📌 홀/짝게임
코드
public class Main5 extends Application {
Parent root;
Scene scene;
Button btn;
TextField tf_user;
TextField tf_com;
TextField tf_result;
@Override
public void start(Stage primaryStage) {
try {
root = FXMLLoader.load(getClass().getResource("main5.fxml"));
scene = new Scene(root, 250, 350);
btn = (Button) scene.lookup("#btn");
tf_user = (TextField) scene.lookup("#tf_user");
tf_com = (TextField) scene.lookup("#tf_com");
tf_result = (TextField) scene.lookup("#tf_result");
btn.setOnMouseClicked(new EventHandler<Event>() {
@Override
public void handle(Event event) {
EvenOdd();
}
});
primaryStage.setScene(scene);
primaryStage.show();
} catch (Exception e) {
e.printStackTrace();
}
}
public void EvenOdd() {
String user = tf_user.getText();
String com = ComRandom();
String result ="";
tf_com.setText(com);
if(user.equals(com)) {
result = "User 승리";
}else {
result = "COM 승리";
}
tf_result.setText(result);
}
public String ComRandom() {
String com = "";
Random rnd = new Random();
int n = rnd.nextInt(2);
if(n == 1) {
com = "홀";
}else {
com = "짝";
}
return com;
}
public static void main(String[] args) {
launch(args);
- 유저와 컴퓨터의 홀 짝을 비교하는 게임
- 컴퓨터의 홀,짝을 생성하기 위해 ComRandom메소드를 생성
- 유저와 컴퓨터의 홀,짝을 비교하기 위해 EvenOdd메소드 생성
fxml 구조
<VBox prefHeight="315.0" prefWidth="245.0" xmlns="http://javafx.com/javafx/18" xmlns:fx="http://javafx.com/fxml/1">
<children>
<AnchorPane maxHeight="-1.0" maxWidth="-1.0" prefHeight="-1.0" prefWidth="-1.0" VBox.vgrow="ALWAYS">
<children>
<Label layoutX="26.0" layoutY="89.0" text="User" />
<Label layoutX="25.0" layoutY="135.0" text="COM" />
<Label layoutX="24.0" layoutY="185.0" text="Result" />
<TextField id="tf_user" layoutX="94.0" layoutY="85.0" prefHeight="25.0" prefWidth="125.0" promptText="홀/짝" />
<TextField id="tf_com" layoutX="94.0" layoutY="131.0" prefHeight="25.0" prefWidth="125.0" />
<TextField id="tf_result" layoutX="94.0" layoutY="181.0" prefHeight="25.0" prefWidth="125.0" />
<Button id="btn" layoutX="27.0" layoutY="244.0" mnemonicParsing="false" prefHeight="25.0" prefWidth="192.0" text="Play" />
<Label layoutX="72.0" layoutY="14.0" prefHeight="47.0" prefWidth="103.0" text="홀/짝 게임" textAlignment="CENTER">
<font>
<Font size="21.0" />
</font>
</Label>
</children>
</AnchorPane>
</children>
</VBox>
실행
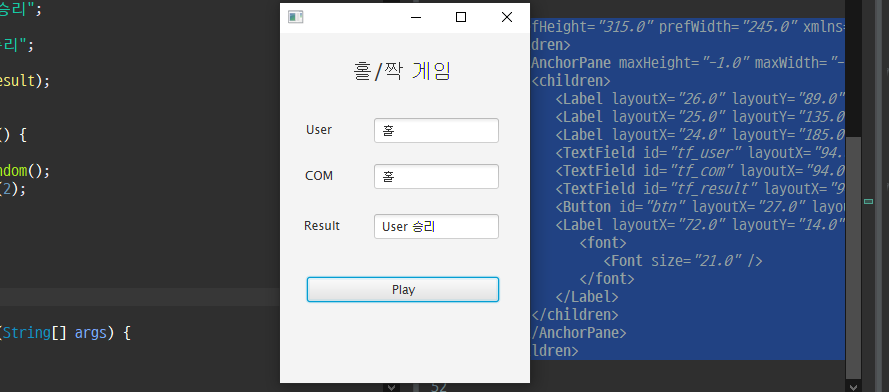
📌 로또번호 생성
코드
public class Main6 extends Application {
Parent root;
