Front-end frameworks and Single Page Applications
Short history of JavaScript Front-end
- Aligned based on their first appearance to the market
- jQuery was very popular
- React from Facebook (Meta)
- Angular from Google

Recent trend on a front-end framework
- In the last 10 years, much of the code that was normally on the server side has migrated into the browser. As that has grown more complex, so have the frameworks to keep things more organized.
- Simultaneously, browsers are also getting faster.
- The rise of SPA (Single Page Application) frameworks
- In a SPA, the browser initially loads one HTML document.
- As users navigate through the site, they stay on the same page.
- It may feel as though you’re jumping from page to page, but you’re still on the same HTML page
- Various libraries and frameworks have emerged:
- jQuery is a JavaScript library (write less, do more) even if it makes the use of DOM control and AJAX much easier.
- Angular, React, Vue are frameworks are modern JavaScript frameworks
Ajax
Addressing WebApp challenges with Ajax (Asynchronous JavaScript And XML)
- Solve key problems of Web apps(기존 문제점)
- Coarse-grained updates (entire web page)
- Synchronous: you are frozen while waiting for the result
- Continued Browser-based interactivity
- Ajax is about “what is the best you can do with what everyone already has in their browser?”
- “Real” browser-based active content
- Early failure: Java Applets
- Not universally supported; can’t interact with the HTML
- Serious alternative: Flash/Flex
- Not preinstalled on all PCs; not available for iPhone/iPad
- Recent failures
- Microsoft Silverlight
- JavaFX
Traditional Web Apps vs. Ajax Apps
- Traditional Web Apps: Infrequent Large Updates
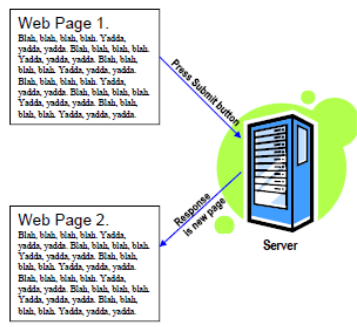
- Before: 사용자가 새로운 요청을 낼 때 마다 서버에 요청
- Ajax:
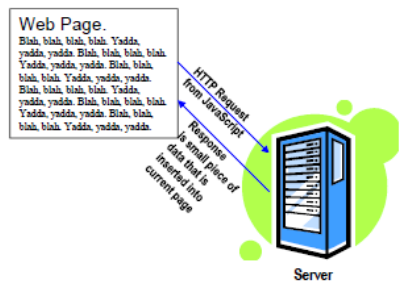
- Ajax
- 갱신될 부분만 요청 -> 응답
- Script만으로도 처리
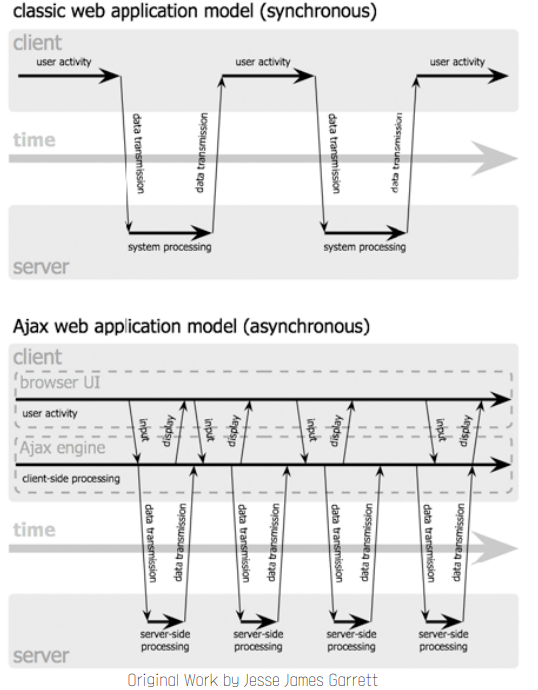
Ajax는 자바스크립트를 이용해서 비동기적(Asynchronous)으로 서버와 브라우저가 데이터를 교환할 수 있는 통신 방식을 의미한다
페이지 전체를 로드하여 렌더링할 필요가 없고 갱신이 필요한 일부만 로드하여 갱신하면 되므로 빠른 퍼포먼스와 부드러운 화면 표시 효과를 기대할 수 있다.
