Stack과 Queue
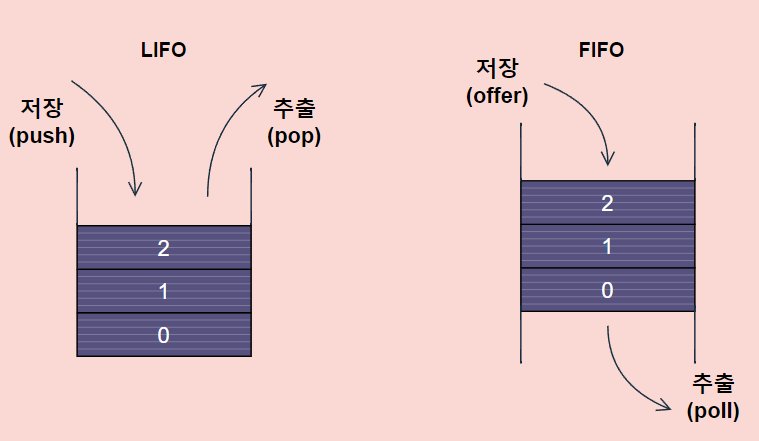
1. Stack
- 스택은 마지막에 저장한 데이터를 가장 먼저 꺼내게 되는 후입선출 LIFO(Last In First Out)구조
- 구현하기 위해선 ArrayList와 같은 배열기반 컬렉션 클래스가 적합
- JAVA에서는 Stack클래스로 구현해 제공
1-1. Stack의 메서드
Method | Description |
---|
boolean empty() | Stack이 비어있는지 알려준다. |
Object peek() | Stack의 맨 위에 저장된 객체를 반환 pop()과 달리 Stack에서 객체를 꺼내지는 않음. 비어있으면 EmptyStackException 발생 |
Object pop() | Stack의 맨 위에 저장된 객체를 꺼낸다. 객체를 스택에서 제거. 비어있으면 EmptyStackException 발생 |
Object push(Object item) | Stack에 객체를 저장한다. |
int search(Object o) | Stack에서 주어진 객체(o)를 찾아서 그 위치를 반환, 못찾으면 -1을 반환 (배열과 달리 위치는 0이 아닌 1부터 시작) |
1-2. Stack의 활용
- 수식 계산, 웹브라우저의 뒤로/앞으로, 웨드프로세서의 undo/redo
import java.util.Stack;
class Coin1 {
private int value;
public Coin1(int value) {
this.value = value;
}
public int getValue() {
return value;
}
}
public class StackExample {
public static void main(String[] args) {
Stack<Coin1> coinBox = new Stack<Coin1>();
coinBox.push(new Coin1(100));
coinBox.push(new Coin1(50));
coinBox.push(new Coin1(500));
coinBox.push(new Coin1(10));
while (!coinBox.isEmpty()) {
Coin1 coin = coinBox.pop();
System.out.println("꺼내온 동전 : " + coin.getValue() + "원");
}
}
}
2. Queue
- 큐는 처음에 저장한 데이터를 가장 먼저 꺼내게 되는 선입선출 FIFO(First In First Out)구조
- 구현하기 위해선 데이터의 추가/삭제가 쉬운 LinkedList가 적합
- JAVA에서는 interface로만 정의해 놓았을 뿐 별도의 클래스를 제공하지 않아, Queue 인터페이스를 구현한 클래스들 중 하나를 선택해서 사용하면 된다. 아래 All known Implementing Classes에 적혀 있는 클래스에서 골라
Queue q =new LinkedList();
와 같은 식을 객체를 생성해서 사용하면 된다.
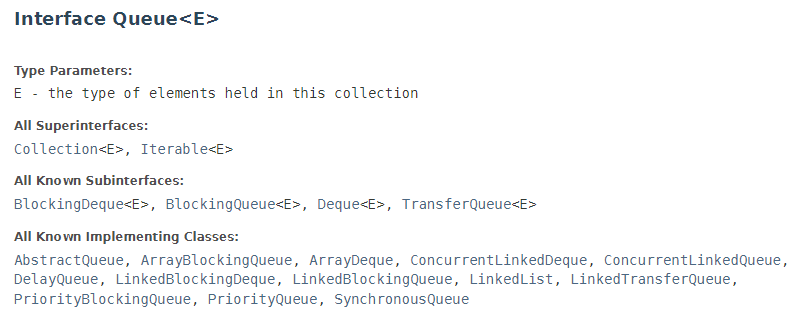
2-1. Que의 메서드
Method | Description |
---|
boolean add(Object o) | 지정된 객체를 Queue에 추가한다. 저장 공간이 부족하면 IllegalStateException 발생 |
boolean offer(Object o) | Queue에 객체를 저장. 성공하면 true, 실패하면 false |
Object remove() | Queue에서 객체를 꺼내 반환. 비어있으면 NoSuchElementException 발생 |
Object poll() | Queue에서 객체를 꺼내서 반환, 비어있으면 null 반환 |
Object peek() | 삭제없이 요소를 읽어온다. Queue가 비었을 때 null을 반환 |
Object element() | 삭제없이 요소를 읽어온다. peek과 달리 Queue가 비었을 때 NoSuchElementException 발생 |
2-2. Queue의 활용
- 최근 사용문서, 인쇄작업 대기목록. 버퍼(buffer)
import java.util.LinkedList;
import java.util.Queue;
class Message1 {
public String command;
public String to;
public Message1(String command, String to) {
this.command = command;
this.to = to;
}
}
public class QueueExample {
public static void main(String[] args) {
Queue<Message1> messageQueue = new LinkedList<Message1>();
messageQueue.offer(new Message1("sendMail", "홍길동"));
messageQueue.offer(new Message1("sendSMS", "홍길순"));
messageQueue.offer(new Message1("sendKakaotalk", "홍두께"));
while (!messageQueue.isEmpty()) {
Message1 message = messageQueue.poll();
switch (message.command) {
case "sendMail":
System.out.println(message.to + "님에게 메일을 보냅니다.");
break;
case "sendSMS":
System.out.println(message.to + "님에게 SMS를 보냅니다.");
break;
case "sendKakaotalk":
System.out.println(message.to + "님에게 카카오톡를 보냅니다.");
break;
}
}
}
}
📖 참고 문헌
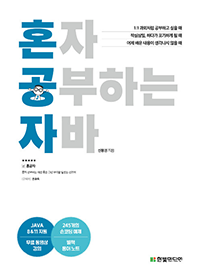