📌 Java AWT
코드
import java.awt.Frame;
public class MyAwt {
public static void main(String[] args) {
Frame f = new Frame();
f.setSize(400, 400);
f.setVisible(true);
}
}
실행
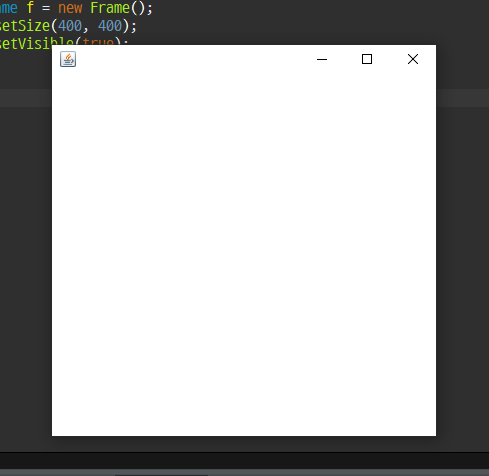
📌 Java Swing
코드
import java.awt.Button;
import java.awt.Panel;
import javax.swing.JFrame;
public class MySwing {
public static void main(String[] args) {
JFrame f = new JFrame();
f.setSize(400, 400);
f.setVisible(true);
Panel p = new Panel();
Button b1 = new Button();
Button b2 = new Button("출력");
Button b3 = new Button("정렬");
Button b4 = new Button("순위");
b1.setLabel("입력");
p.add(b1);
p.add(b2);
p.add(b3);
p.add(b4);
f.add(p);
f.setLocation(300,300);
f.setSize(300,100);
f.setVisible(true);
}
}
실행
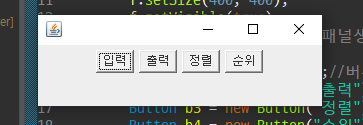
📌 Java FX
코드
import javafx.application.Application;
import javafx.fxml.FXMLLoader;
import javafx.scene.Parent;
import javafx.scene.Scene;
import javafx.stage.Stage;
public class Main extends Application {
@Override
public void start(Stage primaryStage) {
try {
