Command Line Arguments
- 아래와 같이 script를 수정해서 command line으로 argument를 입력받을 수 있음
uint32_t max_packets = 1;
std::string delay = "2ms";
CommandLine cmd;
cmd.AddValue("howmany", "Number of packets to echo", max_packets);
cmd.AddValue("delay", "Link Delay", delay);
cmd.Parse (argc, argv);
// ...
pointToPoint.SetChannelAttribute ("Delay", String Value (delay));
// ...
echoClient.SetAttribute ("MaxPackets", UintegerValue (max_packets));
- 아래의 명령어 형식으로 simulation 실행
./waf --run "scratch/myfirst --howmany=2 --delay=10ms"
Tracing System
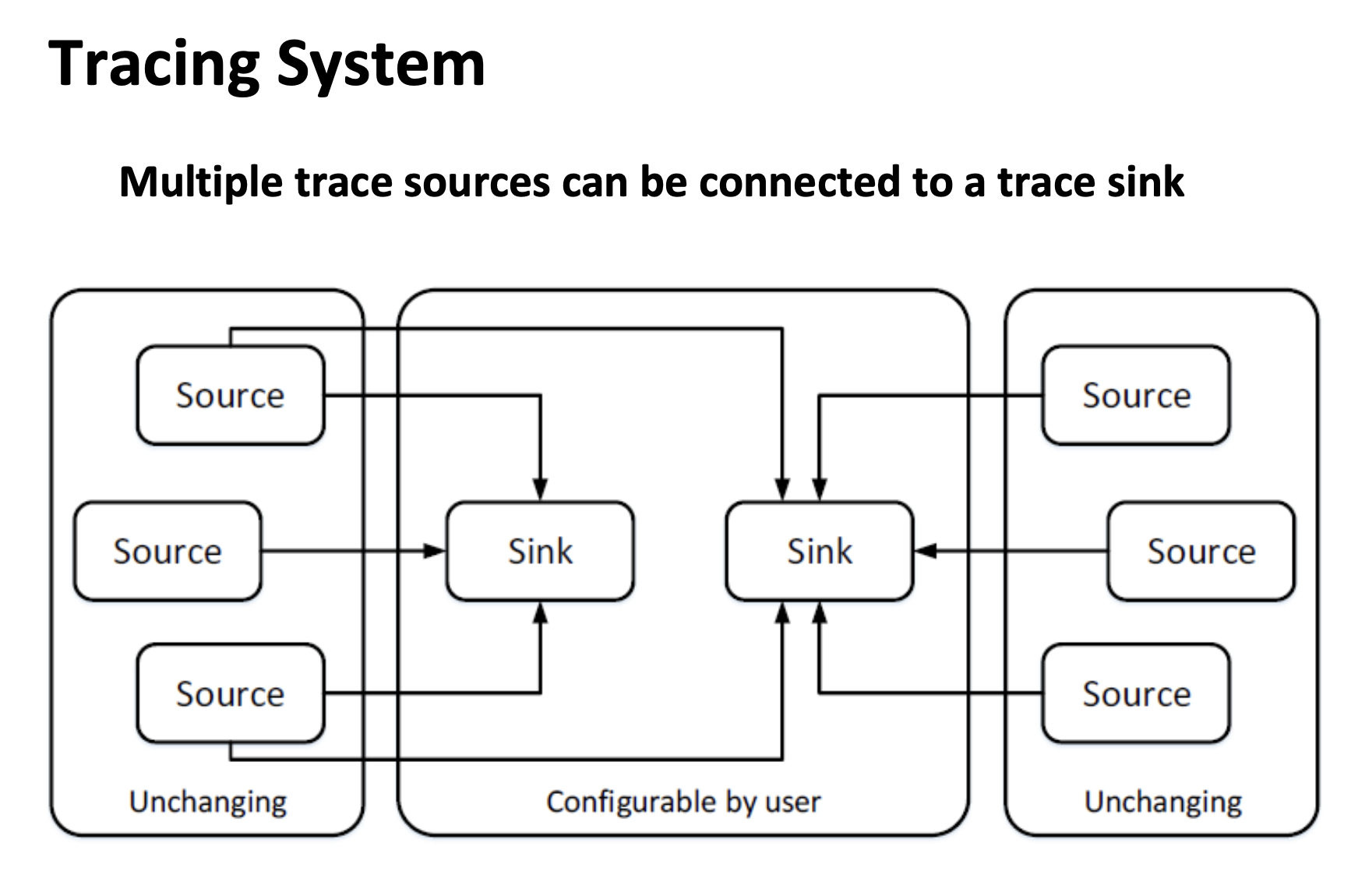
- Generating output after running a simulation
- Measuring the system performance
- Trace source: Signaling events and providing access to interesting data (provider)
- Trace sink: Consuming trace information (consumer)
PCAP tracing (Packet CAPture)
- .pcap file format
- Traffice trace analyze
- Put the following code at the end of the file
pointToPoint.EnablePcapAll("firstcap");
Simulator::Run ();
Simulator::Destroy ();
return 0;
- Result is locate at ns-3.29
./firstcap-1-0.pcap
./firstcap-0-0.pcap
- Reading output using tcpdump

tcpdump -nn -tt -r firstcap-0-0.pcap
Applications in ns-3
- An Application generates packets
- Applications are associated with individual nodes
- Class ns3::Application can be used as a base class for ns3 applications
UdpClientServer
- Applications
- Application Helpers
Attributes for UdpClient
- Interval: The time to wait between pakcets
- RemoteAddress: The destination of the outbound packets
- RemotePort: The destination port of the outbound packets
Attributes for UdpServer
- Port: Port on which we listen for incoming packets
- PacketWindowSize: The size of the window used to compute the packet loss
Functions for Application
- void Application::SetStartTime(Time time);
- void ApplicationContainer::Start(Time start);
- void Application::SetStopTime(Time time);
