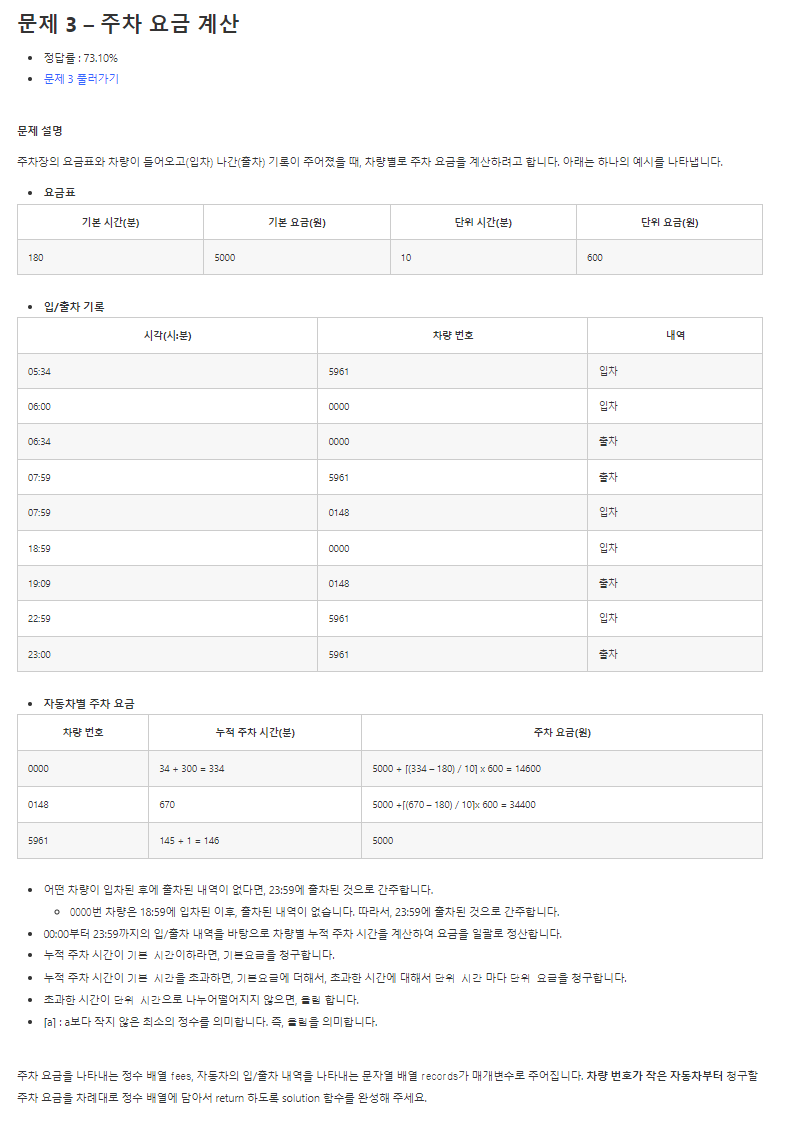
package kakao;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
public class Kakao_ParkingFee {
public static void main(String[] args) {
int[] fees = {180, 5000, 10, 600};
String[] records = {"05:34 5961 IN", "06:00 0000 IN", "06:34 0000 OUT", "07:59 5961 OUT", "07:59 0148 IN", "18:59 0000 IN", "19:09 0148 OUT", "22:59 5961 IN", "23:00 5961 OUT"};
solution(fees, records);
}
static int[] solution(int[] fees, String[] records) {
HashMap<String, String> carInfo = new HashMap<>();
HashMap<String, Integer> usedInfo = new HashMap<>();
for(String record : records) {
String time = record.split(" ")[0];
String carNum = record.split(" ")[1];
String action = record.split(" ")[2];
if("IN".equals(action)) {
carInfo.put(carNum, time);
}else if("OUT".equals(action)) {
String outTime = time;
String inTime = carInfo.get(carNum);
int usedTime = getUsedTime(inTime, outTime);
if(usedInfo.get(carNum) != null) {
int saveTime = usedInfo.get(carNum);
usedInfo.put(carNum, usedTime+saveTime);
}else {
usedInfo.put(carNum, usedTime);
}
carInfo.remove(carNum);
}
}
for(String carNum : carInfo.keySet()) {
var notOutTime = todayIsNotOutCarUsedTime(carInfo.get(carNum));
if(usedInfo.get(carNum) != null) {
int saveTime = usedInfo.get(carNum);
usedInfo.put(carNum, notOutTime+saveTime);
}else {
usedInfo.put(carNum, notOutTime);
}
}
int[] answer = new int[usedInfo.size()];
int i=0;
List<String> keyList = new ArrayList<>(usedInfo.keySet());
keyList.sort((s1, s2) -> s1.compareTo(s2));
for(String key : keyList) {
int usedTime = usedInfo.get(key);
answer[i++] = getUsedFee(fees, usedTime);
}
return answer;
}
static int getUsedTime(String startTime, String endTime) {
int startHour = Integer.parseInt(startTime.split(":")[0]);
int startMin = Integer.parseInt(startTime.split(":")[1]);
int startAllMin = (startHour * 60) + startMin;
int endHour = Integer.parseInt(endTime.split(":")[0]);
int endMin = Integer.parseInt(endTime.split(":")[1]);
int endAllMin = (endHour * 60) + endMin;
return endAllMin - startAllMin;
}
static int getUsedFee(int[] feeInfo, int usedTime) {
int basicMin = feeInfo[0];
int basicFee = feeInfo[1];
int addMin = feeInfo[2];
int addFee = feeInfo[3];
if(usedTime <= basicMin) {
return basicFee;
}
int overTime = usedTime - basicMin;
int overFee = basicFee + (overTime / addMin) * addFee;
if((overTime % addMin) != 0) {
overFee = overFee + addFee;
}
return overFee;
}
static int todayIsNotOutCarUsedTime(String inTime) {
int inHour = Integer.parseInt(inTime.split(":")[0]);
int inMin = Integer.parseInt(inTime.split(":")[1]);
int inAllMin = (inHour * 60) + inMin;
int lastTime = (23 * 60) + 59;
return lastTime - inAllMin;
}
}