WELCOME
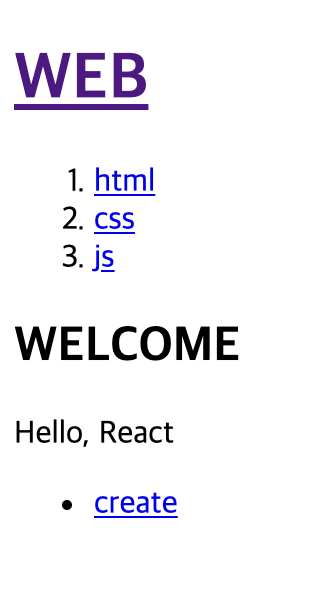
CREATE
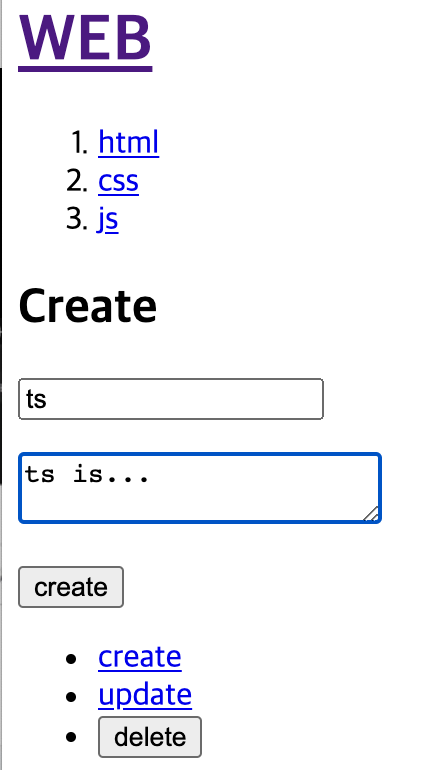
READ
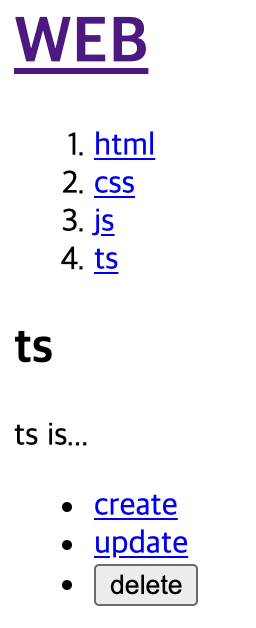
UPDATE
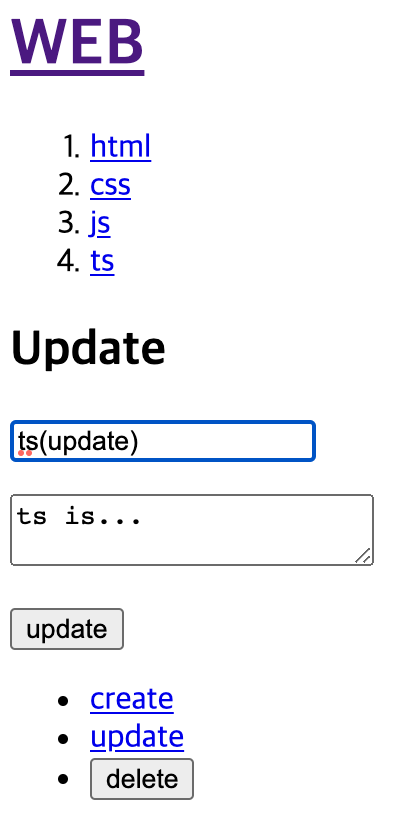
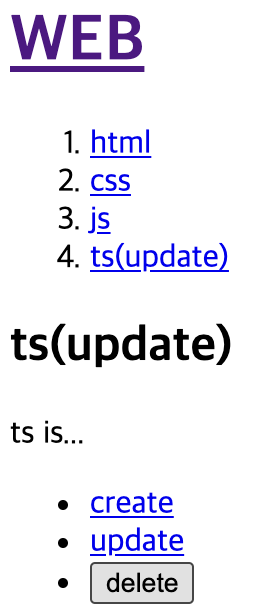
DELETE
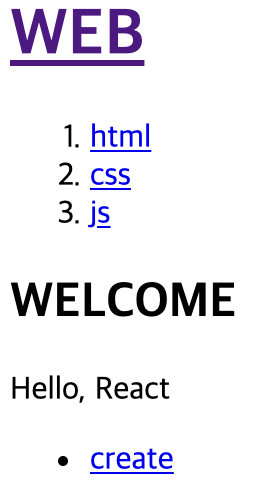
function ComponentTest({children}){
return (
<>
{children}
</>
)
}
function Header({onChangeMode}){
function clickHandler(e) {
e.preventDefault();
onChangeMode('WELCOME');
}
return (
<header>
<h1><a href="index.html" onClick={clickHandler}>WEB</a></h1>
</header>
)
}
function Nav({data, onChangeMode}) {
function clickHandler(e){
e.preventDefault();
onChangeMode("READ", Number(e.target.dataset.id));
}
return (
<nav>
<ol>
{data.map(li =>
<li key={li.id}><a href={`/read/${li.id}`} data-id={li.id} onClick={clickHandler}>{li.title}</a></li>
)}
</ol>
</nav>
);
}
function Article({title, content}) {
return (
<article>
<h2>{title}</h2>
{content}
</article>
);
}
function Control({onChangeMode, id}) {
function clickHandler(e, mode){
e.preventDefault();
onChangeMode(mode, id);
}
let contextUI = null;
if(id > 0) contextUI =
<>
<li><a href="/update" onClick={(e) => clickHandler(e, "UPDATE")}>update</a></li>
<li>
<form onSubmit={e => {
e.preventDefault();
onChangeMode('DELETE', id);
}}>
<input type="submit" value="delete" />
</form>
</li>
</>
return (
<ul>
<li><a href="/create" onClick={(e) => clickHandler(e, "CREATE")}>create</a></li>
{contextUI}
</ul>
)
}
function Create({onSubmit}) {
function submitHandler(e) {
e.preventDefault();
const title = e.target.title.value;
const body = e.target.body.value;
onSubmit(title, body);
e.target.reset();
}
return (
<article>
<h2>Create</h2>
<form onSubmit={submitHandler}>
<p><input name='title' type='text' placeholder='title' /></p>
<p><textarea name='body' placeholder='body' /></p>
<p><input type='submit' value='create' /></p>
</form>
</article>
)
}
function Update({el, onSubmit}) {
const [title, setTitle] = useState(el.title);
const [body, setBody] = useState(el.body);
function submitHandler(e) {
e.preventDefault();
const title = e.target.title.value;
const body = e.target.body.value;
onSubmit(el.id, title, body);
e.target.reset();
}
return (
<article>
<h2>Update</h2>
