리스트
- ArrayList
- LinkedList
- Vector
- Stack
✍️ ArrayList
✏️ ArrayList 란?
ArrayList
는 List
인터페이스를 구현한 클래스 중 하나로, 컬렉션 프레임웍에서 가장 많이 사용되는 컬렉션 클래스입니다.
- 데이터의 저장 순서가 유지되고, 중복을 허용합니다.
- 크기가 고정되어 있지 않으며, 필요에 따라 크기를 자동으로 조절합니다. 기존 배열의 크기는 10이며, 새로운 배열을 생성할 시 1.5배 확장됩니다.
- 인덱스를 통해 요소에 빠르게 접근할 수 있습니다.
- 중간에 요소를 추가하거나 삭제하는 작업에서는 ( 데이터가 연속적으로 리스트에 있어야 하며 중간에 빈공간이 있으면 안되어 ) 해당 위치 이후의 모든 요소를 이동해야 하므로 성능 저하가 발생할 수 있습니다.
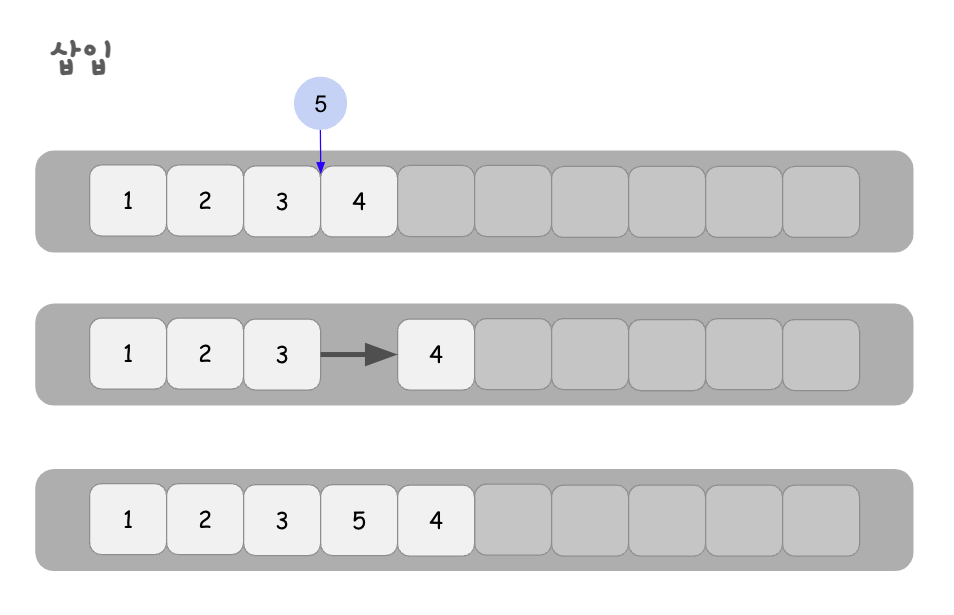
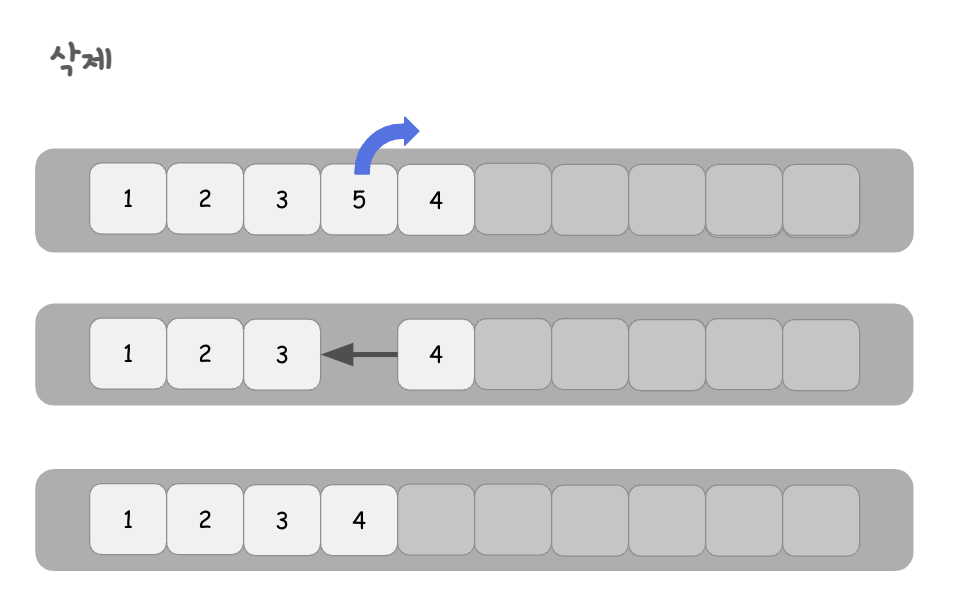
✏️ 선언
ArrayList arrayList = new ArrayList();
ArrayList<Integer> intArrayList = new ArrayList<Integer>();
ArrayList<Integer> intArrayList = new ArrayList<>();
- 1️⃣ : 타입 미설정 시, Object로 선언됩니다.