Scene scene;
Button btn;
Label lbl1;
Label lbl2;
Label lbl3;
Label lbl4;
Label lbl5;
Label lbl6;
@Override
public void start(Stage primaryStage) {
try {
root = FXMLLoader.load(getClass().getResource("main6.fxml"));
scene = new Scene(root, 250, 250);
btn = (Button) scene.lookup("#btn");
lbl1 = (Label) scene.lookup("#lbl1");
lbl2 = (Label) scene.lookup("#lbl2");
lbl3 = (Label) scene.lookup("#lbl3");
lbl4 = (Label) scene.lookup("#lbl4");
lbl5 = (Label) scene.lookup("#lbl5");
lbl6 = (Label) scene.lookup("#lbl6");
btn.setOnMouseClicked(new EventHandler<Event>() {
@Override
public void handle(Event event) {
getLotto();
}
});
primaryStage.setScene(scene);
primaryStage.show();
} catch (Exception e) {
e.printStackTrace();
}
}
public void getLotto() {
Random random = new Random();
int[] lottoNum = new int[6];
int temp;
for (int i = 0; i < lottoNum.length; i++) {
lottoNum[i] = random.nextInt(45) + 1;
for (int j = 0; j < i; j++) {
if (lottoNum[i] == lottoNum[j]) {
i--;
break;
}
}
}
for (int i = 0; i < lottoNum.length; i++) {
for (int j = 0; j < i; j++) {
if (lottoNum[i] < lottoNum[j]) {
temp = lottoNum[i];
lottoNum[i] = lottoNum[j];
lottoNum[j] = temp;
}
}
}
lbl1.setText(String.valueOf(lottoNum[0]));
lbl2.setText(String.valueOf(lottoNum[1]));
lbl3.setText(String.valueOf(lottoNum[2]));
lbl4.setText(String.valueOf(lottoNum[3]));
lbl5.setText(String.valueOf(lottoNum[4]));
lbl6.setText(String.valueOf(lottoNum[5]));
}
public static void main(String[] args) {
launch(args);
}
}
fxml 구조
<VBox prefHeight="184.0" prefWidth="245.0" xmlns="http://javafx.com/javafx/18" xmlns:fx="http://javafx.com/fxml/1">
<children>
<AnchorPane maxHeight="-1.0" maxWidth="-1.0" prefHeight="246.0" prefWidth="245.0" VBox.vgrow="ALWAYS">
<children>
<Label id="lbl1" layoutX="26.0" layoutY="75.0" text="__" />
<Label id="lbl2" layoutX="62.0" layoutY="75.0" text="__" />
<Label id="lbl3" layoutX="94.0" layoutY="75.0" text="__" />
<Label id="lbl4" layoutX="131.0" layoutY="75.0" text="__" />
<Label id="lbl5" layoutX="170.0" layoutY="75.0" text="__" />
<Label id="lbl6" layoutX="200.0" layoutY="75.0" text="__" />
<Button id="btn" layoutX="18.0" layoutY="117.0" mnemonicParsing="false" prefHeight="25.0" prefWidth="209.0" text="Lotto 생성" />
</children>
</AnchorPane>
</children>
</VBox>
실행
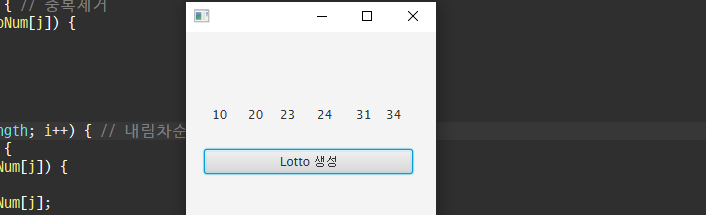
📌 가위바위보
코드
public class Main7 extends Application {
Parent root;
