1. GSAP
- GreenSock에서 만든 자바스크립트 애니메이션 라이브러리
<body>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/gsap/3.11.4/gsap.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/gsap/3.11.4/ScrollTrigger.min.js"></script>
</body>
🔷gsap.to()
- gsap.to()는 대상과 속성값이 필수
- css로 transform: translateX(100px)과 정확히 같은 효과
- duration은 지속 시간
- duration은 작성하지 않으면 기본값인 0.5(초)
- stagger는 각각 애니메이션 시작 시간 사이에 지정한 시간을 추가
.box {
width: 200px;
height: 100px;
margin: 5px;
background: #f00;
}
gsap.to('.box',{
x: 100,
duration: 1,
})
- gsap.to()
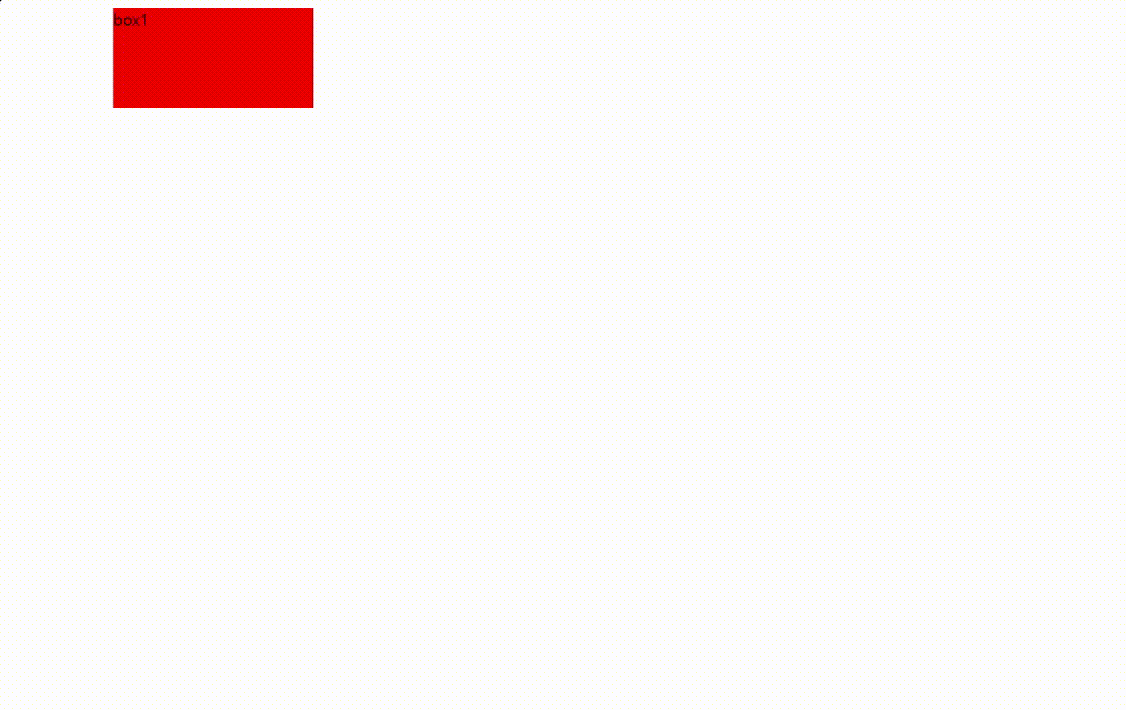
gsap.to('.box',{
xPercent: 100,
stagger: 0.1,
})
- stagger
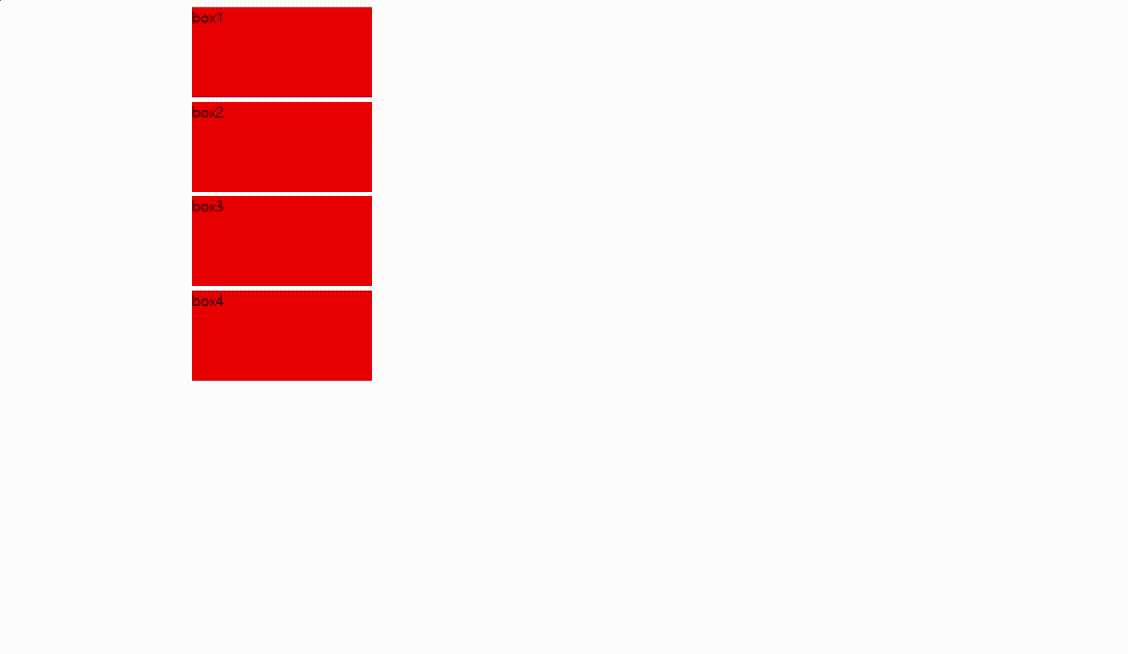
- stagger random
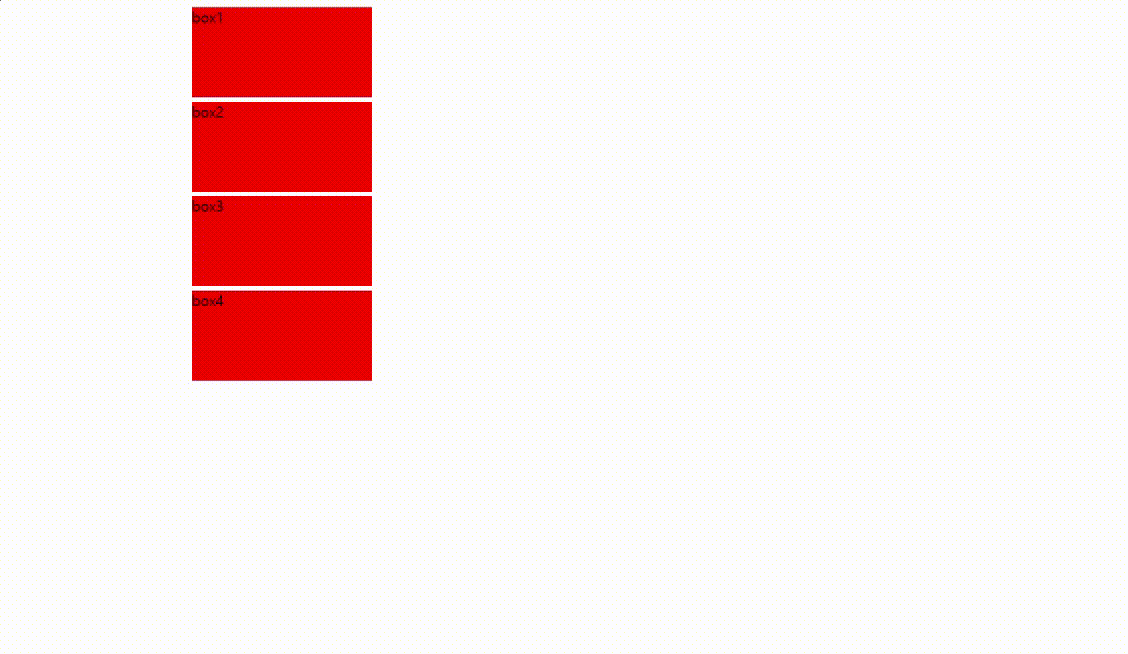
🔷gsap.from()
- 변한 상태에서 속성을 부여해 다시 원래 상태로 돌아오는 것(태초의 상태)
.box {
width: 200px;
height: 100px;
margin: 5px;
background: #f00;
}
gsap.from('.box',{
x: 200,
width: 500,
height: 500,
opacity: .2,
duration: 2,
backgroundColor: '#00f',
})
- gsap.from()
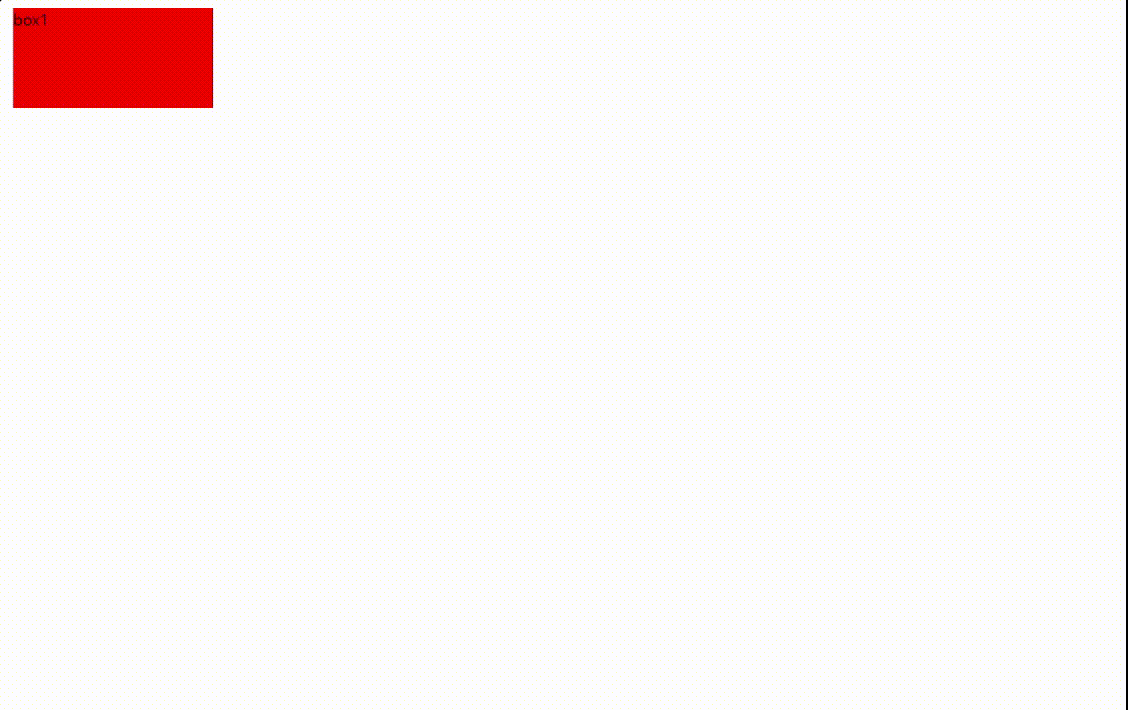
