Recall to Fig. 2.1 for SIC Machine
Machine-dependent Assembler Features
- Assemblers depend on instruction format and addressing mode.
- Figure 2.6 shows the example program from Fig. 2.1 with object code as it might be rewritten to take advantage of the SIC/XE instruction set.
- Indirect addressing is indicated by adding the prefix “@” to the operand
- Immediate operands are denoted with the prefix “#”
- Format 4 instruction (= 4-byte extended format) is specified with the prefix “+” added to the operation code
- The assembler directive “BASE” is used in conjunction with base relative addressing
It is the programmer’s responsibility to specify this form of addressing when it is required!
[Fig. 2.6] SIC/XE Assembly Program with Object Code
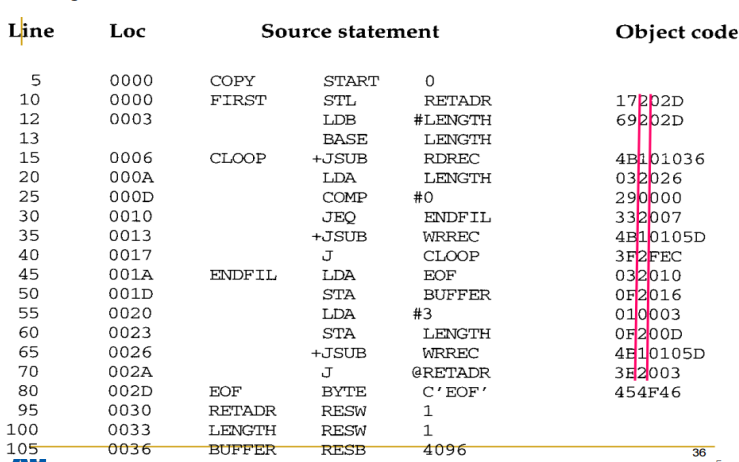
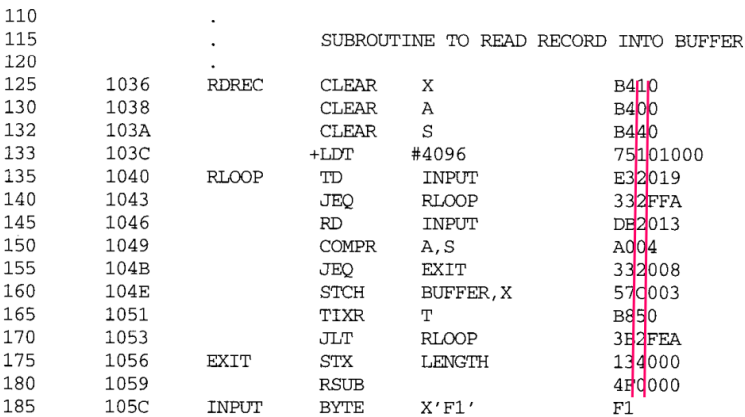
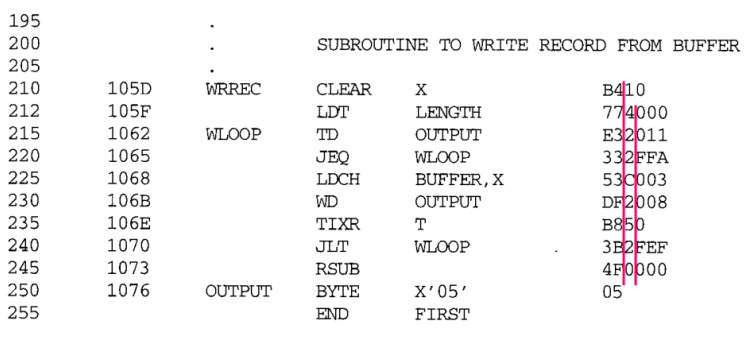
What does SIC/XE Assembler Do?
