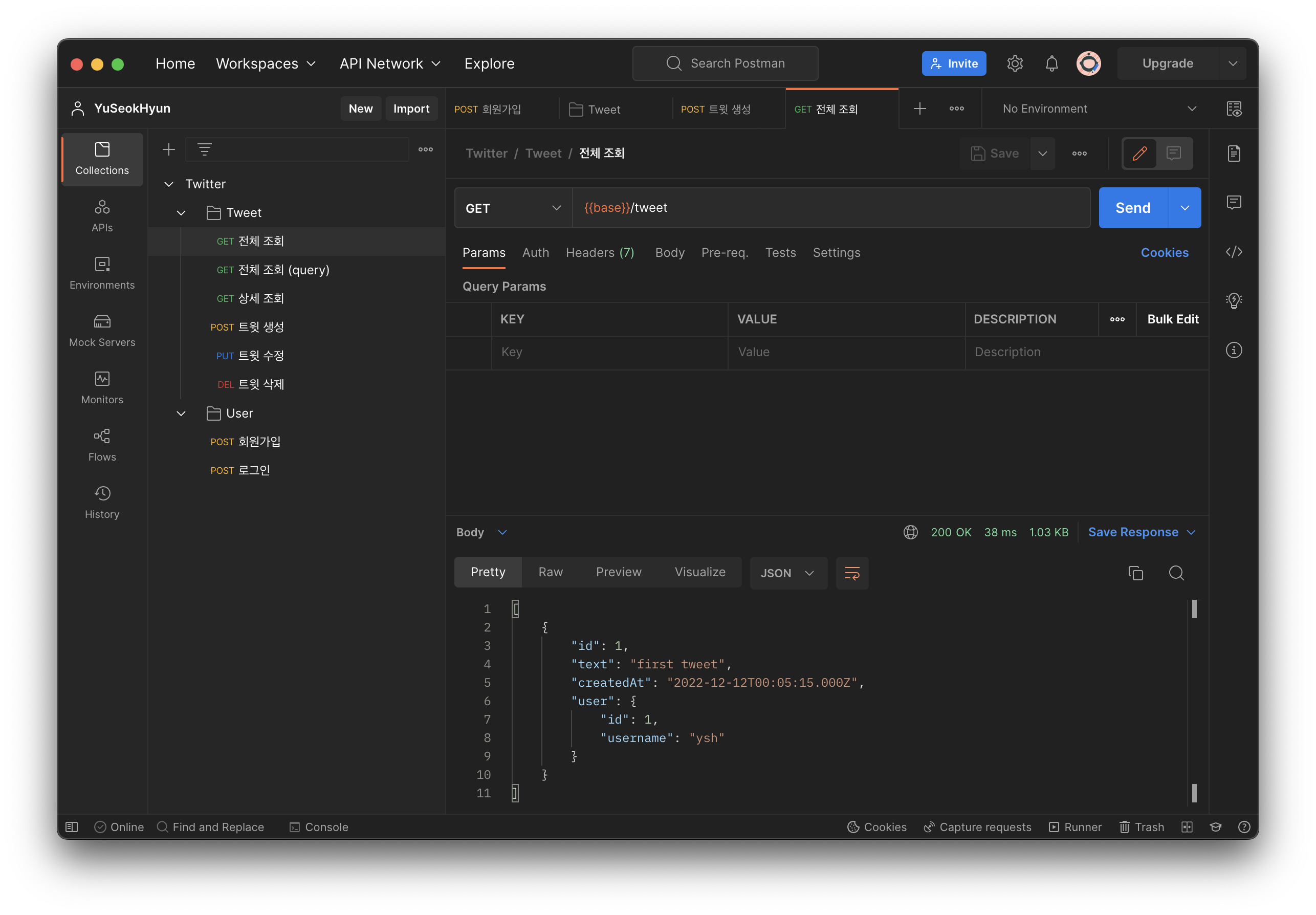
