1. 배열에서의 초기화 방법은?
n 을 자연수라고 할 때,
- 배열은 생성과 동시에 초기화가 된다.
- int[] arrInt = new int[n];
- int[] arrInt2 = {0, 0, 0, 0, 0,};
- String[] arrStr = new String[n];
- String[] arrStr2 = {new String(), new String(), new String(), new String(), new String()};
2. main 함수에서 String[] args 사용 방법은?
- String array 를 parameter 로 받는다.
- 띄어쓰기로 String 을 구분한다.
- " "로 space 를 String 으로 입력시킬 수 있다.
- args 로 받은 String 으로 해당 method 를 작동시킬 수 있다.
3. enhanced for 문이란?
- for(int i : arr) 과 같은 형태로 나온다.
- index 값이 0부터 (length-1)까지 순서대로 모든 값을 읽기 때문에 변칙적인 사용이 어렵다.
4. Box 클래스를 짜시오. (개별진척도 1번)
class Box {
private int boxNum;
private String item;
Box(int boxNum, String item) {
this.boxNum = boxNum;
this.item = item;
}
int getBoxNum() {
return this.boxNum;
}
String getItem() {
return this.item;
}
public String toString() {
return getItem();
}
}
5. 프로그램을 완성 하시오.
양의 정수 100개를 랜덤 생성하여, 배열에 저장하고, 배열에 있는 정수 중에서 3의 배수만 출력해 보자.
import java.util.concurrent.ThreadLocalRandom;
class MultiplesOfThreeFromRandom2 {
private static final int HUNDRED = 100;
private static int[] arrRandom = new int[HUNDRED];
public static void main(String[] args) {
StringBuilder print = new StringBuilder();
for (int i = 0; i < HUNDRED; i++) {
int randomNaturalNum = ThreadLocalRandom.current().nextInt(1, Integer.MAX_VALUE);
arrRandom[i] = randomNaturalNum;
if (arrRandom[i] % 3 == 0) {
print.append("index ");
print.append(i);
print.append(": ");
print.append(arrRandom[i]);
print.append("\n");
};
};
System.out.print(print);
}
}
//
// 출력
//
index 0: 835227867
index 3: 1944759903
index 4: 358584246
index 7: 2137316607
index 11: 1704681981
index 17: 1074307857
index 18: 1167777096
index 31: 1525169562
index 34: 468963195
index 35: 6648606
index 36: 845941845
index 37: 1797471282
index 38: 63993645
index 40: 2033276553
index 44: 113358183
index 45: 1382507586
index 46: 1698093222
index 48: 606871119
index 54: 1435639647
index 55: 1724943513
index 58: 725278119
index 60: 290199156
index 65: 1065570474
index 66: 1526358060
index 70: 1397797557
index 74: 1590899283
index 76: 182503812
index 80: 345397179
index 82: 2018367258
index 85: 1336629279
index 86: 1832680674
index 87: 1524678984
index 89: 334948728
index 93: 1337114223
index 96: 507394308
index 98: 75790347
6. Rectangle 을 배열로 3개 선언
해당 객체에 인덱스 순서대로 가로 세로 설정 -
이번에는 반드시 scanner 로 입력 받을것
//
해당 배열에 있는 Rectangle 의 총넓이의 합을 구하시오.
//
//
또한 아래의 함수도 만들것(static 으로 만들것)
- 파라미터를 Rectangle 배열로 받아서 해당 배열에 들어 잇는
Rectangle 들에 총 넓이를 리턴 하는 함수를 만드시오.