하지만 이렇게 선언할 경우 값을 추출하기 위해서는 캐스팅 (Casting) 연산이 필요하고
잘못된 타입으로 캐스팅 할 경우 에러가 발생하므로 추천하지 않습니다.
- 2️⃣ : 타입 설정 시, int 타입으로만 사용이 가능합니다.
- 3️⃣ :
new
에서 타입 파라미터는 생략이 가능합니다.
✏️ 생성
ArrayList<Integer> intArrayList1 = new ArrayList<>(15);
ArrayList<Integer> intArrayList2 = new ArrayList<>(intArrayList1);
- 1️⃣: 초기 용량 (capacity)를 15로 설정한
ArrayList
를 생성합니다.
- 2️⃣: 다른
ArrayList
의 데이터를 초기값으로 생성합니다.
✏️ 주요 메서드 및 시간 복잡도
🎨 1. boolean add(E element)
- 시간 복잡도: O(1) (평균적인 경우, 배열 크기를 늘리는 작업이 필요할 겅우 O(n)이 될 수도 있습니다.)
- 리스트에 요소를 추가합니다.
🎨 2. void add(int index, E element)
- 시간 복잡도: O(n) (기존에 있는 요소들을 한 칸씩 뒤로 밀어야 하기 때문에 시간이 걸립니다.)
- 지정된 인덱스에 요소를 추가합니다.
🎨 3. int size()
- 시간 복잡도: O(1)
- 리스트에 포함된 요소의 개수를 반환합니다.
🎨 4. boolean isEmpty()
- 시간 복잡도: O(1)
- 리스트가 비어 있는지 확인합니다.
🎨 5. boolean contains(Object o)
- 시간 복잡도: O(n)
- 리스트가 지정한 요소를 포함하고 있는지 여부를 반환합니다.
🎨 6. E get(int index)
- 시간 복잡도: O(1)
- 리스트에서 지정된 인덱스의 요소를 반환합니다.
🎨 7. E set(int index, E element)
- 시간 복잡도: O(1)
- 지정된 인덱스에 있는 요소를 주어진 요소로 대처하고, 이전 요소를 반환합니다.
🎨 8. E remove(int index)
- 시간 복잡도: O(n) (삭제 후에는 배열 요소들을 앞으로 한 칸씩 당겨야 하기 때문에 시간이 걸립니다.)
- 지정된 인덱스의 요소를 삭제하고 삭제된 요소를 반환합니다.
🎨 9. boolean remove(Object o)
- 시간 복잡도: O(n) (리스트를 순회하여 해당 요소를 찾고 삭제하는 과정에서 시간이 걸립니다.)
- 리스트에서 첫 번째로 등장하는 지정된 요소를 삭제합니다.
🎨 10. boolean removeAll(Object o)
- 시간 복잡도: O(n * m) (리스트를 순회하여 해당 요소를 찾고 삭제하는 과정에서 시간이 걸립니다. 여기서 n은 리스트의 크기이고, m은 컬렉션 c의 크기입니다.)
- 리스트에서 포함된 모든 요소들을 삭제합니다. 삭제된 요소가 하나라도 있으면 true를 반환합니다.
🎨 11. void clear()
- 시간 복잡도: O(n) (모든 요소를 순회하며 삭제하는 작업이 필요합니다.)
- 리스트의 모든 요소를 삭제합니다.
