리액트 훅은 리액트 클래스형 컴포넌트에서 이용하던 코드를 작성할 필요없이 함수형 컴포넌트에서 다양한 기능알 사용할 수 있게 만들어준 라이브러리라고 할 수 있는데 React 16.8 버전에 새로 추가된 기능이다. 이는 함수형 컴포넌트에 맞게 만들어진 것으로 함수형 컴포넌에서만 사용 가능하다.
useState는 상태를 관리하는 훅으로 다음과 같은 특징을 가진다.
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
import FirstApp from './components/FirstApp';
import SecondApp from './components/SecondApp';
import ThirdApp from './components/ThirdApp';
import FourthApp from './components/FourthApp';
import FifthApp from "./components/FifthApp";
// const root = ReactDOM.createRoot(document.getElementById('root')); root라는 곳을 찾아보자 public 폴더의 index.html에 있다. 즉, 결국 디자인은 html 파일에서 기입하고. idnex.js에서 import 받아서 한번에 출력하는 것이다.
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<React.StrictMode>
{/* <App /> */}
{/* <App /> 주석처리 하면 화면 안나온다. export한 것을 받아와서 결국 화면에 띄워주는 곳은 여기이다. */}
{/* <FirstApp/> */}
{/* <SecondApp></SecondApp> */}
{/*<ThirdApp/>*/}
{/*<FourthApp/>*/}
<FifthApp/>
</React.StrictMode>
);
// If you want to start measuring performance in your app, pass a function
// to log results (for example: reportWebVitals(console.log))
// or send to an analytics endpoint. Learn more: https://bit.ly/CRA-vitals
reportWebVitals();
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<link rel="icon" href="%PUBLIC_URL%/favicon.ico" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.2/dist/css/bootstrap.min.css" rel="stylesheet">
<meta name="theme-color" content="#000000" />
<meta
name="description"
content="Web site created using create-react-app"
/>
<link rel="apple-touch-icon" href="%PUBLIC_URL%/logo192.png" />
<!--
manifest.json provides metadata used when your web app is installed on a
user's mobile device or desktop. See https://developers.google.com/web/fundamentals/web-app-manifest/
-->
<link rel="manifest" href="%PUBLIC_URL%/manifest.json" />
<!--
Notice the use of %PUBLIC_URL% in the tags above.
It will be replaced with the URL of the `public` folder during the build.
Only files inside the `public` folder can be referenced from the HTML.
Unlike "/favicon.ico" or "favicon.ico", "%PUBLIC_URL%/favicon.ico" will
work correctly both with client-side routing and a non-root public URL.
Learn how to configure a non-root public URL by running `npm run build`.
-->
<title>React App</title>
</head>
<body>
<noscript>You need to enable JavaScript to run this app.</noscript>
<div id="root"></div> <!-- 여기에 root가 있네요 -->
<!--
This HTML file is a template.
If you open it directly in the browser, you will see an empty page.
You can add webfonts, meta tags, or analytics to this file.
The build step will place the bundled scripts into the <body> tag.
To begin the development, run `npm start` or `yarn start`.
To create a production bundle, use `npm run build` or `yarn build`.
-->
</body>
</html>
import React from 'react';
import './MyStyle.css';
import cat1 from '../image/2.jpg';
import cat2 from '../image/3.jpg';
import cat3 from '../image/6.jpg';
function FirstApp(props) {
// 스타일을 변수에 지정
const styleImg1={
width:'200px',
border:'5px solid gray',
marginLeft:'30px',
marginTop:'20px'
}
return (
<div>
{/* <h2 className='alert alert-warning'>FirstApp Component!!!</h2> */}
<h2 className='line2'>FirstApp Component!!!</h2>
<div style={{fontSize:'30px',marginLeft:'100px',backgroundColor:'salmon'}}>
안녕~~ 오늘은 목요일이야!!