- void ApplicationContainer::Stop(Time stop);
- virtual void Application::StartApplication(void);
- virtual void Application::StopApplication(void);
UDP Udpclientserverapplication
- 참고) ./ns-allinone-3.29/ns-3.29/examples에 예제 코드 있음
- p2p link: DataRate 10Mbps, Delay 10us (Command Line Arguments, DataRate, Delay)
- Application & flow
- udp-client-server Application (No echo from server)
- UDP flow: node0 -> node1, App. Transmit. Speed: 1Mbps, 1 - 10s
- Generate .pcap file using PCAP trace
- Check .pcap file with TCPDUMP or Wireshark
/* -*- Mode:C++; c-file-style:"gnu"; indent-tabs-mode:nil; -*- */
/*
* This program is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License version 2 as
* published by the Free Software Foundation;
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
// Network topology
//
// n0 n1
// | |
// =======
// LAN
//
// - UDP flows from n0 to n1
#include <fstream>
#include "ns3/core-module.h"
#include "ns3/csma-module.h"
#include "ns3/applications-module.h"
#include "ns3/internet-module.h"
using namespace ns3;
NS_LOG_COMPONENT_DEFINE ("UdpClientServerExample");
int
main (int argc, char *argv[])
{
//
// Enable logging for UdpClient and
//
LogComponentEnable ("UdpClient", LOG_LEVEL_INFO);
LogComponentEnable ("UdpServer", LOG_LEVEL_INFO);
bool useV6 = false;
Address serverAddress;
uint64_t datarate = 10000000; //10Mbps
uint32_t delay = 0.01; //10us
CommandLine cmd;
//cmd.AddValue ("useIpv6", "Use Ipv6", useV6);
cmd.AddValue ("DataRate", "DataRate", datarate);
cmd.AddValue ("Delay", "Delay", delay);
cmd.Parse (argc, argv);
//
// Explicitly create the nodes required by the topology (shown above).
//
NS_LOG_INFO ("Create nodes.");
NodeContainer n;
n.Create (2);
InternetStackHelper internet;
internet.Install (n);
NS_LOG_INFO ("Create channels.");
//
// Explicitly create the channels required by the topology (shown above).
