Background
[SW Design and Implementation]
- The software engineering process at which an executable SW system is developed.
- SW design: A creative activity in which you identify SW components and their relationships, based on a customer's requirements.
- Implementation: The process of realizing the design as a program.
- An important implementation decision.
- Deciding whether to build or to buy the application software.
ex) A medical records system -> A package that is already used in hospitals.
- Buy -> How to configure the system product to meet the requirements?
[The goals of this chapter]
- How system modeling and architectural design are put into practice in developing an object-oriented SW design?
- What are the important implementation issues?
- Software reuse
- Configuration management
- Open-source development
Object-oriented design using the UML
[The method for developing a system design]
1. Understanding and defining the context and the external interactions with the system.
2. Designing the system architecture (architectural pattern).
3. Identifying the principal objects in the system. (class diagram)
4. Developing design models.
5. Specifying interfaces.
1. System context and interactions
-
The first stage in any SW design process
- Understanding of the relationships between our SW and its external environment.
(= Setting the system boundaries).
-
System context model (class diagram)
- A structural model that demonstrates the other systems in the environment of the system being developed.
-
Interaction model (use-case diagram)
-A dynamic model that shows how the system interacts with its environment.
[A design for the wilderness weather station system]
-
Weather station
-
Collecting weather data
-
Carrying out some initial data processing.
-
Transmitting the data to the data management system.
-
Structural model (class diagram)
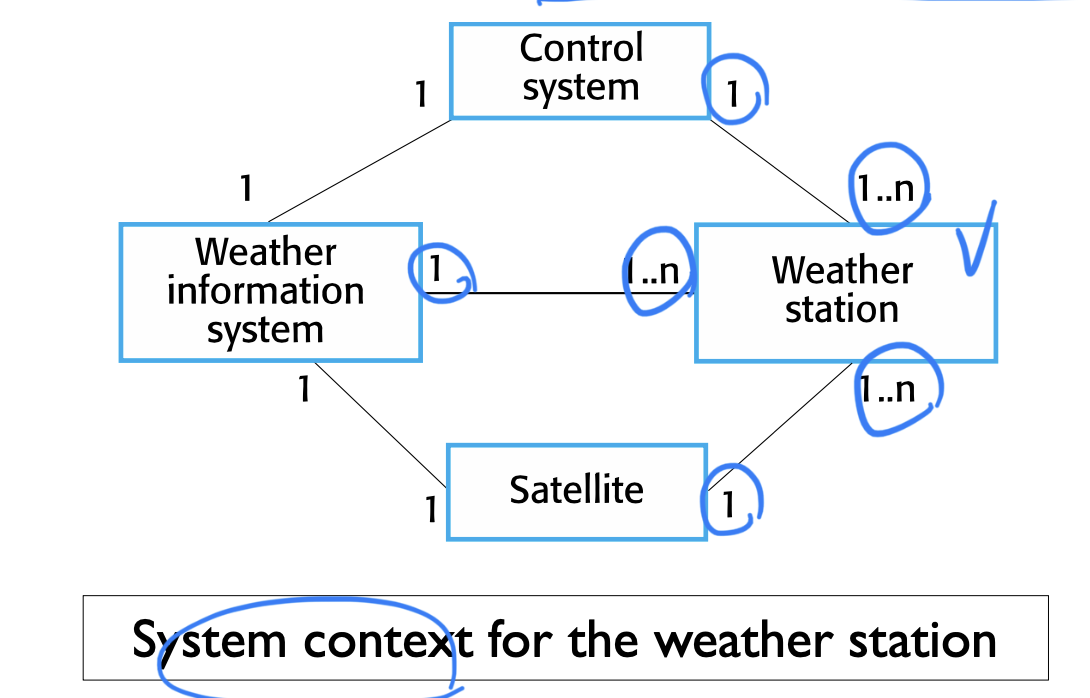
-
Interaction model (use-case diagram)
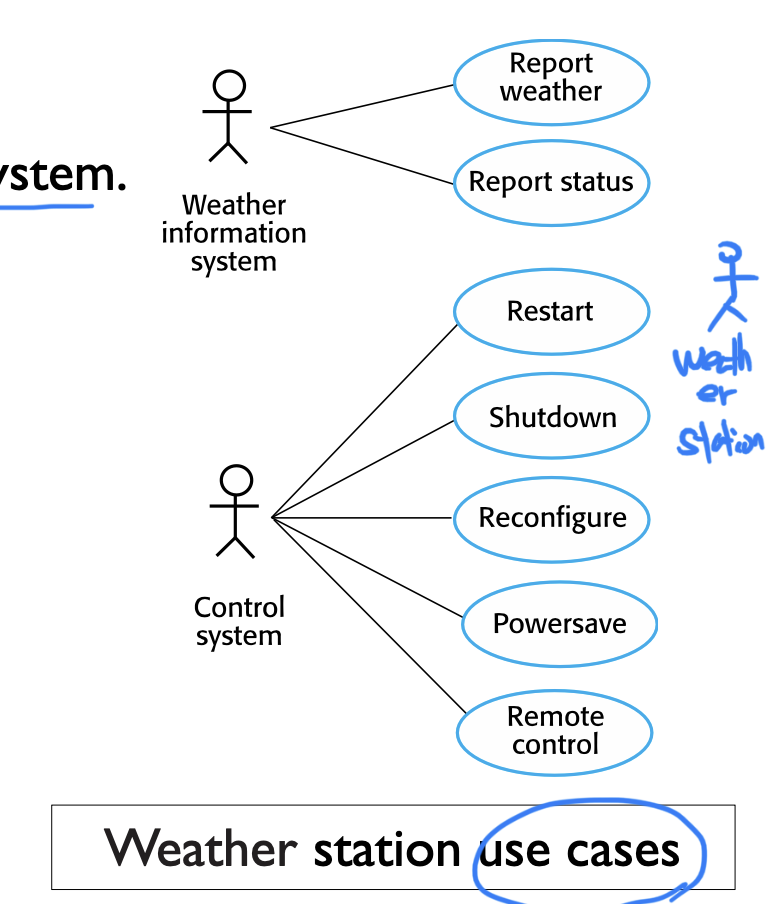
[Weather station use cases]
- Report weather & Report status
- Send weather data to the weather information system.