Scene scene;
Button btn;
TextField tfUser;
TextField tfCom;
TextField tfResult;
@Override
public void start(Stage primaryStage) {
try {
root = FXMLLoader.load(getClass().getResource("main7.fxml"));
scene = new Scene(root, 250, 350);
btn = (Button) scene.lookup("#playButton");
tfUser = (TextField) scene.lookup("#tfUser");
tfCom = (TextField) scene.lookup("#tfCom");
tfResult = (TextField) scene.lookup("#tfResult");
btn.setOnMouseClicked(new EventHandler<Event>() {
@Override
public void handle(Event event) {
RSP();
}
});
primaryStage.setScene(scene);
primaryStage.show();
} catch (Exception e) {
e.printStackTrace();
}
}
public void RSP() {
String user = tfUser.getText();
String com = ComRandom();
String result = "";
tfCom.setText(com);
if (user.equals(com)) {
result = "비김";
} else if (user.equals("가위") && com.equals("보")
|| user.equals("바위") && com.equals("가위")
|| user.equals("보") && com.equals("바위")) {
result = "USER 승리";
} else {
result = "COM 승리";
}
tfResult.setText(result);
}
public String ComRandom() {
String com = "";
Random rnd = new Random();
int n = rnd.nextInt(3);
if (n == 0) {
com = "가위";
} else if (n == 1) {
com = "바위";
} else {
com = "보";
}
return com;
}
public static void main(String[] args) {
launch(args);
}
}
fxml 구조
<VBox prefHeight="315.0" prefWidth="245.0" xmlns="http://javafx.com/javafx/18" xmlns:fx="http://javafx.com/fxml/1">
<children>
<AnchorPane maxHeight="-1.0" maxWidth="-1.0" prefHeight="-1.0" prefWidth="-1.0" VBox.vgrow="ALWAYS">
<children>
<Label layoutX="26.0" layoutY="89.0" text="User" />
<Label layoutX="25.0" layoutY="135.0" text="COM" />
<Label layoutX="24.0" layoutY="185.0" text="Result" />
<TextField id="tfUser" layoutX="94.0" layoutY="85.0" prefHeight="25.0" prefWidth="125.0" promptText="가위/바위/보" />
<TextField id="tfCom" layoutX="94.0" layoutY="131.0" prefHeight="25.0" prefWidth="125.0" />
<TextField id="tfResult" layoutX="94.0" layoutY="181.0" prefHeight="25.0" prefWidth="125.0" />
<Button id="playButton" layoutX="27.0" layoutY="244.0" mnemonicParsing="false" prefHeight="25.0" prefWidth="192.0" text="Play" />
<Label layoutX="67.0" layoutY="14.0" prefHeight="47.0" prefWidth="111.0" text="가위바위보" textAlignment="CENTER">
<font>
<Font size="21.0" />
</font>
</Label>
</children>
</AnchorPane>
</children>
</VBox>
실행
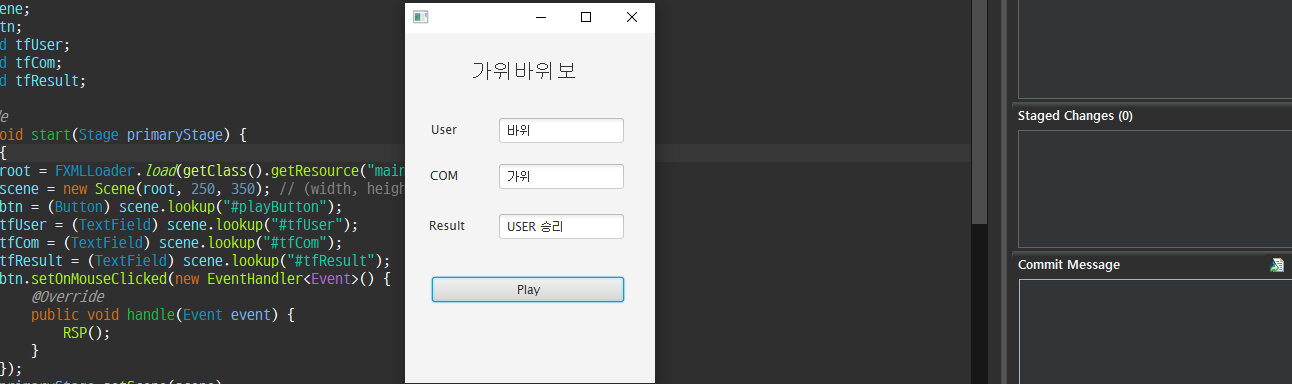
📌 별찍기
코드
public class Main8 extends Application {
