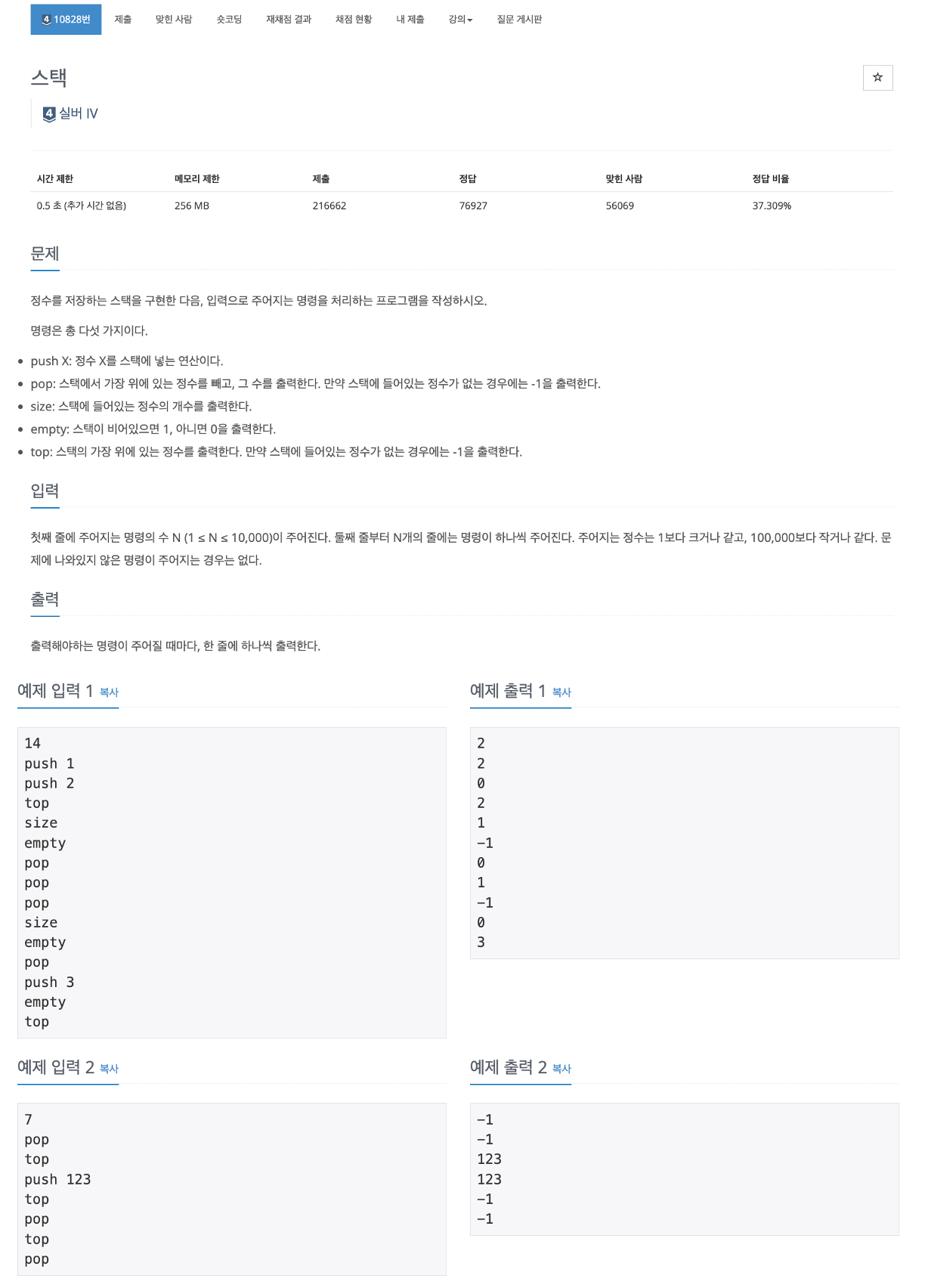
문제 해석
- 첫번째 줄에는 명령어의 수 N을 입력받고, 두번째 줄부터는 명령어를 한줄에 하나씩 주어진다.
- 명령어는 아래와 같다.
1. push X: 정수 X를 스택에 넣는 연산이다.
2. pop: 스택에서 가장 위에 있는 정수를 빼고, 그 수를 출력한다. 만약 스택에 들어있는 정수가 없는 경우에는 -1을 출력한다.
3. size: 스택에 들어있는 정수의 개수를 출력한다.
4. empty: 스택이 비어있으면 1, 아니면 0을 출력한다.
5. top: 스택의 가장 위에 있는 정수를 출력한다. 만약 스택에 들어있는 정수가 없는 경우에는 -1을 출력한다.
- push는 따로 출력할 필요가 없다. (정수 X를 넣기만 하면 된다.)
코드
import java.util.*;
import java.io.*;
public class Main {
static ArrayList<Integer> stack = new ArrayList<>();
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
BufferedWriter bw = new BufferedWriter(new OutputStreamWriter(System.out));
int N = Integer.parseInt(br.readLine());
for(int i = 0; i < N; i++){
String str = br.readLine();
if(str.contains(" ")){
StringTokenizer st = new StringTokenizer(str);
if(st.nextToken().equals("push")) {
push(Integer.parseInt(st.nextToken()));
}
}
if(str.equals("pop")){
bw.write(pop() + "\n");
}else if(str.equals("size")){
bw.write(size() + "\n");
}else if(str.equals("empty")){
bw.write(empty() + "\n");
}else if(str.equals("top")){
bw.write(top() + "\n");
}
}
br.close();
bw.flush();
bw.close();
}
static void push(int num){
stack.add(num);
}
static int pop(){
if(empty() == 1){
return -1;
}
int popNum = top();
stack.remove(stack.size()-1);
return popNum;
}
static int size(){
return stack.size();
}
static int empty(){
if(stack.size() <= 0){
return 1;
}
return 0;
}
static int top(){
if(empty() == 1){
return -1;
}
return stack.get(stack.size()-1);
}
}
- 코드에 대한 설명은 주석으로 자세히 작성해두었다.
결과

느낀 점
- 스택이라해서 조금 처음엔 겁먹었는데, 내 방식대로 쉽게 풀어 작성하니까 금방 풀었다.
- 대신 효율적인 코드인지는 잘 모르겠다..ㅎ