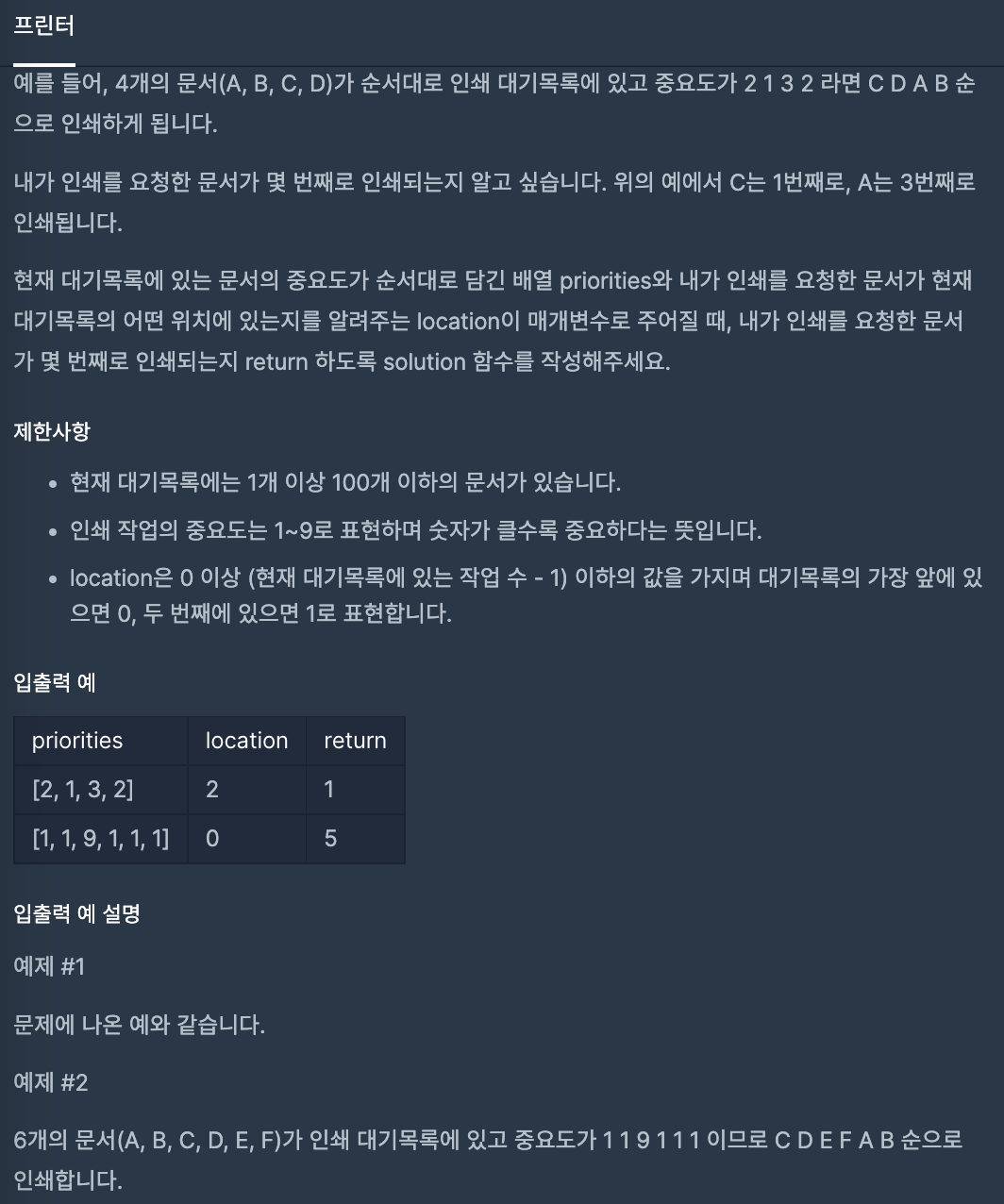
import java.util.*;
class Paper {
int location;
int priority;
public Paper (int location, int priority) {
this.location = location;
this.priority = priority;
}
}
class Solution {
public int solution(int[] priorities, int location) {
int answer = 0;
Queue<Paper> q = new LinkedList<>();
for (int i=0; i<priorities.length; i++) {
q.offer(new Paper(i, priorities[i]));
}
while (!q.isEmpty()) {
Paper target = q.poll();
for (Paper x: q) {
if (x.priority > target.priority) {
q.offer(target);
target = null;
break;
}
}
if (target != null) {
answer++;
if (target.location == location) {
return answer;
}
}
}
return answer;
}
}