Parent root = FXMLLoader.load(getClass().getResource("hello.fxml"));
Scene scene = new Scene(root,400,400);
scene.getStylesheets().add(getClass().getResource("application.css").toExternalForm());
primaryStage.setScene(scene);
primaryStage.show();
} catch(Exception e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
launch(args);
}
}
<?import javafx.scene.control.Button?>
<?import javafx.scene.control.Label?>
<?import javafx.scene.layout.AnchorPane?>
<?import javafx.scene.layout.VBox?>
<VBox prefHeight="400.0" prefWidth="640.0" xmlns="http://javafx.com/javafx/18" xmlns:fx="http://javafx.com/fxml/1">
<children>
<AnchorPane maxHeight="-1.0" maxWidth="-1.0" prefHeight="-1.0" prefWidth="-1.0" VBox.vgrow="ALWAYS">
<children>
<Label id="ldl" layoutX="117.0" layoutY="79.0" text="Good Morning" />
<Button layoutX="250.0" layoutY="75.0" mnemonicParsing="false" text="Click" />
</children>
</AnchorPane>
</children>
</VBox>
Scene Builder
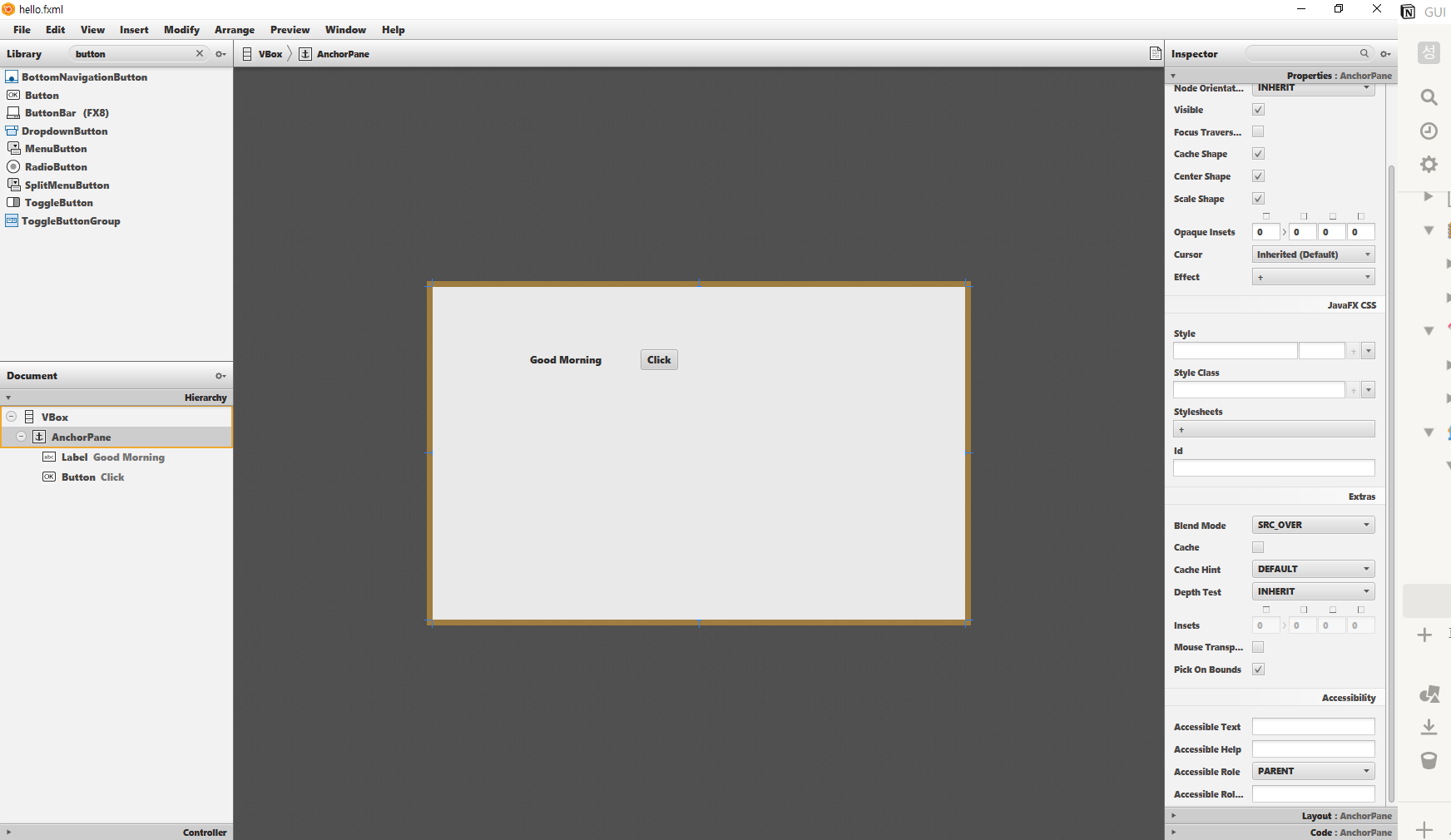
실행
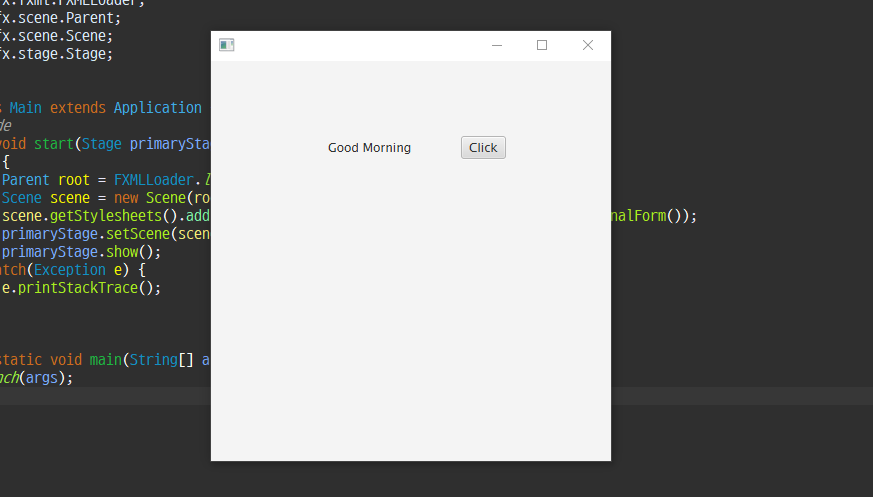
- java에서 fxml파일을 띄우기 위해서 root요소를 지정해줘야한다.