Parent root;
Scene scene;
Button btn;
TextField tf1;
TextField tf2;
TextArea ta;
@Override
public void start(Stage primaryStage) {
try {
root = FXMLLoader.load(getClass().getResource("main8.fxml"));
scene = new Scene(root, 400, 600);
btn = (Button) scene.lookup("#btn");
tf1 = (TextField) scene.lookup("#tf1");
tf2 = (TextField) scene.lookup("#tf2");
ta = (TextArea) scene.lookup("#ta");
btn.setOnMouseClicked(new EventHandler<Event>() {
@Override
public void handle(Event event) {
shiningStar();
}
});
primaryStage.setScene(scene);
primaryStage.show();
} catch (Exception e) {
e.printStackTrace();
}
}
public void shiningStar() {
int n1 = Integer.parseInt(tf1.getText());
int n2 = Integer.parseInt(tf2.getText());
String result = drawStar(n1, n2);
ta.setText(result);
}
public String drawStar(int n1, int n2) {
String result = "";
if (n1 < n2) {
for (int i = n1; i <= n2; i++) {
for (int j = 1; j <= i; j++) {
result += "*";
}
result += "\n";
}
} else if (n1 > n2) {
for (int i = 1; i <= n1 - n2 + 1; i++) {
for (int j = n1; j >= i; j--) {
result += "*";
}
result += "\n";
}
}
return result;
}
public static void main(String[] args) {
launch(args);
}
}
fxml 구조
<VBox prefHeight="470.0" prefWidth="370.0" xmlns="http://javafx.com/javafx/18" xmlns:fx="http://javafx.com/fxml/1">
<children>
<AnchorPane maxHeight="-1.0" maxWidth="-1.0" prefHeight="441.0" prefWidth="469.0" VBox.vgrow="ALWAYS">
<children>
<Label layoutX="60.0" layoutY="72.0" text="첫 별 수" />
<Label layoutX="60.0" layoutY="112.0" text="끝 별 수" />
<TextField id="tf1" layoutX="135.0" layoutY="68.0" />
<TextField id="tf2" layoutX="135.0" layoutY="108.0" />
<Button id="btn" layoutX="64.0" layoutY="156.0" mnemonicParsing="false" prefHeight="25.0" prefWidth="238.0" text="별빛이 내린다" />
<TextArea id="ta" layoutX="64.0" layoutY="208.0" prefHeight="234.0" prefWidth="238.0" />
<Label layoutX="60.0" layoutY="8.0" prefHeight="41.0" prefWidth="238.0" text="Shining Star~">
<font>
<Font size="21.0" />
</font>
</Label>
</children>
</AnchorPane>
</children>
</VBox>
실행 (1)
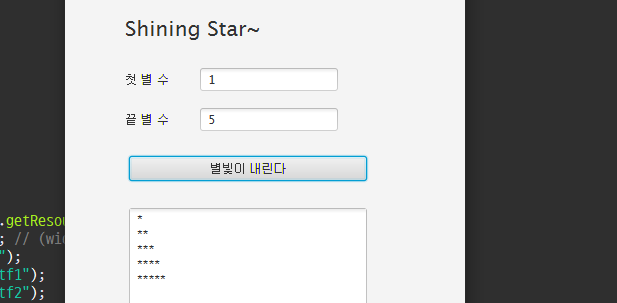
실행(2)
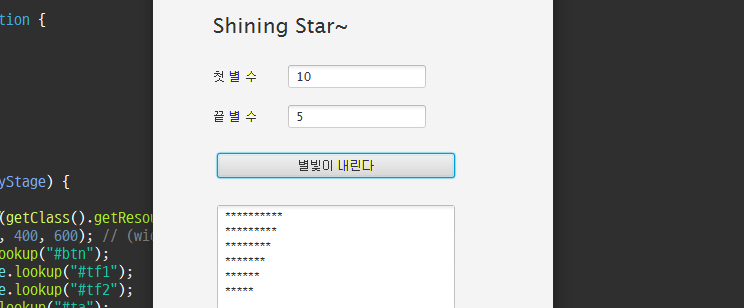
📌 전화기
코드
public class Main9 extends Application {
Parent root;
Scene scene;
Button btn1;
Button btn2;
Button btn3;
Button btn4;
Button btn5;
Button btn6;
Button btn7;
Button btn8;
Button btn9;
Button btn0;
Button call;
TextField tf;
@Override
public void start(Stage primaryStage) {
