클래스와 인스턴스의 일반적 개념
Constructor
- Class의 생성자 함수
- 객체를 초기화하는 역할
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
}
sayHello() {
console.log(`Hello, my name is ${this.name} and I'm ${this.age} years old.`);
}
}
const person = new Person('Alice', 20);
Getter와 Setter
class Rectangle {
constructor(height, width) {
this._height = height;
this._width = width;
}
get width() {
return this._width;
}
set width(value) {
if (value <= 0) {
console.log("[오류] 가로길이는 0보다 커야 합니다!");
return;
} else if (typeof value !== "number") {
console.log("[오류] 가로길이로 입력된 값이 숫자타입이 아닙니다!");
return;
}
this._width = value;
}
get height() {
return this._height;
}
set height(value) {
if (value <= 0) {
console.log("[오류] 세로길이는 0보다 커야 합니다!");
return;
} else if (typeof value !== "number") {
console.log("[오류] 세로길이로 입력된 값이 숫자타입이 아닙니다!");
return;
}
this._height = value;
}
getArea() {
const a = this._width * this._height;
console.log(`넓이는 => ${a}입니다.`);
}
}
const rect1 = new Rectangle(10, 7);
rect1.getArea();
- 주의!
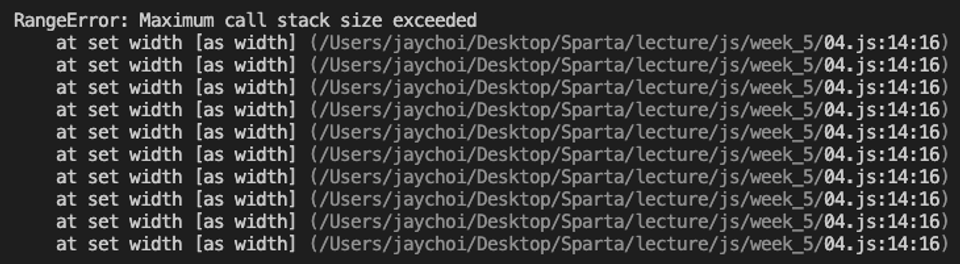
- 이런 오류가 발생한다면
this
로 접근하는 property에 underscore(_)
를 사용하지 않았을 확률이 높다!
상속
- Class는 상속을 통해 다른 Class의 기능을 물려받을 수 있다
subclass
또는 derived class
superclass
또는 base class
class Animal {
constructor(name) {
this.name = name;
}
speak() {
console.log(`${this.name} makes a noise.`);
}
}
class Dog extends Animal {
speak() {
console.log(`${this.name} barks.`);
}
}
let d = new Dog('Mitzie');
d.speak();
Static Method
static
키워드를 사용하여 Class 레벨의 메소드를 정의
- 인스턴스를 만들 필요가 없을 떄 사용
class Calculator {
static add(a, b) {
return a + b;
}
static subtract(a, b) {
return a - b;
}
}
console.log(Calculator.add(1, 2));
console.log(Calculator.subtract(3, 2));