database.js
import mysql from "mysql2";
const pool = mysql.createPool({
host: "127.0.0.1",
user: "root",
database: "twitter",
password: "0000",
});
const db = pool.promise();
export default db;
app.js
import express from "express";
import helmet from "helmet";
import morgan from "morgan";
import cors from "cors";
import tweetController from "./tweet/tweet.controller.js";
import authController from "./auth/auth.controller.js";
import { config } from "../config.js";
import db from "../database.js";
const app = express();
app.use(express.json());
app.use(morgan("dev"));
app.use(helmet());
app.use(cors());
app.use("/tweet", tweetController);
app.use("/auth", authController);
app.use((req, res, next) => {
res.sendStatus(404);
});
app.use((err, req, res, next) => {
res.sendStatus(500);
});
db.getConnection();
app.listen(config.port, () => {
console.log("Server On...");
});
user.repository.js
import db from "../../database.js";
export const findByUsername = async (username) => {
return db
.execute("SELECT * FROM user WHERE username=?", [username])
.then((data) => data[0][0]);
};
export const findById = async (id) => {
return db
.execute("SELECT * FROM user WHERE id=?", [id])
.then((data) => data[0][0]);
};
export const createUser = async (user) => {
const { username, password, name, email } = user;
return db
.execute(
`INSERT INTO user(username, password, name, email) VALUES(?, ?, ?, ?)`,
[username, password, name, email]
)
.then((data) => {
return data[0].insertId;
});
};
auth.service.js
import * as userRepository from "../user/user.repository.js";
import bcrypt from "bcrypt";
import jwt from "jsonwebtoken";
import { config } from "../../config.js";
const createToken = (id) => {
return jwt.sign({ id }, config.jwtSecretKey, {
expiresIn: config.jwtExpires,
});
};
export const signup = async (req, res) => {
const { username, password, name, email } = req.body;
const user = await userRepository.findByUsername(username);