import java.util.*;
class Rectangle {
private double length;
private double width;
Rectangle(double[] rectangle) {
this.length = rectangle[0];
this.width = rectangle[1];
}
double getArea() {
return (this.length * this.width);
}
}
class RectangleArea {
private static final int HOW_MANY = 10;
private final static String COMMA = ", ";
private final static String STR_SITUATION_OK = "군요. 알겠습니다.\n";
private final static String STR_SITUATION_ASK = "니까?(Y/N)\n";
private final static String STR_SITUATION_PARDON = "...? 다시 한번 말씀해주시겠습";
private final static String STR_SITUATION_NEWLINE = "\n";
private final static String STR_SITUATION_BR = "\n\n";
private final static String STR_SITUATION_SPACE = " ";
private final static String STR_SITUATION_ESC = "니까?(x로 탈출): ";
private final static double sumOfRectangles(Rectangle[] rectangles) {
double sum = 0.;
for (int i = 0; i < rectangles.length; i++) {
Rectangle tempRec = rectangles[i];
if (tempRec != null) {
sum += rectangles[i].getArea();
} else {
break;
};
};
return sum;
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
StringBuilder print = new StringBuilder();
boolean run = true;
Rectangle[] rectangles = new Rectangle[HOW_MANY];
for (int i = 0; i < HOW_MANY; i++) {
int[] arrRec = new int[2];
print.append("사각형을 배열에 넣겠습니까?: ");
System.out.print(print);
print.setLength(0);
run = yesOrNo();
if (run == true) {
print.append("가로길이를 입력해주세요: ");
System.out.print(print);
print.setLength(0);
int tempLength = scanner.nextInt();
print.append("세로길이를 입력해주세요: ");
System.out.print(print);
print.setLength(0);
int tempWidth = scanner.nextInt();
double[] tempLW = new double[2];
tempLW[0] = (double)tempLength;
tempLW[1] = (double)tempWidth;
Rectangle rectangle = new Rectangle(tempLW);
rectangles[i] = rectangle;
} else {
break;
};
};
print.append("사각형들의 총 넓이는 ");
print.append(sumOfRectangles(rectangles));
print.append("입니다.");
System.out.print(print);
print.setLength(0);
}
private static boolean yesOrNo() {
Scanner scanner = new Scanner(System.in, "Cp949");
StringBuilder print = new StringBuilder();
boolean yn = false;
boolean asking = true;
while (asking) {
String answer = (scanner.nextLine().replaceAll("\\s+","")).toLowerCase();
switch (answer) {
case "네": case "예": case "yes": case "y": case "ㅇ": case "ㅇㅇ": case "어": case "어어": case "응": case "그래": {
asking = false;
yn = true;
break;
}
case "아니오": case "아니요": case "아뇨": case "아닙니다": case "no": case "n": case "ㄴ": case "ㄴㄴ": case "아니": {
asking = false;
yn = false;
break;
}
default: {
print.append(STR_SITUATION_NEWLINE);
print.append(answer);
print.append(STR_SITUATION_PARDON);
print.append(STR_SITUATION_ASK);
System.out.print(print);
print.setLength(0);
}
};
};
return yn;
}
}
//
// 출력
//
사각형을 배열에 넣겠습니까?: 3
//
3...? 다시 한번 말씀해주시겠습니까?(Y/N)
4
//
4...? 다시 한번 말씀해주시겠습니까?(Y/N)
ㅇ
가로길이를 입력해주세요: 4
세로길이를 입력해주세요: 56
사각형을 배열에 넣겠습니까?: ㅇ
가로길이를 입력해주세요: 4
세로길이를 입력해주세요: 3
사각형을 배열에 넣겠습니까?: ㅇ
가로길이를 입력해주세요: 4
세로길이를 입력해주세요: 5
사각형을 배열에 넣겠습니까?: ㄴ
사각형들의 총 넓이는 256.0입니다.
7. 아래를 코딩 하시오.
4행 4열짜리 정수형 배열을 선언 및 할당하고
1) 1 ~ 16까지 값을 차례대로 저장하세요.
2) 저장된 값들을 차례대로 출력하세요.
//
1 2 3 4
5 6 7 8
9 10 11 12
13 14 15 16
class PrintArray {
private static final int LENGTH_FIRST = 4;
private static final int LENGTH_SECOND = 4;
public static void main(String[] args) {
StringBuilder print = new StringBuilder();
int[][] arrInt2d = new int[LENGTH_FIRST][LENGTH_SECOND];
int num = 0;
for (int i = 0; i < LENGTH_FIRST; i++) {
for (int j = 0; j < LENGTH_SECOND; j++) {
arrInt2d[i][j] = ++num;
print.append(arrInt2d[i][j]);
if (j != LENGTH_SECOND - 1) {
print.append(" ");
};
};
if (i != LENGTH_FIRST - 1) {
print.append("\n");
};
};
System.out.print(print);
}
}
//
// 출력
//
1 2 3 4
5 6 7 8
9 10 11 12
13 14 15 16
8. 아래의 배열을 선언후 출력이 다음과 같이 되도록 하시오.
int[][] arr = new int[3][3];
//
출력====================
(0, 0)(0, 1)(0, 2)
(1, 0)(1, 1)(1, 2)
(2, 0)(2, 1)(2, 2)
class PrintArray2d {
private static final int LENGTH_FIRST = 3;
private static final int LENGTH_SECOND = 3;
public static void main(String[] args) {
StringBuilder print = new StringBuilder();
int[][] arr = new int[LENGTH_FIRST][LENGTH_SECOND];
for (int i = 0; i < LENGTH_FIRST; i++) {
for (int j = 0; j < LENGTH_SECOND; j++) {
print.append("(");
print.append(i);
print.append(", ");
print.append(j);
print.append(")");
};
if (i != LENGTH_FIRST - 1) {
print.append("\n");
};
};
System.out.print(print);
print.setLength(0);
}
}
//
// 출력
//
(0, 0)(0, 1)(0, 2)
(1, 0)(1, 1)(1, 2)
(2, 0)(2, 1)(2, 2)
9. 아래와 같이 선언시, 메모리 그림을 그리시오.