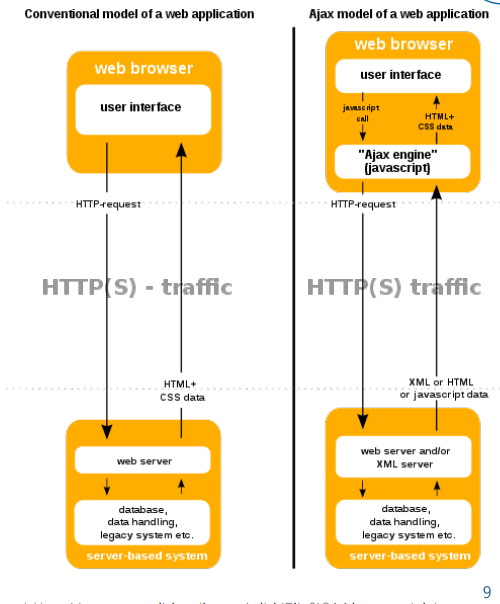
Ajax Request
- Define an object for sending HTTP requests
- To make an HTTP request to the server with JavaScript, you need an instance of an object with the necessary functionality, commonly with XMLHttpRequest
- Initiate request: request method (GET or POST) + the target url
- Setting the response handler function: Define a function that will handle the response received from the server
- Assigning the Response Handler: Set the previously defined response handler function as the onreadystatechange attribute of your request object.
- This ensures that the function is called when the request state changes.
- Initiating the request
- Sending data
-> Whenever an AJAX request is sent to the server, a special header named XRequested-With with a value of XMLHttpRequest is attached to the request
ReactJS Overview
- JavaScript framework for writing the web application
- Created by Jordan Walke, a software engineer at Facebook. At JSConf 2013, React was made open source.
- At that time, React was described as “the V in MVC.” In other words, React components act as the view layer or the user interface for your JavaScript applications.
- Use Model-View-Controller pattern
- View constructed from Components using pattern
- Optional, but commonly used HTML templating
- Focus on support for programming in large-scale application and single-page applications
Example: a default single-page application
$ npx create-react-app my-app
$ cd my-app
$ npm start
- npx is a package runner tool that comes with npm 5.2+
- Create React App: a powerful tool for setting up React applications.
- doesn’t handle backend logic or databases; it just creates a frontend build pipeline
- Under the hood:
- It uses Babel(Compiler) and webpack(Bundler) However, for most developers, it's not necessary to delve(조사,탐구하다) into the intricate(복잡한) details of these technologies
Files in the project
- public/index.html
- The HTML file that is loaded by the browser. It contains an element where the application is displayed and a script element that loads the application’s JavaScript files.
- src/index.js
- The JavaScript files that are responsible for configuring and starting the React application.
- src/App.js
- The React component, which contains the HTML content that will be displayed to the user and the JavaScript code required by the HTML
React and React-DOM
- React is a library designed to update the browser DOM for us.
- With React, we do not interact with the DOM API
directly. Instead, we provide declarative instructions to React, specifying what we want it to construct and React assumes(~을 맡다) responsibility for rendering and reconciling(조정하다) the elements we've defined.
- The traditional browser DOM is made up of individual DOM elements.
Similarly, in React, we work with React elements, which serve as blueprints(청사진) for the actual DOM elements.
- A React element is a description of what the actual DOM element should look like.
React 요소는 실제 DOM 요소의 모양에 대한 묘사입니다.
- React elements are the instructions for how the browser DOM should be created.
- Create a React element to represent an h1 and React will convert this to an actual DOM element
React.createElement(“h1”, {id: “recipe-0”}, “Baked Salmon”);
<h1 id=“recipe-0”>Baked Salmon<h1>
- React-dom package provides DOM-specific methods that can be used at the top level of your app and as an escape hatch to get outside the React model if you need to.
"React-dom" 패키지는 앱의 최상위 수준에서 사용할 수 있는 DOM 특화 메서드를 제공하며, 필요한 경우 React 모델을 벗어나기 위한 탈출구(escape hatch) 역할을 할 수 있습니다.
const root = ReactDOM.createRoot(document.getElementById('root'));
- ReactDOM.createRoot is new API in React 18. ReactDOM.render is no longer supported in React 18.
const element = <App />;
ReactDOM.render(element, document.getElementById('root'));
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(<App />);
https://velog.io/@yeum0523/React-vs-React-DOM
React는 User Interface 컴포넌트 형태로 구성하는 라이브러리일 뿐이며 웹 또는 브라우저를 위한 라이브러리는 아닙니다.
React는 컴포넌트와 props, state, context를 관리하며, 이들의 변경 사항을 파악하고 변경 사항 snap shot을 React-DOM에 전달합니다
React-DOM은 웹 인터페이스로 웹과 직접적인 연관이 있으며 실제 HTML 요소를 화면에 불러오는 역할을 합니다.