- Two main differences of Fig. 2.6 version from Fig. 2.1
- The use of register-to-register instructions, wherever possible
- Ex) COMP ZERO (in Fig. 2.1) -> COMPR A,S (in Fig. 2.6)
- The use of immediate & indirect addressing, as much as possible
- These changes take advantage of the more advanced SIC/XE architecture to improve the execution speed of the program
- Register-to-register instructions are faster than the corresponding register-to-memory operations because they are shorter and, more importantly,
because they do not require another memory reference
- When using immediate addressing, the operand is already present as part of the instruction and need not be fetched from anywhere
- When using indirect addressing, it often avoids the need for another
instruction (as in the “return” operation on line 70).
The Magic
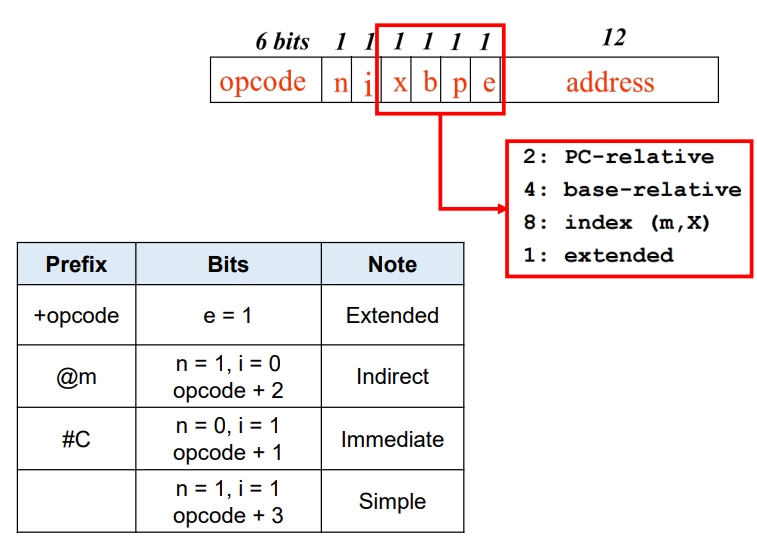
Translation of Register-to-Register Instructions
- The assembler must simply convert the mnemonic operation code to machine language (using OPTAB), and change each register mnemonic to its numeric equivalent.
- Such a change of register mnemonic to numbers can be done with a separate table; however, it is often convenient to use SYMTAB for this purpose.
- To do this, SYMTAB would be preloaded with the register names (A, X, etc) and their values (0, 1, etc).
- A(0), X(1), L(2), B(3), S(4), T(5), F(6), PC(8), SW(9)
- Ex) Line 150: 1049
COMPR A,S
A004
COMPR
-> A0,
A
-> 0 ,
S
-> 4
Translation of Register-to-Memory Instructions
- Format 3 Instructions that refer to memory are normally assembled using either the program-counter relative or the base relative addressing mode.
- In either case, the assembler must calculate a displacement to get the correct target address (i.e., to be assembled as part of the object instruction)
- Target Address = (PC) or (B) + displacement
- The resulting displacement must be small enough to fit in the 12-bit field in the instruction.
- Program-counter relative (disp: -2048 to +2047)
- Base relative (disp: 0 to 4095)
- The assembler attempts to translate format 3 instructions using
- PC relative, first;
- Base relative (if the required displacement is out of range for PC relative mode)
- Error message generated (if displacement is invalid in both relative modes)
Example of PC Relative Addressing 1
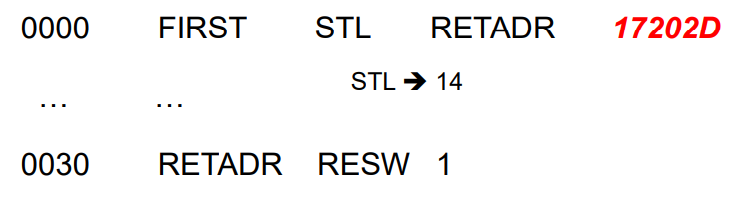
17 => SIC/XE
이므로 n=1, i=1이므로 기존 STL(14)에 3을 더해준 17이 된다.
2 => PC relative Addressing
이므로 2이다. The magic을 참고하자.
02D => 현재 PC 값은 0003이고 RETADR의 주소 값은 0030이다. 따라서 0030-0003 = 002D가 된다.
