1. 객체의 종류
- 객체는 자바스크립트가 명령 가능한 모든 대상을 말한다.
1) 객체의 큰 부분
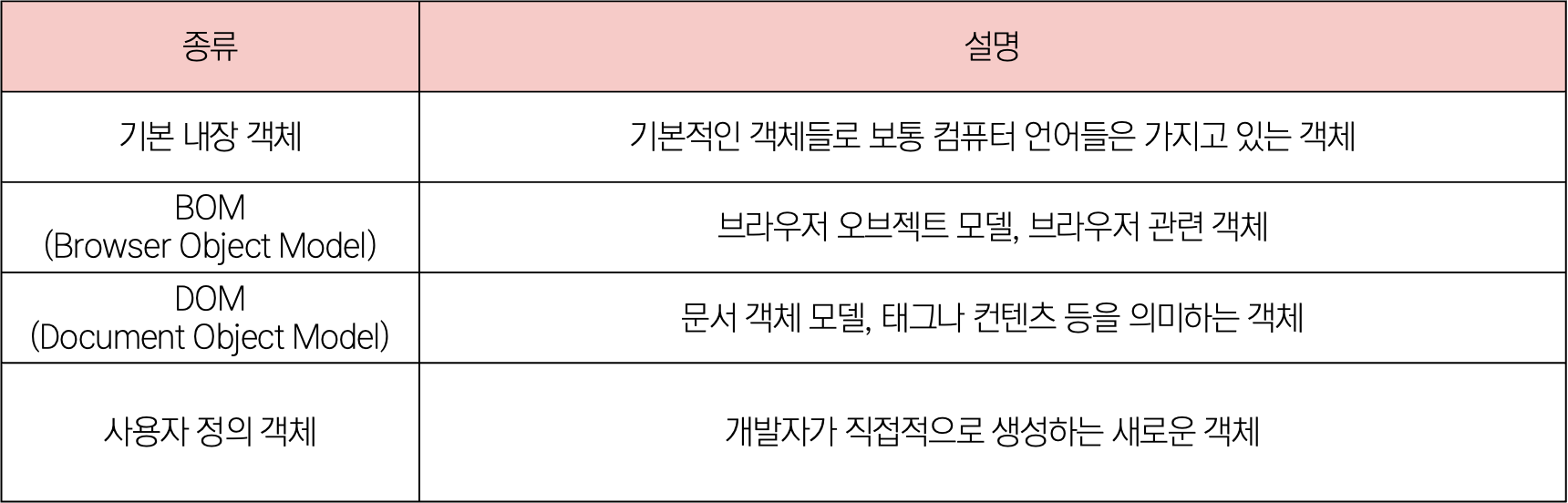
2) 기본내장객체
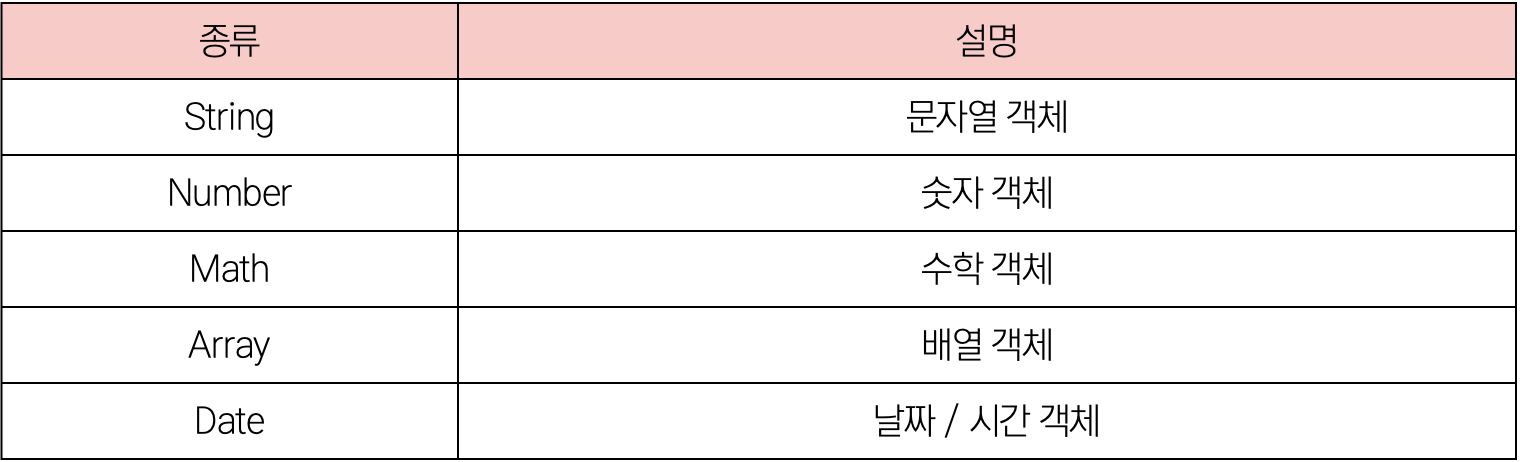
3) BOM 객체
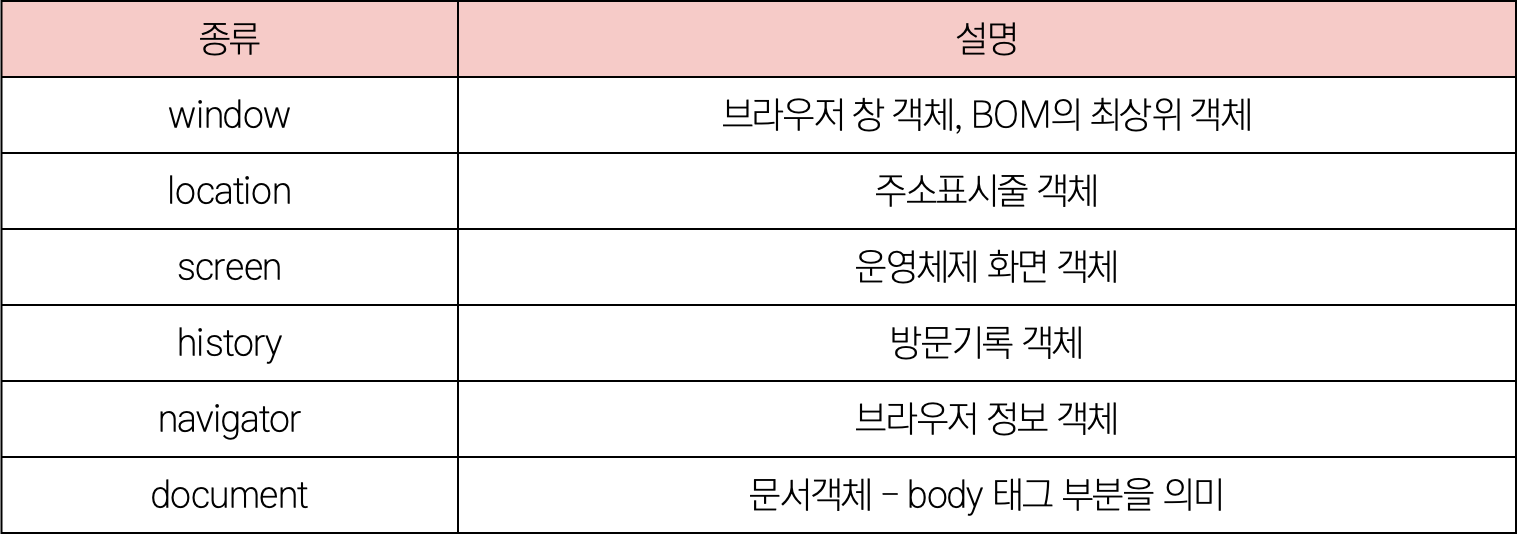
- 전부 소문자로 작성
- DOM은 BOM의 일부지만 매우 중요하기 때문에 구분해서 본다.
2. 객체 - Object
- 여기서 말하는 객체는 개발자가 만드는 사용자 정의 객체를 의미한다.
- 객체 종류를 확인하면 위와 같이 첫글자가 대문자인 [Object]로 처리된다.
1) 객체 모델링
- 보통 컴퓨터 언어에서 말하는 객체 모델링이란 현실 세계 객체로 변경하는 것을 의미한다.
- 자동차를 예시로 들어보자.