</div>
{/* src의 이미지는 import */}
<img src={cat1} style={styleImg1}/>
<img src={cat2} style={{width:'200px',border:'3px dotted pink',marginLeft:'20px'}}/>
{/* public image는 직접 호출 가능합니다. */}
<hr/>
<h3> public image </h3>
<img src='../image2/17.jpg' style={{width:'250px',marginLeft:'20px'}}/>
</div>
);
}
export default FirstApp;
import React from 'react';
import cat4 from '../image/6.jpg'
function SecondApp(props) {
const catStyle={
width:'200px',
marginLeft:'30px'
}
let helloEle=<h2 className='alert alert-info'>Hello~~</h2>
return (
<div>
<h2 className='alert alert-danger'>SecondApp 입니다.</h2>
<img src='../image2/19.jpg' style={catStyle}/>
<img src={cat4} style={catStyle}/>
{helloEle}
{helloEle}
{helloEle}
{helloEle}
{helloEle}
</div>
);
}
export default SecondApp;
const enterEvent=(e)=>{ // 엔터 이벤트 함수
if(e.key==='Enter'){
setMessage('');
e.target.value='';
}
}
// const enterEvent=(e)=>{
// if(e.keyCode===13){
// setMessage('');
// e.target.value='';
// }
// }
onChange={(e)=>{
console.log(e.target.value); // 인터넷 콘솔창 확인용
// message 변수에 입력값 넣기
setMessage(e.target.value); // 실제 event
}}
import React, { useState } from 'react';
function ThirdApp(props) {
// 상태관리를 위한 변수설정
const [message,setMessage]=useState('기본값');
// 엔터 이벤트 함수 (Enter 도 가능하고, 13도 가능하다)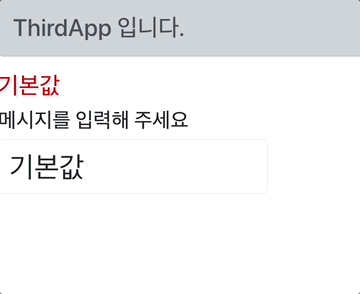
const enterEvent=(e)=>{ // 엔터 이벤트 함수
if(e.key==='Enter'){
setMessage('');
e.target.value='';
}
}
// const enterEvent=(e)=>{
// if(e.keyCode===13){
// setMessage('');
// e.target.value='';
// }
// }
return (
<div>
<h3 className='alert alert-dark'>ThirdApp 입니다.</h3>
<h3 style={{color:'red'}}>{message}</h3>
<h4>메시지를 입력해 주세요</h4>
<input className='form-control' type='text'
style={{width:'300px',fontSize:'2em'}} defaultValue={message}
onChange={(e)=>{
console.log(e.target.value);
// message 변수에 입력값 넣기
setMessage(e.target.value);
}}
onKeyUp={enterEvent}
/>
</div>
);
}
export default ThirdApp;
import React, { useState } from 'react';
import cat from '../image/3.jpg'
function FourthApp(props) {
const [name,setName]=useState('뽀로로');
const [age,setAge]=useState('22');
return (
<div>
<h3 className='alert alert-danger'>FourthApp 입니다.
<img className='rounded-circle' src={cat} style={{width:'200px',marginLeft:'50px'}}/>
</h3>
<br/>
<b style={{fontSize:'30px'}}>이름:{name} <span style={{marginLeft:'20px'}}>나이: {age}세</span></b>
<br/>
<button type='button' className='btn btn-info' onClick={()=>{
setName("리처드");
setAge(25);
}}>값변경</button>
<button type='button' className='btn btn-danger' style={{marginLeft:"20px"}}
onClick={()=>{
setName("");
setAge('');
}}>초기화</button>
</div>
);
}
export default FourthApp;
import React, {useState} from 'react';
function FifthApp(props) {
const [name,setName]=useState('');
const [resultName,setResultName]=useState('');
const [java,setJava]=useState(0);
const [react,setReact]=useState(0);
const [total,setTotal]=useState(0);
const [avg,setAvg]=useState(0);
return (
<div>
<h2 className='alert alert-danger' >FifthApp 입니다.</h2>
<div className={'inp'}>
이름: <input type={"text"} style={{width:"150px"}} onChange={(event)=>{
setName(event.target.value);
}}/>
<span style={{marginLeft:"10px"}}>{name}</span>
<br/><br/>
java점수: <input type={"text"} style={{width:"150px"}} onChange={(event)=>{
setJava(event.target.value);
}}/>
<span style={{marginLeft:"10px"}}>{java}</span>
<br/><br/>
react점수: <input type={"text"} style={{width:"150px"}} onChange={(event)=>{
setReact(event.target.value);
}}/>
<span style={{marginLeft:"10px"}}>{react}</span>
<br/><br/>
<button type={"button"} className={'btn btn-outline-info'} onClick={()=>{
// setTotal(parseInt({react})+parseInt({java}));
setResultName(name);
setTotal(parseInt(react)+parseInt(java));
setAvg((parseInt(react)+parseInt(java))/2);
}}>결과보기</button>
</div>
<div className='result'>
이름 : {resultName} <br/>
총합 : {total} 점 <br/>
평균 : {avg} 점
</div>
</div>
);
}
export default FifthApp;