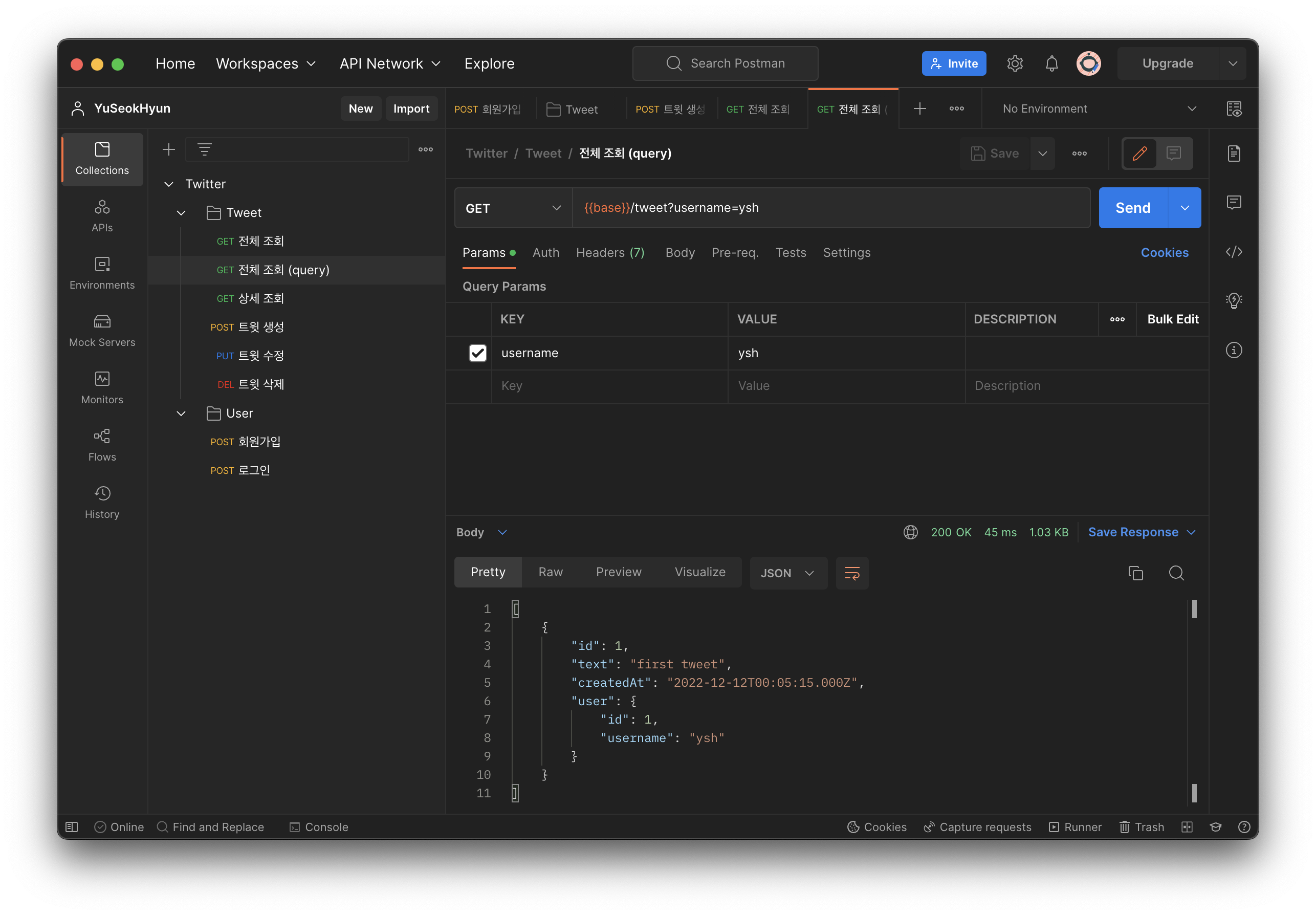
