props
- props 를 사용하여 부모 컴포넌트의 데이터를 자식 컴포넌트로 전달할 수 있다.
<template>
<Mybtn :bgColor="bgColor1" />
<Mybtn large />
<Mybtn :bgColor="bgColor2" :color="color1" />
</template>
<script>
import Mybtn from '~/components/MyBtn'
export default {
components: {
Mybtn
},
data() {
return {
bgColor1: 'royalblue',
bgColor2: 'red',
color1: 'black'
}
}
}
</script>
- type과 default 값을 설정할 수 있다.
<template>
<button
type="button"
:style="styleObj"
class="btn"
:class="classObj">
Banana
</button>
</template>
<script>
export default {
props: {
bgColor: {
type: String,
default: 'gray'
},
color: {
type: String,
default: 'white'
},
large: {
type: Boolean,
default: false
}
},
data() {
return {
styleObj: {
backgroundColor: this.bgColor,
color: this.color
},
classObj: {
btn_large: this.large
}
}
}
}
</script>
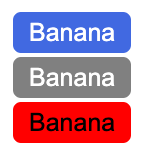
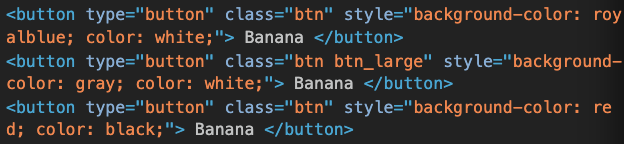