try {
root = FXMLLoader.load(getClass().getResource("main9.fxml"));
scene = new Scene(root, 300, 500);
btn1 = (Button) scene.lookup("#btn1");
btn2 = (Button) scene.lookup("#btn2");
btn3 = (Button) scene.lookup("#btn3");
btn4 = (Button) scene.lookup("#btn4");
btn5 = (Button) scene.lookup("#btn5");
btn6 = (Button) scene.lookup("#btn6");
btn7 = (Button) scene.lookup("#btn7");
btn8 = (Button) scene.lookup("#btn8");
btn9 = (Button) scene.lookup("#btn9");
btn0 = (Button) scene.lookup("#btn0");
call = (Button) scene.lookup("#btnCall");
tf = (TextField) scene.lookup("#tf");
btn1.setOnMouseClicked(new EventHandler<Event>() {public void handle(Event event) {input(event);}});
btn2.setOnMouseClicked(new EventHandler<Event>() {public void handle(Event event) {input(event);}});
btn3.setOnMouseClicked(new EventHandler<Event>() {public void handle(Event event) {input(event);}});
btn4.setOnMouseClicked(new EventHandler<Event>() {public void handle(Event event) {input(event);}});
btn5.setOnMouseClicked(new EventHandler<Event>() {public void handle(Event event) {input(event);}});
btn6.setOnMouseClicked(new EventHandler<Event>() {public void handle(Event event) {input(event);}});
btn7.setOnMouseClicked(new EventHandler<Event>() {public void handle(Event event) {input(event);}});
btn8.setOnMouseClicked(new EventHandler<Event>() {public void handle(Event event) {input(event);}});
btn9.setOnMouseClicked(new EventHandler<Event>() {public void handle(Event event) {input(event);}});
btn0.setOnMouseClicked(new EventHandler<Event>() {public void handle(Event event) {input(event);}});
call.setOnMouseClicked(new EventHandler<Event>() {public void handle(Event event) {calling();}});
primaryStage.setScene(scene);
primaryStage.show();
} catch (Exception e) {
e.printStackTrace();
}
}
public void input(Event event){
Button temp = (Button) event.getSource();
tf.appendText(temp.getText());
}
public void calling() {
Alert alert = new Alert(AlertType.INFORMATION);
alert.setTitle("Calling...");
alert.setHeaderText("Calling to " + tf.getText());
alert.setContentText("Please Wait to Connect");
alert.show();
}
public static void main(String[] args) {
launch(args);
}
}
fxml 구조
<VBox prefHeight="363.0" prefWidth="291.0" xmlns="http://javafx.com/javafx/18" xmlns:fx="http://javafx.com/fxml/1">
<children>
<AnchorPane maxHeight="-1.0" maxWidth="-1.0" prefHeight="441.0" prefWidth="469.0" VBox.vgrow="ALWAYS">
