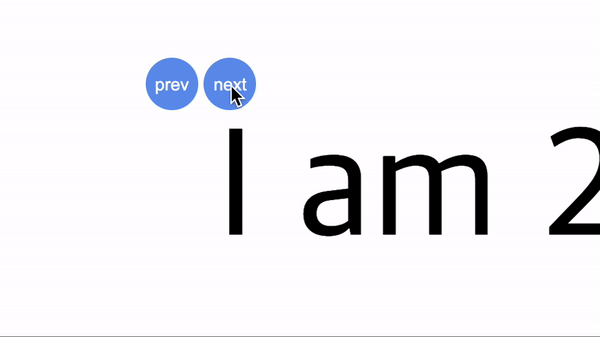
HTML + CSS
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>무한루프 슬라이더</title>
<script src="./main.js" defer></script>
<style>
body {
margin: 0;
}
.slide__conatiner {
position: relative;
height: 100vh;
overflow: hidden;
}
.slide__list {
height: 100%;
overflow: hidden;
}
.slide {
display: flex;
justify-content: center;
align-items: center;
float: left;
height: 100%;
font-size: 120px;
border: 1px solid black;
box-sizing: border-box;
}
.slide__controler {
position: absolute;
top: 20%;
left: 35%;
z-index: 1;
}
.slide__controler button {
width: 40px;
height: 40px;
background-color: cornflowerblue;
color: white;
border-radius: 50%;
border: 1px solid cornflowerblue;
}
</style>
</head>
<body>
<div class="slide__conatiner">
<div class="slide__list">
<div class="slide">I am 1</div>
<div class="slide">I am 2</div>
<div class="slide">I am 3</div>
<div class="slide">I am 4</div>
</div>
<div class="slide__controler">
<button class="btn__prev">prev</button>
<button class="btn__next">next</button>
</div>
</div>
</body>
</html>
JavaScript
const contanier = document.querySelector('.slide__contanier');
const list = document.querySelector('.slide__list');
const slide = document.querySelectorAll('.slide');
const nextBtn = document.querySelector('.btn__next');
const prevBtn = document.querySelector('.btn__prev');
const transitionTime = 200;
let currentIndex = 0;
let newIndex = slide.length + 2;
let newWidth = 100 / newIndex;
setLayout();
function setLayout() {
list.style.width = `${100 * newIndex}%`;
let fristclone = slide[0].cloneNode(true);
let lastclone = slide[slide.length - 1].cloneNode(true);
list.appendChild(fristclone);
list.prepend(lastclone);
const newSlide = document.querySelectorAll('.slide');
for (value of newSlide) {
value.style.width = `${newWidth}%`;
}
currentIndex = 1;
list.style.transform = `translateX(-${newWidth * currentIndex}%)`;
}
nextBtn.addEventListener('click', () => {
list.style.transition = `${transitionTime}ms`;
currentIndex++;
if (currentIndex == slide.length + 1) {
list.style.transform = `translateX(-${newWidth * currentIndex}%)`;
currentIndex = 1;
reset();
} else {
list.style.transform = `translateX(-${newWidth * currentIndex}%)`;
}
});
prevBtn.addEventListener('click', () => {
list.style.transition = `${transitionTime}ms`;
currentIndex--;
if (currentIndex <= 0) {
list.style.transform = `translateX(-${newWidth * currentIndex}%)`;
currentIndex = 4;
reset();
} else {
list.style.transform = `translateX(-${newWidth * currentIndex}%)`;
}
});
function reset() {
setTimeout(() => {
list.style.transition = `none`;
list.style.transform = `translateX(-${newWidth * currentIndex}%)`;
}, transitionTime);
}