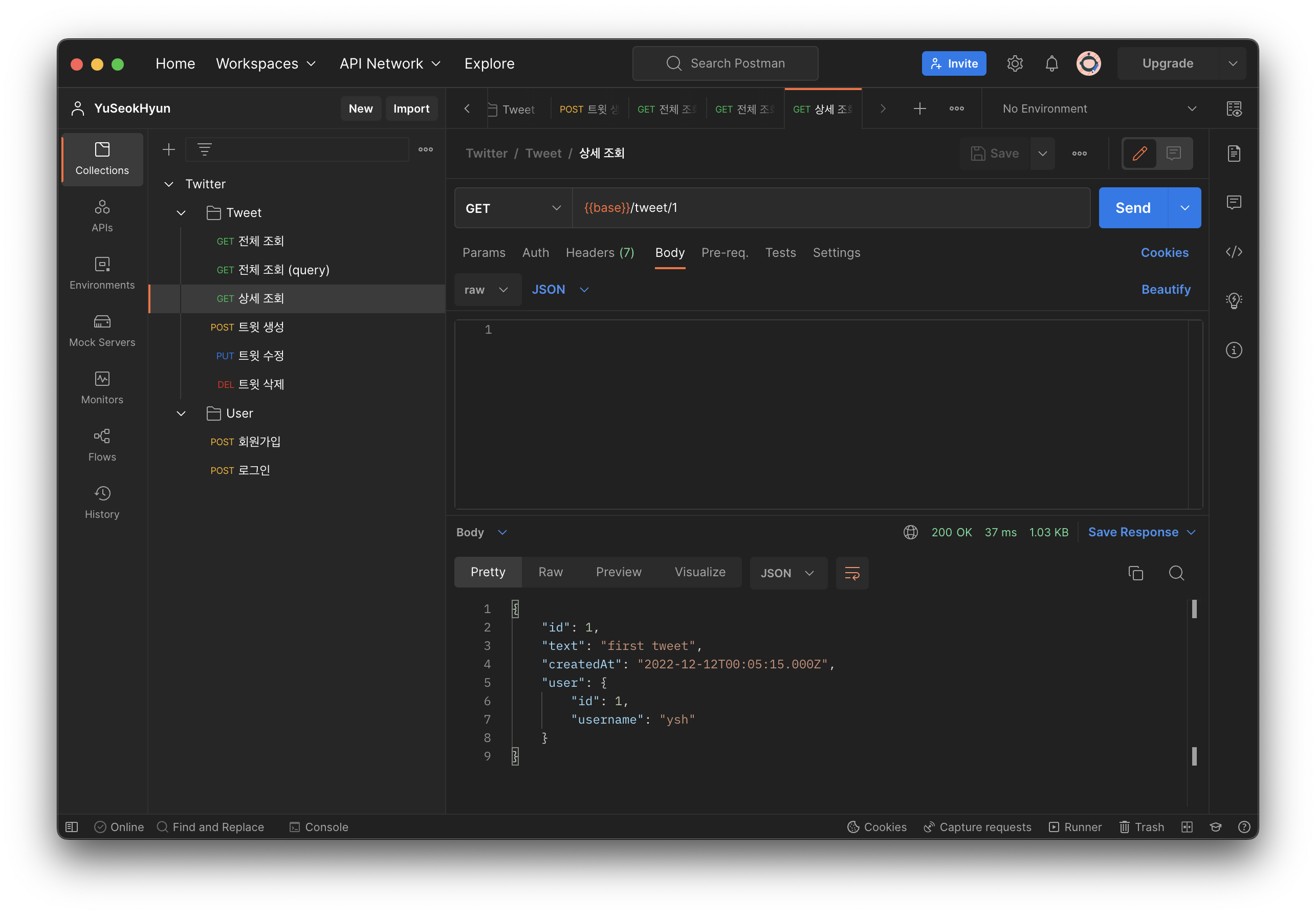