- Send status information to the weather information system.
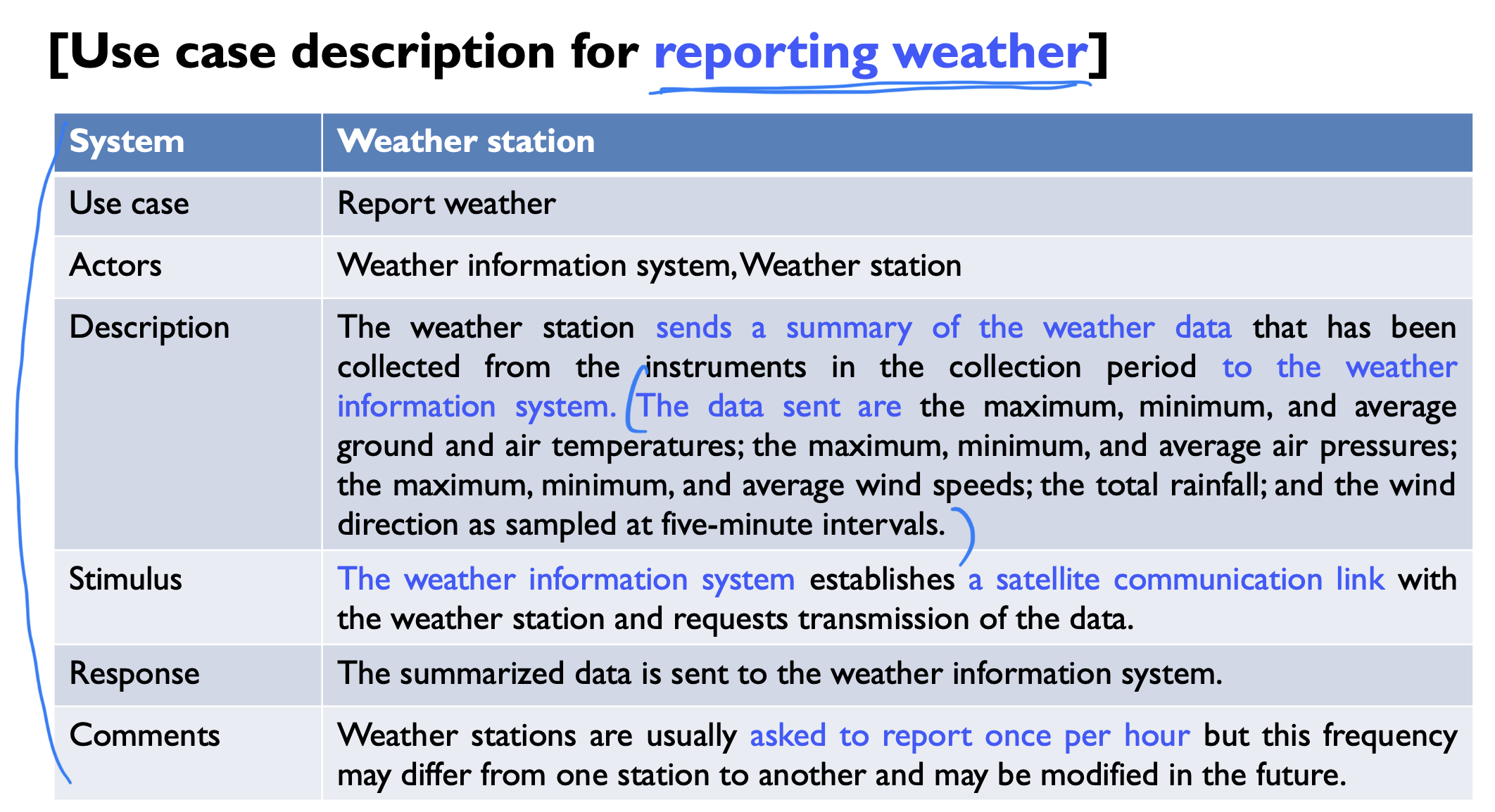
- Restart & Shutdown
- Reconfigure
- Reconfigure the weather station software.
- Powersave
- Put the weather station into power-saving mode.
- Remote control
- Send control commands to any weather station subsystem.
2. Architectural design
- Identifying the major components that make up the system and their interactions.
