- MsTest 프로젝트에서 ErCore Repository에서 만든 CRUD 메소드 테스트 하기
- 실제 DB랑 연결하면 테스트 마다 DB에 데이터에 영향이 있어 Inmemory를 사용하며 메소드가 종료하면 데이터가 남지 않는 휘발성 데이터.
nuget에서 Microsoft.EntityFrameworkCore.InMemory, Microsoft.EntityFrameworkCore.SqlServer을 Model프로젝트와 Test프로젝트 모두 설치
버전은 5.0.17버전설치 했으며 이유는 5.0.17까지 .netstandard2.1를 지원하기 떄문 현재 Model 프로젝트가 .netStandard2.1로 되어 있기떄문.
- github 소스코드
1. Test프로젝트 생성
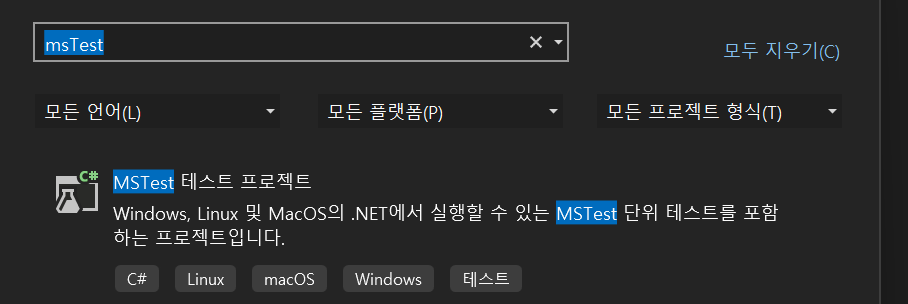
- Model 프로젝트에 video객체및 VideoContext를 사용하기 떄문에 Model 프로젝트 참조 추가.
- testc + tab+ tab하면 클래스가 생성되며 클래스 위에
TestClass
라는 어노테이션이 생성됨. 해당 어노테이션이 없으면 테스트 불가.
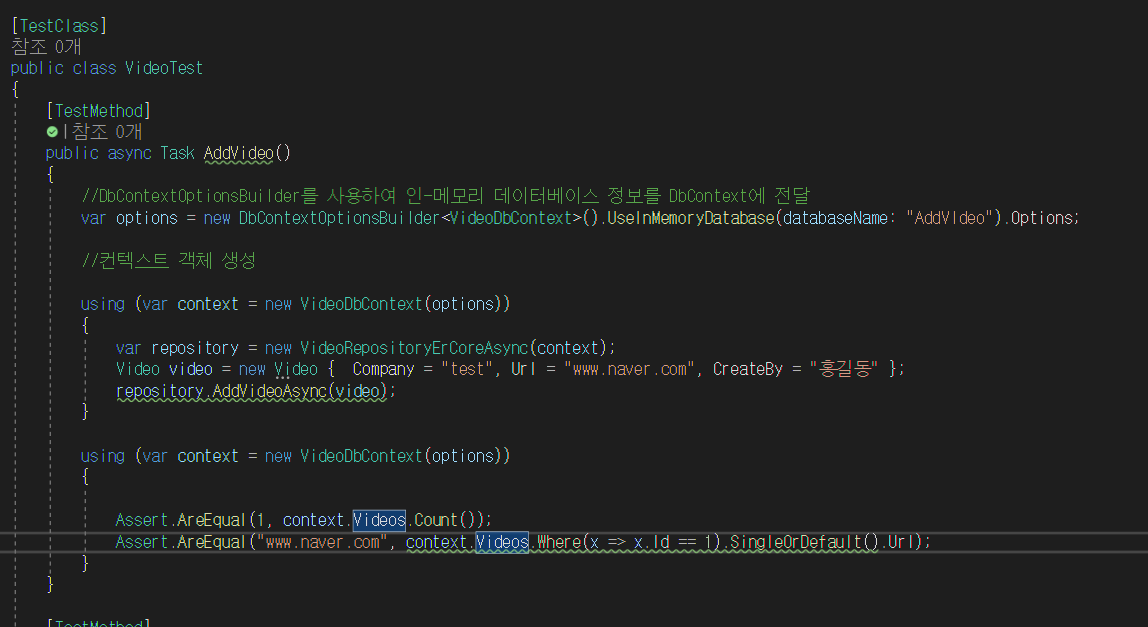
- testm + tab + tab을 하면 테스트 매소드가 생성되며
TestMethod
어노테이션이 같이 생성됨.