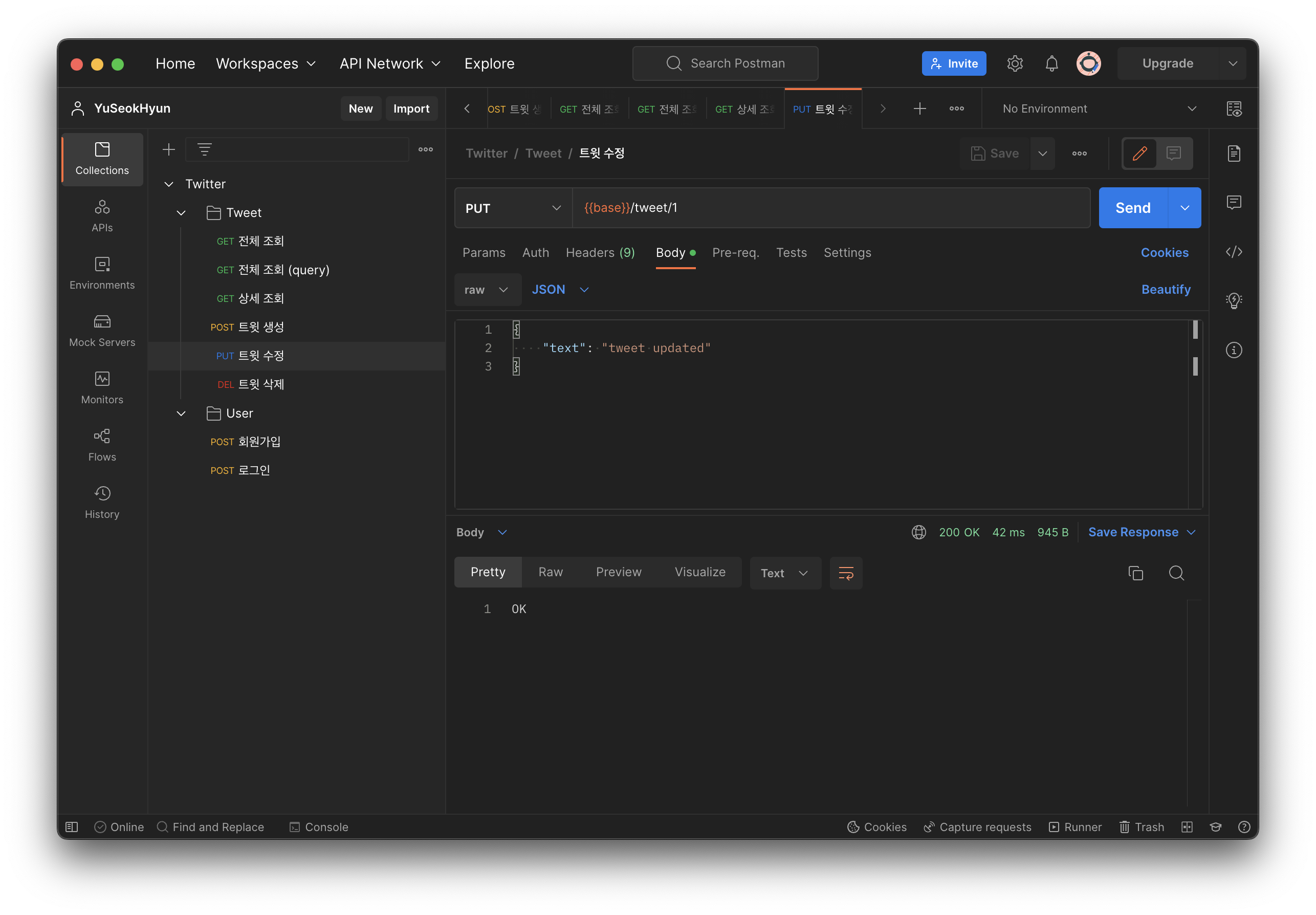
