🔒 문제
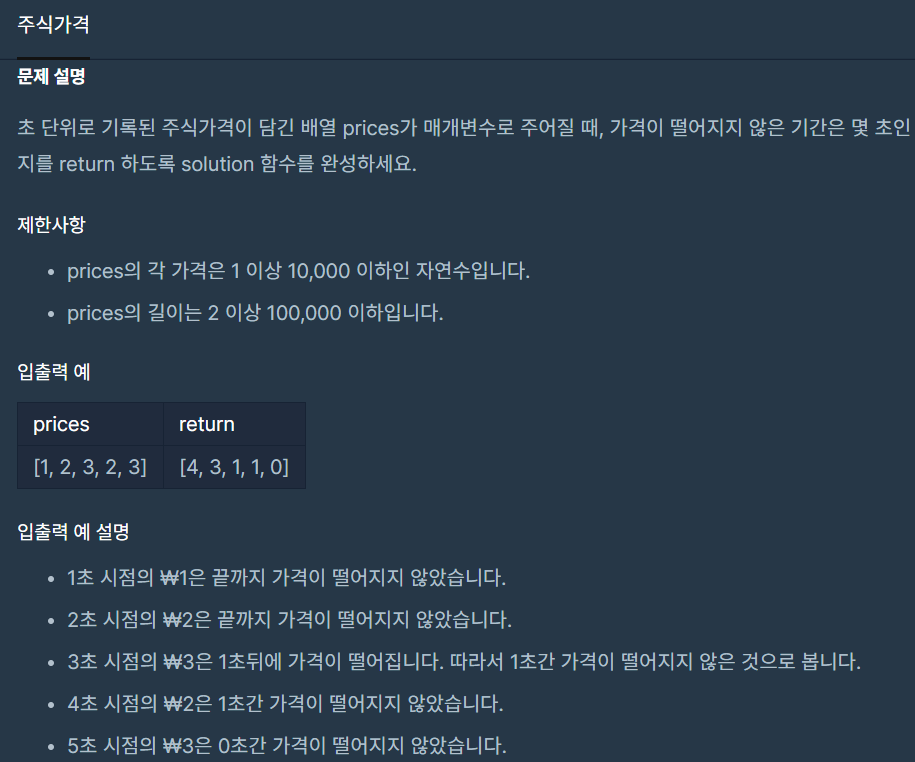
🔑 풀이
import java.util.stream.IntStream;
class Solution {
public int[] solution(int[] prices) {
for (int index = 0; index < prices.length ; index++) {
int targetPrice = prices[index];
int nextLowerPriceIndex = IntStream.range(index, prices.length)
.filter(i->prices[i] < targetPrice)
.findFirst()
.orElse(-1);
int distance = (nextLowerPriceIndex == -1) ? prices.length - index - 1 : nextLowerPriceIndex - index;
prices[index] = distance;
}
return prices;
}
}
🔧 풀이 최적화
import java.util.Stack;
public class Solution2 {
public int[] solution(int[] prices) {
var timeStack = new Stack<Integer>();
timeStack.push(0);
for (int index = 1; index < prices.length ; index++) {
while (!timeStack.empty() && prices[timeStack.peek()] > prices[index]) {
int recentElementIndex = timeStack.pop();
prices[recentElementIndex] = index - recentElementIndex;
}
timeStack.push(index);
}
while (!timeStack.empty()) {
var remainTimeIndex = timeStack.pop();
prices[remainTimeIndex] = prices.length - remainTimeIndex - 1;
}
return prices;
}
public static void main(String[] args) {
var testArray = new int[]{1,2,3,2,3};
var answerArray = new Solution2().solution(testArray);
for (int element : answerArray) {System.out.print(element);}
}
}