Object
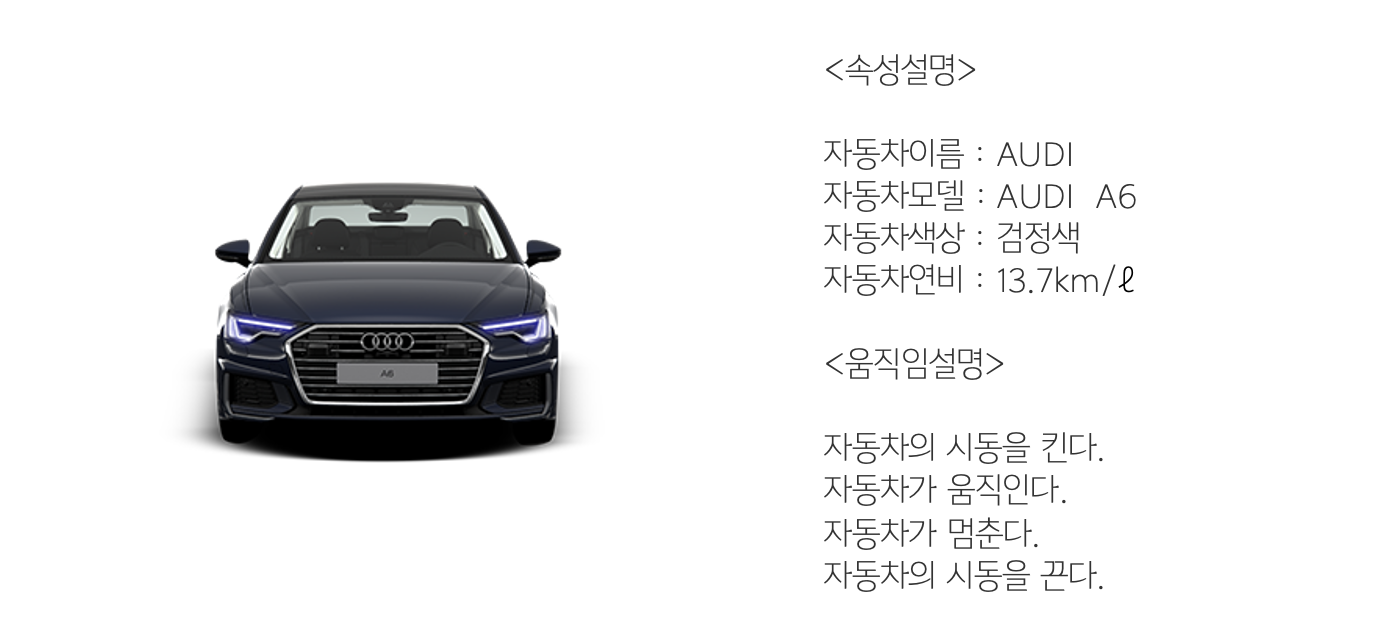
자바스크립트식 문법 정리
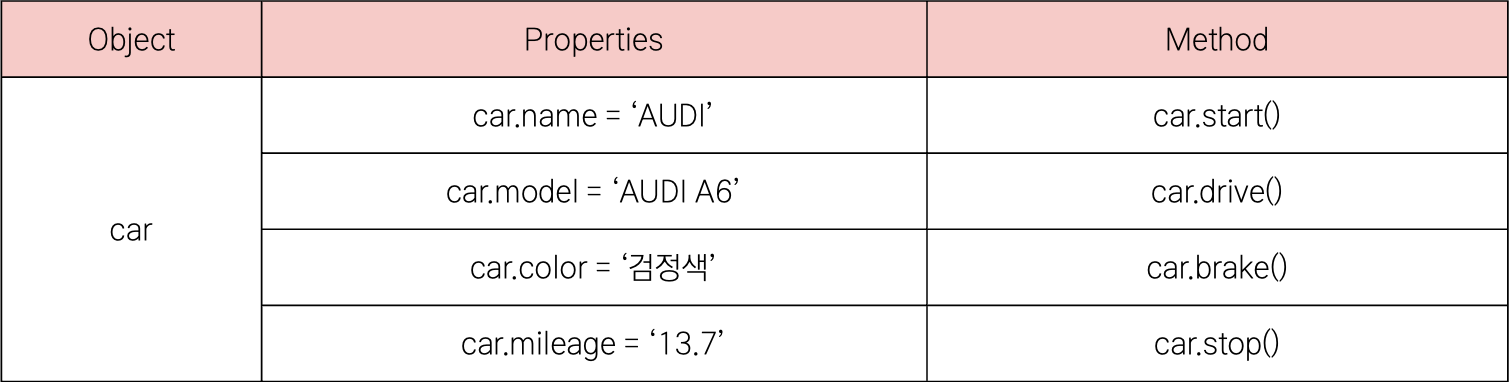
속성과 메서드
- 속성(properties) : 객체가 가지고 있는 고유한 특징을 기술한 것
- 메서드(method) : 객체가 가지고 있는 동작 혹은 객체에 주는 명령
2) 객체선언
📌 문법
<script>
var 객체명 = {
속성명: 값,
메서드: function(){
}
}
</script>
- 자바스크립트에서의 속성 : 값이 함수가 아닌 숫자, 문자열, 불, 객체로 이루어진 것
- 자바스크립트에서의 메서드 : 값이 함수인 것
✏️ 코드
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<title>JS 객체</title>
<script>
var car = {
name: 'AUDI',
model: 'AUDI A6',
color: ['black','white','blue'],
mileage: 13.7,
starting: function(x){
if(x == 'on'){
alert('자동차시동이 켜집니다.');
}else if(x == 'off'){
alert('자동차시동이 꺼집니다.');
}
}
}
</script>
</head>
<body>
</body>
</html>
3) 객체 호출
📌 문법
<script>
객체명.속성명;
객체명["속성명"];
객체명.메서드명();
</script>
✏️ 코드
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<title>JS 객체</title>
<script>