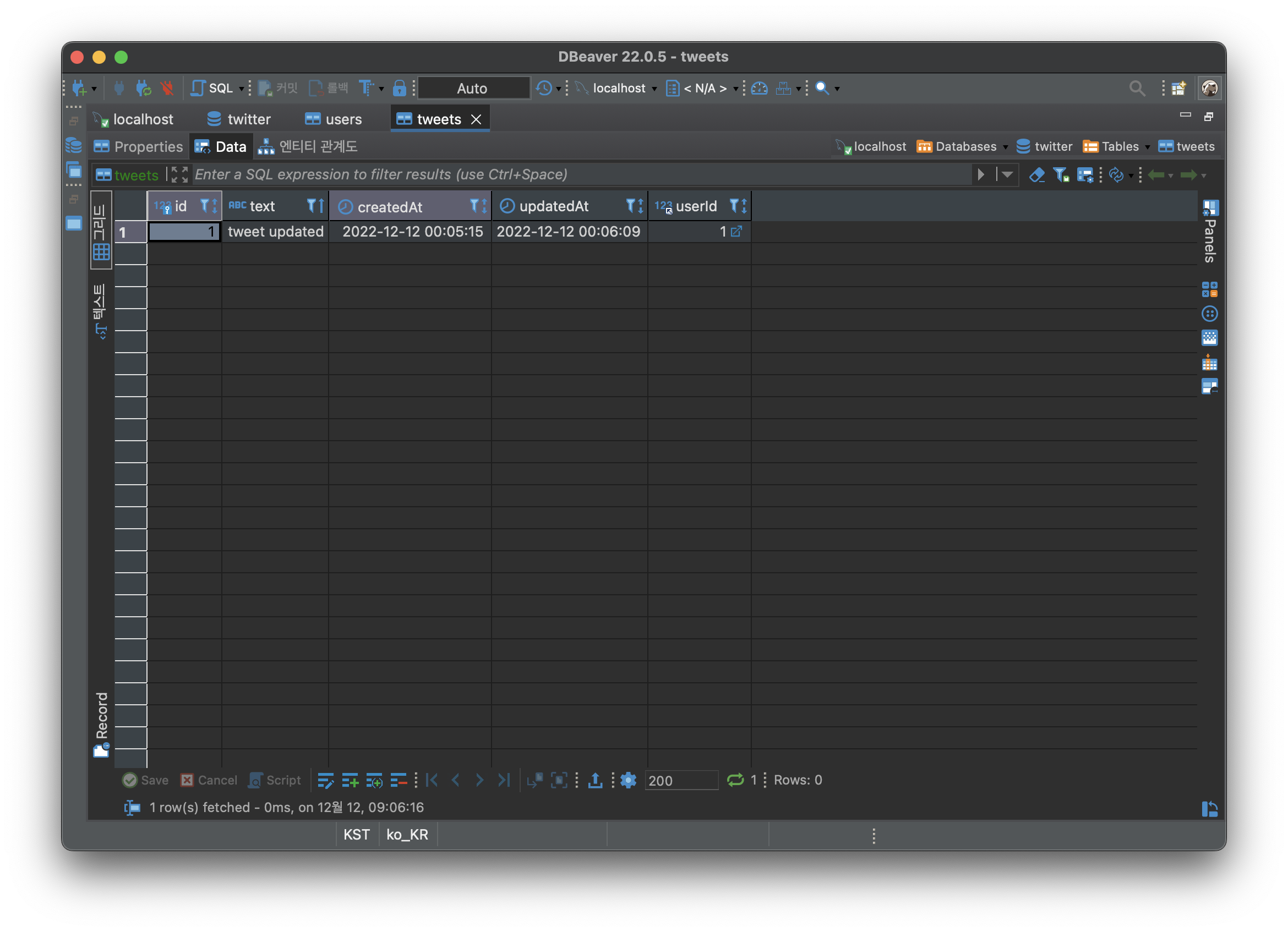