<form onSubmit={submitHandler}>
<p><input name='title' type='text' placeholder='title' value={title || ''} onChange={e => setTitle(e.target.value)}/></p>
<p><textarea name='body' placeholder='body' value={body || ''} onChange={e => setBody(e.target.value)} /></p>
<p><input type='submit' value='update' /></p>
</form>
</article>
)
}
function App() {
const [mode, setMode] = useState('WELCOME');
const [customId, setCustomId] = useState(0);
const [nextId, setNextId] = useState(4);
const [topics, setTopics] = useState([
{id: 1, title:'html', body:'html is ...'},
{id: 2, title:'css', body:'css is ...'},
{id: 3, title:'js', body:'js is ...'}]);
function changeModeHandler(mode, id) {
if(mode === 'DELETE'){
setTopics((curTopics) => {
const topics = curTopics.filter(topic => topic.id !== id);
return topics;
});
setMode('WELCOME');
setCustomId(0);
return;
}
setMode(mode);
if(id) setCustomId(id);
}
let articleTag;
if(mode === 'WELCOME'){
articleTag = <Article title='WELCOME' content='Hello, React' />;
} else if(mode === "READ"){
const curTopic = topics.filter(topic => topic.id === customId);
if(curTopic.length > 0){
const {title, body} = curTopic[0];
articleTag = <Article title={title} content={body} />;
}
} else if(mode === 'CREATE'){
function createHandler(title, body) {
setTopics((curTopics) => {
const newTopic = {
id: nextId,
title,
body
};
const topics = [...curTopics, newTopic];
return topics;
});
setMode('READ');
setCustomId(nextId);
setNextId(nextId+1);
}
articleTag = <Create onSubmit={createHandler} />
} else if(mode === 'UPDATE'){
const curTopic = topics.filter(topic => topic.id === customId);
function updateHandler(id, title, body) {
setTopics((curTopics) => {
const topics = curTopics.map(topic => {
if(topic.id === id){
topic.title = title;
topic.body = body;
}
return topic;
})
return topics;
});
setMode('READ');
}
if(curTopic.length > 0){
const {title, body} = curTopic[0];
articleTag = <Update el={{id: customId, title, body}} onSubmit={updateHandler} />
}
}
return (
<ComponentTest>
<Header onChangeMode={changeModeHandler}/>
<Nav data={topics} onChangeMode={changeModeHandler} />
{articleTag}
<Control onChangeMode={changeModeHandler} id={customId} />
</ComponentTest>
);
}