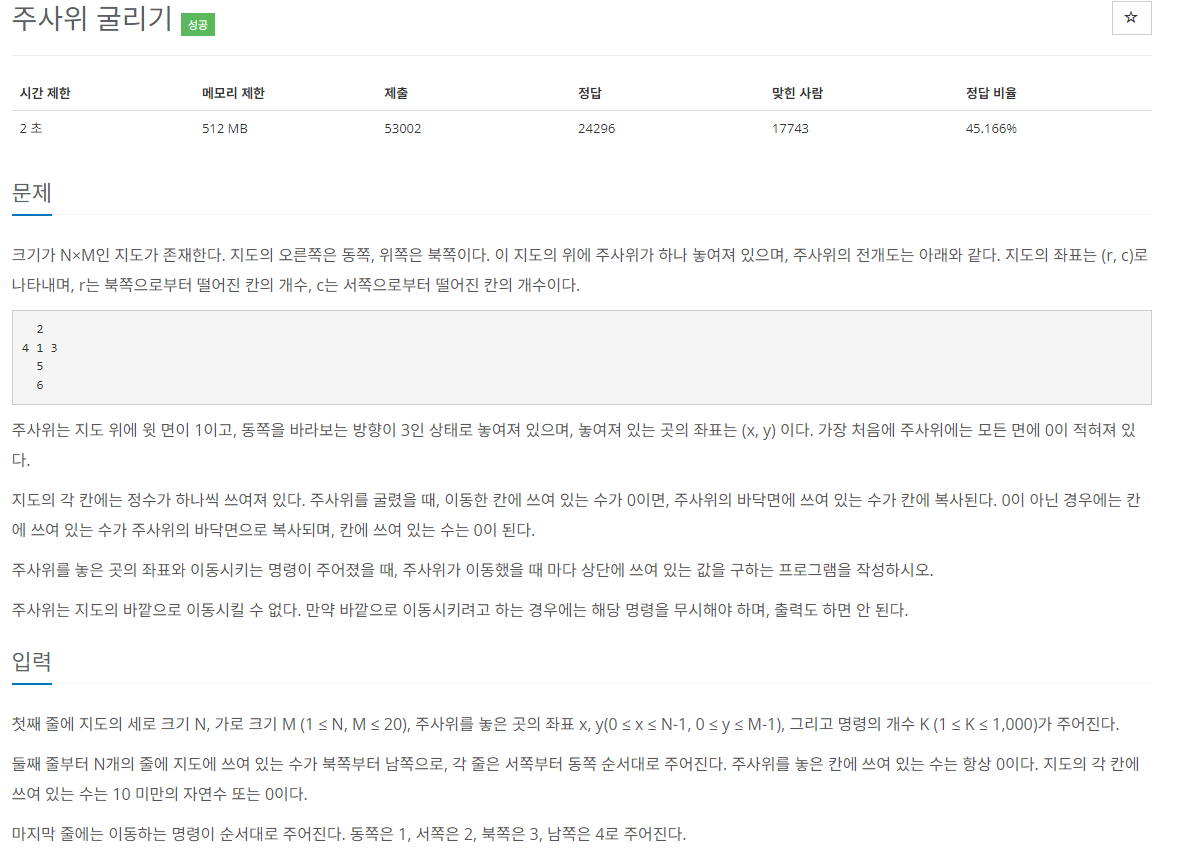
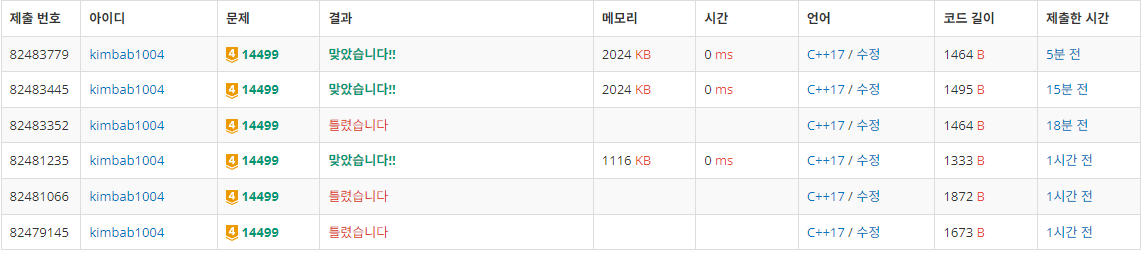
#include <iostream>
#include <vector>
using namespace std;
int n, m, x, y, k;
int d;
int map[21][21];
int dice[6] = { 0 };
bool check(int d) {
if (d == 1) {
if (y + 1 < m) {
return true;
}
}
else if (d == 2) {
if (y - 1 >= 0) {
return true;
}
}
if (d == 3) {
if (x - 1 >= 0) {
return true;
}
}
if (d == 4) {
if (x + 1 < n) {
return true;
}
}
return false;
}
void dice_move(int d) {
int temp = dice[0];
if (d == 1) {
y++;
dice[0] = dice[3];
dice[3] = dice[5];
dice[5] = dice[2];
dice[2] = temp;
}
else if (d == 2) {
y--;
dice[0] = dice[2];
dice[2] = dice[5];
dice[5] = dice[3];
dice[3] = temp;
}
else if (d == 3) {
x--;
dice[0] = dice[4];
dice[4] = dice[5];
dice[5] = dice[1];
dice[1] = temp;
}
else if (d == 4) {
x++;
dice[0] = dice[1];
dice[1] = dice[5];
dice[5] = dice[4];
dice[4] = temp;
}
if (map[x][y] == 0) {
map[x][y] = dice[5];
}
else {
dice[5] = map[x][y];
map[x][y] = 0;
}
cout << dice[0] << "\n";
}
int main() {
ios_base::sync_with_stdio(false);
cin.tie(NULL);
cout.tie(NULL);
cin >> n >> m >> x >> y >> k;
for (int i = 0; i < n; i++) {
for (int j = 0; j < m; j++) {
cin >> map[i][j];
}
}
for (int i = 0; i < k; i++) {
cin >> d;
if (check(d) == true) {
dice_move(d);
}
}
return 0;
}