🎨 12. int indexOf(Object o)
- 시간 복잡도: O(n)
- 인자로 전달된 객체가 리스트에 존재한다면, 해당 객체 인덱스를 반환하고 없으면 -1을 반환합니다.
🎨 13. int lastIndexOf(Object o)
- 시간 복잡도: O(n)
- 리스트에서 지정한 요소가 마지막으로 나타나는 위치의 인덱스를 반환합니다. 요소가 리스트에 없으면 -1을 반환합니다.
🎨 14. Object[] toArray()
- 시간 복잡도: O(n)
- 리스트의 요소들을 배열로 반환합니다.
🎨 15 boolean equals(Object o)
- 시간 복잡도: O(n) (리스트 전체를 비교해야 하므로 시간이 걸립니다.)
- 지정된 객체가 이 리스트와 같은지 여부를 반환합니다.
🎨 16. int hashCode()
- 시간 복잡도: O(n) (리스트의 모든 요소를 순회하여 해시 코드를 계산하는 작업이 필요합니다.)
- 리스트의 해시 코드 값을 반환합니다.
✏️ 예시 코드
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
public class Main {
public static void main(String[] args) {
List<Integer> numList = new ArrayList<>();
List<Integer> numList2 = new ArrayList<>(Arrays.asList(2, 3));
List<Integer> numListCopy = new ArrayList<>();
numList.add(1);
numList.add(2);
numList.add(3);
numList.add(4);
numList.add(5);
System.out.println("numList: " + numList);
numList.add(2, 3);
numList.add(6, 2);
numList.add(6, 4);
System.out.println("numList: " + numList);
int listSize = numList.size();
System.out.println("리스트의 사이즈: " + listSize);
boolean isEmpty = numList.isEmpty();
System.out.println("리스트가 비어있는지? " + isEmpty);
boolean containsOne = numList.contains(1);
boolean containsSix = numList.contains(6);
System.out.println("리스트에 1이 포함되어 있는지? " + containsOne);
System.out.println("리스트에 6이 포함되어 있는지? " + containsSix);
int getValueAtIndex1 = numList.get(1);
System.out.println("인덱스 1의 값: " + getValueAtIndex1);
int previousValueAtIndex0 = numList.set(0, 10);
System.out.println("인덱스 0의 변경 이전 값: " + previousValueAtIndex0);
int removedElementAtIndex0 = numList.remove(0);
System.out.println("삭제되는 값: " + removedElementAtIndex0);
boolean removedFirstTwo = numList.remove(Integer.valueOf(2));
System.out.println("첫 번째로 나타나는 2 삭제 여부: " + removedFirstTwo + ", numList: " + numList);
boolean removedSix = numList.remove(Integer.valueOf(6));
System.out.println("6 삭제 여부: " + removedSix + ", numList: " + numList);
ArrayList<Integer> elementsToRemove = new ArrayList<>();
elementsToRemove.add(3);
boolean isRemoveAll = numList.removeAll(elementsToRemove);
System.out.println("3 전체 삭제 여부: " + isRemoveAll + ", numList: " + numList);
System.out.println("numList2 clear 이전: " + numList2);
numList2.clear();
System.out.println("numList2 clear 이후: " + numList2);
int indexOfTypeFour = numList.indexOf(4);
System.out.println("값이 4인 요소의 인덱스: " + indexOfTypeFour);
int indexOfTypeNine = numList.indexOf(9);
System.out.println("값이 9인 요소의 인덱스: " + indexOfTypeNine);
int lastIndexOfTypeFour = numList.lastIndexOf(4);
System.out.println("값이 4인 요소의 마지막 인덱스: " + lastIndexOfTypeFour);
int lastIndexOfTypeTen = numList.lastIndexOf(10);
System.out.println("값이 10인 요소의 마지막 인덱스: " + lastIndexOfTypeTen);
Integer[] intArray = numList.toArray(new Integer[numList.size()]);
System.out.println("배열로 변경: " + Arrays.toString(intArray));
numListCopy.addAll(numList);
boolean isEqual = numList.equals(numListCopy);
System.out.println("두 리스트가 같은지? " + isEqual);
int hashCode = numList.hashCode();
System.out.println("numList의 hashCode: " + hashCode);
}
}