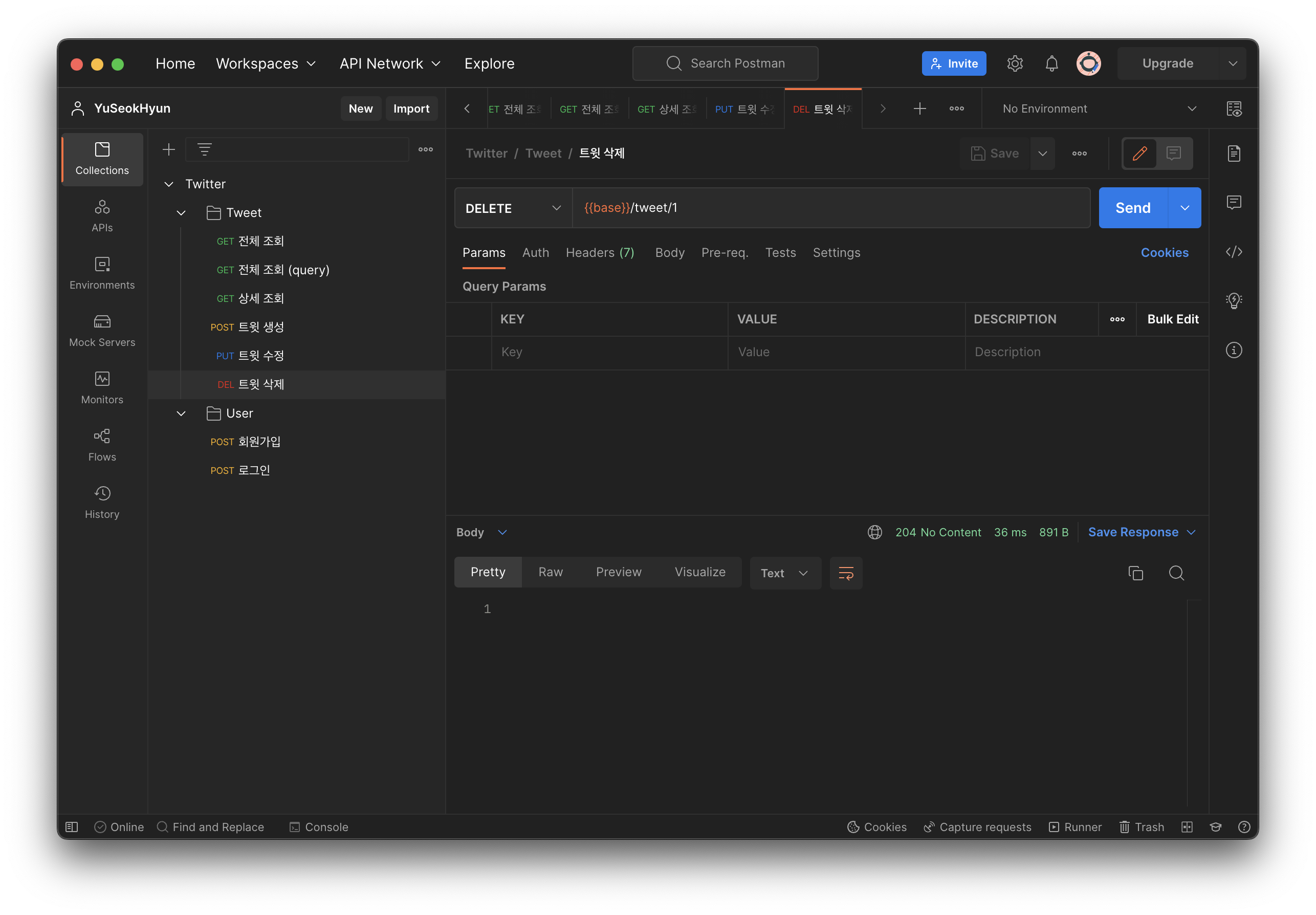
