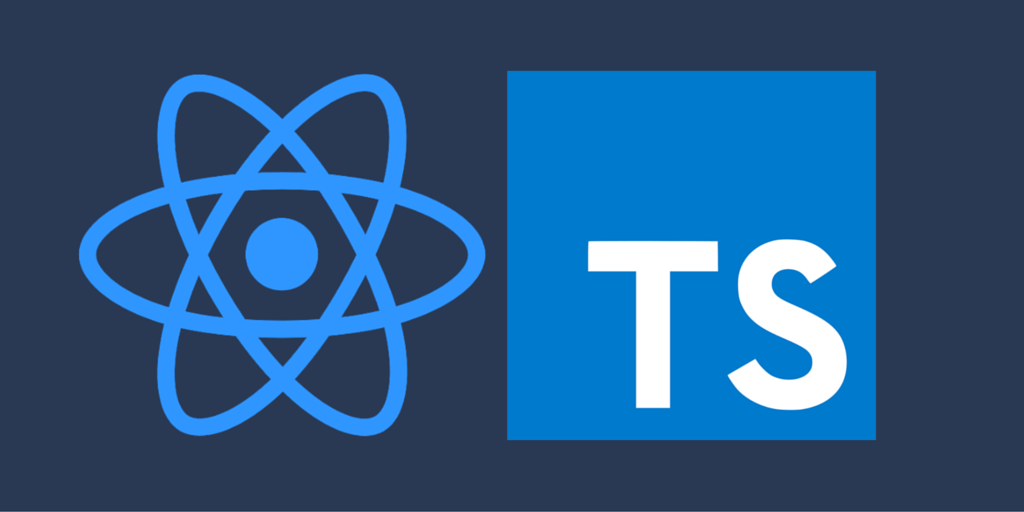
Advanced Props
import { Status } from './components/Status'
import { Heading } from './components/Heading'
import { Oscar } from './components/Oscar'
import { Greet } from './components/Greet'
function App() {
return (
<div className='App'>
<Status status='loading' />
<Heading>Placeholder text</Heading>
<Oscar>
<Heading>Actor name</Heading>
</Oscar>
<Greet name='John' isLoggedIn={false} />
</div>
)
}
Union of string literals
type StatusProps = {
status: 'loading' | 'success' | 'error'
}
export const Status = (props: StatusProps) => {
let message
if (props.status === 'loading') {
message = 'Loading...'
} else if (props.status === 'success') {
message = 'Data fetched successfully!'
} else if (props.status === 'error') {
message = 'Error fetching data'
}
return <h2>Status - {message}</h2>
}
Children props
type HeadingProps = {
children: string
}
export const Heading = (props: HeadingProps) => {
return <h2>{props.children}</h2>
}
Children as react component node
type OscarProps = {
children: React.ReactNode
}
export const Oscar = (props: OscarProps) => {
return <div>{props.children}</div>
}
Optional type
type GreetProps = {
name: string
messageCount?: number
isLoggedIn: boolean
}
export const Greet = (props: GreetProps) => {
const { messageCount = 0 } = props
return (
<div>
{props.isLoggedIn ? (
<h2>
Hey {props.name}! You have {messageCount} unread messages
</h2>
) : (
<h2>Welcome Guest</h2>
)}
</div>
)
}