- Designing the system organization using an architectural pattern.
- "A variant of client and server" Architecture
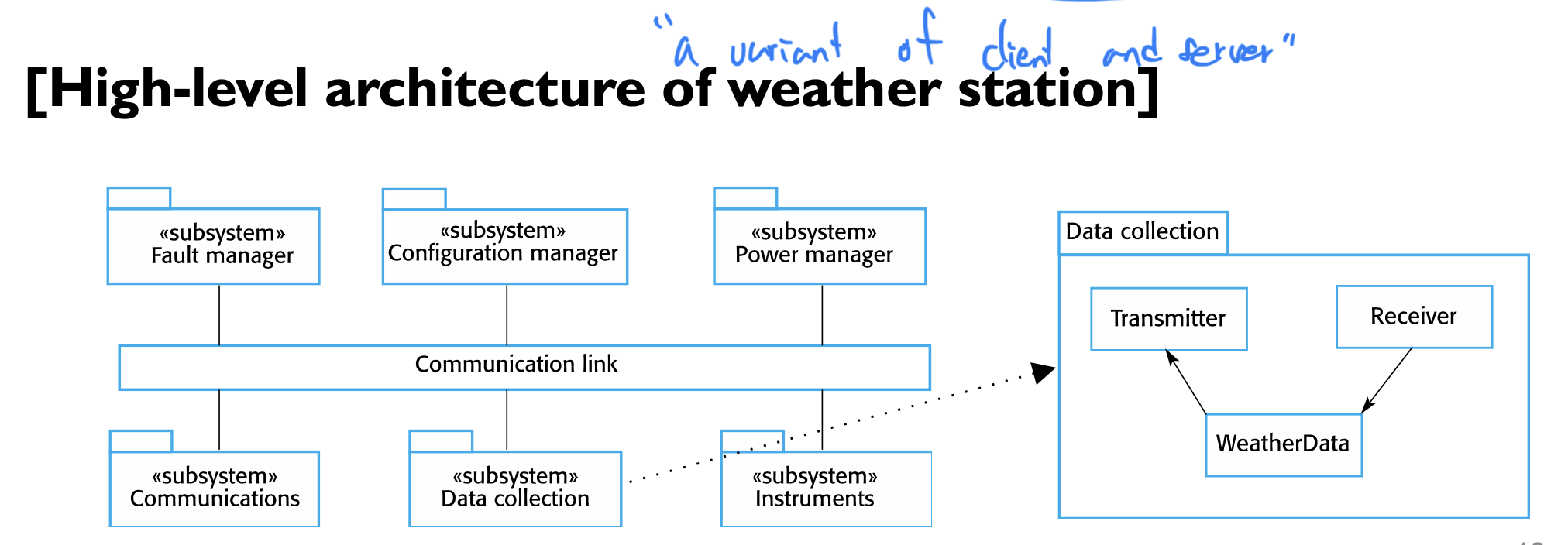
3. Object class identification
- Identifying high-level system objects that encapsulate the system interactions defined in the use cases.
- 3 ways of identifying object classes in object-oriented systems
- Using a grammatical analysis of a natural language description of the system.
(e.g., objects or attributes = nouns, operations or services = verbs)
- Using tangible entities (things).
(e.g., domain -> aircraft, role -> manager, location -> office)
- Using a scenario-based analysis.
[Example for weather station objects]
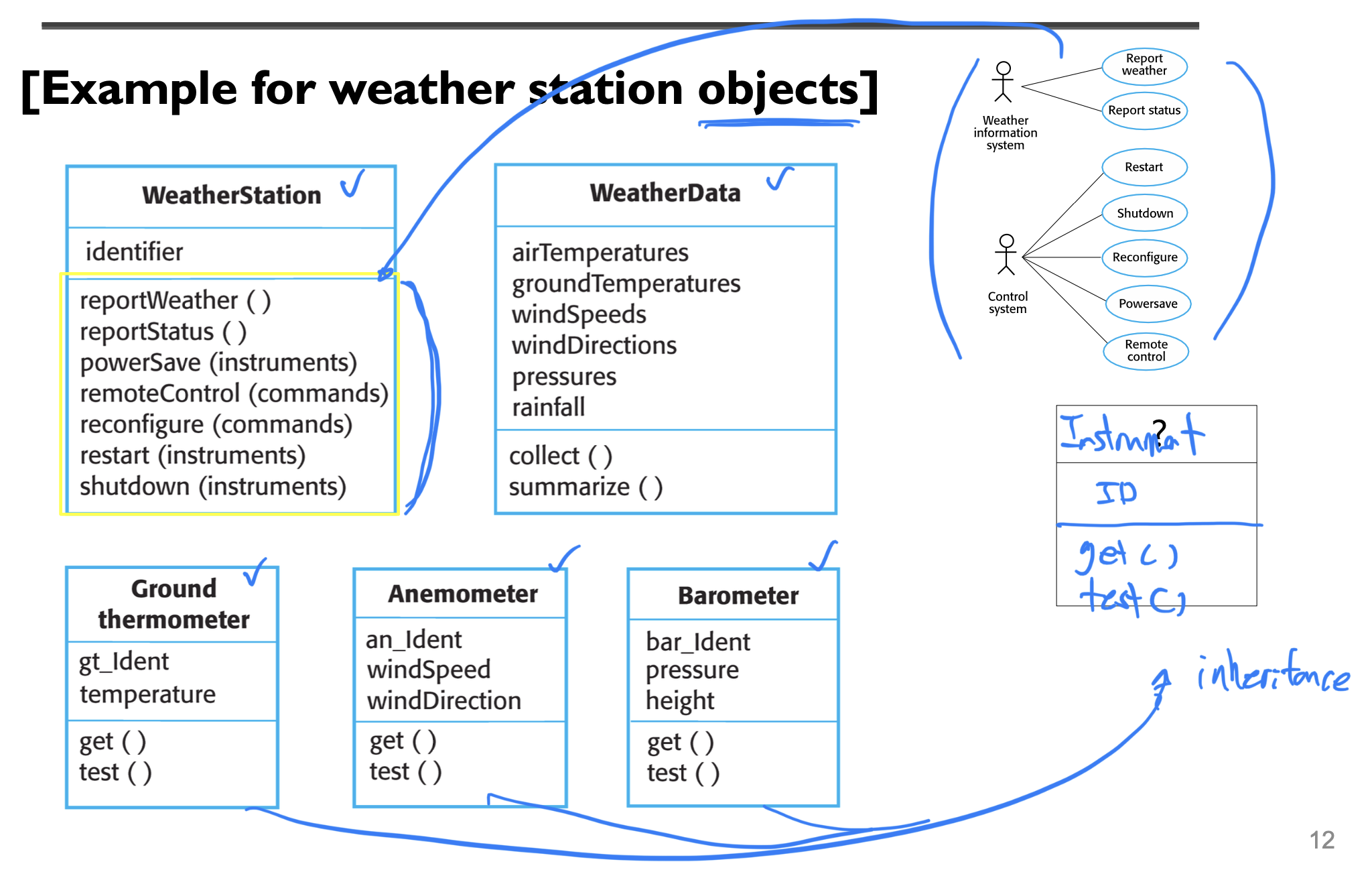
- Weather Station object class
- Providing the basic interface of the weather station with its environment.
- Weather Data object class
- Processing the report weather command.
(Sending the summarized data from the weather station instruments to the weather information system.)
- Ground thermometer, Anemometer, and Barometer object classes
- Reflecting tagible hardware entities in the system.
- Operating autonomously to collect data at the specified frequency.