var car = {
name: 'AUDI',
model: 'AUDI A6',
color: ['black','white','blue'],
mileage: 13.7,
starting: function(x){
if(x == 'on'){
alert('자동차시동이 켜집니다.');
}else if(x == 'off'){
alert('자동차시동이 꺼집니다.');
}
}
}
alert(car.model);
alert(car.color[0]);
car.starting('on');
</script>
</head>
<body>
</body>
</html>
🖥️ 결과
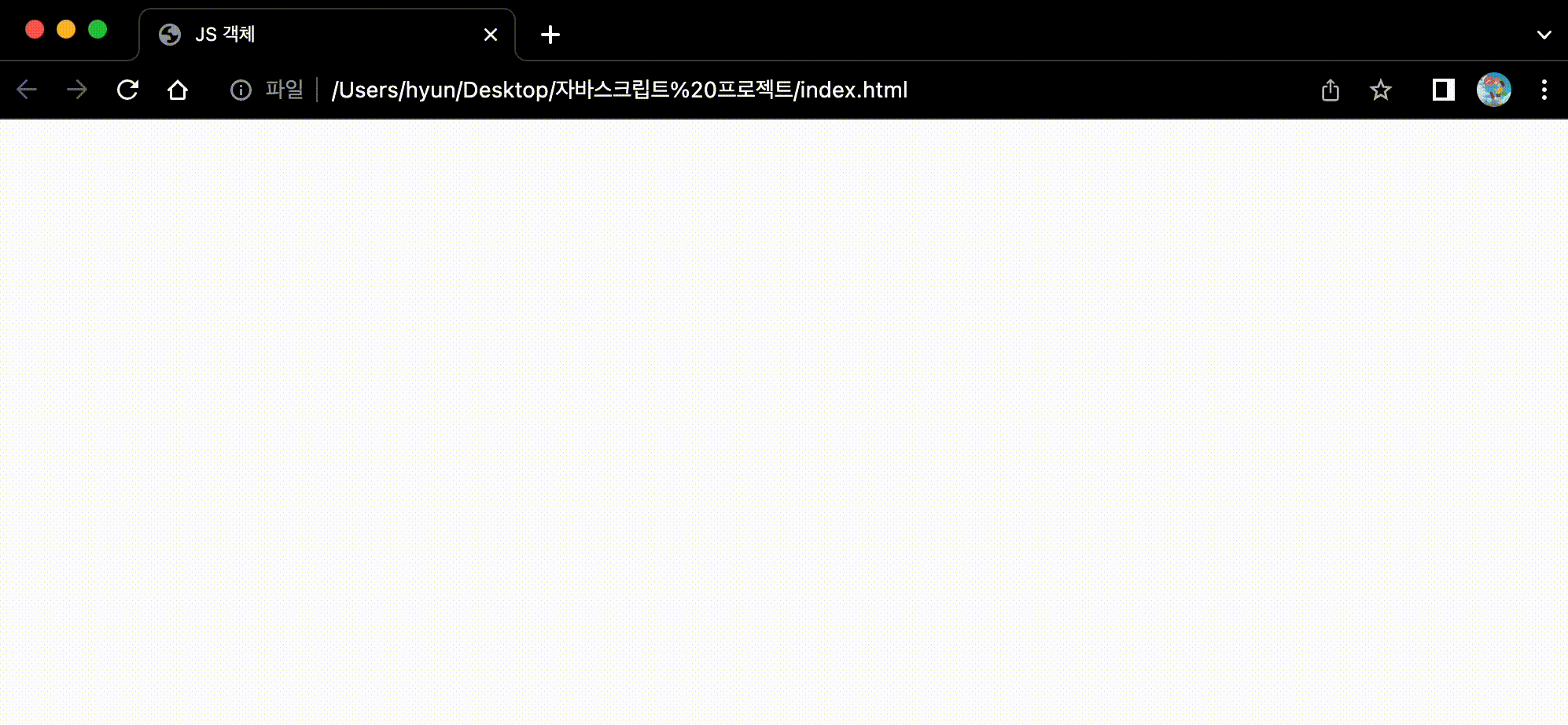
4) this 키워드
- this 키워드는 함수에서 함수의 '소유자'를 의미한다.
- 객체에서는 이 함수를 소유하고 있는 객체를 의미한다.
✏️ 코드
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<title>JS 객체</title>
<script>
var car = {
name: 'AUDI',
model: 'AUDI A6',
color: ['black','white','blue'],
mileage: 13.7,
myCar: function(){
alert('내 차이름은 ' + this.name + '입니다');
}
}
car.myCar();
</script>
</head>
<body>
</body>
</html>
- [this.name] 부분에 [car.name]을 써도 인식은 잘 된다.
- 하지만 객체가 한가지 사용되는 것이 아니라 여러개 사용되는 경우 선택이 애매해진다.
- 그래서 this 키워드를 사용하는 것이 좋다.
