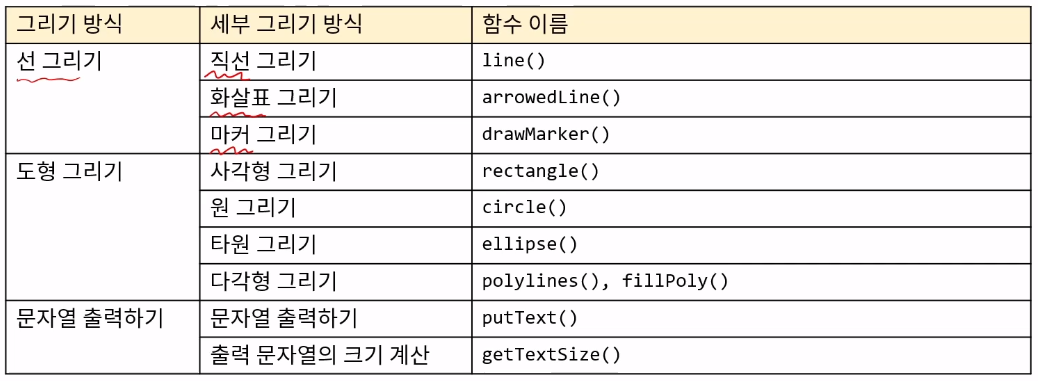
1. 선 그리기
직선그리기
void line(InputOutputArray img, Point pt1, Point pt2, const Scalar& color, int thickness = 1,
int lineType = LINE_8, int shift = 0);
- img: 입출력 영상
- pt1: 시작점 좌표
- pt2: 끝점 좌표
- color: 선 색상
- thickness: 선 두께
- lineType: 선 타입. LINE_4, LINE_8, LINE_AA 중 하나 지정.
- shift: 그리기 좌표값 축소 비율.
2. 도형 그리기
사각형 그리기
void rectangle(InputOutputArray img, Rect rec, const Scalar& color, int thickness = 1,
int lineType = LINE_8, int shift = 0);
- img: 입출력 영상
- rec: 사각형 위치정보(x,y,w,h)
- color: 선 색상
- thickness: 선 두께(음수면 내부를 채움)
- lineType: 선 타입. LINE_4, LINE_8, LINE_AA 중 하나 지정.
- shift: 그리기 좌표값 축소 비율.
원 그리기 함수
void circle(InputOutputArray img, Point center, int radius, const Scalar& color,
int thickness = 1, int lineType = LINE_8, int shift = 0);
- img: 입출력 영상
- center: 원 중심좌표
- radius: 원 반지름
- color: 선 색상
- thickness: 선 두께(음수면 내부를 채움)
- lineType: 선 타입. LINE_4, LINE_8, LINE_AA 중 하나 지정.
- shift: 그리기 좌표값 축소 비율.
다각형 그리기
void polylines(InputOutputArray img, InputArrayOfArrays pts, bool isClosed, const Scalar& color,
int thickness = 1, int lineType = LINE_8, int shift = 0);
- img: 입출력 영상
- pts: 다각형 외곽선 점들의 집합 vector
- isClosed: ture면 시작점과 끝점을 서로 이음
- color: 선 색상
- thickness: 선 두께(음수면 내부를 채움)
- lineType: 선 타입. LINE_4, LINE_8, LINE_AA 중 하나 지정.
- shift: 그리기 좌표값 축소 비율.
3. 문자열 출력하기
void putText(InputOutArray img, const String& text, Point org, int fontFace,
double fontScale, Scalar color, int thickness = 1, int lineType = LINE_8,
bool bottomLeftOrigin = false);
- img: 문자열을 출력할 영상
- text: 출력할 문자열
- org: 문자열이 출력될 좌측하단 좌표
- fontFace: 폰트종류
- fontScale: 폰트크기지정
- color: 문자열 색상
- thickness: 폰트 두께
- lineType: 선 타입. LINE_4, LINE_8, LINE_AA 중 하나 지정.
실습코드
int main()
{
VideoCapture cap("../../data/test_video.mp4");
if (!cap.isOpened()) {
cerr << "Video open failed!" << endl;
return -1;
}
Mat frame;
while (true) {
cap >> frame;
if (frame.empty()) {
cerr << "Empty frame!" << endl;
break;
}
line(frame, Point(570, 280), Point(0, 560), Scalar(255, 0, 0), 2);
line(frame, Point(570, 280), Point(1024, 720), Scalar(255, 0, 0), 2);
int pos = cvRound(cap.get(CAP_PROP_POS_FRAMES));
String text = format("frame number: %d", pos);
putText(frame, text, Point(20, 50), FONT_HERSHEY_SIMPLEX,
0.7, Scalar(0, 0, 255), 1, LINE_AA);
imshow("frame", frame);
if (waitKey(10) == 27)
break;
}
cap.release();
destroyAllWindows();
}
결과영상
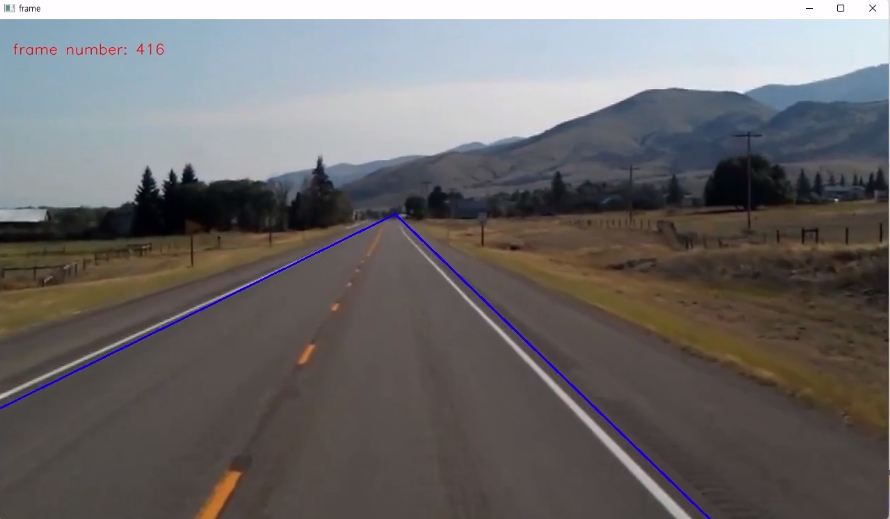