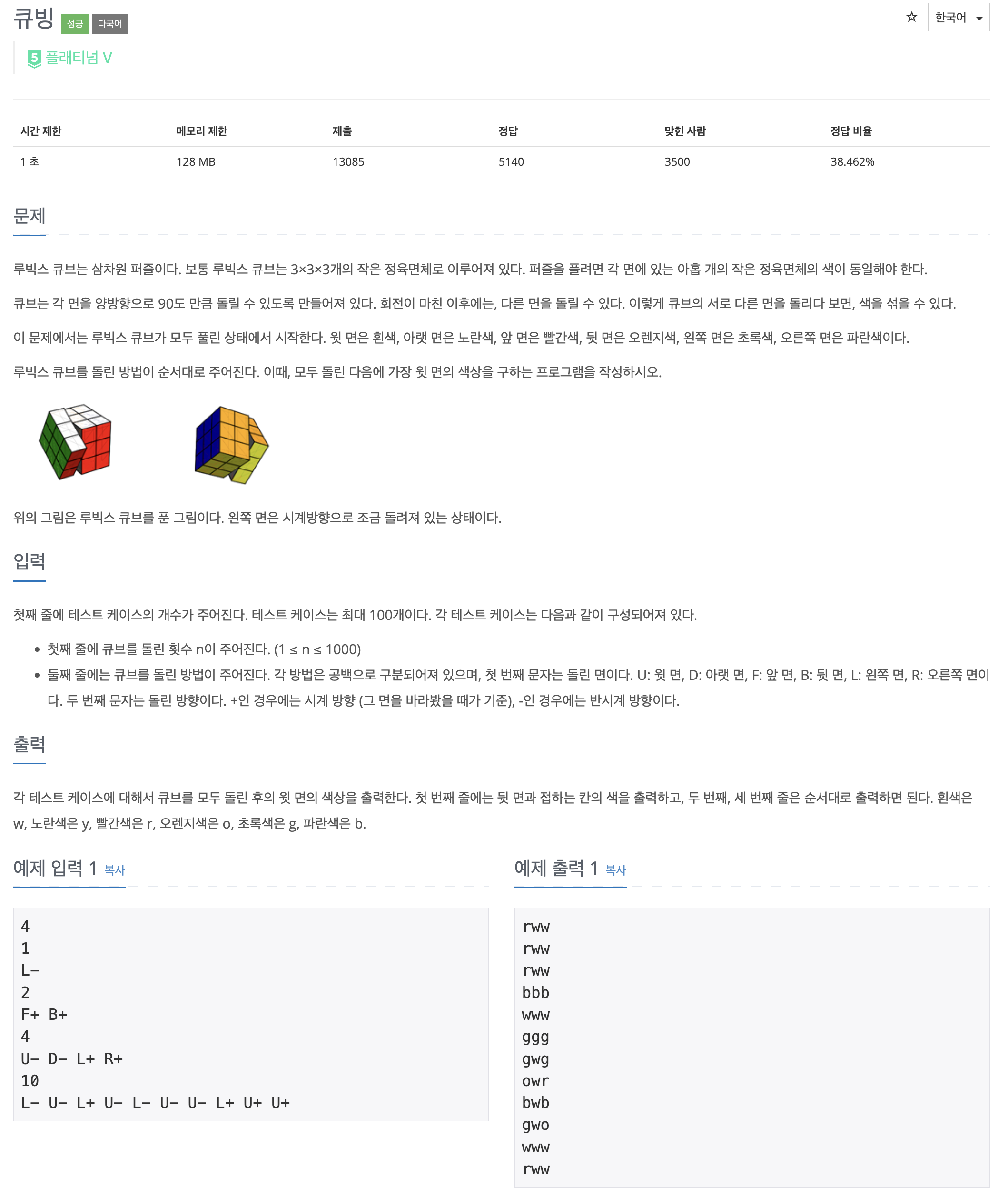
풀이 시간
구현 방식
- 특별한 알고리즘은 없는 빡구현 문제. 모든 연산을 구현해주면 된다
A = reversed(A); A = list(map(list, zip(*A)))
A = list(reversed(list(map(list, zip(*A)))))
- 처음엔 deepcopy를 이용해서 나머지 부분을 구현해주려 했으나 deepcopy의 연산 시간이 짧지가 않아서, 하드코딩을 통해 원소 하나하나마다 swap 해줌
코드
import sys
import copy
def cal_U(direction):
global up
if direction == '+':
up = reversed(up)
up = list(map(list, zip(*up)))
front[0][0], front[0][1], front[0][2], right[0][0], right[0][1], right[0][2], back[0][0], back[0][1], back[0][2], left[0][0], left[0][1], left[0][2] = right[0][0], right[0][1], right[0][2], back[0][0], back[0][1], back[0][2], left[0][0], left[0][1], left[0][2], front[0][0], front[0][1], front[0][2]
elif direction == '-':
up = list(reversed(list(map(list, zip(*up)))))
front[0][0], front[0][1], front[0][2], right[0][0], right[0][1], right[0][2], back[0][0], back[0][1], back[0][2], left[0][0], left[0][1], left[0][2] = left[0][0], left[0][1], left[0][2], front[0][0], front[0][1], front[0][2], right[0][0], right[0][1], right[0][2], back[0][0], back[0][1], back[0][2]
def cal_D(direction):
global down
if direction == '+':
down = reversed(down)
down = list(map(list, zip(*down)))
front[2][0], front[2][1], front[2][2], right[2][0], right[2][1], right[2][2], back[2][0], back[2][1], back[2][2], left[2][0], left[2][1], left[2][2] = left[2][0], left[2][1], left[2][2], front[2][0], front[2][1], front[2][2], right[2][0], right[2][1], right[2][2], back[2][0], back[2][1], back[2][2]
elif direction == '-':
down = list(reversed(list(map(list, zip(*down)))))
front[2][0], front[2][1], front[2][2], right[2][0], right[2][1], right[2][2], back[2][0], back[2][1], back[2][2], left[2][0], left[2][1], left[2][2] = right[2][0], right[2][1], right[2][2], back[2][0], back[2][1], back[2][2], left[2][0], left[2][1], left[2][2], front[2][0], front[2][1], front[2][2]
def cal_F(direction):
global front
if direction == '+':
front = reversed(front)
front = list(map(list, zip(*front)))
right[0][0], right[1][0], right[2][0], up[2][0], up[2][1], up[2][2], left[2][2], left[1][2], left[0][2], down[2][0], down[2][1], down[2][2] = up[2][0], up[2][1], up[2][2], left[2][2], left[1][2], left[0][2], down[2][0], down[2][1], down[2][2], right[0][0], right[1][0], right[2][0]
elif direction == '-':
front = list(reversed(list(map(list, zip(*front)))))
right[0][0], right[1][0], right[2][0], up[2][0], up[2][1], up[2][2], left[2][2], left[1][2], left[0][2], down[2][0], down[2][1], down[2][2] = down[2][0], down[2][1], down[2][2], right[0][0], right[1][0], right[2][0], up[2][0], up[2][1], up[2][2], left[2][2], left[1][2], left[0][2]
def cal_B(direction):
global back
if direction == '+':
back = reversed(back)
back = list(map(list, zip(*back)))
