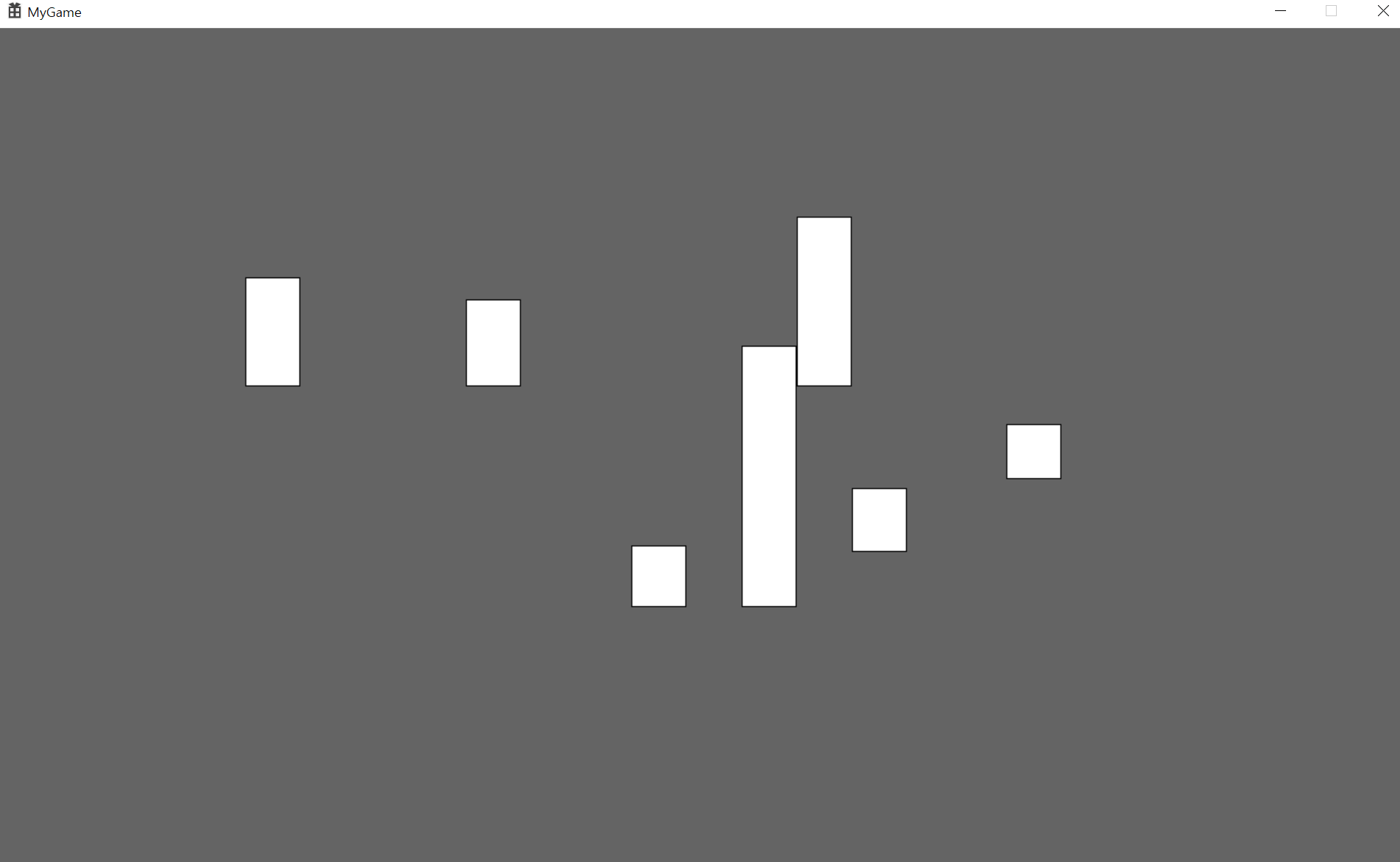
CLevelMGR.cpp
#include "pch.h"
#include "CLevelMgr.h"
#include "CLevel.h"
#include "CEngine.h"
#include "CPlayer.h"
#include "CMonster.h"
#include "CMissile.h"
#include "CThorn.h"
CLevelMgr::CLevelMgr()
: m_arrLevel{}
, m_CurLevel(nullptr)
{
}
CLevelMgr::~CLevelMgr()
{
Delete_Array(m_arrLevel);
}
void CLevelMgr::Init()
{
Vec2 vResolution = CEngine::GetInst()->GetResolution();
CLevel* pLevel = new CLevel;
CObj* pObject = new CPlayer;
pObject->SetPos(vResolution.x / 2.f + 300.f, vResolution.y / 2.f);
pObject->SetScale(50.f, 50.f);
pLevel->AddObject(pObject);
CMonster* pMonster = new CMonster;
pMonster->SetPos(600.f, 300.f);
pMonster->SetScale(100.f, 100.f);
pMonster->SetDistance(200.f);
pMonster->SetSpeed(300.f);
CThorn* pThorn = new CThorn;
pThorn->SetPos(250.f, 300.f);
pThorn->SetScale(50.f, 50.f);
pLevel->AddObject(pThorn);
CThorn* pThorn1 = new CThorn;
pThorn1->SetPos(450.f, 300.f);
pThorn1->SetScale(50.f, 50.f);
pThorn1->SetDurationTime(1500.f);
pThorn1->SetDistance(100.f);
pLevel->AddObject(pThorn1);
CThorn* pThorn2 = new CThorn;
pThorn2->SetPos(750.f, 300.f);
pThorn2->SetScale(50.f, 50.f);
pThorn2->SetDurationTime(1200.f);
pThorn2->SetDistance(150.f);
pLevel->AddObject(pThorn2);
CThorn* pThorn3 = new CThorn;
pThorn3->SetPos(600.f, 500.f);
pThorn3->SetScale(50.f, 50.f);
pThorn3->SetDurationTime(1700.f);
pThorn3->SetDistance(170.f);
pLevel->AddObject(pThorn3);
CThorn* pThorn5 = new CThorn;
pThorn5->SetPos(700.f, 500.f);
pThorn5->SetScale(50.f, 50.f);
pThorn5->SetDurationTime(1250.f);
pThorn5->SetDistance(300.f);
pLevel->AddObject(pThorn5);
CThorn* pThorn4 = new CThorn;
pThorn4->SetPos(800.f, 450.f);
pThorn4->SetScale(50.f, 50.f);
pThorn4->SetDurationTime(1700.f);
pThorn4->SetDistance(230.f);
pLevel->AddObject(pThorn4);
m_CurLevel = m_arrLevel[(UINT)LEVEL_TYPE::START] = pLevel;
m_CurLevel->Begin();
}
void CLevelMgr::Progress()
{