- 현재 fxml 파일에서 가장 상위 요소는 VBox이기 때문에 java에서 root를 VBox로 캐스팅 해줘야한다.
- Parent로 캐스팅 해줘도 된다.
FXML에 만든 요소 Java에서 사용하기
public class Main2 extends Application {
Parent root;
Scene scene;
Button btn;
TextField tf;
@Override
public void start(Stage primaryStage) {
try {
root = FXMLLoader.load(getClass().getResource("main2.fxml"));
scene = new Scene(root, 400, 400);
btn = (Button)scene.lookup("#btn");
tf = (TextField)scene.lookup("#tf");
btn.setOnMouseClicked(new EventHandler<Event>() {
@Override
public void handle(Event event) {
plusOne();
}
});
scene.getStylesheets().add(getClass().getResource("application.css").toExternalForm());
primaryStage.setScene(scene);
primaryStage.show();
} catch (Exception e) {
e.printStackTrace();
}
}
public void plusOne() {
int n = Integer.parseInt(tf.getText());
String txt = String.valueOf(++n);
tf.setText(txt);
}
public static void main(String[] args) {
launch(args);
}
}btn = (Button)scene.lookup("#btn");
tf = (TextField)scene.lookup("#tf");
요소를 가져오는 메소드 : lookup
btn = (Button)scene.lookup("#btn");
tf = (TextField)scene.lookup("#tf");
- lookup(”#id값”)형태로 불러올 수 있다.
- id 는 fxml에서 요소안에 id=”id값”의 형태로 선언해야한다.
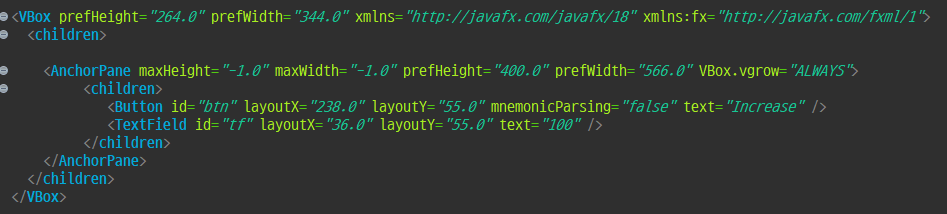
이벤트를 등록하는 클래스 : EventHandler
btn = (Button)scene.lookup("#btn");
btn.setOnMouseClicked(new EventHandler<Event>() {
@Override
public void handle(Event event) {
plusOne();
}
});
public void plusOne() {
int n = Integer.parseInt(tf.getText());
String txt = String.valueOf(++n);
tf.setText(txt);
}
- 선언한 요소에 다양한 이벤트를 등록할 수 있다.
- 원하는 이벤트메소드를 사용하고 익명객체로 EventHandler의 handle메소드를 오버라이드 해줘야한다.
📌 예제
1) 덧셈 출력
자바코드
public class Main3 extends Application {
Parent root;
Scene scene;
Button btn;
TextField tf1;
TextField tf2;
TextField tf3;
@Override
public void start(Stage primaryStage) {
try {
root = FXMLLoader.load(getClass().getResource("main3.fxml"));
scene = new Scene(root, 800, 400);
btn = (Button)scene.lookup("#btn");
tf1 = (TextField)scene.lookup("#tf1");
tf2 = (TextField)scene.lookup("#tf2");
tf3 = (TextField)scene.lookup("#tf3");
btn.setOnMouseClicked(new EventHandler<Event>() {
@Override
public void handle(Event event) {
sum();
}
});
scene.getStylesheets().add(getClass().getResource("application.css").toExternalForm());
primaryStage.setScene(scene);
primaryStage.show();
} catch (Exception e) {
e.printStackTrace();
}
}
public void sum() {
long n1 = Long.parseLong(tf1.getText());
long n2 = Long.parseLong(tf2.getText());
String txt = String.valueOf(n1 + n2);