따라서, React-DOM은 React로 부터 받은 변경 사항 snap shot과 실제 브라우저 DOM을 비교하며 차이점을 확인 후, 실제 DOM을 조작합니다.
Example: Simple ToDo
App.js
import ToDo from './components/ToDo';
function App() {
const todo = [
{ item: 'Send notification', urgent: 'Yes'},
{ item: 'Submit revision', urgent: 'No'}
];
return (
<div>
<h2>What a wonderful world!</h2>
<ToDo item={todo[0].item} urgent={todo[0].urgent}></ToDo>
<ToDo item={todo[1].item} urgent={todo[1].urgent}></ToDo>
</div>
);
}
export default App;
Example: Simple ToDo
Components/ToDo.js
import './ToDo.css';
export default function ToDo(props) {
return (
<div>
<div className="todoItems"> {props.item}
</div>
<div className="todoUrgency"> {props.urgent}
</div>
</div>
);
}
Deploying a React-based frontend application with an Express-based backend
- Prepare Your Frontend and Backend Code
- Make sure your backend API endpoints are correctly defined and tested.
- Deploy the Backend and configure CORS (if you deploy on different origins)
- To enable cross-origin requests from your React frontend to your Express backend, configure Cross-Origin Resource Sharing (CORS) settings on your Express server.
- Serve the React Build with Express
Express 서버를 사용하여 React 애플리케이션의 빌드(static files)를 제공
- Configure your Express server to serve the production build of your React app. You can use the
express.static
middleware to serve the static files from the build directory.
- Configure the Frontend to Use the Backend API
- Build and Deploy the Frontend:
- Deploy your React production build to the same server or a different hosting service, depending on your choice. Make sure your frontend can make requests to your Express backend using the correct URL
Appendix (XMLHttpRequest)
Example
<button id="ajaxButton" type="button">Make a request</button>
<script>
(() => {
let httpRequest;
document .getElementById("ajaxButton") .addEventListener("click", makeRequest);
function makeRequest() {
httpRequest = new XMLHttpRequest();
if (!httpRequest) {
alert("Giving up :( Cannot create an XMLHTTP instance");
return false;
}
httpRequest.onreadystatechange = alertContents;
httpRequest.open("GET", "test.html");
httpRequest.send();
}
function alertContents() {
if (httpRequest.readyState === XMLHttpRequest.DONE) {
if (httpRequest.status === 200) {
alert(httpRequest.responseText);
} else {
alert("There was a problem with the request.");
}
}
}
})();
</script>
Initiate Request
function sendRequest() {
var request = getRequestObject();
request.onreadystatechange = function() { handleResponse(request) };
request.open("GET", “http://www.example.org/some.file”, true);
request.send();
}
request.open("GET","demo_get2.asp?fname=Henry&lname=Ford",true);
request.send();
request.open("POST","ajax_test.asp",true);
request.setRequestHeader("Content-type","application/x-www-form-urlencoded");
request.send("fname=Henry&lname=Ford");
The Basic Ajax Process (response)
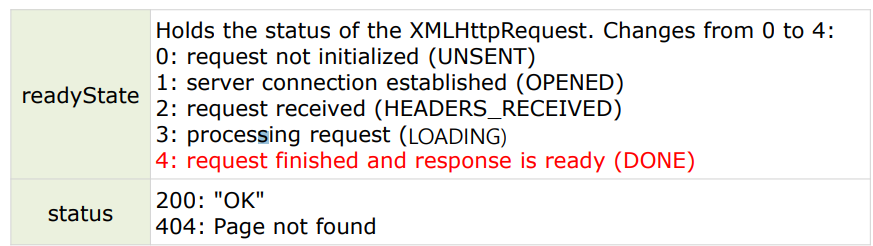
- Handle response
- Wait for readyState of 4 and HTTP status of 200
- Extract return text with responseText or responseXML
- Do something with result
Check readyState and status codes
if (httpRequest.readyState === XMLHttpRequest.DONE) {
} else {
}
if (httpRequest.status === 200) {
} else {
}
Processing data
- two options to access that data
- httpRequest.responseText – returns the server response as a string of text
- httpRequest.responseXML – returns the response as an XMLDocument object you can traverse with JavaScript DOM functions
Appendix (MVC)
Model View Controller (MVC)
- It started as a framework developed by Trygve Reenskaug for the Smalltalk platform in the late 1970s. Since then it has played an influential role in most UI frameworks and in the thinking about UI design
- Specially in Web Application Development
MVC Misconceptions
- An elaborate framework is necessary
- Frameworks are often useful
- However, they are not must-be-used!
