Layered Architecture🔧
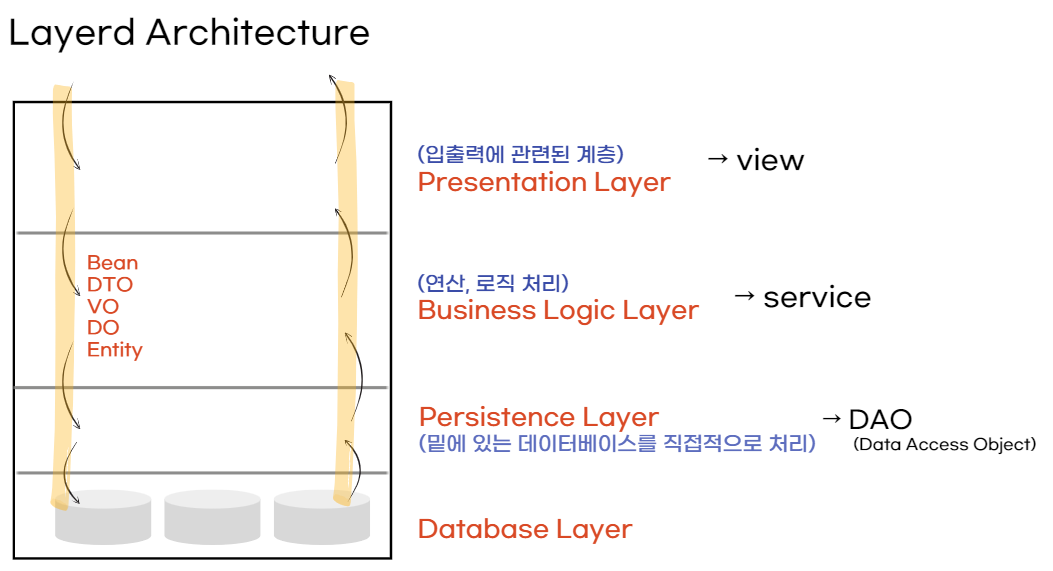
- 각 레이어는 자기가 맡은 역할을 해야 된다.
- 데이터가 왔다갔다할 때 DTO, VO, DO, Entity, Bean 등 여러가지 표현을 사용한다.
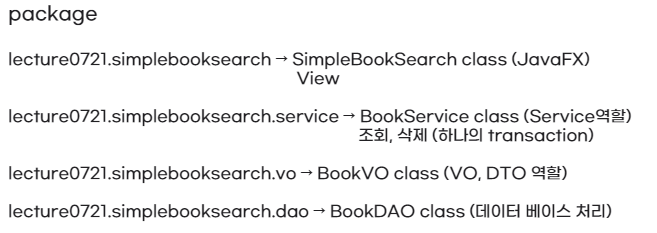
SimpleBookSearch (View)
package lecture0721.simplebookserarch;
import java.util.ArrayList;
import org.apache.commons.dbcp2.BasicDataSource;
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.TextArea;
import javafx.scene.control.TextField;
import javafx.scene.layout.BorderPane;
import javafx.scene.layout.FlowPane;
import javafx.stage.Stage;
import lecture0721.simplebookserarch.service.BookService;
import lecture0721.simplebookserarch.vo.BookVO;
public class SimpleBookSearch extends Application{
TextArea textarea;
Button connBtn, connBtn2;
TextField textfield;
private static BasicDataSource basicDS;
@Override
public void start(Stage primaryStage) throws Exception {
BookService service = new BookService();
BorderPane root = new BorderPane();
root.setPrefSize(700, 500);
textarea = new TextArea();
root.setCenter(textarea);
textfield = new TextField();
textfield.setPrefSize(350, 40);
connBtn = new Button("키워드 검색");
connBtn.setPrefSize(150, 40);
connBtn.setOnAction(e ->{
textarea.clear();
ArrayList<BookVO> result = service.bookSearchByKeyword(textfield.getText());
for(BookVO i: result) {
textarea.appendText(i.getBtitle()+", "+i.getBauthor()+", "+i.getBisbn()+"\n");
}
textfield.clear();
});
connBtn2 = new Button("ISBM으로 삭제");
connBtn2.setPrefSize(150, 40);
connBtn2.setOnAction(e ->{
textarea.clear();
String result = service.bookDeleteByISBN(textfield.getText());
textarea.appendText(result);
textfield.clear();
});
FlowPane flowPane = new FlowPane();
flowPane.setPadding(new Insets(10, 10, 10, 10));
flowPane.setPrefSize(700, 40);
flowPane.setHgap(10);
flowPane.getChildren().add(connBtn);
flowPane.getChildren().add(textfield);
flowPane.getChildren().add(connBtn2);
root.setBottom(flowPane);
Scene scene = new Scene(root);
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch();
}
}
BookService (Service)
package lecture0721.simplebookserarch.service;
import java.util.ArrayList;
import lecture0721.simplebookserarch.dao.BookDAO;
import lecture0721.simplebookserarch.vo.BookVO;
public class BookService {
private BookDAO dao;
public BookService() {
this.dao = new BookDAO();
}
public ArrayList<BookVO> bookSearchByKeyword(String keyword) {
ArrayList<BookVO> result = dao.select(keyword);
return result;
}
public String bookDeleteByISBN(String bisbn) {
String result = dao.delete(bisbn);
return result;
}
}
BookDAO
package lecture0721.simplebookserarch.dao;
import java.io.FileInputStream;
import java.io.InputStream;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.Properties;
import javax.sql.DataSource;
import org.apache.commons.dbcp2.BasicDataSource;
import lecture0721.simplebookserarch.vo.BookVO;
public class BookDAO {
private static BasicDataSource basicDS;
public BookDAO(){
try {
basicDS = new BasicDataSource();
