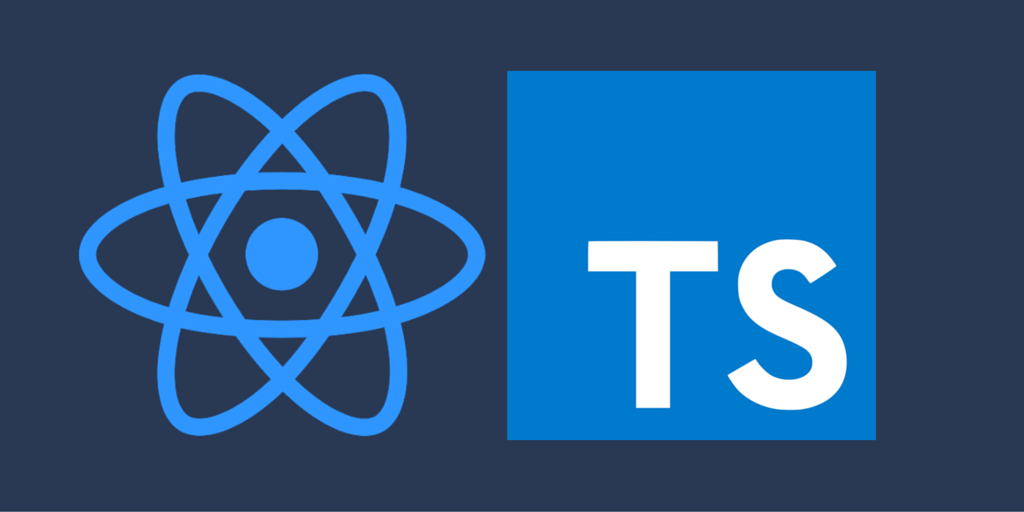
Event props
import { Button } from './components/Button'
import { Input } from './components/Input'
function App() {
return (
<div className='App'>
<Button handleClick={(event, id) => {
console.log('Button clicked', event, id)
}}
/>
<Input value='' handleChange={event => console.log(event)} />
</div>
)
}
Mouse event
type ButtonProps = {
handleClick: (event: React.MouseEvent<HTMLButtonElement>, id: number) => void
}
export const Button = (props: ButtonProps) => {
return <button onClick={event => props.handleClick(event, 1)}>Click</button>
}
Change event
type InputProps = {
value: string
handleChange: (event: React.ChangeEvent<HTMLInputElement>) => void
}
export const Input = ({ value, handleChange }: InputProps) => {
return <input type='text' value={value} onChange={handleChange} />
}