🖥️ 결과
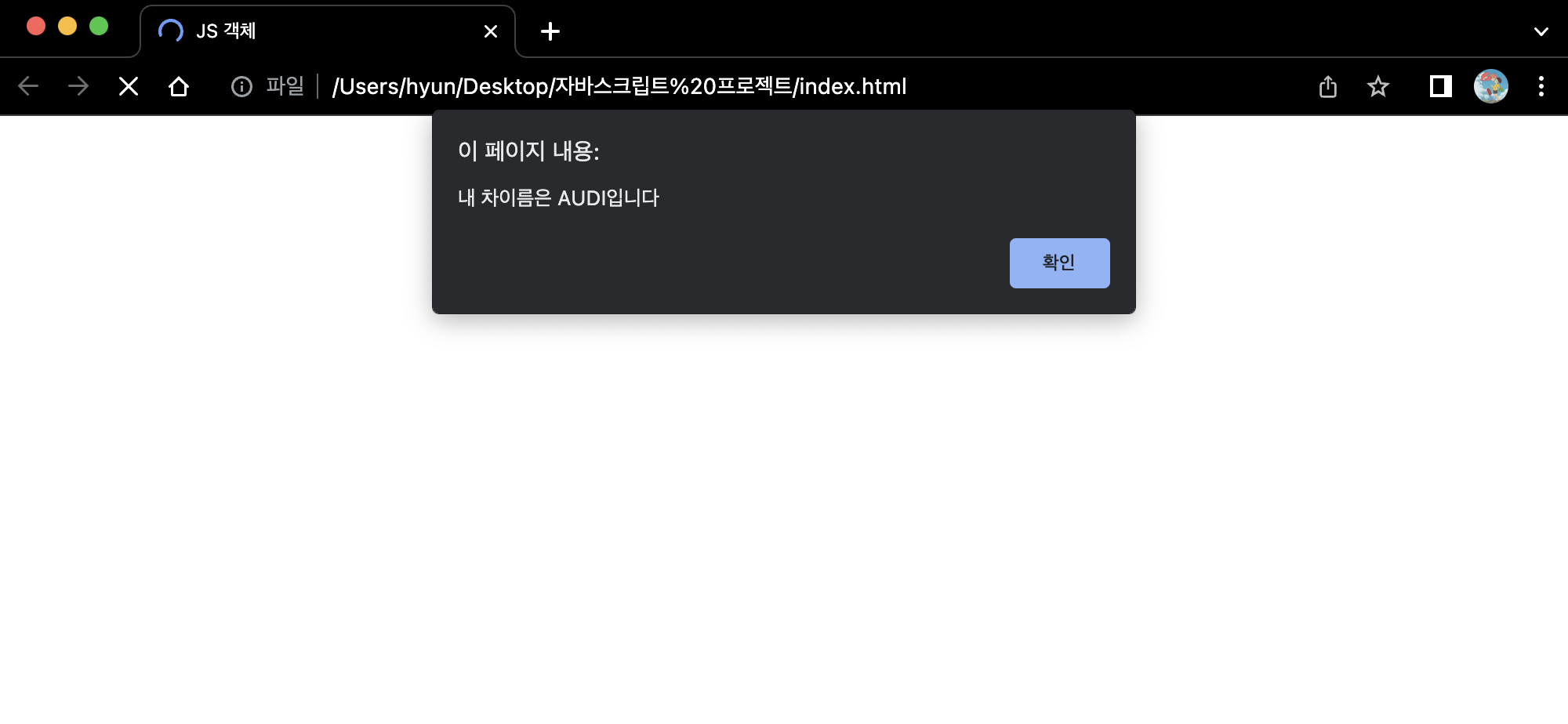
3. 객체 생성자함수
1) 생성자 함수
- 생성자 함수란 [객체]를 생성할 때 사용하는 함수
- 여러가지 통일된 속성을 가지는 객체를 생성하기 위해 필요하다.
📌 선언 문법
<script>
function 생성자함수명(){
this.속성 = 값;
}
</script>
- 생성자 함수명은 대문자로 시작하는 것이 관례이다. (함수와 구분짓기 위함)
📌 호출 문법
<script>
var 변수명 = new 생성자함수명();
</script>
- new라는 생성자 함수 키워드를 사용해서 객체를 새로운 인스턴스로 생성한다.