//
CsmaHelper csma;
//csma.SetChannelAttribute ("DataRate", DataRateValue (DataRate (5000000)));
//csma.SetChannelAttribute ("Delay", TimeValue (MilliSeconds (2)));
csma.SetChannelAttribute ("DataRate", DataRateValue (DataRate (datarate)));
csma.SetChannelAttribute ("Delay", TimeValue (MilliSeconds (delay)));
csma.SetDeviceAttribute ("Mtu", UintegerValue (1400));
NetDeviceContainer d = csma.Install (n);
//
// We've got the "hardware" in place. Now we need to add IP addresses.
//
NS_LOG_INFO ("Assign IP Addresses.");
if (useV6 == false)
{
Ipv4AddressHelper ipv4;
ipv4.SetBase ("10.1.1.0", "255.255.255.0");
Ipv4InterfaceContainer i = ipv4.Assign (d);
serverAddress = Address (i.GetAddress (1));
}
else
{
Ipv6AddressHelper ipv6;
ipv6.SetBase ("2001:0000:f00d:cafe::", Ipv6Prefix (64));
Ipv6InterfaceContainer i6 = ipv6.Assign (d);
serverAddress = Address(i6.GetAddress (1,1));
}
NS_LOG_INFO ("Create Applications.");
//
// Create one udpServer applications on node one.
//
uint16_t port = 4000;
UdpServerHelper server (port);
ApplicationContainer apps = server.Install (n.Get (1));
apps.Start (Seconds (1.0));
apps.Stop (Seconds (10.0));
//
// Create one UdpClient application to send UDP datagrams from node zero to
// node one.
//
uint32_t MaxPacketSize = 1024;
Time interPacketInterval = Seconds (0.05);
uint32_t maxPacketCount = 320;
UdpClientHelper client (serverAddress, port);
client.SetAttribute ("MaxPackets", UintegerValue (maxPacketCount));
client.SetAttribute ("Interval", TimeValue (interPacketInterval));
client.SetAttribute ("PacketSize", UintegerValue (MaxPacketSize));
apps = client.Install (n.Get (0));
apps.Start (Seconds (2.0));
apps.Stop (Seconds (10.0));
csma.EnablePcapAll("2019315447");
//
// Now, do the actual simulation.
//
NS_LOG_INFO ("Run Simulation.");
Simulator::Run ();
Simulator::Destroy ();
NS_LOG_INFO ("Done.");
}
- uint64_t 형식으로 datarate 정의
- uint32_t 형식으로 delay 정의
- datarate와 delay를 "DataRate", "Delay"로 command line으로 입력 받도록 설정함
- csma.EnablePcapAll();으로 .pcap 파일 생성하도록 함
- 아래의 명령어로 simulator 실행
./waf --run "scratch/week4_homework --DataRate=10000000 --Delay=0.01"
- 생성된 .pcap 파일 확인
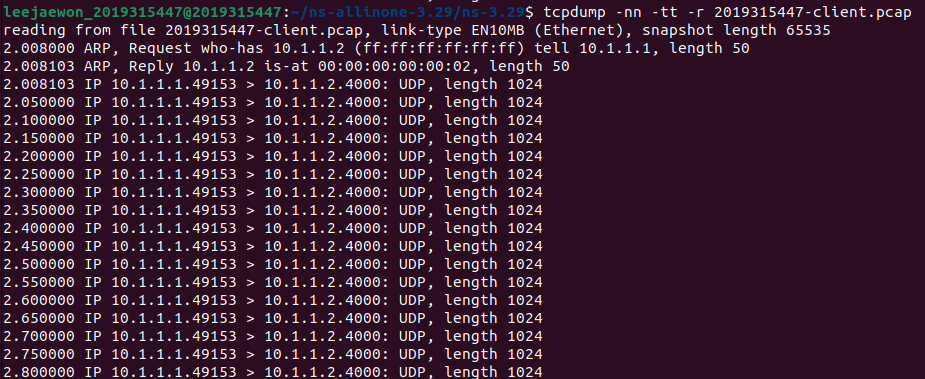