- Storing the collected data locally.
4. Design models
-
Bridge between the system requirements and its implementation.
- Showing the objects or object classes in a system.
- Showing the associations and relationships between these entities.
-
Deciding which design models to use.
- Use-case, sequence, state, class and activity diagrams.
-
Level of detail in a design model depends on the design process.
- Agile development (e.g., whiteboard)
- Plan-based development (detailed models)
-
Minimizing the number of models.
- Reducing the costs of the design and the time.
[2 kinds of design model]
5. Interface specification
-
Specifying the detail of the interface to an object or a group of objects.
-
Defining the semantics of services provided by a group of objects.
-
Not including details of the data representation in an interface design.
data representation은 포함하지 않음
- We can easily change it without affecting the objects that use that data.
-
Including operations to access and update data.
operation은 포함함
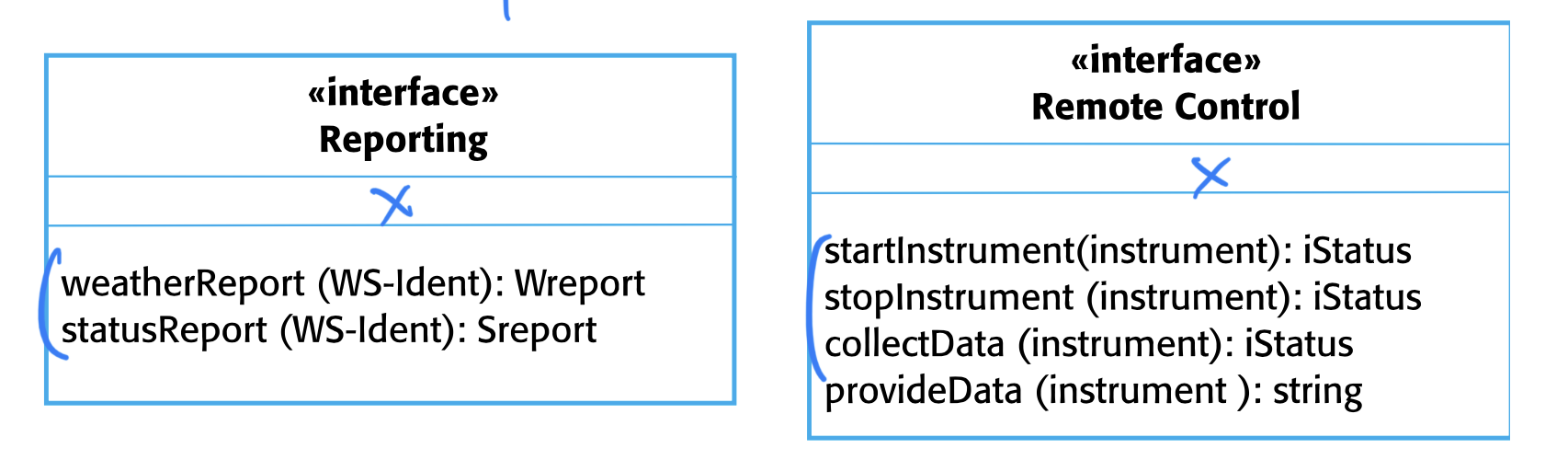
-
Interfaces can be specified in the UML using the same notation as a class diagram.