- Inmemory데이터 베이스를 사용하기 위해
DbContextOptionsBuilder<VideoDbContext>().UseInMemoryDatabase()
메소드를 사용 하며 2번 사진과 같이 데이터베이스명을 입력하고 VideoContext객체에 테이터베이스 연결 option을 넣어줌.
1.1. 코드
- VideoRepositoryErCoreAsync객체를 가져와 repository변수로 사용
- Assert를 사용하여 코드 검증
using Microsoft.EntityFrameworkCore;
using Microsoft.VisualStudio.TestTools.UnitTesting;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace VideoAppCoreModels.Test
{
[TestClass]
public class VideoTest
{
[TestMethod]
public async Task AddVideo()
{
var options = new DbContextOptionsBuilder<VideoDbContext>().UseInMemoryDatabase(databaseName: "AddVIdeo").Options;
using (var context = new VideoDbContext(options))
{
var repository = new VideoRepositoryErCoreAsync(context);
Video video = new Video { Company = "test", Url = "www.naver.com", CreateBy = "홍길동" };
repository.AddVideoAsync(video);
}
using (var context = new VideoDbContext(options))
{
Assert.AreEqual(1, context.Videos.Count());
Assert.AreEqual("www.naver.com", context.Videos.Where(x => x.Id == 1).SingleOrDefault().Url);
}
}
[TestMethod]
public async Task GetVideos()
{
var options = new DbContextOptionsBuilder<VideoDbContext>().UseInMemoryDatabase(databaseName: "GetVIdeos").Options;
using (var context = new VideoDbContext(options))
{
var repository = new VideoRepositoryErCoreAsync(context);
await repository.AddVideoAsync(new Video { Title = "Test", Company = "test", Url = "www.naver.com", CreateBy = "홍길동" });
await repository.AddVideoAsync(new Video { Title = "Test1", Company = "test", Url = "www.naver.com", CreateBy = "홍길동1" });
await repository.AddVideoAsync(new Video { Title = "Test2", Company = "test", Url = "www.naver.com", CreateBy = "홍길동2" });
}
using (var context = new VideoDbContext(options))
{
var repository = new VideoRepositoryErCoreAsync(context);
var videos = await repository.GetVideosAsync();
Assert.AreEqual(3, context.Videos.Count());
Assert.AreEqual("홍길동", context.Videos.Where(x => x.Id == 1).SingleOrDefault().CreateBy);
}
}
[TestMethod]
public async Task GetVideo()
{
