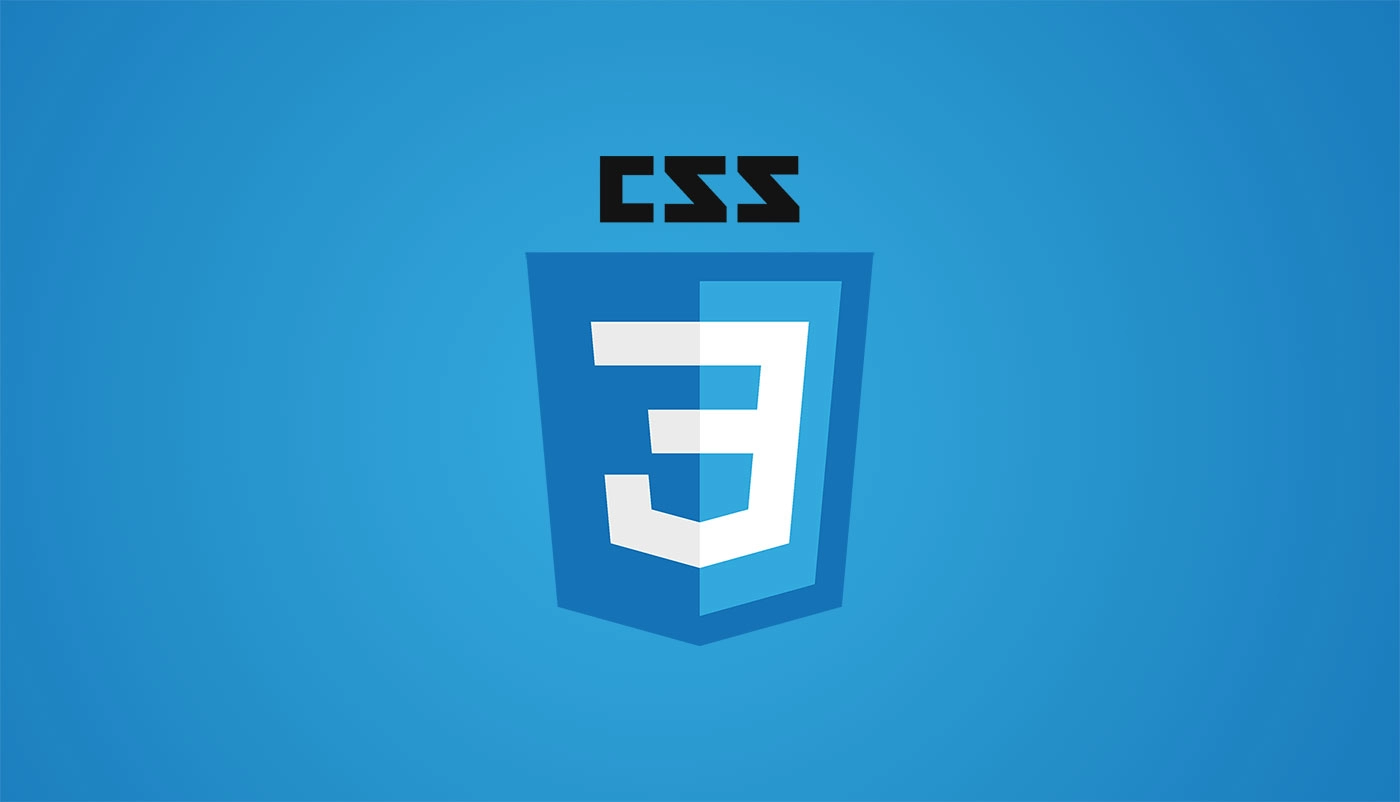
🖋️ CSS 의 Flex 와 Grid 로 반응형 레이아웃 설계 기록하기
FLEX
"한 방향 레이아웃"
Flex Container
- Flexbox 레이아웃을 적용할 부모 요소
- "display: flex" 또는 "display: inline-flex" 지정
Flex Items
- Flex Container 내부의 직접적인 자식 요소들
주 축과 교차 축
- Flex Box 의 레이아웃 방향을 결정
- 주 축은 "flex-direction" 속성으로 설정
- 교차 축은 주 축에 수직
@ 설계 방법
- 핵심 요소 정의
- flex-container 와 flex-items 결정
- 레이아웃 방향 설정
- flex-direction 을 사용하여 주 축의 방향 설정
- row 또는 column
- 공간 분배
- justify-content, align-items, align-content 를 사용하여 항목 간 간격과 정렬 조정
- 항목 크기 조정
- flex-grow, flex-shrink, flex-basis 를 사용하여 flex items 의 확장 및 축소 비율 조정
import React from 'react';
import './App.css';
function App() {
return (
<div className="flex-container">
<header className="header">Header</header>
<aside className="sidebar">Sidebar</aside>
<main className="content">Content</main>
<footer className="footer">Footer</footer>
</div>
);
}
export default App;
.flex-container {
display: flex;
flex-direction: column;
min-height: 100vh;
}
.header, .footer {
background-color: #f3f3f3;
text-align: center;
padding: 1rem;
}
.content {
flex: 1;
background-color: #fff;
padding: 1rem;
}
.sidebar {
background-color: #e9e9e9;
padding: 1rem;
order: -1;
}
@media (min-width: 768px) {
.flex-container {
flex-direction: row;
}
.content, .sidebar {
flex: 1;
}
}
GRID
"두 방향 레이아웃"
Grid Container
- Grid 레이아웃을 적용할 부모 요소
- "display: grid" 또는 "display: inline-grid" 지정
Grid Lines
- Grid의 행과 열을 구분하는 선
- 항목을 배치할 때 이 선을 기준
Grid Tracks
Grid Cells
- Grid의 단일 단위 공간
- Grid 아이템이 위치할 수 있는 가장 작은 단위
Grid Areas
- 여러 개의 Grid Cell을 결합한 영역
- 복잡한 레이아웃을 쉽게 구성
@ 설계 방법
- 그리드 구조 정의
- grid-template-rows, grid-template-columns를 사용
- 그리드의 행과 열 크기를 정의
- 항목 배치
- grid-row, grid-column, grid-area 속성을 사용
- grid items를 정확한 위치에 배치
- 반응형 디자인
- auto-fill, auto-fit과 같은 키워드와 함께 minmax() 함수를 사용
- 유연한 그리드 트랙 크기를 정의하고, 다양한 화면 크기에 맞게 레이아웃을 조정
import React from 'react';
import './App.css';
function App() {
return (
<div className="grid-container">
<header className="header">Header</header>
<aside className="sidebar">Sidebar</aside>
<main className="content">Content</main>
<footer className="footer">Footer</footer>
</div>
);
}
export default App;
.grid-container {
display: grid;
grid-template-columns: 1fr;
grid-template-rows: auto 1fr auto;
grid-template-areas:
"header"
"content"
"footer";
min-height: 100vh;
}
.header {
grid-area: header;
background-color: #f3f3f3;
text-align: center;
padding: 1rem;
}
.content {
grid-area: content;
background-color: #fff;
padding: 1rem;
}
.sidebar {
grid-area: sidebar;
background-color: #e9e9e9;
padding: 1rem;
display: none;
}
.footer {
grid-area: footer;
background-color: #f3f3f3;
text-align: center;
padding: 1rem;
}
@media (min-width: 768px) {
.grid-container {
grid-template-columns: 1fr 3fr;
grid-template-rows: auto 1fr auto;
grid-template-areas:
"header header"
"sidebar content"
"footer footer";
}
.sidebar {
display: block;
}
}