1. 상속을 UML로 표시하면?
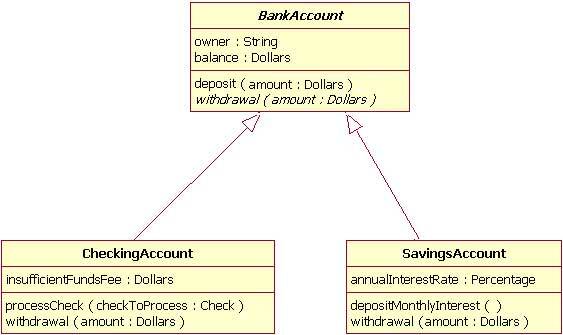
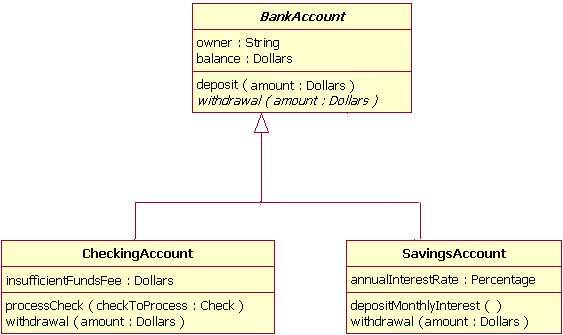
2. 부모 클래스와 자식 클래스의 다른 용어들은?
- parent class: 상위 class, 기초 class
- child class: 하위 class, 유도 class
3. this 키워드와 super 키워드의 차이는 무엇인가요?
- this: "this" can call the class and its parent class at the same time.
- super: "super" can call only its parent class.
4. 다음 main() 메소드와 실행 결과를 참고하여 TV를 상속받은 ColorTV 클래스를 작성하라. 다음 TV 클래스가 있다.
class TV{
private int size;
public TV(int size) { this.size = size; }
protected int getSize() { return size; }
}
public static void main(String[] args) {
ColorTV myTV = new ColorTV(32, 1024);
myTV.printProperty();
}
public class ColorTV extends TV {
private int color;
protected int getColor() {
return this.color;
}
protected ColorTV(int size, int color) {
super(size);
this.color = color;
}
protected void printProperty() {
StringBuilder print = new StringBuilder();
print.append(super.getSize());
print.append("인치 ");
print.append(this.color);
print.append("컬러");
System.out.print(print);
print.setLength(0);
}
}
//
// 출력
//
32인치 1024컬러
4-2. 단일 상속과 다중 상속 이란 무엇인가요? UML 로의 표기는?
- Single inheritance: Child class inherits from only one parent class.
- Multiple inheritance: Child class inherits from multiple parent classes.
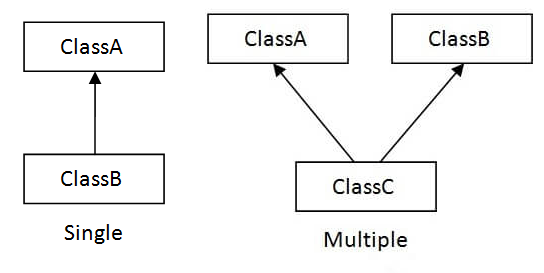
5. 다음 main() 메소드와 실행 결과를 참고하여 ColorTV를 상속받는 IPTV 클래스를 작성하라.
public static void main(String[] args) {
IPTV iptv = new IPTV("192.1.1.2", 32, 2048);
iptv.printProperty();
}
public class IPTV extends ColorTV {
private String ip;
protected IPTV(String ip, int size, int color) {
super(size, color);
this.ip = ip;
}
protected void printProperty() {
StringBuilder print = new StringBuilder();
print.append("\"");
print.append(this.ip);
print.append("\" 주소에 ");
System.out.print(print);
super.printProperty();
}
}
//
// 출력
//
"192.1.1.2" 주소에 32인치 2048컬러
6. 아래가 에러가 나는 이유는
class Man {
String name;
public Man(String name) {
this.name = name;
}
public void tellYourName() {
System.out.println("My name is " + name);
}
}
class BusinessMan extends Man {
String company;
String position;
public BusinessMan(String name, String company, String position) {
this.name = name;
this.company = company;
this.position = position;
}
public void tellYourInfo() {
System.out.println("My company is " + company);
System.out.println("My position is " + position);
tellYourName();
}
}
- Default super constructor is declared when there is no super constructor in child class. But the constructor declared in "class Man" needs parameter String. But there is no String in defualt super constructor in the constructor of "class BusinessMan". So Javac is not able to compile this one.
7.코딩 연습 문제:
- 2차원 배열 4 * 3 을 선언하고, 각각의 방을 랜덤으로 값을 넣은뒤 모두 출력하시오.
class ArrRandom {
private static final int INDEX_FIRST = 4;
private static final int INDEX_SECOND = 3;
private static double random() {
return Math.random();
}
public static void main(String[] args) {
double[][] arrRandom = new double[INDEX_FIRST][INDEX_SECOND];
StringBuilder print = new StringBuilder();
for (int i = 0; i < INDEX_FIRST; i++) {
for (int j = 0; j < INDEX_SECOND; j++) {
arrRandom[i][j] = random();
print.append(arrRandom[i][j]);
print.append(" ");
};
print.append("\n");
};
System.out.print(print);
}
}
//
// 출력
//
0.027639788134656396 0.764505341321175 0.8175310367182298
0.6124643025460227 0.47160828190343973 0.5429076669072928
0.5812432719619075 0.8331101041350073 0.6503092946397562
0.9777596013842536 0.6061075618088604 0.1257666348490034