실전에서 유용한 표준 라이브러리
- 내장 함수 : 기본 입출력 함수부터, 정렬 함수까지 기본적인 함수들을 제공한다.
- 파이썬 프로그램을 작성할 때 없어서는 안 되는 필수적인 기능을 포함하고 있다.
- itertools : 파이썬에서 반복되는 형태의 데이터를 처리하기 위한 유용한 기능들을 제공한다.
- 특히 순열과 조합 라이브러리는 코딩 테스트에서 자주 사용된다.
- heapq : 힙 자료구조를 제공한다.
- 일반적으로 우선순위 큐 기능을 구현하기 위해 사용된다.
- bisect : 이진 탐색 기능을 제공한다.
- collections : deque, Counter 등의 유용한 자료구조를 포함한다.
- math : 필수적인 수학적 기능을 제공한다.
- 팩토리얼, 제곱근, 최대공약수, 삼각함수 관련 함수부터, 파이와 같은 상수를 제공한다.
자주 사용되는 내장 함수
res = sum([1, 2, 3, 4, 5])
print(res)
min_res = min(7, 3, 5, 2)
max_res = max(7, 3, 5, 2)
print("min_res is", min_res)
print("max_res is", max_res)
res = eval("(3+5)*7")
print(res)
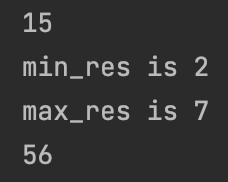
- sum() : 합계
- min() : 최소
- max() : 최대
- eval() : 문자열을 숫자로 인식하여 계산
res = sorted([9, 1, 8, 5, 4])
reverse_res = sorted([9, 1, 8, 5, 4], reverse=True)
print(res)
print(reverse_res)
arr = [('홍길동', 35), ('이순신', 75), ('아무개', 50)]
res = sorted(arr, key=lambda x: x[1], reverse=True)
print(res)
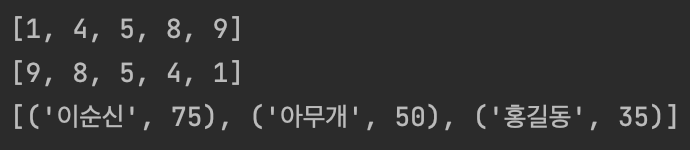
- sorted(list) : 정렬 함수, 기본 값은 오름 차순 정렬
- sorted(list, reverse=True) : 내림 차순 정렬
- sorted(list, key=lambda x: x[n]) : 정렬 with key값
순열과 조합
- 순열(Permutation) : 서로 다른 n개에서, 서로 다른 r개를 선택하여 일렬로 나열하는 것 -> 순서가 상관이 있음
ex) {'A', 'B', 'C'}에서 3P2 : AB, BA, AC, CA, BC, CB
- 조합(Combination) : 서로 다른 n개에서, 순서에 상관 없이 서로 다른 r개를 선택하는 것 -> 순서가 상관이 없음
ex) {'A', 'B', 'C'}에서 3C2 : AB, AC, BC
순열
from itertools import permutations
data = ['A', 'B', 'C']
res = list(permutations(data, 3))
print(res)

- permutations(n개의 원소를 갖는 list, r) : n개에서 r개를 선택하는 순열
조합
from itertools import combinations
data = ['A', 'B', 'C']
res = list(combinations(data, 2))
print(res)

- combinations(n개의 원소를 갖는 list, r) : n개에서 r개를 선택하는 조합
중복 순열과 중복 조합
- 중복 순열 : 원소의 중복을 허용하는, 순열
- 중복 조합 : 원소의 중복을 허용하는, 조합
중복 순열
from itertools import product
data = ['A', 'B', 'C']
res = list(product(data, repeat=2))
print(res)

- product(n개의 원소를 갖는 list, repeat=r) : n개에서 r개를 선택하는 중복 조합
중복 조합
from itertools import combinations_with_replacement
data = ['A', 'B', 'C']
res = list(combinations_with_replacement(data, r=2))
print(res)

- combinations_with_replacement(n개의 원소를 갖는 list, r) : n개에서 r개를 선택하는 중복 순열
Counter
- 등장 횟수를 세는 기능을 제공
- 리스트와 같은 반복 가능한(iterable) 객체가 주어졌을 때, 내부의 원소가 몇 번씩 등장했는지를 알려준다.
from collections import Counter
counter = Counter(['red', 'blue', 'red', 'green', 'blue', 'blue'])
print(counter['blue'])
print(counter['green'])
print(dict(counter))
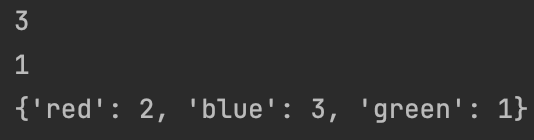
- Counter(list) : Counter 객체를 생성
- dict(Counter 객체) : Counter 객체를 사전 자료형으로 변환
최대 공약수와 최소 공배수
- 최소 공배수는 바로 쓸 수 있는 함수가 없기 때문에, 최대 공약수를 이용하여 구한다.
import math
def lcm(a, b):
return a * b // math.gcd(a, b)
a = 21
b = 14
print(math.gcd(21, 14))
print(lcm(21, 14))
print((lambda a, b: a * b // math.gcd(a, b))(21, 14))
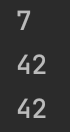