- MVC totally(?) changes your system design
- You can use MVC for individual requests
- Think of it as the MVC approach, not the MVC architecture
MVC: Model-View-Controller
- 3 parts: proper places for every piece of code
- MVC defines the way objects communicate with each other. Each type of
object is separated from the others and communicates with other types
across those boundaries.
Model View Controller
- Decouples data and presentation
- Eases the development
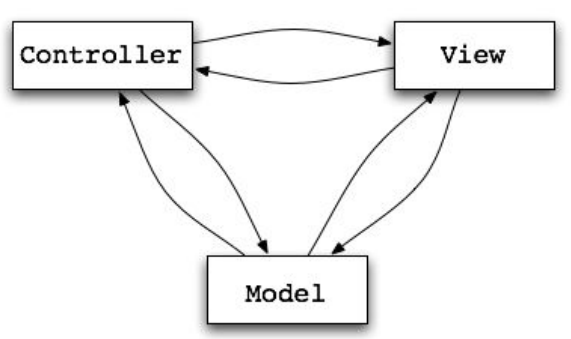
First Thought MVC
- Tier 1: View (Client)
- Tier 2: Controller (Server)
- Tier 3: Model (Database)
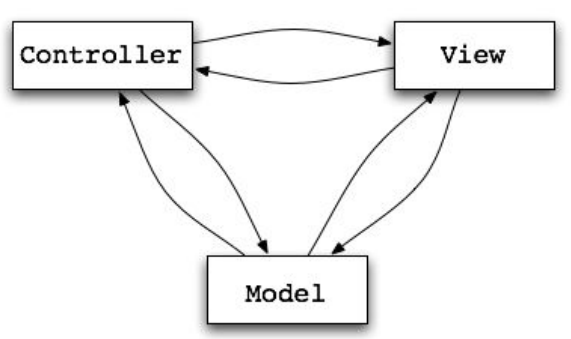
Becomes
- Presentation:
- View is the user interface (e.g. button)
- Controller is the code (e.g. callback for button)
- Data:
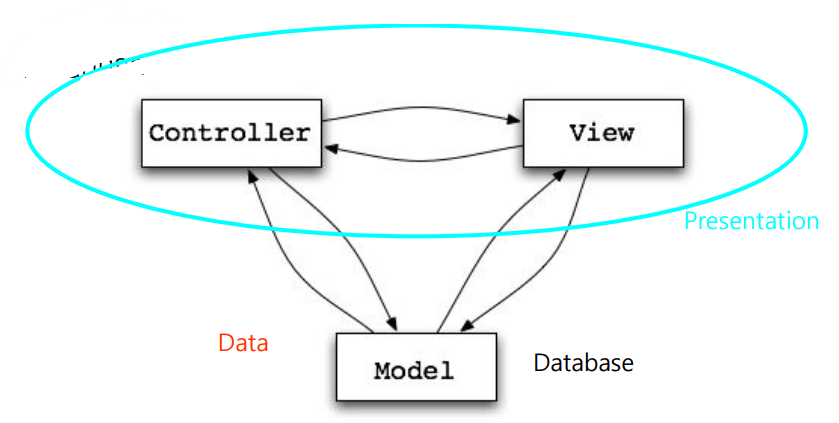
Example Control Flow in MVC
- User interacts with the VIEW UI
- CONTROLLER handles the user input (often a callback function attached to UI elements)
- CONTROLLER updates the MODEL
- VIEW uses MODEL to generate new UI
- UI waits for user interaction
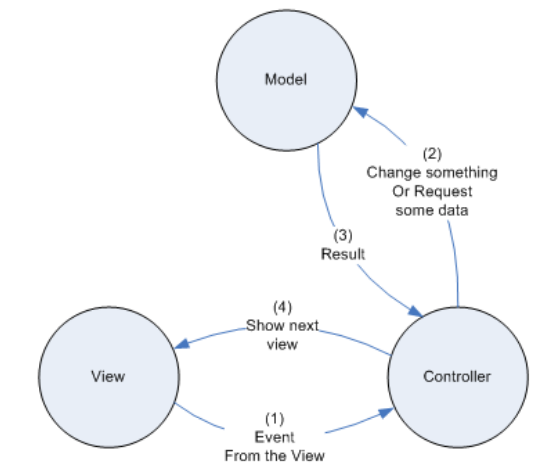