<children>
<Button id="btn1" layoutX="48.0" layoutY="84.0" mnemonicParsing="false" prefHeight="47.0" prefWidth="55.0" text="1" />
<TextField id="tf" alignment="CENTER_RIGHT" layoutX="48.0" layoutY="36.0" prefHeight="25.0" prefWidth="197.0" />
<Button id="btn2" layoutX="121.0" layoutY="84.0" mnemonicParsing="false" prefHeight="47.0" prefWidth="55.0" text="2" />
<Button id="btn3" layoutX="193.0" layoutY="84.0" mnemonicParsing="false" prefHeight="47.0" prefWidth="55.0" text="3" />
<Button id="btn4" layoutX="48.0" layoutY="145.0" mnemonicParsing="false" prefHeight="47.0" prefWidth="55.0" text="4" />
<Button id="btn7" layoutX="48.0" layoutY="205.0" mnemonicParsing="false" prefHeight="47.0" prefWidth="55.0" text="7" />
<Button id="btn5" layoutX="121.0" layoutY="145.0" mnemonicParsing="false" prefHeight="47.0" prefWidth="55.0" text="5" />
<Button id="btn6" layoutX="193.0" layoutY="145.0" mnemonicParsing="false" prefHeight="47.0" prefWidth="55.0" text="6" />
<Button id="btn8" layoutX="121.0" layoutY="205.0" mnemonicParsing="false" prefHeight="47.0" prefWidth="55.0" text="8" />
<Button id="btn9" layoutX="193.0" layoutY="205.0" mnemonicParsing="false" prefHeight="47.0" prefWidth="55.0" text="9" />
<Button id="btn0" layoutX="48.0" layoutY="265.0" mnemonicParsing="false" prefHeight="47.0" prefWidth="55.0" text="0" />
<BorderPane layoutX="108.0" layoutY="263.0" prefHeight="54.0" prefWidth="153.0">
<center>
<Button id="btnCall" mnemonicParsing="false" prefHeight="47.0" prefWidth="118.0" text="CALL" BorderPane.alignment="CENTER" />
</center>
</BorderPane>
</children>
</AnchorPane>
</children>
</VBox>
- Event 객체를 이용하면 내가 실행한 이벤트가 일어난 요소가 무엇인지 반환받을 수 있다.
public void input(Event event){
Button temp = (Button) event.getSource();
tf.appendText(temp.getText());
}
- Event객체의 event.getSource() 를 하면 Button이 리턴된다.
- 리턴된 값을 Button으로 캐스팅한뒤 Button객체에 담으면 내가 누른 버튼이 변수에 담긴다.
- 그 버튼을 getText()하여 텍스트 값을 가져올 수 있다.
Alert
public void calling() {
Alert alert = new Alert(AlertType.INFORMATION);
alert.setTitle("Calling...");
alert.setHeaderText("Calling to " + tf.getText());
alert.setContentText("Please Wait to Connect");
alert.show();
}
- Alert를 사용하면 팝업창을 띄울 수 있다.
- AlertType으로 타입을 정해줄 수 있다.(ERROR, INFORMATION, NONE 등)
- NONE으로 설정할 경우 팝업창을 끌 수 없으므로 주의.
실행
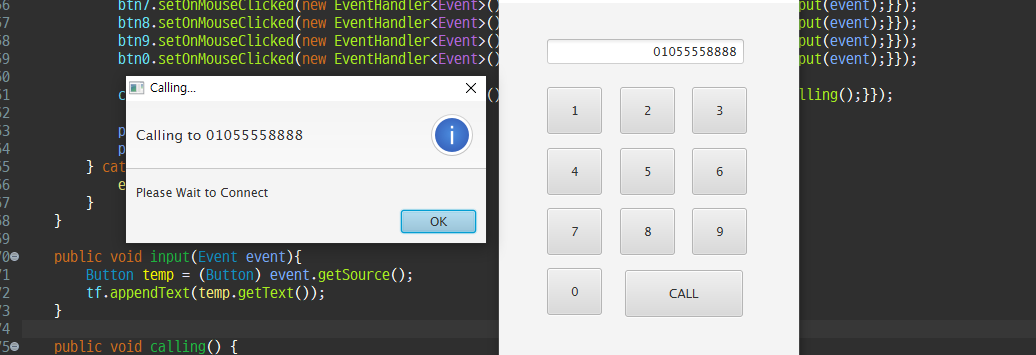
- 번호를 입력하고 CALL을 누르면 알림팝업이 뜬다.
