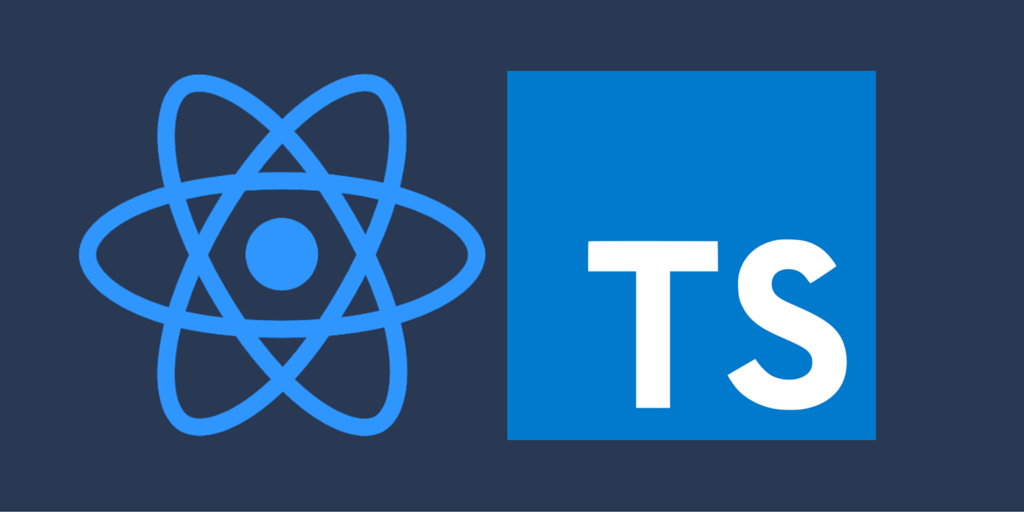
- Provides static type checking and get to learn about potential bugs as one is typing the code without the need to detect the bugs at runtime.
- Provides a way to describe the shape of an object and therefore provide better documentation and autocompletion.
- Provides maintenance and refactoring easier when it comes to large code bases.
Props
Basic props
import { Greet } from './components/Greet'
import { Person } from './components/Person'
import { PersonList } from './components/PersonList'
function App() {
const personName = {
first: 'Bruce',
last: 'Wayne',
}
const nameList = {
{
first: 'Bruce',
last: 'Wayne',
},
{
first: 'Jay',
last: 'Lays',
},
{
first: 'Clark',
last: 'Kent',
},
}
return (
<div className='App'>
<Greet name='John' messageCount={10} isLoggedIn={true} />
<Person name={personName} />
</div>
)
}
String / number / boolean
type GreetProps = {
name: string
messageCount: number
isLoggedIn: boolean
}
export const Greet = (props: GreetProps) => {
return (
<div>
{props.isLoggedIn ? (
<h2>
Hey {props.name}! You have {messageCount} unread messages
</h2>
) : (
<h2>Welcome Guest</h2>
)}
</div>
)
}
Object
import { PersonProps } from './Person.types'
export const Person = (props: PersonProps) => {
return (
<h2>
{props.name.first} {props.name.last}
</h2>
)
}
export type Name = {
first: string
last: string
}
export type PersonProps = {
name: Name
}
Array
import { Name } from './Person.types'
type PersonListProps = {
names: Name[]
}
export const PersonList = (props: PersonListProps) => {
return (
<div>
{props.names.map(name => {
return (
<h2 key={name.first}>
{name.first} {name.last}
</h2>
)
})}
</div>
)
}
export type Name = {
first: string
last: string
}
export type PersonProps = {
name: Name
}