HW60
#pragma warning (disable : 4996)
#include <stdio.h>
#include <string.h>
#define TRUE 1
#define FALSE 0
#define RESIDENT_NUMBER_LENGTH 13 /* 주민등록번호 길이*/
int availabilityCheck(char *resident_number);
int checkLengthCharacter(char *resident_number);
int checkDate(char *resident_number);
int checkIdentification(char *resident_number);
int checkGender(char *resident_number);
int checkYear(int year);
int main()
{
char resident_number[][20] = { "0402291000008", "870401102321", "00031541949179",
"0003154194917", "801203#201122", "7804155328845", "7804150328840",
"9612241068382", "9902292194322", "0230174326176", "8811391042219",
"8100122042213", "8112002042213", "9210101069415", "0802294012345",
"8806311069417","8807311069418" };
int i, count;
/* 검사할 주민등록번호의 개수 계산*/
count = sizeof(resident_number) / sizeof(resident_number[0]);
for (i = 0; i < count; i++) /* 주민등록번호 유효성 검사를 반복적으로 수행 함*/
{
if (availabilityCheck(resident_number[i]) == TRUE)
{
printf("(+) 주민번호%s는(은) 유효한 번호입니다.\n", resident_number[i]);
}
else
{
printf("(-) 주민번호%s는(은) 유효하지 않은 번호입니다.\n", resident_number[i]);
}
}
return 0;
}
/*----------------------------------------------------------------
availabilityCheck()함수: 주민등록번호 유효성 검사 함수
전달인자: 유효성 검사할 주민등록번호(문자열)
리턴값: 유효하면TRUE, 유효하지 않으면FALSE 리턴
------------------------------------------------------------------*/
int availabilityCheck(char *resident_number) {
if (checkLengthCharacter(resident_number) == TRUE) {
if (checkDate(resident_number) == TRUE) {
if (checkIdentification(resident_number) == TRUE)
return TRUE;
}
}
return FALSE;
}
/*-------------------------------------------------------------------------
checkLengthCharacter()함수: 주민등록번호 길이 및 문자 유효성검사 함수
전달인자: 검사할 주민등록번호(문자열)
리턴값: 주민등록번호의 길이가 맞고 숫자문자로만 구성되어 있으면TRUE,
길이가 짧거나 길고, 숫자 문자가 아닌 문자가 섞여 있으면FALSE 리턴
--------------------------------------------------------------------------*/
int checkLengthCharacter(char *resident_number) {
for (int i = 0;i < 13;i++) {
if (resident_number[i] >= 48 && resident_number[i] <= 57);
else return FALSE;
}
if (resident_number[13] == '\0') return TRUE;
else return FALSE;
}
/*----------------------------------------------------------------
checkDate()함수: 첫6자리(연,월,일)의 유효성 검사 함수
전달인자: 유효성 검사할 주민등록번호(문자열)
리턴값: 유효한 날짜이면TRUE, 유효하지 않은 날짜이면FALSE 리턴
------------------------------------------------------------------*/
int checkDate(char *resident_number) {
int allMonth[12] = { 31,28,31,30,31,30,31,31,30,31,30,31 };
int year = (10 * (resident_number[0] - 48)) + (resident_number[1] - 48);
int month = (10 * (resident_number[2] - 48)) + (resident_number[3] - 48);
int day = (10 * (resident_number[4] - 48)) + (resident_number[5] - 48);
if (checkGender(resident_number) == TRUE) {
switch (resident_number[6] - 48) {
case 1: year += 1900; break;
case 2: year += 1900; break;
case 3: year += 2000; break;
case 4: year += 2000; break;
}
}
else return FALSE;
if (checkYear(year) == TRUE) {
if (month >= 1 && month <= 12) {
if (month != 2) {
if (day > 0 && day <= allMonth[month - 1]) return TRUE;
}
else {
if (day > 0 && day <= 29) return TRUE;
}
}
}
else {
if (month >= 1 && month <= 12) {
if (day > 0 && day <= allMonth[month - 1]) return TRUE;
}
}
return FALSE;
}
/*----------------------------------------------------------------------
checkGender()함수: 7번째 자리의 성별식별번호 유효성 검사함수
전달인자: 유효성 검사할 주민등록번호(문자열)
리턴값: 성별식별번호가'1'~'4'이면TRUE, 그 외 숫자 문자이면FALSE 리턴
-----------------------------------------------------------------------*/
int checkGender(char *resident_number) {
int gender = resident_number[6] - 48;
if (gender >= 1 && gender <= 4) return TRUE;
return FALSE;
}
/*------------------------------------------------------------------------
checkIdentification()함수: 주민등록번호 끝자리(인식자) 유효성 검사 함수
전달인자: 유효성 검사할 주민등록번호(문자열)
리턴값: 유효한 인식자이면TRUE, 유효하지 않은 인식자이면 FALSE 리턴
-------------------------------------------------------------------------*/
int checkIdentification(char *resident_number) {
int sum = 0;
for (int i = 0;i < 12;i++) {
sum += (resident_number[i] - 48);
}
if ((sum % 10) == (resident_number[12] - 48)) return TRUE;
return FALSE;
}
/*----------------------------------------------------------------
checkYear ()함수: 년도의 윤,평년 여부 검사
전달인자: 윤,평년 검사할 년도
리턴값: 윤년이면 TRUE, 평년이면FALSE 리턴
------------------------------------------------------------------*/
int checkYear(int year) {
if (year % 4 != 0) {
return FALSE;
}
else {
if (year % 100 != 0) {
return TRUE;
}
else {
if (year % 400 == 0) {
return TRUE;
}
else {
return FALSE;
}
}
}
}
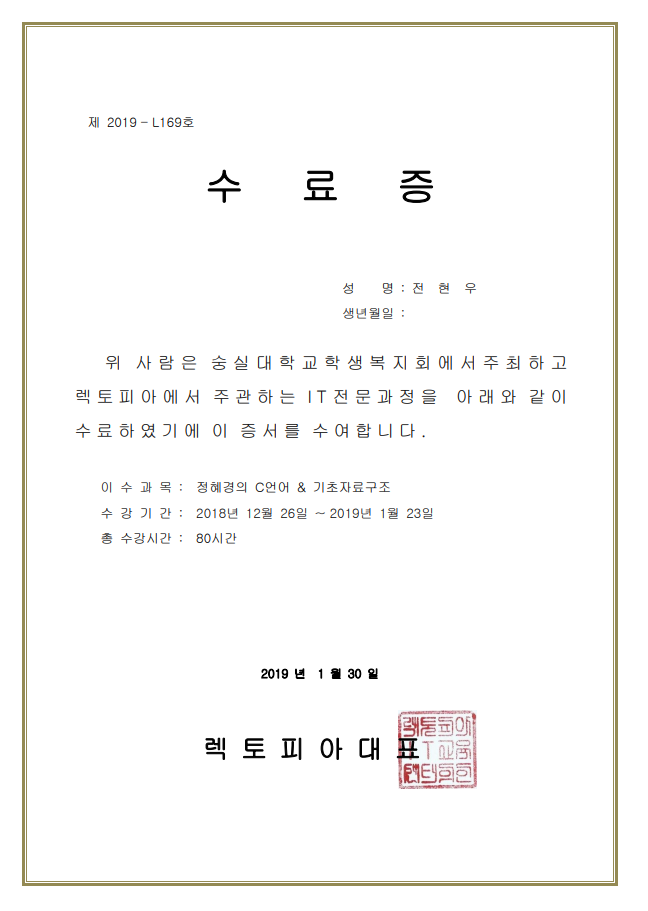