if (nullptr == m_CurLevel)
return;
m_CurLevel->Tick();
m_CurLevel->FinalTick();
}
void CLevelMgr::Render()
{
m_CurLevel->Render();
}
CThorn.h
#pragma once
#include "CObj.h"
class CThorn :
public CObj
{
private:
float GrowTime;
float DurationTime;
Vec2 m_InitPos;
float Distance;
float Direction;
private:
float xPlus;
float yPlus;
float xMinus;
float yMinus;
public:
void SetGrowTime(float _in) { GrowTime = _in; }
void SetDurationTime(float _in) { DurationTime = _in; }
void SetDistance(float _in) { Distance = _in; }
public:
void Set_xPlus(float _in) { xPlus = _in; }
void Set_yPlus(float _in) { yPlus = _in; }
void Set_xMinus(float _in) { xMinus = _in; }
void Set_yMinus(float _in) { yMinus = _in; }
public:
virtual void Tick() override;
virtual void Begin() override;
virtual void Render() override;
public:
CThorn();
~CThorn();
};
CThorn.cpp
#include "pch.h"
#include "CThorn.h"
#include "CEngine.h"
#include "CTimeMgr.h"
#include "CKeyMgr.h"
#include "CLevelMgr.h"
#include "CLevel.h"
#include "CObj.h"
CThorn::CThorn()
:GrowTime(100),
DurationTime(1000),
Distance(50.f),
Direction(1.0f),
xPlus(0.f),
yPlus(0.f),
xMinus(0.f),
yMinus(0.f)
{
}
CThorn::~CThorn()
{
}
void CThorn::Begin()
{
m_InitPos = GetPos();
}
void CThorn::Tick()
{
Vec2 Size = GetPos();
float GrowSpeed = DT * Direction * Distance;
if (GrowTime < DurationTime) {
if (Direction == 1) {
Set_yPlus(GrowSpeed + yPlus);
GrowTime++;
}
else if (Direction == -1) {
Set_yMinus(GrowSpeed + yMinus);
GrowTime++;
}
}
else if (GrowTime >= DurationTime) {
GrowTime = 0;
if (Direction == 1) {
Direction = -1;
}
else if(Direction == -1){
Direction = 1;
}
Set_yPlus(0.f);
Set_yMinus(0.f);
}
}
void CThorn::Render()
{
HDC dc = CEngine::GetInst()->GetSecondDC();
Vec2 vPos = GetPos();
Vec2 vScale = GetScale();
Rectangle(dc, vPos.x - vScale.x / 2.f + xMinus, vPos.y - vScale.y / 2.f + yMinus
, vPos.x + vScale.x / 2.f + xPlus, vPos.y + vScale.y / 2.f + yPlus);
}