🔷gsap.fromTo()
.box {
width: 200px;
height: 100px;
margin: 5px;
background: #f00;
}
gsap.fromTo('.box2', {
scale: 0.85,
opacity: 0,
x: 80,
y: 80,
backgroundColor: '#00f',
}, {
scale: 1,
opacity: 1,
x: 0,
y: 0,
backgroundColor: '#0f0',
duration: 2,
});
- gsap.fromTo()
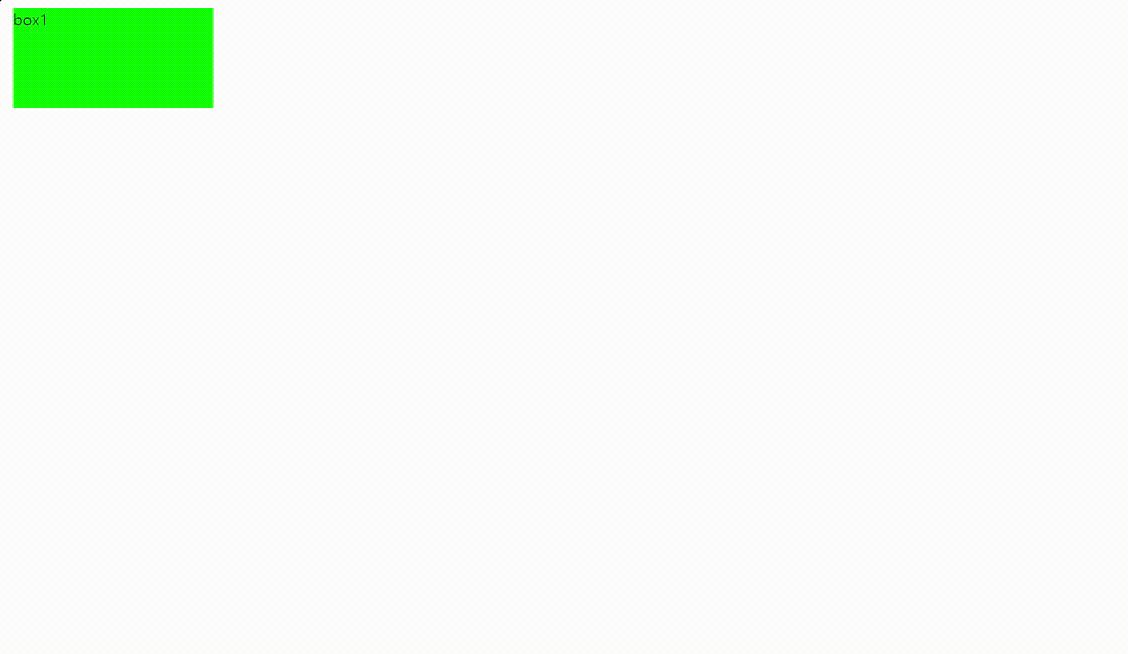
🔷gsap.play(), .pause(), .resume(), .reverse(), .restart() + 🔷.timeline
- 애니메이션을 멈추거나 재실행하는 등의 핸들링을 할 수 있음
- 순차적으로 애니메이션을 실행하고자 할 때 timeline 사용
<button class="btn1">재생</button>
<button class="btn2">정지</button>