✏️ 코드
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<title>JS 객체</title>
<script>
function AUDI(model, color, mileage){
this.model = model;
this.color = color;
this.mileage = mileage;
this.starting = function(x){
if(x == 'on'){
alert(this.color + '인 ' + this.model + '가 시동이 켜졌습니다.');
}else if(x == 'off'){
alert(this.color + '인 ' + this.model + '가 시동이 꺼졌습니다.');
}
}
}
var hyunCar = new AUDI('AUDI A6','검정색',13.7);
var chanCar = new AUDI('AUDI Q5','하얀색',12.1);
hyunCar.starting('on');
chanCar.starting('on');
</script>
</head>
<body>
</body>
</html>
🖥️ 결과
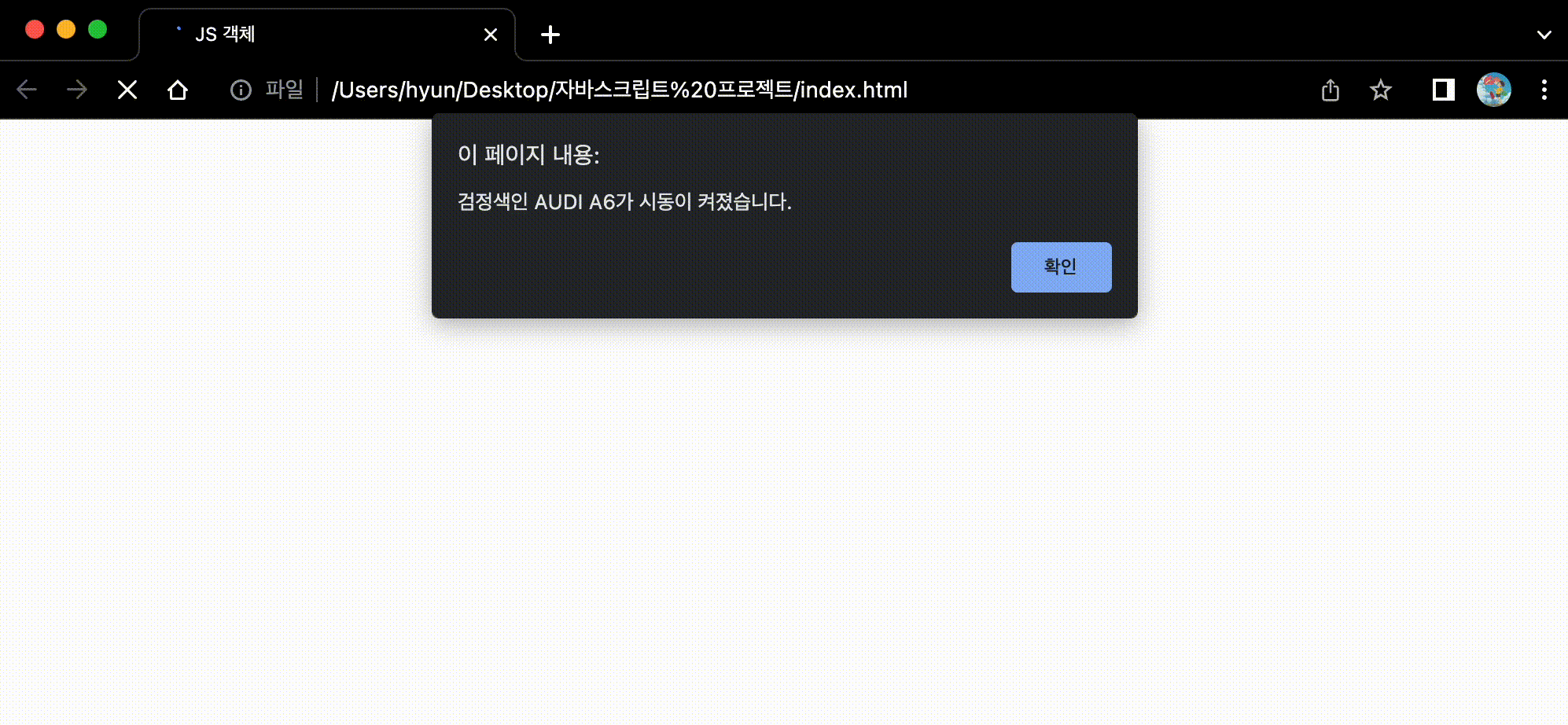
2) Prototype(프로토타입)
- Prototype이란 생성자 함수로 생성한 객체들이 properties(속성)과 method(메서드)를 공유하기 위해 사용하는 객체
- new를 통해 새롭게 생성한 객체의 속성과 메서드가 복사된 객체만큼 계속 생성되어 메모리를 많이 차지하기 때문에 비효율적이다.
- 같은 속성과 메서드이기 때문에 메모리를 잡아먹지 않도록, 이를 해결하기 위해 나온 것이 프로토타입이다.