Example of PC Relative Addressing 2
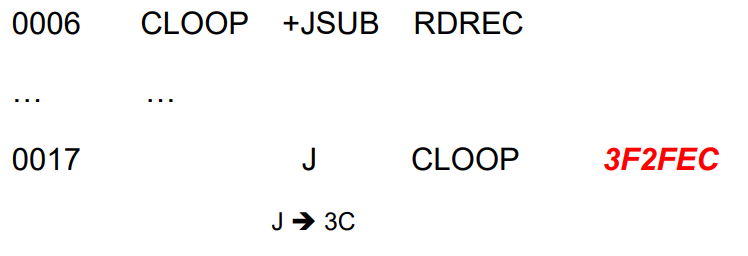
3F => SIC/XE
이므로 n=1, i=1이므로 기존 J(3C)에 3을 더해준 3F가 된다.
2 => PC relative Addressing
이므로 2이다.
FEC => 현재 PC 값은 001A이고 CLOOP의 주소 값은 0006이다. 따라서 006-01A = -014
- -(014) = - (0000 0001 0100) = 1111 1110 1100 = F E C
- The displacement calculation process for base relative addressing is much the same as for PC relative addressing
- The assembler knows what the contents of the program counter will be at execution time.
- The base register, on the other hand, is under control of the programmer.
Therefore, the programmer must tell the assembler what the base register will contain during execution of the program so that the assembler can compute displacement
- This is done with the assembler directive BASE
- Ex) The statement BASE LENGTH (line 13) informs the assembler that the base register will contain the address of LENGTH. The preceding instruction (LDB #LENGTH) loads this value into the register during program execution
- The directive NOBASE can be used to free the B register
Example of Base Relative Addressing
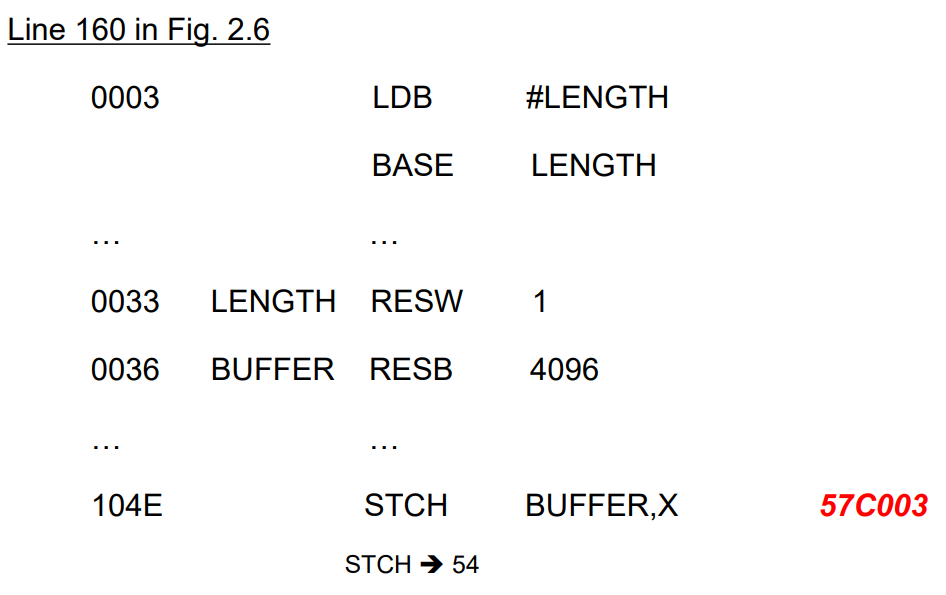
57 => SIC/XE
이므로 n=1, i=1이므로 기존 STCH(54)에 3을 더해준 57이 된다.
C => base addressing(4)과 indexed addressing(8)을 함께 쓰므로 4+8 = C가 된다. 정확히는 x=1, b=1, p=e=0이므로 1100 = C
003 => disp
= TA
- Base
= 0036 - 0033 = 0003
- If neither PC relative nor base relative addressing can be used (because the displacements required for both relative addressing modes are too large to fit into a 3-byte instruction), then the 4-byte extended instruction format must be used.