- No attribute section.
- The UML stereotype <<interface>> inclusion.
- (Reporting) Weather and status reports map directly to operations.
- (Remote Control) Four operations map onto a single method in the object.
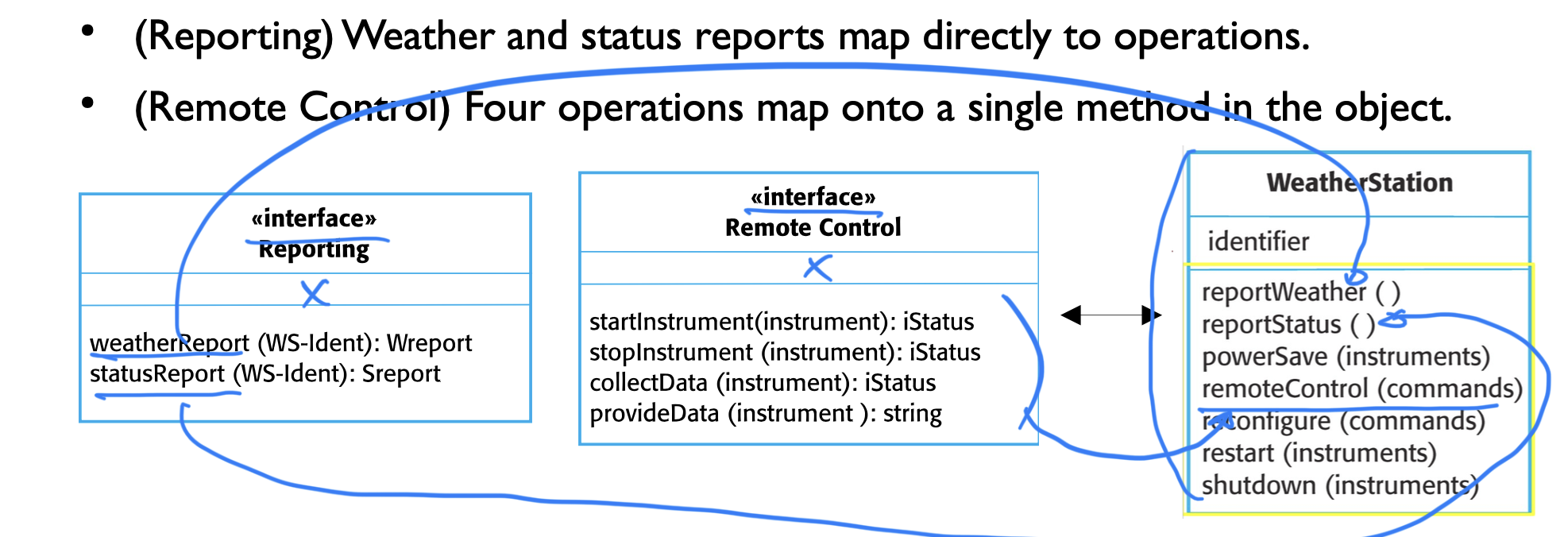
Design patterns
[4 essential elements of design patterns]
- A name
- A meaningful reference to the pattern.
- A problem description
- The problem area that explains when the pattern may be applied.
- A solution description
- The parts of the design solution, their relationships and their responsibilities.
- A statement of the consequences (The results and trade-offs)
- Helping designers understand whether or not a pattern can be used in a particular situation.
Need to recognize that your design problem has an associated pattern that can be applied.
- Tell several objects that the state of some other object has changed (Observer pattern).
객체 중 하나의 상태가 변경되었을 때 다른 여러 객체에게 알려야할 필요가 있다면 "Observer pattern"을 사용. 이 pattern은 subject, object의 상태 변경을 관찰하고, 이러한 변경을 observer object에게 통지하여 상호 작용을 지원함
- Tidy up the interfaces to a number of related objects that have often been developed incrementally (Facade pattern).
관련된 여러 객체의 인터페이스를 간결하게 만들어야 하며 이들 객체는 종종 점진적으로 개발되었다면 "Facade pattern"을 사용. 이 pattern은 복잡한 서브시스템의 인터페이스를 단순화하고 클라이언트에게 통일된 접근 방법을 제공함
- Allow for the possibility of extending the functionality of an existing class at runtime (Decorator pattern).
기존 클래스의 기능을 런타임에 확장할 필요가 있다면 "Decorator pattern"을 사용. 이 pattern은 기존 클래스의 인스턴스에 동적으로 새로운 기능을 추가하고, 기능을 누적하는 방법을 제공함
Implementation issues
[Implementation]
- Developing programs in high or low-level programming languages.
- Tailoring off-the-shelf systems to meet the specific requirements.
[Important aspects of implementation in SW engineering]
- Reuse
- Configuration management
- Managing many differenct versions of SW created during the development process.