✏️ proto 속성
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<title>JS 객체</title>
<script>
function AUDI(model, color, mileage){
this.model = model;
this.color = color;
this.mileage = mileage;
this.starting = function(x){
if(x == 'on'){
alert(this.color + '인 ' + this.model + '가 시동이 켜졌습니다.');
}else if(x == 'off'){
alert(this.color + '인 ' + this.model + '가 시동이 꺼졌습니다.');
}
}
}
var hyunCar = new AUDI('AUDI A6','검정색',13.7);
var chanCar = new AUDI('AUDI Q5','하얀색',12.1);
console.log(hyunCar);
console.log(chanCar);
</script>
</head>
<body>
</body>
</html>
🖥️ 결과
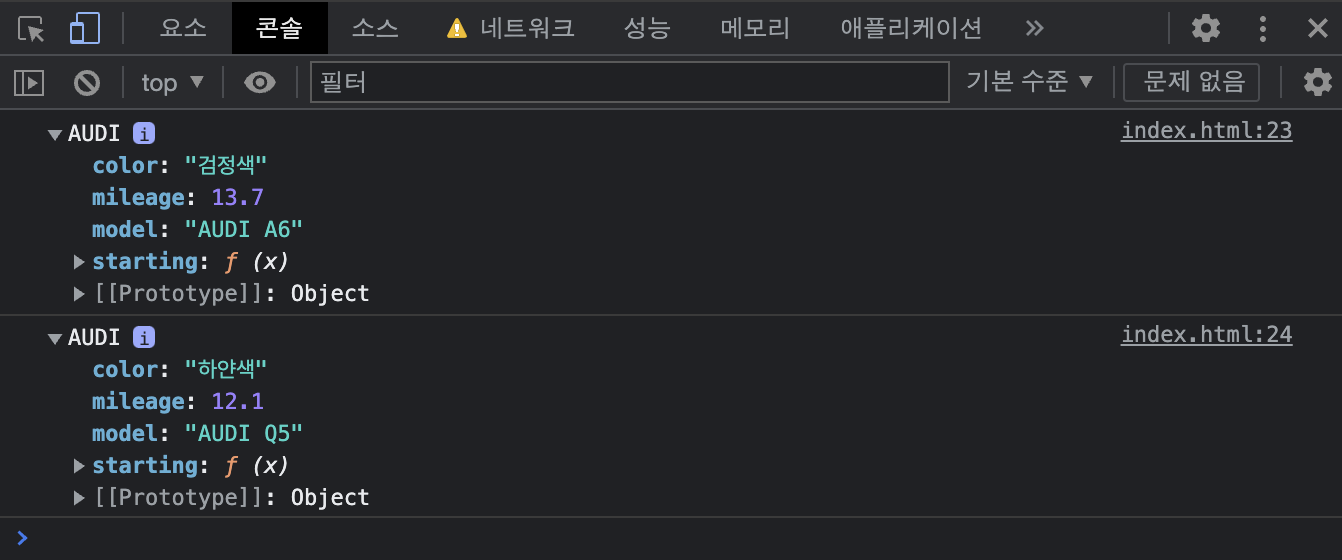
- 모든 자바스크립트 객체는 프로토타입의 속성과 메서드를 상속한다.
- 동일한 생성자 함수로 생성된 객체들은 내부적으로 [proto]라는 속성을 사용하여 생성자 함수에 존재하는 prototype이라는 속성을 참조하여 같은 공간을 공유한다.
✏️ prototype으로 빼기
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<title>JS 객체</title>
<script>
function AUDI(model, mileage){
this.model = model;
this.mileage = mileage;
}
AUDI.prototype.color = 'Black;
AUDI.prototype.starting = function starting(x){
if(x == 'on'){
alert(this.color + '인 ' + this.model + '가 시동이 켜졌습니다.');
}else if(x == 'off'){
alert(this.color + '인 ' + this.model + '가 시동이 꺼졌습니다.');
}
}
var hyunCar = new AUDI('AUDI A6',13.7);
var chanCar = new AUDI('AUDI Q5',12.1);
console.log(hyunCar);
console.log(chanCar);
</script>
</head>
<body>
</body>
</html>
- color가 Black으로 같다고 보고, 시동을 키는 것도 공통 메서드로 프로토타입으로 뺐다.
🖥️ 결과
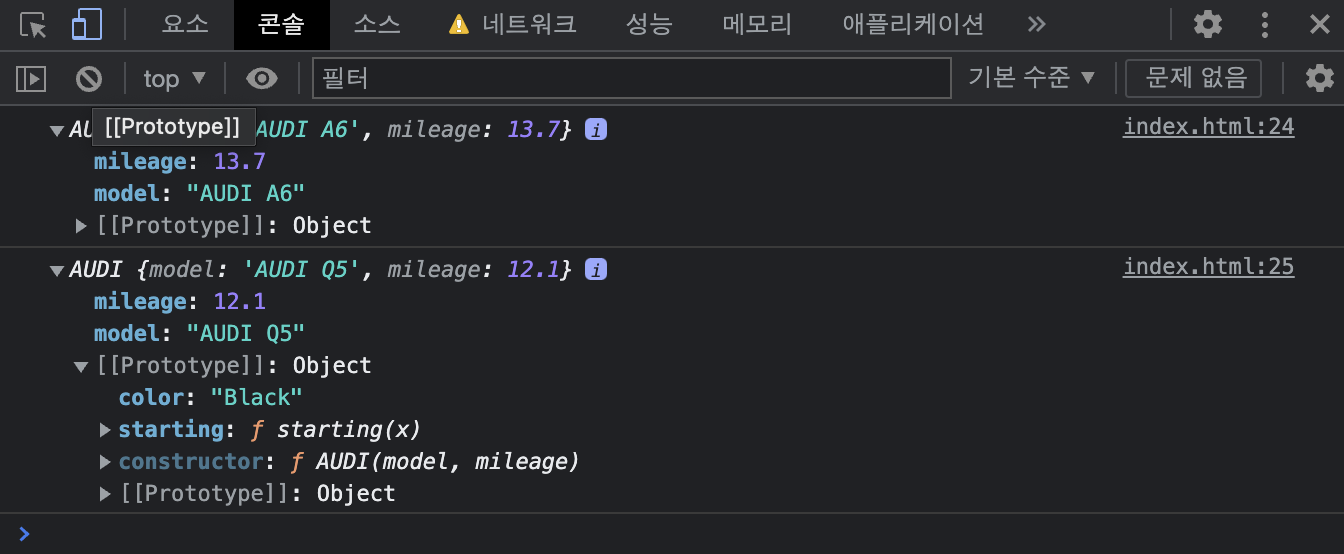
- 호출은 생성자 함수 내부의 코드만 호출이 되고, 나머지는 프로토타입으로 들어간다.