tf3.setText(txt);
}
public static void main(String[] args) {
launch(args);
}
}
fxml 태그
<VBox prefHeight="307.0" prefWidth="632.0" xmlns="http://javafx.com/javafx/18" xmlns:fx="http://javafx.com/fxml/1">
<children>
<AnchorPane maxHeight="-1.0" maxWidth="-1.0" prefHeight="212.0" prefWidth="632.0" VBox.vgrow="ALWAYS">
<children>
<TextField id="tf1" layoutX="42.0" layoutY="126.0" prefHeight="25.0" prefWidth="115.0" />
<TextField id="tf2" layoutX="251.0" layoutY="126.0" prefHeight="25.0" prefWidth="115.0" />
<TextField id="tf3" layoutX="461.0" layoutY="126.0" prefHeight="25.0" prefWidth="115.0" />
<Button id="btn" layoutX="401.0" layoutY="126.0" mnemonicParsing="false" text="=" />
<Label layoutX="193.0" layoutY="124.0" prefHeight="30.0" prefWidth="15.0" text="+">
<font>
<Font size="20.0" />
</font>
</Label>
</children>
</AnchorPane>
</children>
</VBox>
결과
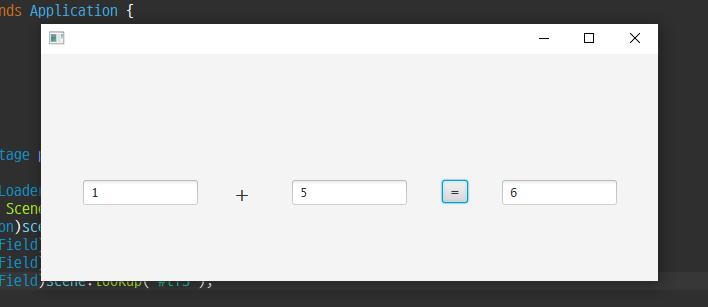
2) 구구단 출력
자바코드
public class Main4 extends Application {
Parent root;
Scene scene;
Button btn;
TextField tf;
TextArea ta;
@Override
public void start(Stage primaryStage) {
try {
root = FXMLLoader.load(getClass().getResource("main4.fxml"));
scene = new Scene(root, 250, 350);
btn = (Button) scene.lookup("#btn");
tf = (TextField) scene.lookup("#tf");
ta = (TextArea) scene.lookup("#ta");
btn.setOnMouseClicked(new EventHandler<Event>() {
@Override
public void handle(Event event) {
multiple();
}
});
primaryStage.setScene(scene);
primaryStage.show();
} catch (Exception e) {
e.printStackTrace();
}
}
public void multiple() {
int n = Integer.parseInt(tf.getText());
String txt = "";
for (int i = 1; i < 10; i++) {
txt += (n + " * " + i + " =" + n * i + "\n");
}
ta.setText(txt);
}
public static void main(String[] args) {
launch(args);
}
}
fxml 태그
<VBox prefHeight="315.0" prefWidth="245.0" xmlns="http://javafx.com/javafx/18" xmlns:fx="http://javafx.com/fxml/1">
<children>
<AnchorPane maxHeight="-1.0" maxWidth="-1.0" prefHeight="-1.0" prefWidth="-1.0" VBox.vgrow="ALWAYS">
<children>
<TextField id="tf" layoutX="110.0" layoutY="23.0" prefHeight="22.0" prefWidth="80.0" />
<Label layoutX="31.0" layoutY="27.0" text="단수 :" />
<Button id="btn" layoutX="34.0" layoutY="54.0" mnemonicParsing="false" prefHeight="22.0" prefWidth="155.0" text="출력하기" />
<TextArea id="ta" layoutX="31.0" layoutY="86.0" prefHeight="200.0" prefWidth="162.0" />
</children>
</AnchorPane>
</children>
</VBox>
결과
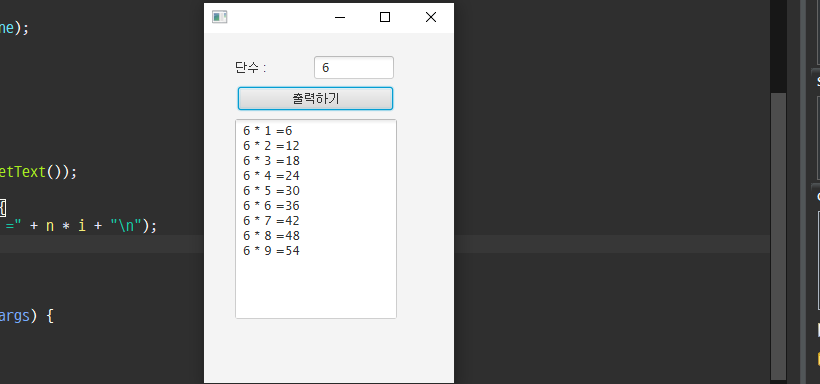