- Host-target development
- Developing the SW on one computer (the host system)
- Executing the SW on a separate computer (the target system)
Reuse
[Different levels of SW reuse]
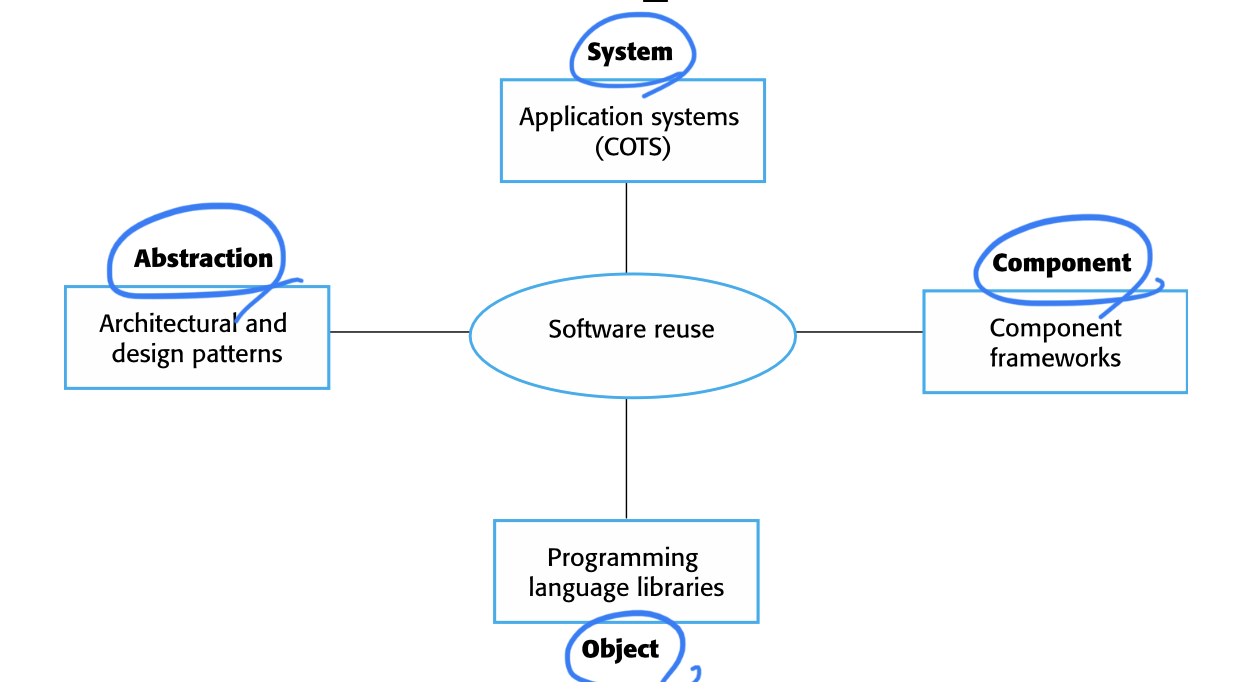
[Reuse costs]
- The costs of the time spent in looking for SW to reuse and assessing whether or not it meets your needs.
- The costs of buying the reusable SW.
- The costs of configuring the reusable SW systems to reflect the requirements of the system.
- The costs of integrating reusable software elements with each otehr and with the new code.
Configuration management
- The general process of managing a changing software system.
- Change management is absolutely essential in SW development.
- Goal
- Supporting the system integration process so that all developers can access the project code in a controlled way. (e.g., conflict in git)
- Ensuring that everyone can access the most up-to-date versions of SW.
- Finding out what changes have been made.
4 fundamental configuration management activities
1. Version management
- Keeping track of the different versions of software components.
- Coordinating development by several programmers.
- Stopping one developer from overwritting code submitted by someone else.
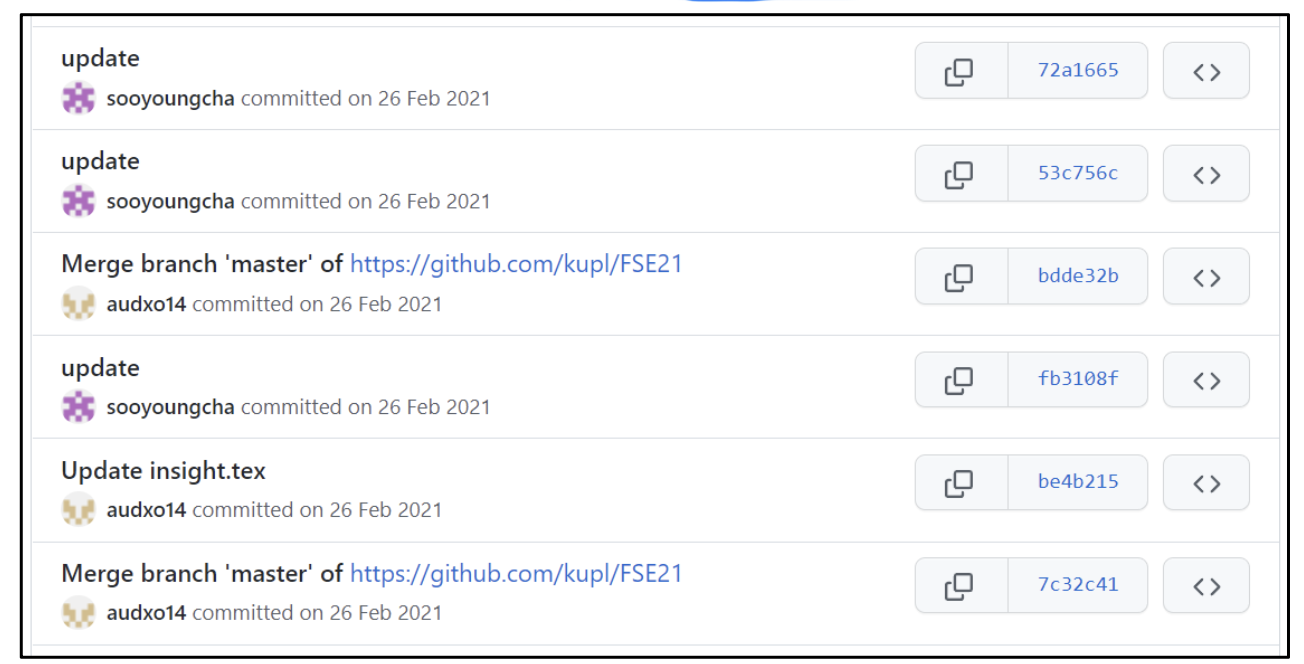
2. System integration
- Helping developers define what versions of components are used to create each version of a system.