var options = new DbContextOptionsBuilder<VideoDbContext>().UseInMemoryDatabase(databaseName: "GetVIdeo").Options;
using (var context = new VideoDbContext(options))
{
var repository = new VideoRepositoryErCoreAsync(context);
await repository.AddVideoAsync(new Video { Title = "Test", Company = "test", Url = "www.naver.com", CreateBy = "홍길동" });
await repository.AddVideoAsync(new Video { Title = "Test1", Company = "test", Url = "www.naver.com", CreateBy = "홍길동1" });
await repository.AddVideoAsync(new Video { Title = "Test2", Company = "test", Url = "www.naver.com", CreateBy = "홍길동2" });
}
using (var context = new VideoDbContext(options))
{
var repository = new VideoRepositoryErCoreAsync(context);
var video = await repository.GetVideoByIdAsync(1);
Assert.AreEqual("www.naver.com1", video.Url);
Assert.AreEqual("홍길동", context.Videos.Where(x => x.Id == 1).SingleOrDefault().CreateBy);
}
}
[TestMethod]
public async Task DeleteVideo()
{
var options = new DbContextOptionsBuilder<VideoDbContext>().UseInMemoryDatabase(databaseName: "DeleteVideo").Options;
using (var context = new VideoDbContext(options))
{
var repository = new VideoRepositoryErCoreAsync(context);
await repository.AddVideoAsync(new Video { Title = "Test", Company = "test", Url = "www.naver.com", CreateBy = "홍길동" });
await repository.AddVideoAsync(new Video { Title = "Test1", Company = "test", Url = "www.naver.com", CreateBy = "홍길동1" });
await repository.AddVideoAsync(new Video { Title = "Test2", Company = "test", Url = "www.naver.com", CreateBy = "홍길동2" });
}
using (var context = new VideoDbContext(options))
{
var repository = new VideoRepositoryErCoreAsync(context);
await repository.RemoveVideoAsync(1);
var vidoes = await repository.GetVideosAsync();
Assert.AreEqual(2, vidoes.Count());
Assert.IsNull(vidoes.Where(x => x.Id == 1).SingleOrDefault());
}
}
[TestMethod]
public async Task UpdateVideo()
{
var options = new DbContextOptionsBuilder<VideoDbContext>().UseInMemoryDatabase(databaseName: "UpdateVideo").Options;
using (var context = new VideoDbContext(options))
{
var repository = new VideoRepositoryErCoreAsync(context);