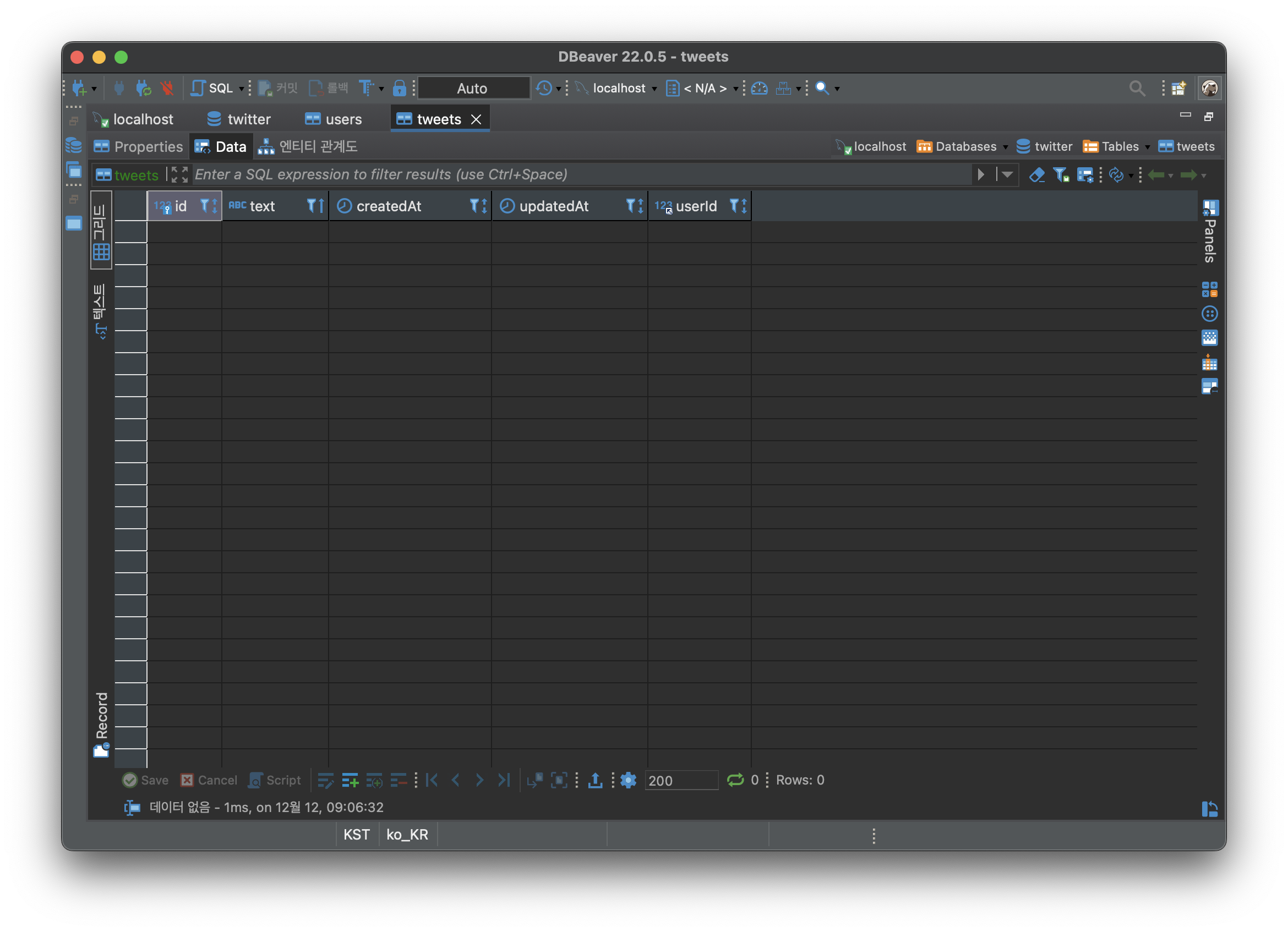