if (user) {
return res.status(409).json({ message: `이미 가입된 유저입니다.` });
}
const hashed = await bcrypt.hash(password, config.bcryptSaltRounds);
const createdUserId = await userRepository.createUser({
username,
password: hashed,
name,
email,
});
const token = createToken(createdUserId);
res.status(201).json({ token, username });
};
export const login = async (req, res) => {
const { username, password } = req.body;
const user = await userRepository.findByUsername(username);
if (!user) {
return res
.status(401)
.json({ message: "아이디 혹은 비밀번호가 틀렸습니다." });
}
const isValidPassword = await bcrypt.compare(password, user.password);
if (!isValidPassword) {
return res
.status(401)
.json({ message: "아이디 혹은 비밀번호가 틀렸습니다." });
}
const token = createToken(user.id);
res.status(200).json({ token, username });
};
export const me = async (req, res) => {
const user = await userRepository.findById(req.userId);
if (user) {
return res.status(200).json({ token: req.token, username: user.username });
}
res.status(404).json({ message: "유저가 존재하지 않습니다." });
};
user 데이터베이스
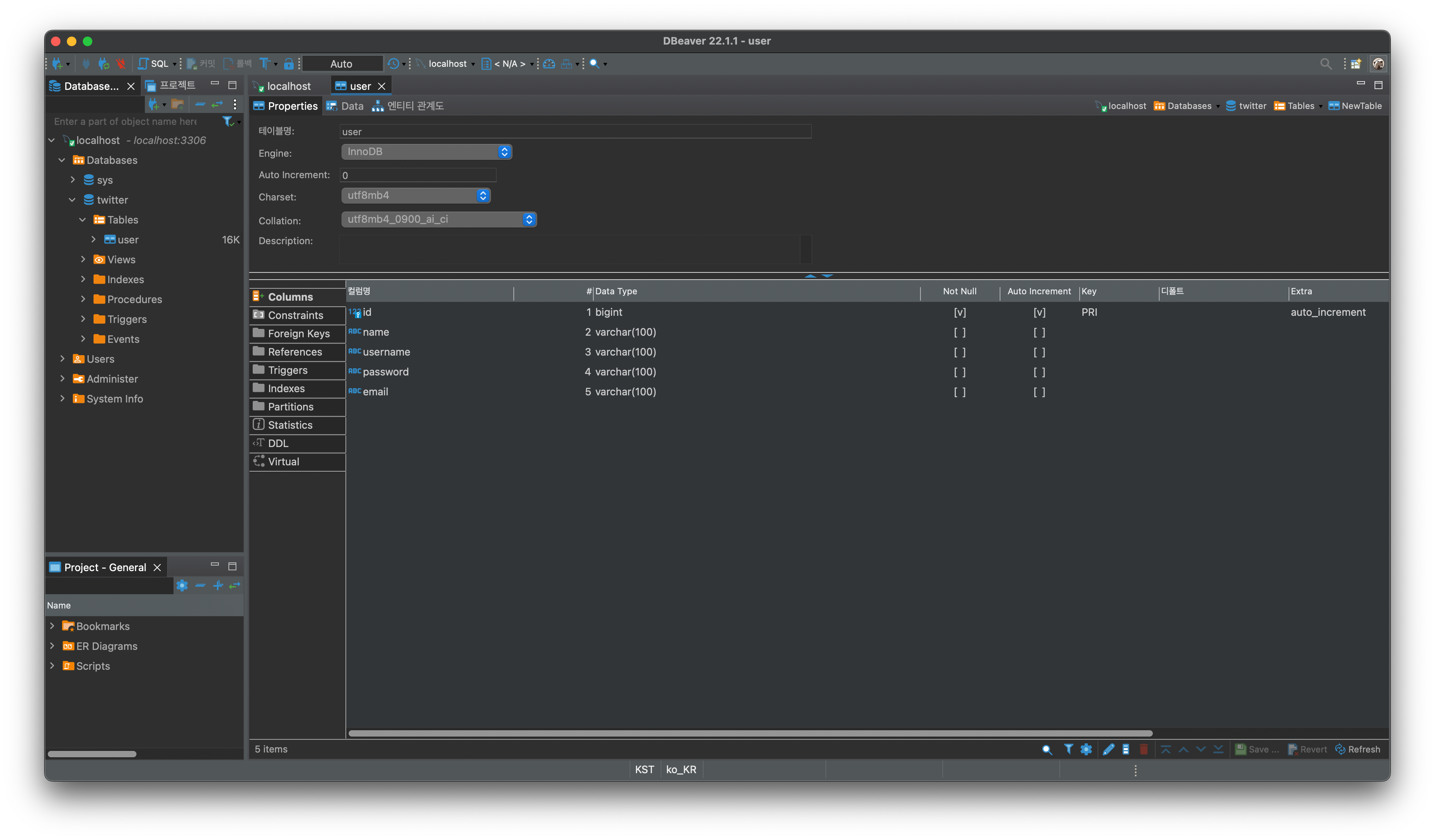
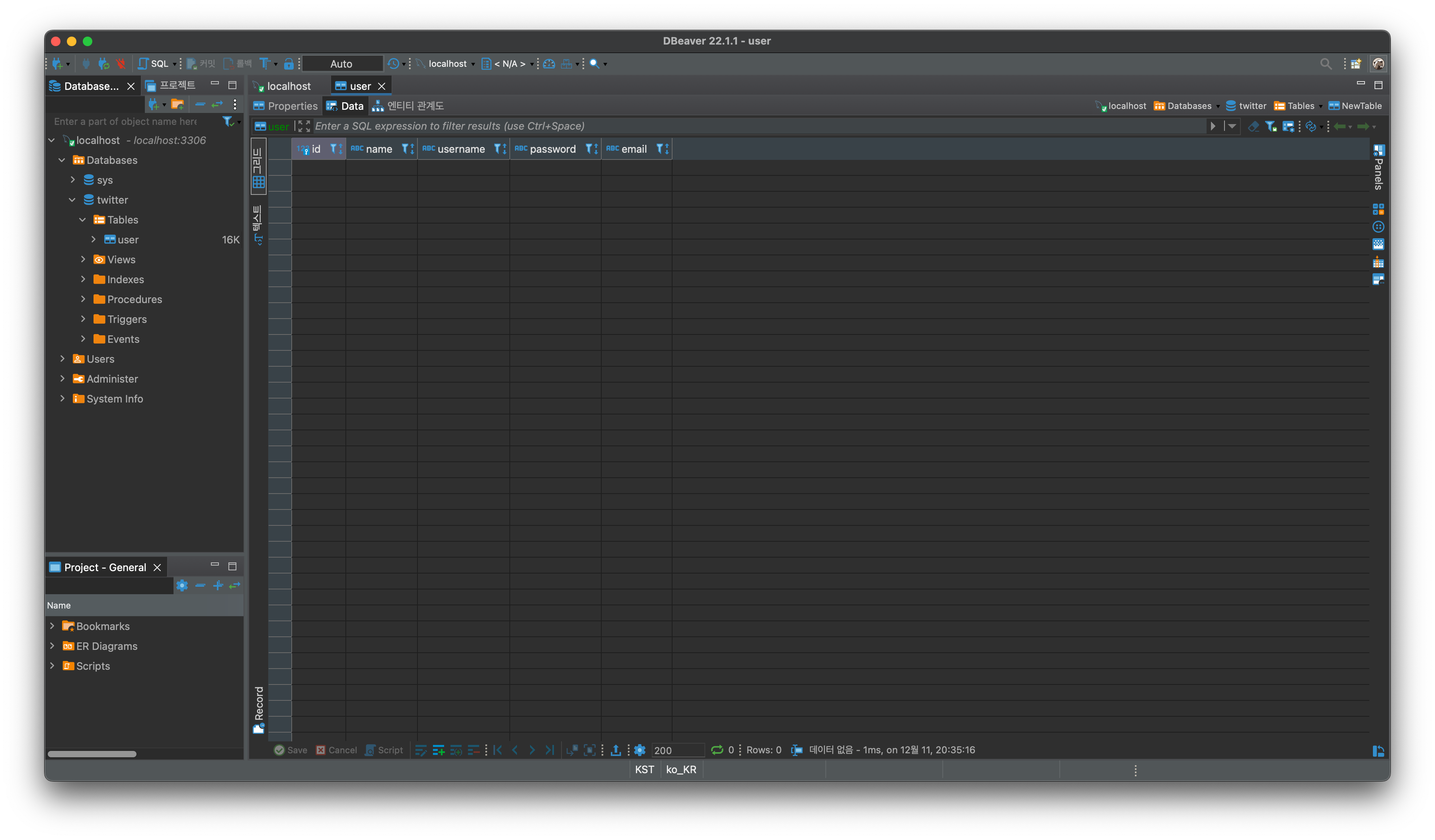
회원가입
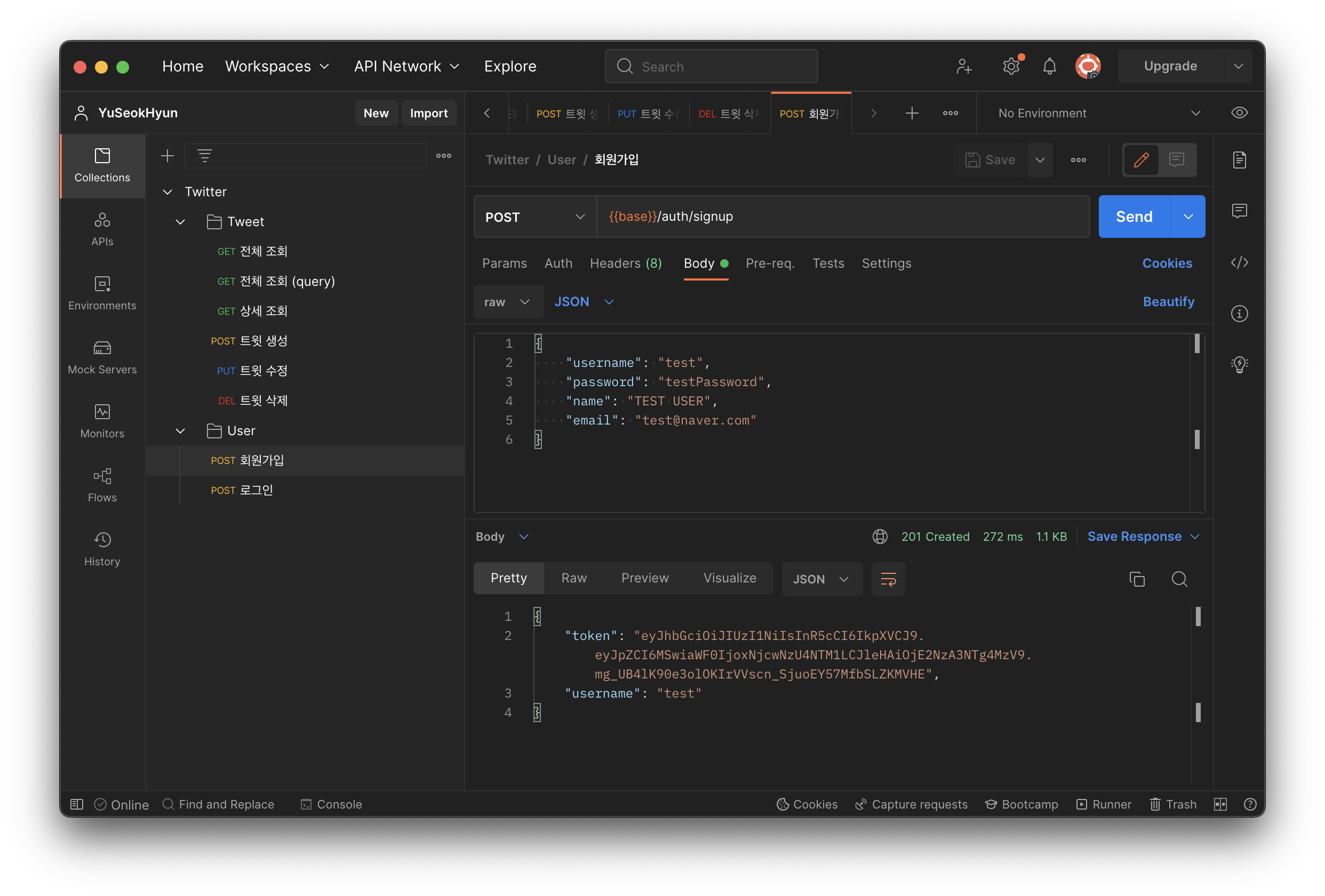
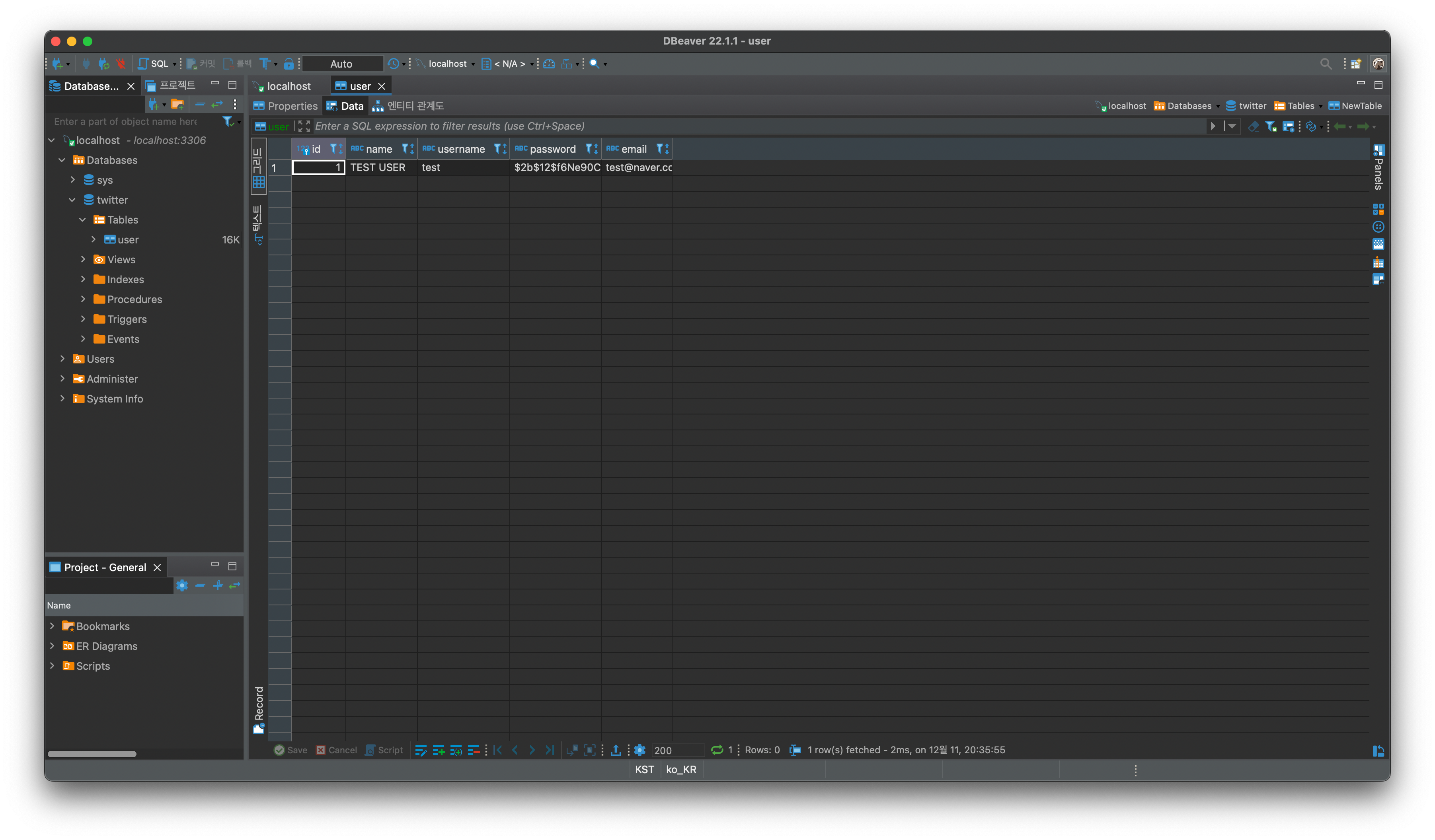
회원가입 (409)
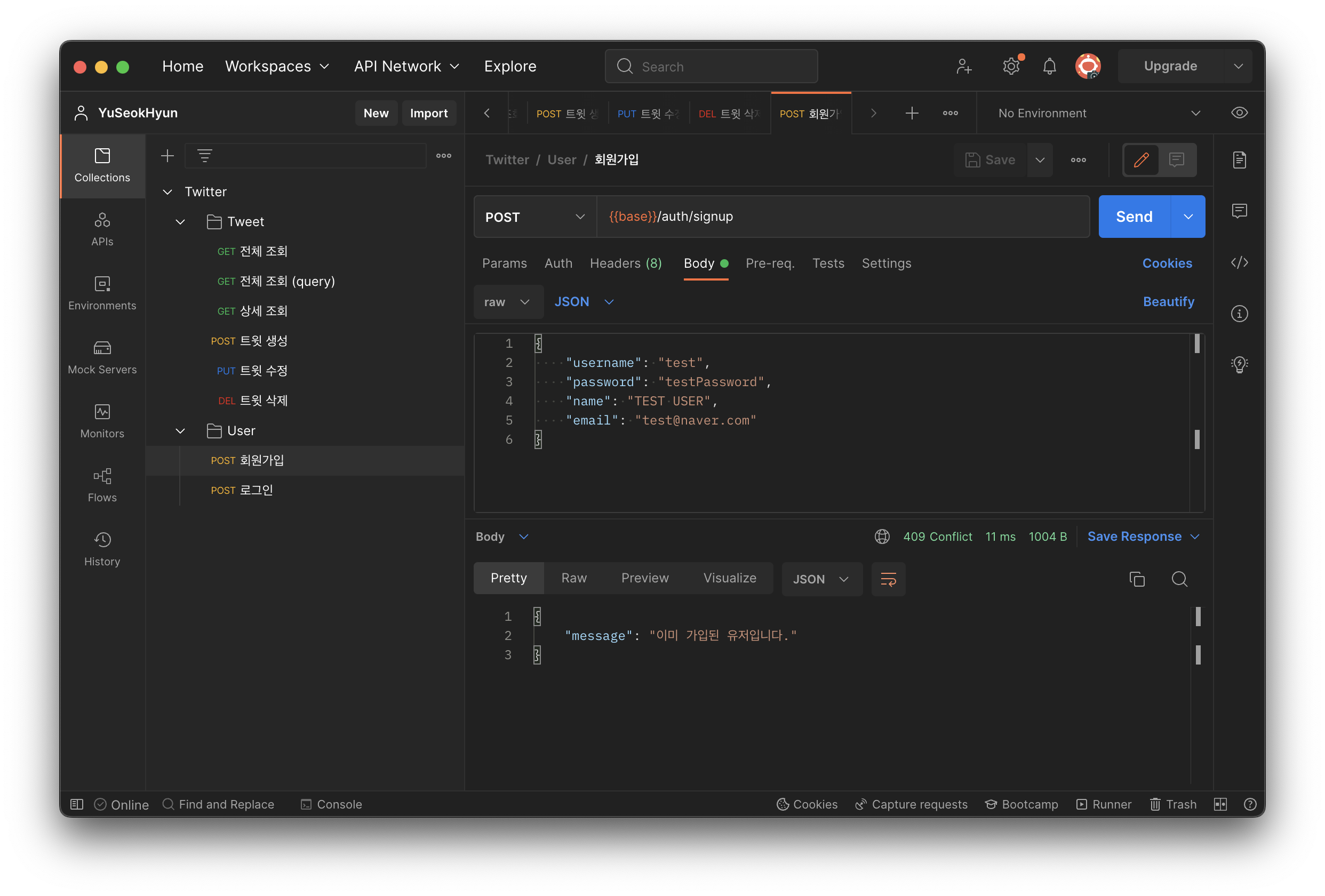