- 이렇게 되면 호출시 바뀌는 값만 찾기 때문에 메모리 절감이 된다.
📃 문제
- 학생들의 국어, 영어, 수학 점수를 통해 평균을 구하여라. (단, 객체로 생성하라.)
✏️ 코드
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<title>JS 객체</title>
<script>
function Score(name,kor,eng,math){
this.name = name;
this.kor = kor;
this.eng = eng;
this.math = math;
}
Score.prototype.avg = function avg(){
return "학생이름 : " + this.name + '<br>' + '평균 : ' + ((this.kor + this.eng + this.math) / 3).toFixed(2) + '<br>' + '===============<br>';
}
var student01 = new Score('배주현',90,80,75);
var student02 = new Score('변백현',100,80,90);
var student03 = new Score('정수정',100,90,100);
document.write(student01.avg());
document.write(student02.avg());
document.write(student03.avg());
</script>
</head>
<body>
</body>
</html>
- 각각 학생의 이름과 국어, 영어, 수학점수는 모두 다르기 때문에 각각 받도록 작성하였다.
- 평균은 그 점수로 인한 결과이므로 어떤 학생이든지 수식이 같으므로 prototype으로 작성하여였다.
🖥️ 결과
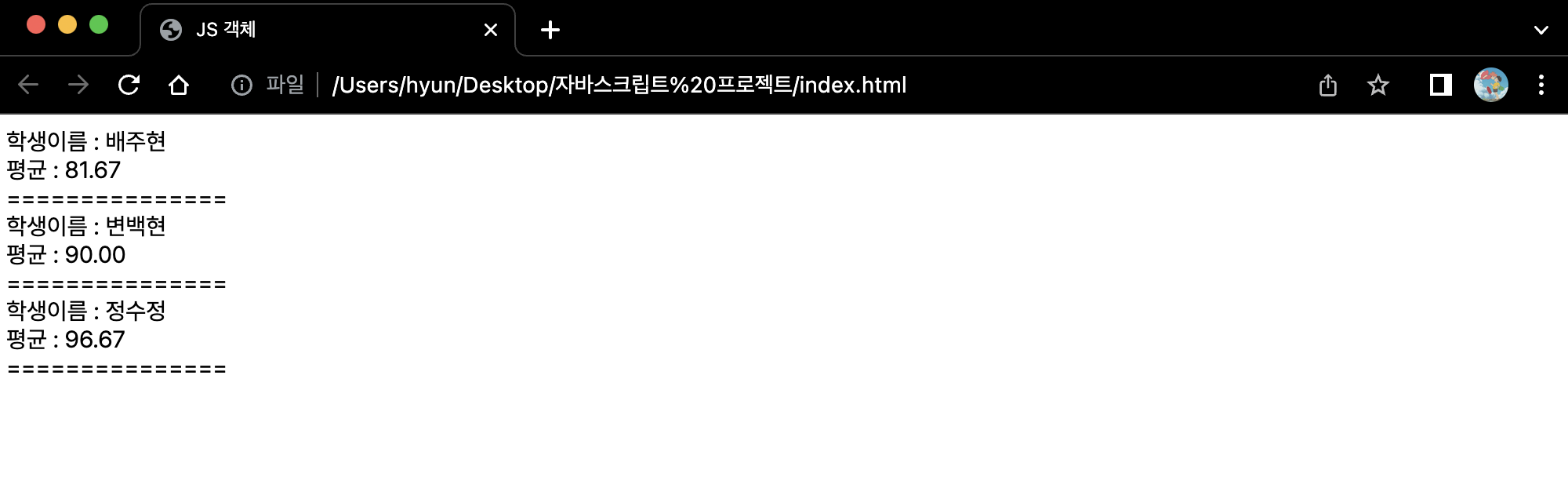