<button class="btn3">재실행</button>
<button class="btn4">되돌리기</button>
<div class="box box1">box1</div>
<div class="box box2">box2</div>
<div class="box box3">box3</div>
<div class="box box4">box4</div>
.box {
width: 200px;
height: 100px;
margin: 5px;
background: #f00;
}
button{
cursor: pointer;
const motion1 = gsap.timeline({
paused:true,
});
motion1
.to('.box1',{x:100,})
.addLabel('a')
.to('.box2',{x:100,},'a')
.to('.box3',{x:100,},'a')
.to('body',{background:'#00f'});
$('.btn1').click(function(){
motion1.play();
});
$('.btn2').click(function(){
motion1.pause();
});
$('.btn3').click(function(){
motion1.restart();
});
$('.btn4').click(function(){
motion1.reverse();
});
- timeline
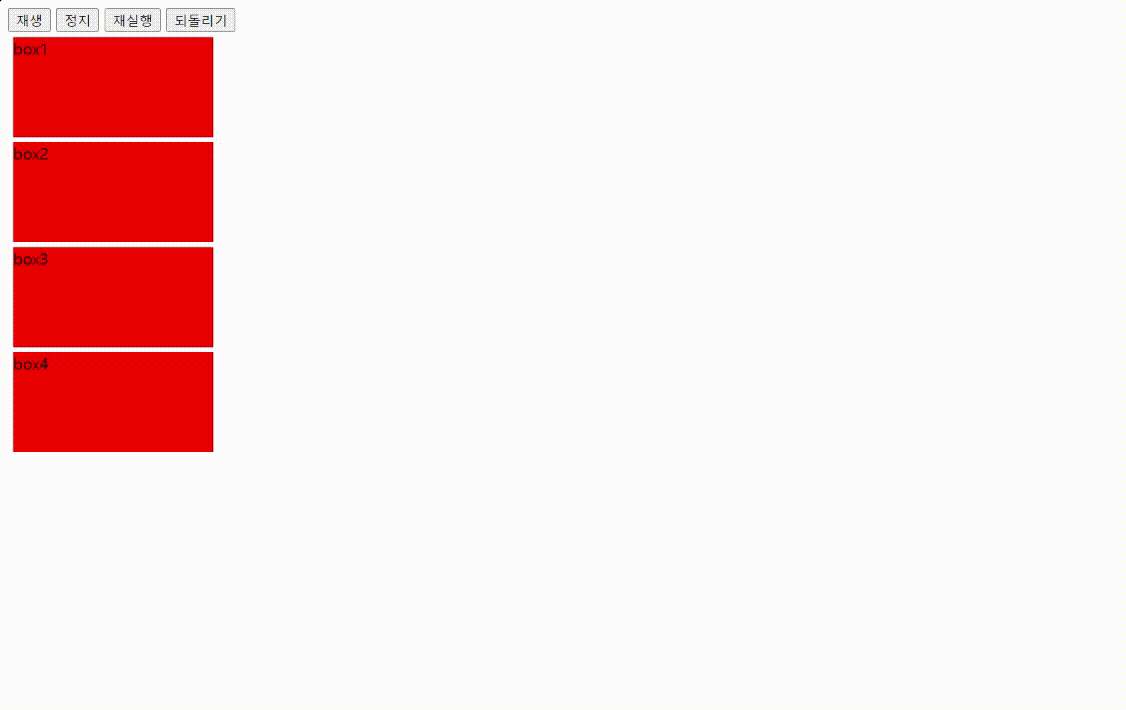
🔷요소 한 개에만 효과 주기
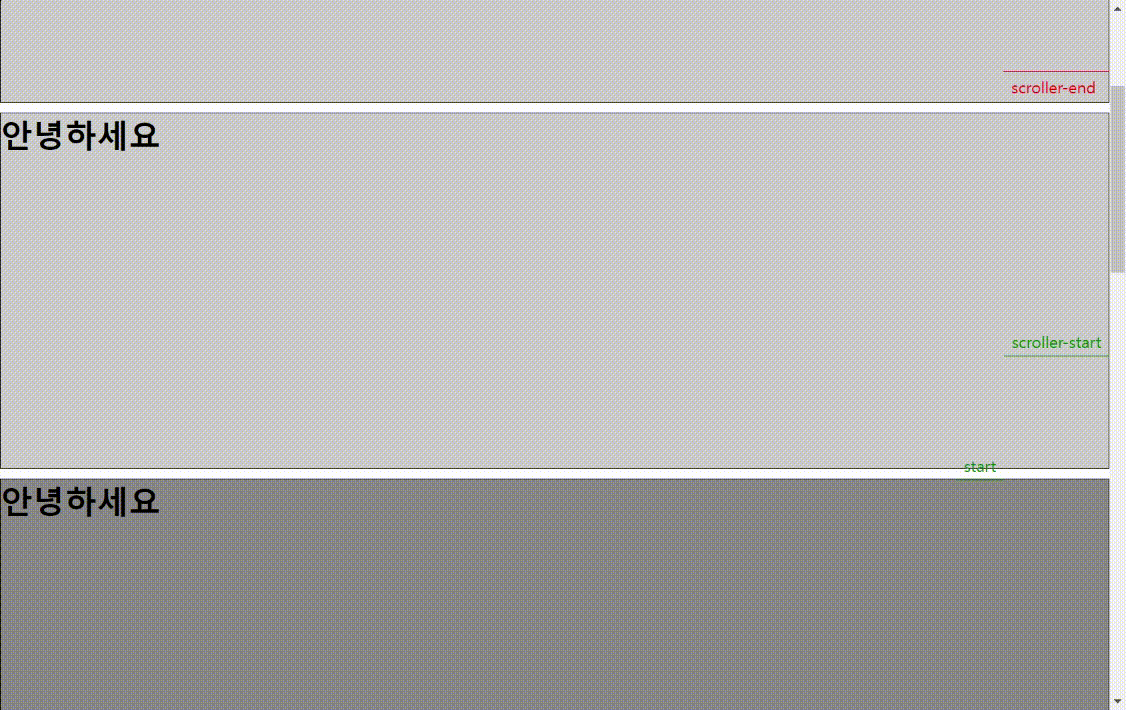
gsap.to('.here h1',{
scrollTrigger:{
trigger:'.here',
start:'0% 50%',
end:'100% 10%',
markers:true,
scrub:1,
},
fontSize:100,
});
🔷두가지 효과를 한 번에 주기
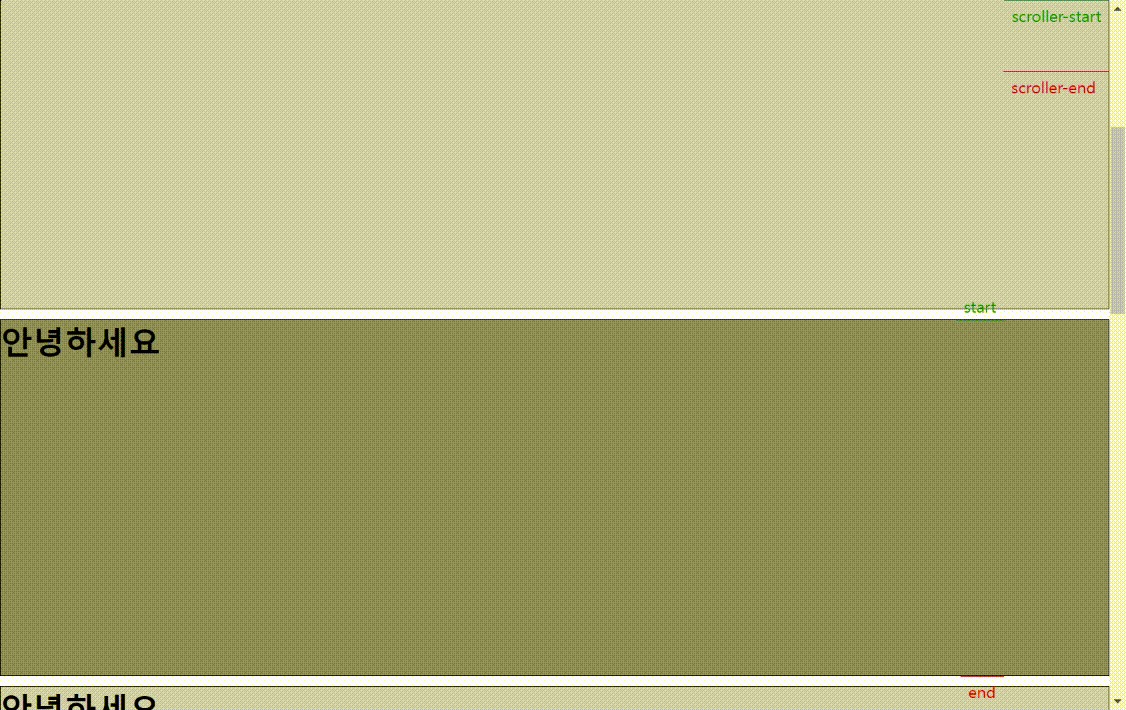
const motion1 = gsap.timeline({