up[0][0], up[0][1], up[0][2], right[0][2], right[1][2], right[2][2], down[0][0], down[0][1], down[0][2], left[2][0], left[1][0], left[0][0] = right[0][2], right[1][2], right[2][2], down[0][0], down[0][1], down[0][2], left[2][0], left[1][0], left[0][0], up[0][0], up[0][1], up[0][2]
elif direction == '-':
back = list(reversed(list(map(list, zip(*back)))))
right[0][2], right[1][2], right[2][2], down[0][0], down[0][1], down[0][2], left[2][0], left[1][0], left[0][0], up[0][0], up[0][1], up[0][2] = up[0][0], up[0][1], up[0][2], right[0][2], right[1][2], right[2][2], down[0][0], down[0][1], down[0][2], left[2][0], left[1][0], left[0][0]
def cal_L(direction):
global left
if direction == '+':
left = reversed(left)
left = list(map(list, zip(*left)))
front[0][0], front[1][0], front[2][0], down[2][2], down[1][2], down[0][2], back[2][2], back[1][2], back[0][2], up[0][0], up[1][0], up[2][0] = up[0][0], up[1][0], up[2][0], front[0][0], front[1][0], front[2][0], down[2][2], down[1][2], down[0][2], back[2][2], back[1][2], back[0][2]
elif direction == '-':
left = list(reversed(list(map(list, zip(*left)))))
up[0][0], up[1][0], up[2][0], front[0][0], front[1][0], front[2][0], down[2][2], down[1][2], down[0][2], back[2][2], back[1][2], back[0][2] = front[0][0], front[1][0], front[2][0], down[2][2], down[1][2], down[0][2], back[2][2], back[1][2], back[0][2], up[0][0], up[1][0], up[2][0]
def cal_R(direction):
global right
if direction == '+':
right = reversed(right)
right = list(map(list, zip(*right)))
up[0][2], up[1][2], up[2][2], front[0][2], front[1][2], front[2][2], down[2][0], down[1][0], down[0][0], back[2][0], back[1][0], back[0][0] = front[0][2], front[1][2], front[2][2], down[2][0], down[1][0], down[0][0], back[2][0], back[1][0], back[0][0], up[0][2], up[1][2], up[2][2]
elif direction == '-':
right = list(reversed(list(map(list, zip(*right)))))
front[0][2], front[1][2], front[2][2], up[0][2], up[1][2], up[2][2], back[2][0], back[1][0], back[0][0], down[2][0], down[1][0], down[0][0] = up[0][2], up[1][2], up[2][2], back[2][0], back[1][0], back[0][0], down[2][0], down[1][0], down[0][0], front[0][2], front[1][2], front[2][2]
T = int(sys.stdin.readline()[:-1])
for t in range(T):
N = int(sys.stdin.readline()[:-1])
up = [['w', 'w', 'w'], ['w', 'w', 'w'], ['w', 'w', 'w']]
down = [['y', 'y', 'y'], ['y', 'y', 'y'], ['y', 'y', 'y']]
front = [['r', 'r', 'r'], ['r', 'r', 'r'], ['r', 'r', 'r']]
back = [['o', 'o', 'o'], ['o', 'o', 'o'], ['o', 'o', 'o']]
left = [['g', 'g', 'g'], ['g', 'g', 'g'], ['g', 'g', 'g']]
right = [['b', 'b', 'b'], ['b', 'b', 'b'], ['b', 'b', 'b']]
method = sys.stdin.readline()[:-1].split()
for n in range(N):
if method[n][0] == 'U': cal_U(method[n][1])
elif method[n][0] == 'D': cal_D(method[n][1])
elif method[n][0] == 'F': cal_F(method[n][1])
elif method[n][0] == 'B': cal_B(method[n][1])
elif method[n][0] == 'L': cal_L(method[n][1])
elif method[n][0] == 'R': cal_R(method[n][1])
for i in range(len(up)):
print(''.join(up[i]))
결과