int[][] arr = {
{11},
{22, 33},
{44, 55, 66}
};
class DrawArray {
private static final String HASHCODE = "메모리주소는 ";
private static final String IS = " 입니다.";
public static void main(String[] args) {
int[][] arr = {
{11},
{22, 33},
{44, 55, 66}
};
printHashCode(arr);
printHashCode(arr[0]);
printHashCode(arr[0][0]);
printHashCode(arr[1]);
printHashCode(arr[1][0]);
printHashCode(arr[1][1]);
printHashCode(arr[2]);
printHashCode(arr[2][0]);
printHashCode(arr[2][1]);
printHashCode(arr[2][2]);
}
private static void printHashCode(int[][] arrInt2d) {
System.out.println(HASHCODE + System.identityHashCode(arrInt2d) + IS);
}
private static void printHashCode(int[] arrInt) {
System.out.println(HASHCODE + System.identityHashCode(arrInt) + IS);
}
private static void printHashCode(int integer) {
System.out.println(HASHCODE + System.identityHashCode(integer) + IS);
}
}
//
// 출력
//
메모리주소는 664740647 입니다.
메모리주소는 791452441 입니다.
메모리주소는 834600351 입니다.
메모리주소는 471910020 입니다.
메모리주소는 531885035 입니다.
메모리주소는 1418481495 입니다.
메모리주소는 303563356 입니다.
메모리주소는 135721597 입니다.
메모리주소는 142257191 입니다.
메모리주소는 1044036744 입니다.
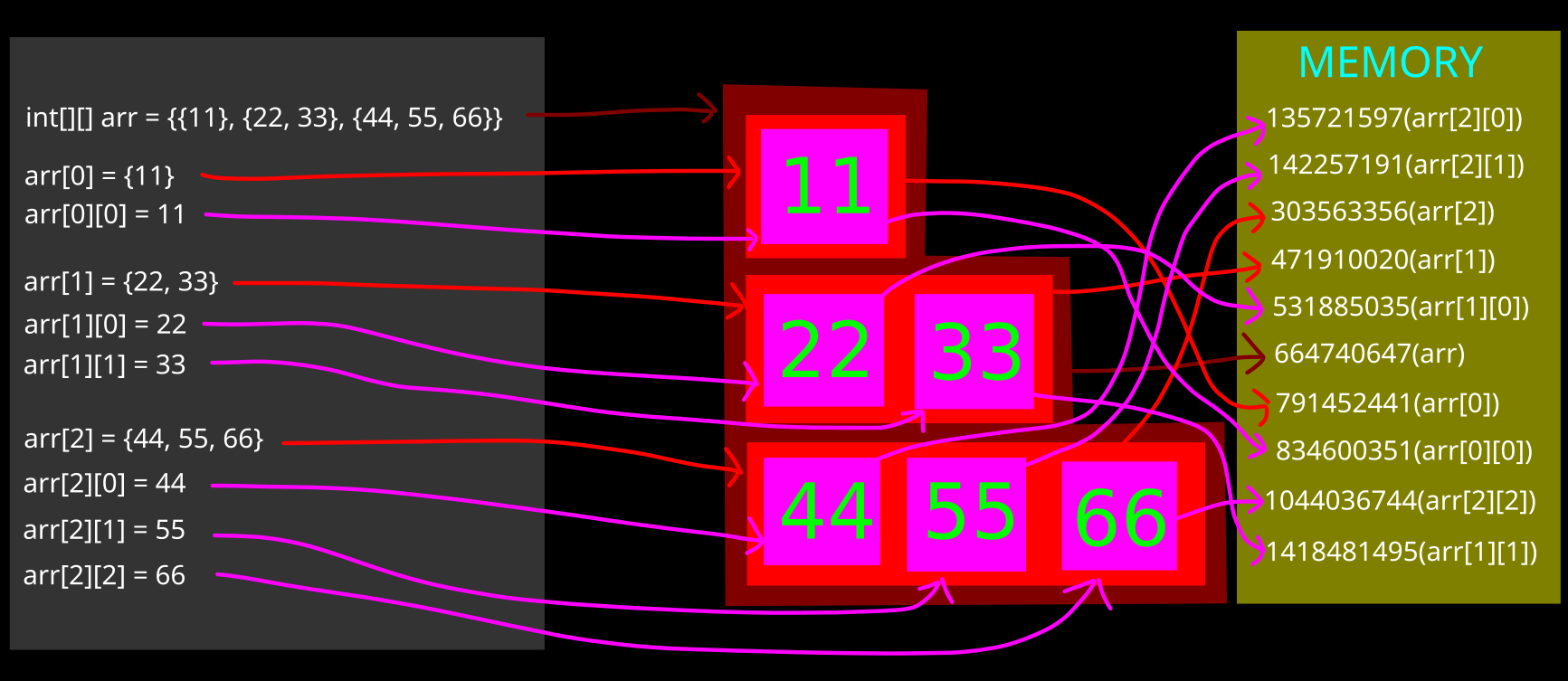