scrollTrigger:{
trigger:'.here',
start:'0% 0%',
end:'100% 10%',
markers:true,
scrub:1,
},
});
motion1
.to('.here h1',{fontSize:100})
.to('.here',{background:'#333'});
🔷* JavaScript 요소 선택
document.querySelector('h1').style.color = '#f00';
document.querySelectorAll('h1').forEach(element => {
element.style.color = '#f00';
})
🔷개별로 다 효과 주기
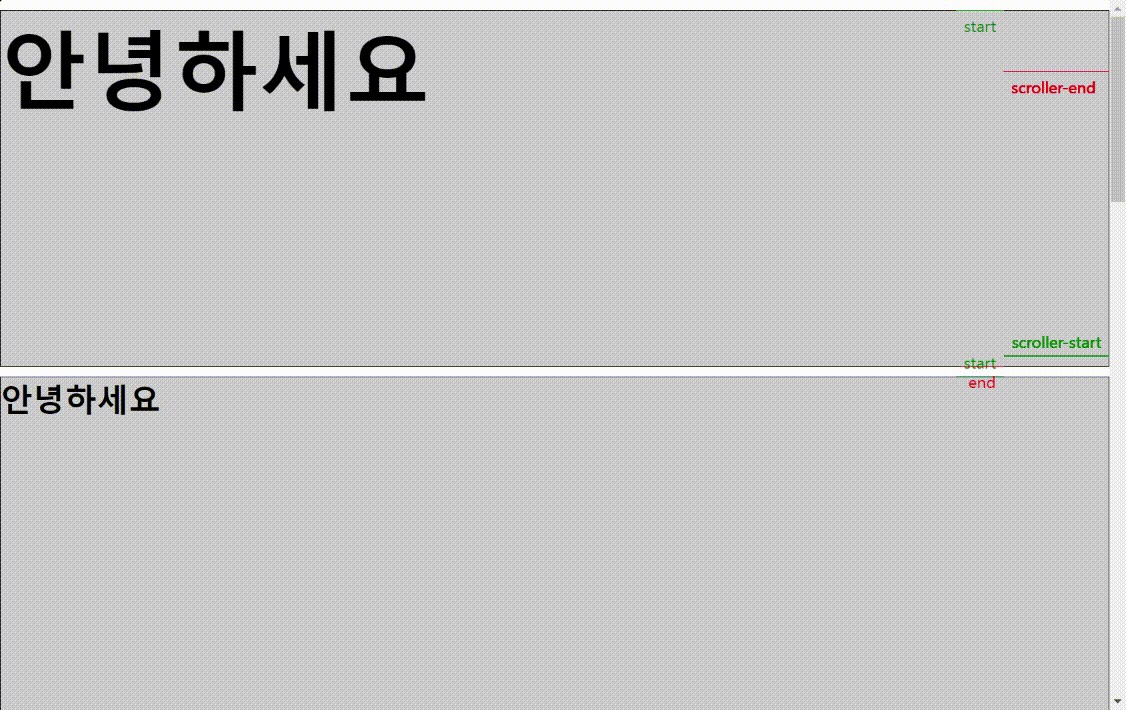
document.querySelectorAll('.box').forEach(element => {
gsap.to(element.children[0],{
scrollTrigger:{
trigger:element,