await repository.AddVideoAsync(new Video { Title = "Test", Company = "test", Url = "www.naver.com", CreateBy = "홍길동" });
await repository.AddVideoAsync(new Video { Title = "Test1", Company = "test", Url = "www.naver.com", CreateBy = "홍길동1" });
await repository.AddVideoAsync(new Video { Title = "Test2", Company = "test", Url = "www.naver.com", CreateBy = "홍길동2" });
}
using (var context = new VideoDbContext(options))
{
var repository = new VideoRepositoryErCoreAsync(context);
var video = await repository.GetVideoByIdAsync(1);
video.CreateBy = "T1";
await repository.UpdateVideoAsync(video);
var updateVideo = await repository.GetVideoByIdAsync(1);
Assert.AreEqual("T1", updateVideo.CreateBy);
}
}
}
}
1.2. 코드 테스트 하기
- 오른쪽 마우스를 누르고 테스트 실행을 누르면 테스트 탐색기창 팝업
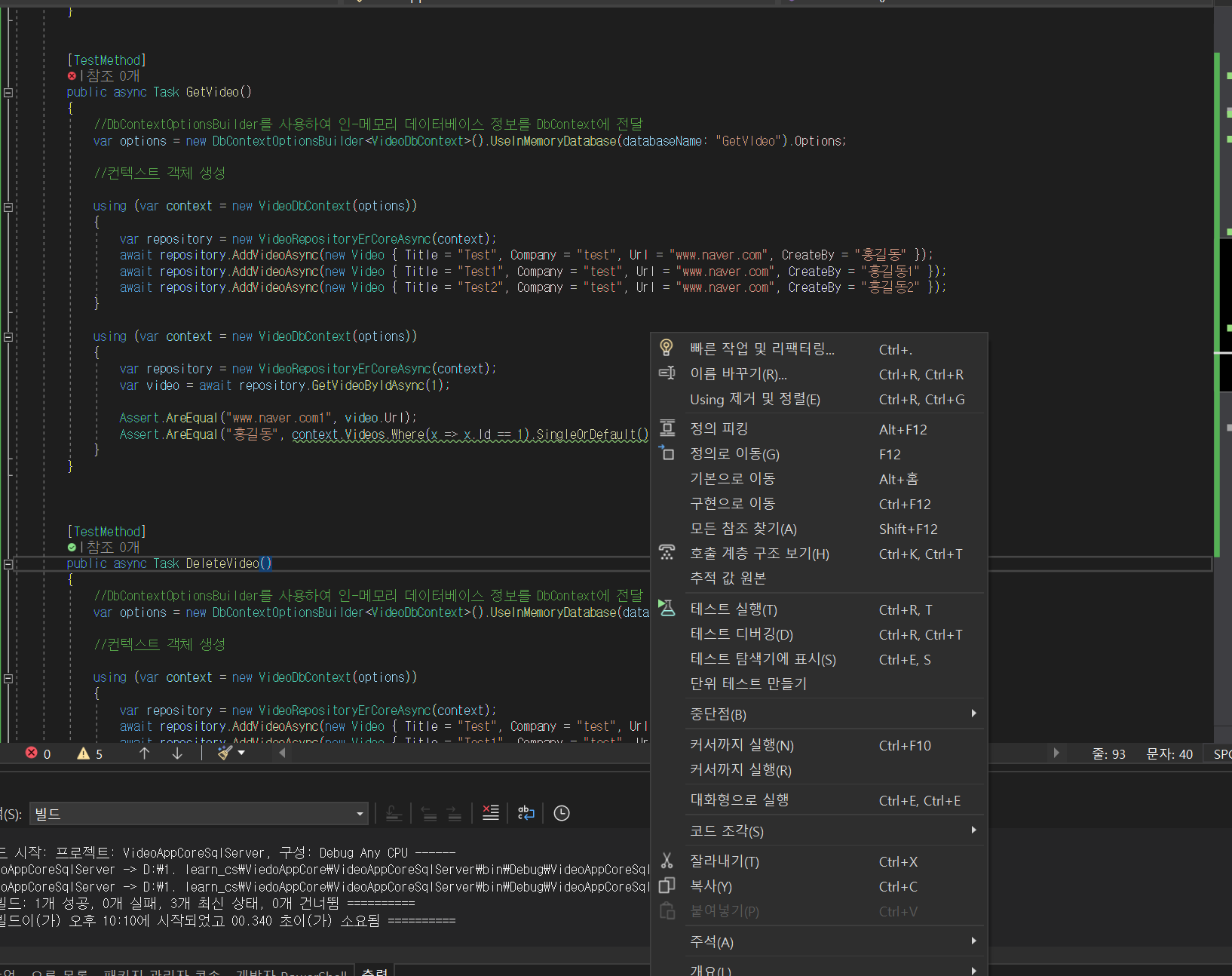
- 메소드 단위로 테스트 가능 하며 전체 메소드 테스트 실행도 가능.
- 에러가난 메소드는 아래와 같이 붉은색 x로 표시되며 통과된 메소드는 녹색의 체크표시가 됨.
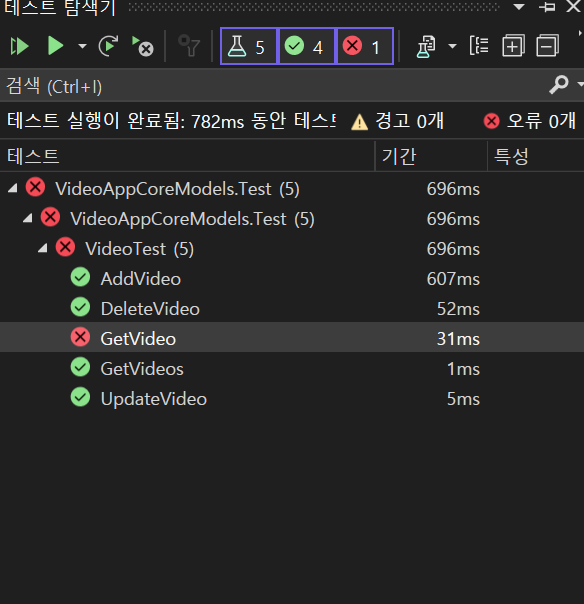
- GetVideo 메소드는 일부로 에러를 냈으며 아래 코드에서
Assert.AreEqual("www.naver.com1", video.Url);
해당 코드때문에 에러가 났으며 사유는 id가 1번인 객체의 url = "www.naver.com"인데 검사 코드에서는 com1로 끝나기 때문에 검사 코드에서 에러가 발생 함.
[TestMethod]
public async Task GetVideo()
{
var options = new DbContextOptionsBuilder<VideoDbContext>().UseInMemoryDatabase(databaseName: "GetVIdeo").Options;
using (var context = new VideoDbContext(options))
{
var repository = new VideoRepositoryErCoreAsync(context);
await repository.AddVideoAsync(new Video { Title = "Test", Company = "test", Url = "www.naver.com", CreateBy = "홍길동" });
await repository.AddVideoAsync(new Video { Title = "Test1", Company = "test", Url = "www.naver.com", CreateBy = "홍길동1" });
await repository.AddVideoAsync(new Video { Title = "Test2", Company = "test", Url = "www.naver.com", CreateBy = "홍길동2" });
}
using (var context = new VideoDbContext(options))
{
var repository = new VideoRepositoryErCoreAsync(context);
var video = await repository.GetVideoByIdAsync(1);
Assert.AreEqual("www.naver.com1", video.Url);
Assert.AreEqual("홍길동", context.Videos.Where(x => x.Id == 1).SingleOrDefault().CreateBy);
}
}