type
type Admin = {
name:string;
privileges: string[];
};
type Emp = {
name: string;
startDate: Date;
};
type ElevatedEmp = Admin & Emp;
const e1 : ElevatedEmp = {
name: '정우',
privileges: ['create-server'],
startDate: new Date(),
}
- type을
&
연산자로 연결하여 새로운 타입을 지정할 수 있다.
interface Admin {
name:string;
privileges: string[];
};
interface Emp {
name: string;
startDate: Date;
};
interface ElevatedEmp extends Admin, Emp
const e1 : ElevatedEmp = {
name: '정우',
privileges: ['create-server'],
startDate: new Date(),
}
type의 & 연산
- 만약 당신이 type에 union 타입을 지정하였고
&
연산자를 사용한다면 교집합에 해당되는 부분만 적용된다.
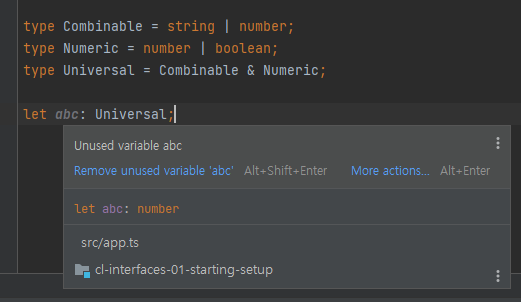
type guard
typeof
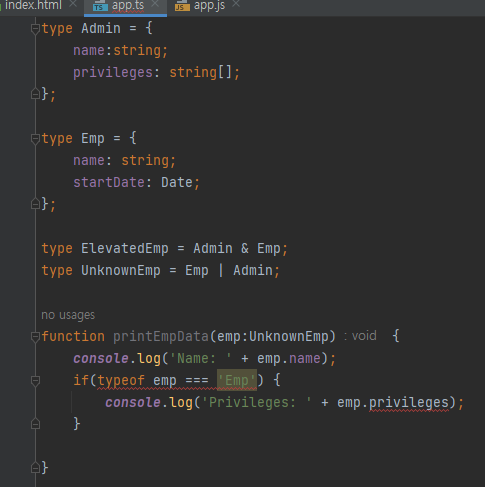
- 위 코드에서 typeof의 값은 어디까지나 runtime 중인 JS에서 돌아간다는 것을 숙지해야한다. 이는 custom type은 안 되고 object, string, number, 등등 기본적으로 JS가 갖고있는 type만 typeof로 걸러낼 수 있다.
그렇다면 custom 타입의 유효성을 검사하는 다른 방법은 없을까?
in
- 놀랍게도 JS에 built in 메서드이며 해당 obj에 지정한 속성값이 들어있는지 check해주는 메서드이다.
type Admin = {
name:string;
privileges: string[];
};
type Emp = {
name: string;
startDate: Date;
};
type ElevatedEmp = Admin & Emp;
type UnknownEmp = Emp | Admin;
function printEmpData(emp:UnknownEmp) {
console.log('Name: ' + emp.name);
if('privileges' in emp) {
console.log('Privileges: ' + emp.privileges);
}
}
- 하지만 이 in 메서드도 한가지 단점이 있다 => 오타를 내면 안 된다는 것. 그렇다면 또 더 좋은 방법은 없을까?
- 또 하지만 type이 아닌interface로 설정할 땐 매우 용이하다.
instanceof
class Car {
drive() {
console.log('Driving...');
}
}
class Truck {
drive() {
console.log('Driving a tuck...');
}
loadCargo(amount: number) {
console.log('Loading cargo...' + amount);
}
}
type Vehicle = Car | Truck;
const v1 = new Car();
const v2 = new Truck();
function useVehicle(vehicle: Vehicle) {
vehicle.drive();
if (vehicle instanceof Truck) {
vehicle.loadCargo(1000);
}
}
- 바로 instanceof 메서드가 있다. in과 비슷한 메서드로 instanceof의 오른편에 나오는 truck 타입은 class로 생성자 함수 (
new Tuck()
) 이 해당 class와 이어주기 때문에 JS에서 인식이 가능하다.
Discriminated Unions
- 개념은 모든 interface에 type이나 어떠한 공통된 속성을 추가하여 해당 속성값을 유효성 검사를 통하여 보다 안정적으로 로직을 짤 수 있다.
interface Bird {
type: 'bird';
flyingSpeed: number;
}
interface Horse {
type: 'horse';
runningSpeed: number;
}
type Animal = Bird | Horse;
function moveAnimal(animal: Animal) {
let speed;
switch (animal.type){
case "bird":
speed = animal.flyingSpeed;
break;
case "horse":
speed = animal.runningSpeed;
break;
}
console.log(speed);
}
moveAnimal({type: 'bird', flyingSpeed: 100});