start:'0% 50%',
end:'100% 10%',
markers:true,
scrub:1,
},
fontSize:100,
})
});
🔷트리거 지점만 만들기
ScrollTrigger.create({
trigger:'.here',
start:'0% 50%',
end:'100% 0%',
markers:true,
toggleClass:{targets:'.footer',className:'on'},
})
🔷특정 지점에 도달했을 때만 효과 주기
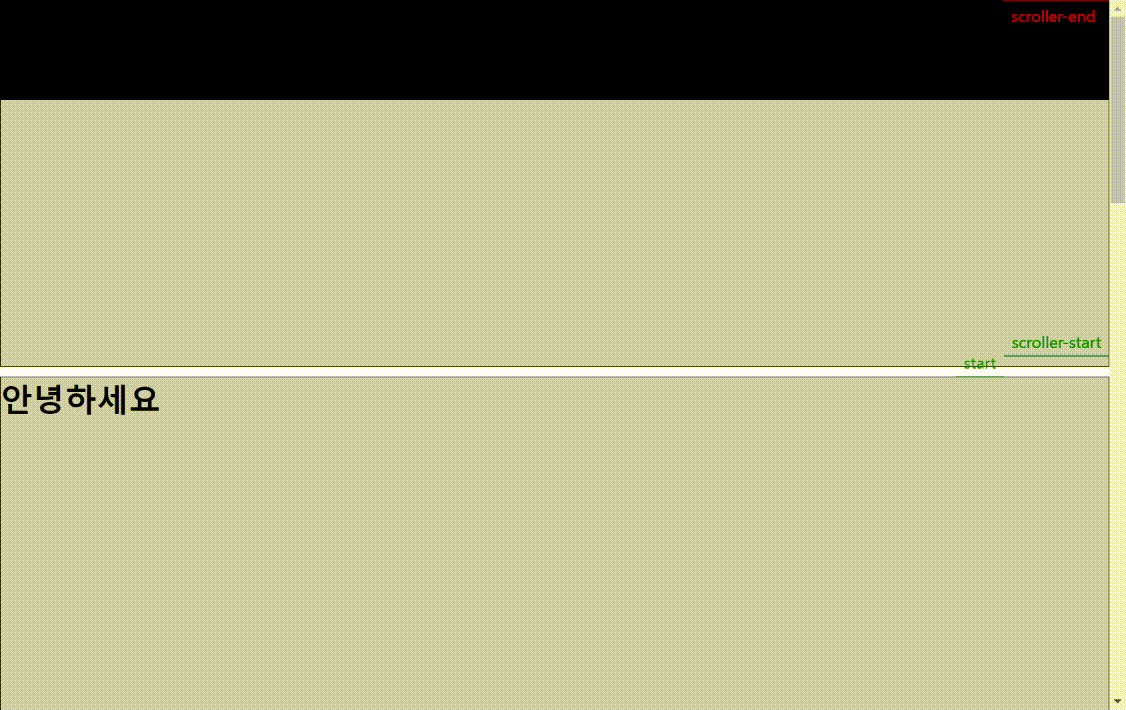
document.querySelectorAll('[data-theme=white]').forEach(element => {
ScrollTrigger.create({
trigger:element,
start:'0% 50%',
end:'100% 0%',
markers:true,
toggleClass:{targets:'.header',className:'on'},
})
});