📌 배수의 합
코드
public class MainA extends Application {
Parent root;
Scene scene;
Button btn;
TextField tf1;
TextField tf2;
TextField tf3;
TextField tf4;
@Override
public void start(Stage primaryStage) {
try {
root = FXMLLoader.load(getClass().getResource("mainA.fxml"));
scene = new Scene(root, 800, 150);
btn = (Button) scene.lookup("#btn");
tf1 = (TextField) scene.lookup("#tf1");
tf2 = (TextField) scene.lookup("#tf2");
tf3 = (TextField) scene.lookup("#tf3");
tf4 = (TextField) scene.lookup("#tf4");
btn.setOnMouseClicked(new EventHandler<Event>() {
public void handle(Event event) {
calc();
}
});
primaryStage.setScene(scene);
primaryStage.show();
} catch (Exception e) {
e.printStackTrace();
}
}
public void calc() {
int n1 = Integer.parseInt(tf1.getText());
int n2 = Integer.parseInt(tf2.getText());
int n3 = Integer.parseInt(tf3.getText());
int result = 0;
for (int i = n1; i <= n2; i++) {
if (i % n3 == 0) {
result += i;
}
}
String str = String.valueOf(result);
tf4.setText(str);
}
public static void main(String[] args) {
launch(args);
}
}
fxml 구조
<VBox prefHeight="181.0" prefWidth="712.0" xmlns="http://javafx.com/javafx/18" xmlns:fx="http://javafx.com/fxml/1">
<children>
<AnchorPane prefHeight="271.0" prefWidth="638.0">
<children>
<Label layoutX="125.0" layoutY="78.0" text="에서" />
<Label layoutX="259.0" layoutY="78.0" text="까지" />
<Button id="btn" layoutX="400.0" layoutY="74.0" mnemonicParsing="false" text="의 배수의 합은" />
<TextField id="tf1" layoutX="38.0" layoutY="74.0" prefHeight="25.0" prefWidth="75.0" />
<TextField id="tf2" layoutX="167.0" layoutY="74.0" prefHeight="25.0" prefWidth="75.0" />
<TextField id="tf3" layoutX="305.0" layoutY="74.0" prefHeight="25.0" prefWidth="75.0" />
<TextField id="tf4" layoutX="515.0" layoutY="74.0" />
</children>
</AnchorPane>
</children>
</VBox>
실행
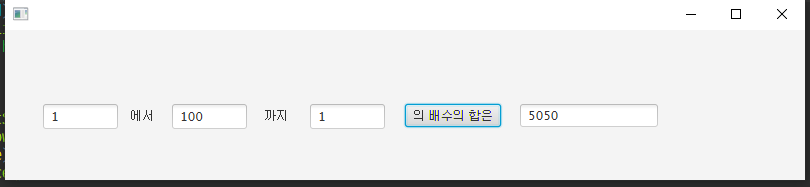
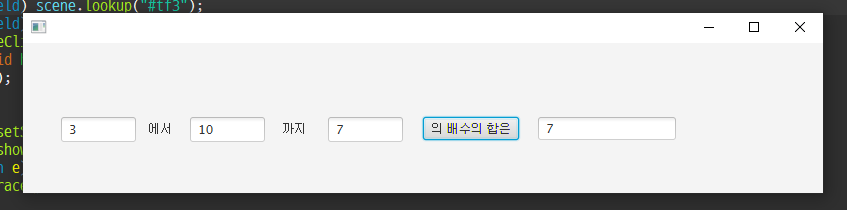