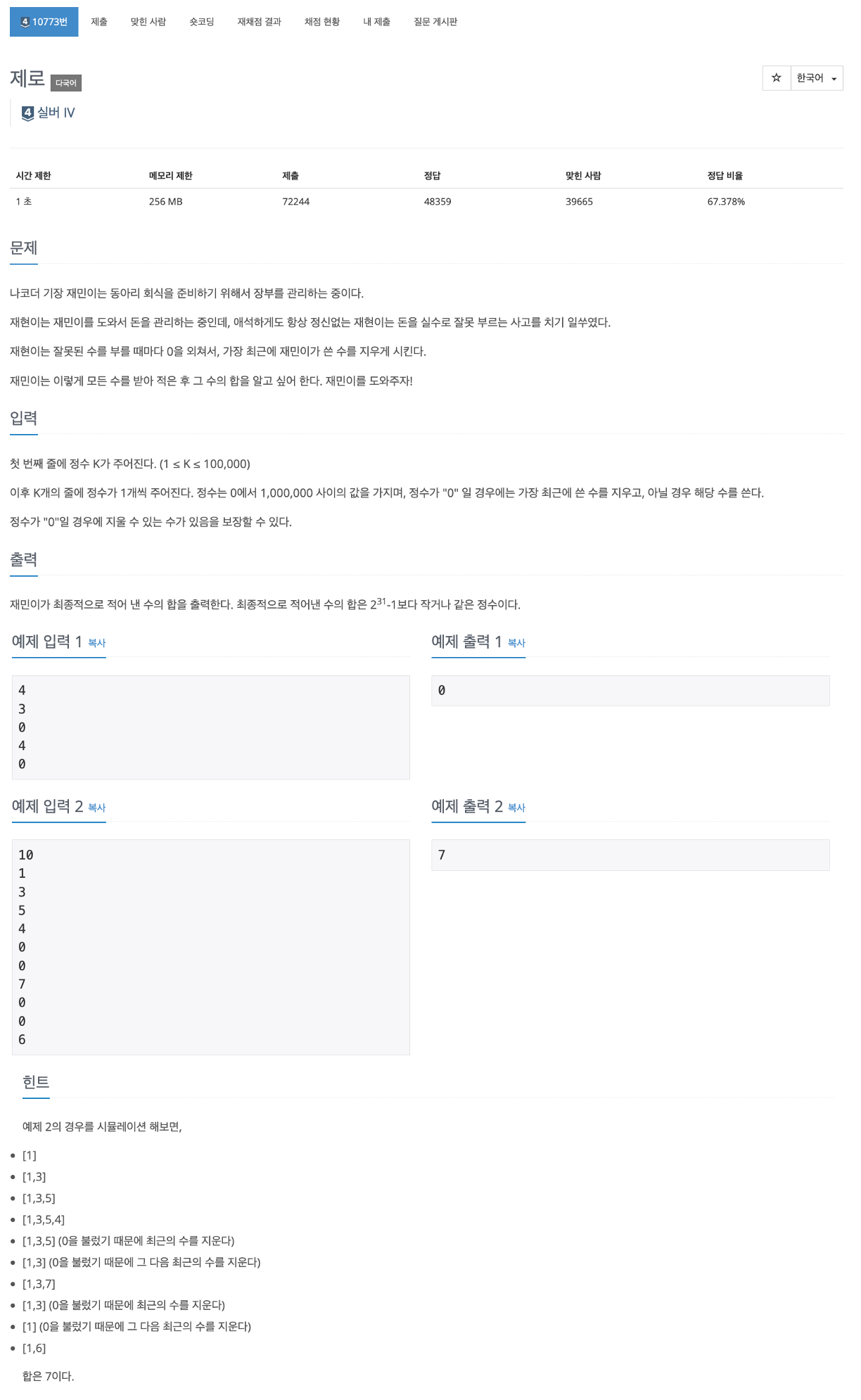
문제 해석
- 첫번째 줄에는 입력받을 테스트의 수 K을 입력받는다.
- K개 만큼 정수를 입력받는데 잘못된 수를 부를 때마다 0을 부르게 된다.
- 만약, 0을 부르면 가장 최근에 들어간 정수를 취소하면된다. (단, 취소할 정수가 하나도 없을 경우는 0을 추가하면 된다.)
- 이 문제를 읽어보면 push와, pop을 사용해야하는 문제임을 알 수 있다.
코드
import java.io.*;
public class Main {
static int size = 0;
static int[] stack;
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
BufferedWriter bw = new BufferedWriter(new OutputStreamWriter(System.out));
int K = Integer.parseInt(br.readLine());
int result = 0;
stack = new int[K];
for(int i = 0; i < K; i++){
int num = Integer.parseInt(br.readLine());
if(empty() == 1 && num == 0){
push(num);
}else if(num == 0){
result -= pop();
}else{
result += push(num);
}
}
br.close();
bw.write(result + "\n");
bw.flush();
bw.close();
}
static int push(int num){
stack[size] = num;
size++;
return num;
}
static int pop(){
int popNum = top();
stack[stack.length-1] = 0;
size--;
return popNum;
}
static int empty(){
if(stack.length <= 0){
return 1;
}
return 0;
}
static int top(){
if(empty() == 1){
return -1;
}
return stack[size-1];
}
}
- 전 포스트 10828 스택에선 ArrayList를 사용했는데, 이번에는 기본 배열을 사용해서 작성해 보았다.
- 코드에 대한 자세한 설명은 주석으로 작성해두었다.
결과

느낀 점
- 어제랑 거의 똑같은 문제여서 어려운 점이 없었다