3. Problem tracking
- Allowing users to report bugs and other problems.
- Allowing all developers to see who is working on these problems and when they are fixed.
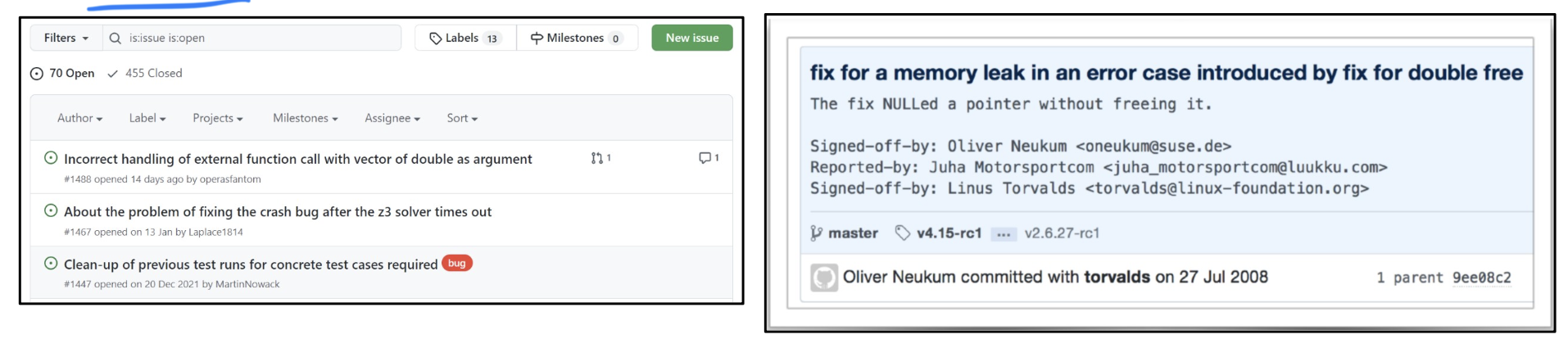
4. Release management
- Planning the functionality of new releases and organizing the software for distribution
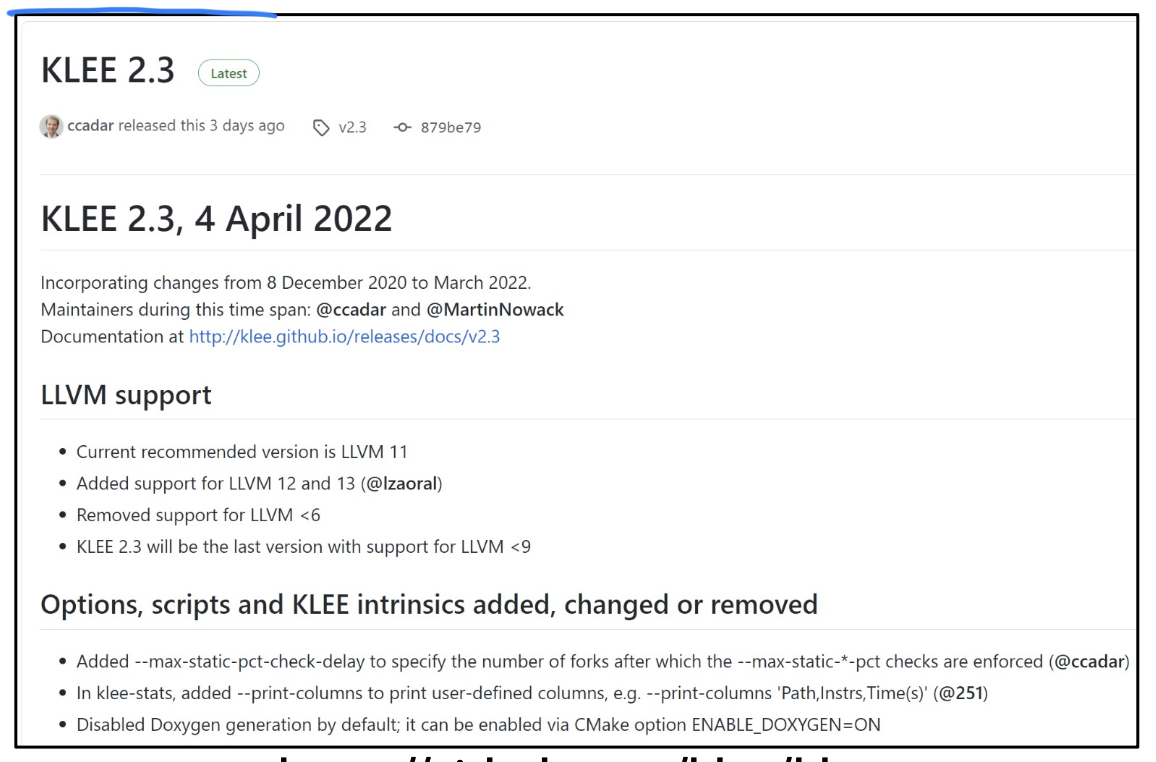
Open-source development
- The source code of a SW system is published and volunteers are invited to participate in the development process.