- This 4-byte format contains a 20-bit address field, which is large enough to contain the full memory address
- In this case, there is no displacement to be calculated
- Note that the programmer must specify the extended format by using the prefix “+”. What if not specified by the programmer? … Our assembler attempts to translate the instruction as format 3 using
- PC relative, first;
- Base relative (if the required displacement is out of range for PC relative mode)
- Error message generated (if displacement is invalid in both relative modes)
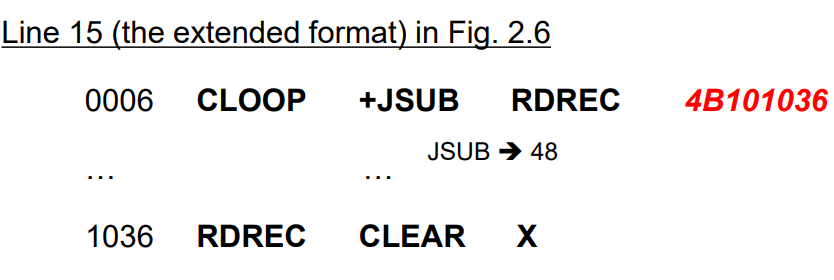
4B => SIC/XE
이므로 n=1, i=1이므로 기존 JSUB(48)에 3을 더해준 4B이 된다
1 => extended이므로 1이다.
01036 => RDREC의 메모리 주소가 1036이므로 그대로 집어넣는다.
- The assembly of an instruction that specifies immediate addressing is simpler because no memory reference is involved.
- The assembler will convert the immediate operand to its internal representation and insert it into the instruction
- Ex) Line 55: 0020 LDA #3 010003
- In the case the operand is too large to fit into the 12-bit displacement field, the 20-bit extended instruction format is called for.
- Ex) Line 133: 103C +LDT #4096 75101000
- What if the operand is too large even for the 20-bit address field?
» Just define the operand value in a memory region, and use relative or direct addressing
- In the case that the operand is the symbol, its address value is inserted into the instruction
- If necessary, the displacement calculation is performed.
- Ex) Line 12 : 0003 LDB #LENGTH 69202D
» Immediate addressing + PC-relative addressing
Program Relocation
-
Multiprogramming
- Executing more than one program at a time while sharing the memory and other resources
- Important to use memory without overlap or wasted space
- Load program into memory wherever there is room for it
-
The assembler does not know the actual location of the memory where the program will be loaded.
-
어셈블러는 프로그램이 로딩될 메모리의 실제 위치를 알지 못합니다.
- The actual starting address of the program is NOT known until load time.
- 로드 시간이 지나야 프로그램의 실제 시작 주소를 알 수 있습니다.
-
The assembler informs the loader about the parts of the object program that need modification (-> relocatable object program)
-
어셈블러는 로더에게 수정이 필요한 객체 프로그램의 일부에 대해 알려줍니다
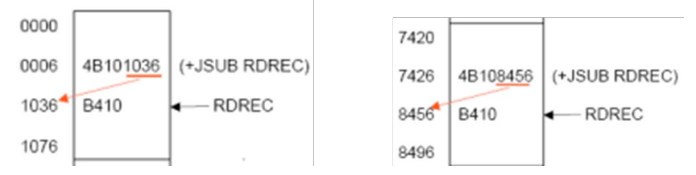
-
Solution for relocation problem
- The assembler produces command for the loader to add the beginning address to some instructions’ address fields -> This command will be a part of the object program (with Modification record).
- 어셈블러는 로더가 일부 명령어의 주소 필드에 시작 주소를 추가하는 명령을 생성합니다 -> 이 명령어는 개체 프로그램의 일부가 됩니다(수정 레코드 포함)
- The loader generates addresses relative to the start of the program and modifies the instructions.
- 로더는 프로그램 시작과 관련된 주소를 생성하고 명령을 수정합니다.
-
Modification records
- “M”, starting location, length of the address field to be modified
- Col. 1 M
- Col. 2-7 Starting location of the address field to be modified, relative to the beginning of the program (Hex)
» The starting location is the location of the byte containing the leftmost bits of the address field to be modified.
- Col. 8-9 Length of the address field to be modified, in half-bytes (Hex)
-
No modification
- Either when an instruction operand is not a memory address, like the statement ‘CLEAR S’
- Or when the operand is specified using PC relative or base relative addressing, like the statement ‘STL RETADR’
-
In summary, the only parts of the program that require modification at load time are those that specify direct addresses (not relative addresses).