Properties properties = new Properties();
InputStream is = new FileInputStream("resources/db.properties");
properties.load(is);
basicDS.setDriverClassName(properties.getProperty("DRIVER_CLASS"));
basicDS.setUrl(properties.getProperty("JDBC_URL"));
basicDS.setUsername(properties.getProperty("DB_USER"));
basicDS.setPassword(properties.getProperty("DB_PASSWORD"));
basicDS.setInitialSize(10);
basicDS.setMaxTotal(10);
} catch (Exception e) {
}
}
public static DataSource getDataSource() {
return basicDS;
}
public ArrayList<BookVO> select(String keyword) {
ArrayList<BookVO> list = null;
ResultSet rs = null;
PreparedStatement pstmt = null;
Connection con = null;
try {
DataSource ds = getDataSource();
try {
con = ds.getConnection();
con.setAutoCommit(false);
String sql = "SELECT * FROM book WHERE btitle LIKE '%"+keyword+"%'";
pstmt = con.prepareStatement(sql);
rs = pstmt.executeQuery();
con.commit();
list = new ArrayList<BookVO>();
while (rs.next()) {
BookVO tmp = new BookVO();
tmp.setBtitle(rs.getString("btitle"));
tmp.setBauthor(rs.getString("bauthor"));
tmp.setBisbn(rs.getString("bisbn"));
list.add(tmp);
}
} catch (SQLException e1) {
System.out.println(e1);
}
} catch (Exception e2) {
} finally {
try {
if (rs != null) rs.close();
if (pstmt != null) pstmt.close();
if (con != null) con.close();
} catch (Exception e3) {
}
}
return list;
}
public String delete(String bisbn) {
ArrayList<BookVO> list = null;
ResultSet rs = null;
PreparedStatement pstmt = null;
Connection con = null;
String msg = "";
try {
DataSource ds = getDataSource();
try {
con = ds.getConnection();
con.setAutoCommit(false);
String sql = "DELETE FROM BOOK where bisbn = '"+bisbn+"'";
pstmt = con.prepareStatement(sql);
int result = pstmt.executeUpdate();
con.commit();
if (result >= 1) {
msg = "삭제 완료!\n";
} else {
msg = "/n 삭제 할 자료가 없습니다..!/n";
}
} catch (SQLException e1) {
System.out.println(e1);
}
} catch (Exception e2) {
} finally {
try {
if (rs != null) rs.close();
if (pstmt != null) pstmt.close();
if (con != null) con.close();
} catch (Exception e3) {
}
}
return msg;
}
}
BookVO
package lecture0721.simplebookserarch.vo;
public class BookVO {
private String bisbn;
private String btitle;
private String bdate;
private int bpage;
private int bprice;
private String bauthor;
public BookVO() {
}
public String getBisbn() {
return bisbn;
}
public void setBisbn(String bisbn) {
this.bisbn = bisbn;
}
public String getBtitle() {
return btitle;
}
public void setBtitle(String btitle) {
this.btitle = btitle;
}
public String getBdate() {
return bdate;
}
public void setBdate(String bdate) {
this.bdate = bdate;
}
public int getBpage() {
return bpage;
}
public void setBpage(int bpage) {
this.bpage = bpage;
}
public int getBprice() {
return bprice;
}
public void setBprice(int bprice) {
this.bprice = bprice;
}
public String getBauthor() {
return bauthor;
}
public void setBauthor(String bauthor) {
this.bauthor = bauthor;
}
}