- The benefits of using open-source SW
- It is usually cheap or even free to acquire open-source SW. (Sparrow는 1억..)
- Widely used open-source systems are very reliable.
- The issues when using open-source SW in a company.
- Should our product make use of open-source components?
- Shoud an open-source approach be used for its own software development?
[Open-source licensing]
Summary
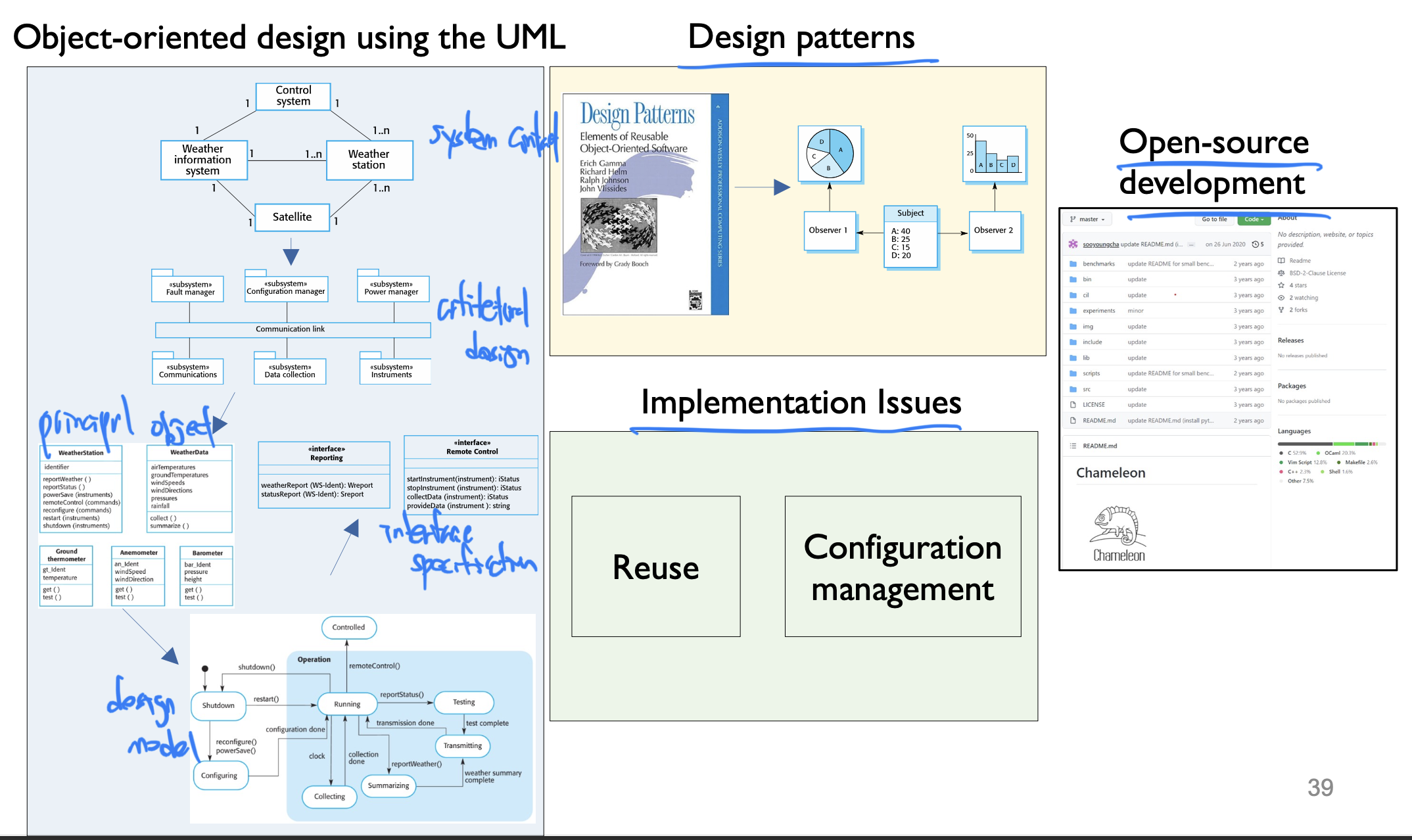