- For SIC/XE program, direct addresses are found in Format 4 instructions.
Fig. 2.7: Examples of Program Relocation
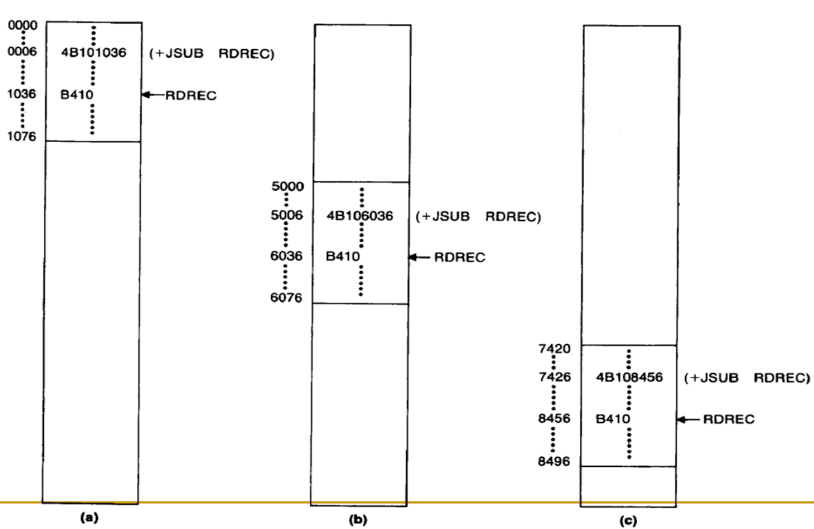
Fig. 2.8: Object Program (corresponding to Fig. 2.6)
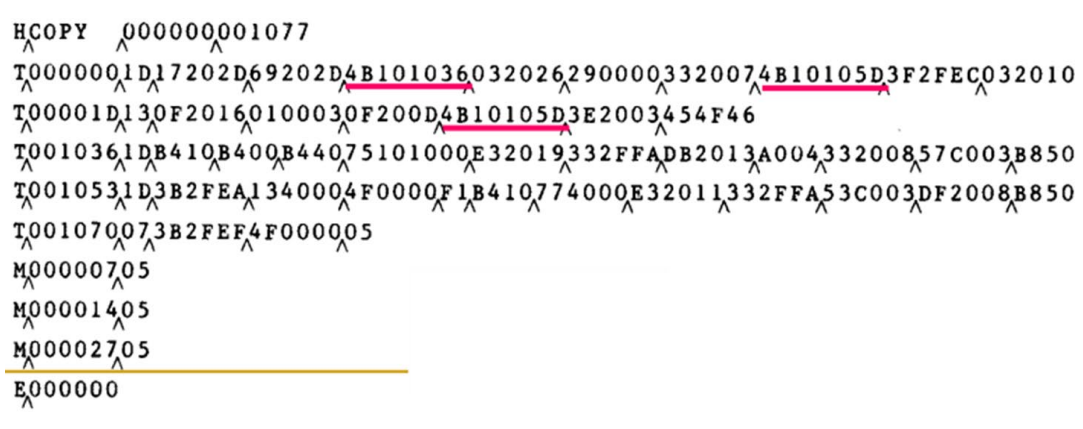
M 000007 05
시작 지점(0으로 시작한다)을 기준으로 7바이트 이동한다.
17(0번째) 20(1번째) 2D(2번째) .... 10(7번째)
첫번째 빨간줄 10에 도착한다.
앞의 1은 xbpe 비트 구간이고 뒤의 01036이 변경 되어야 한다.
여기서 05는 5바이트 이지만 수정 format에서는 half-byte인 것을 기억하자.
나머지는 로직 동일함.
이러한 수정과정은 multiprograming 때문이라는 것을 명심.
format 3의 해석 순서 pc -> base
error 발생시 프로그래머가 처리한다.
relocation 할때 어떤 방식을 한다던지
각 필드가 어떤 의미인지.
어떤 instruction을 수정하려고 하는건지