문제
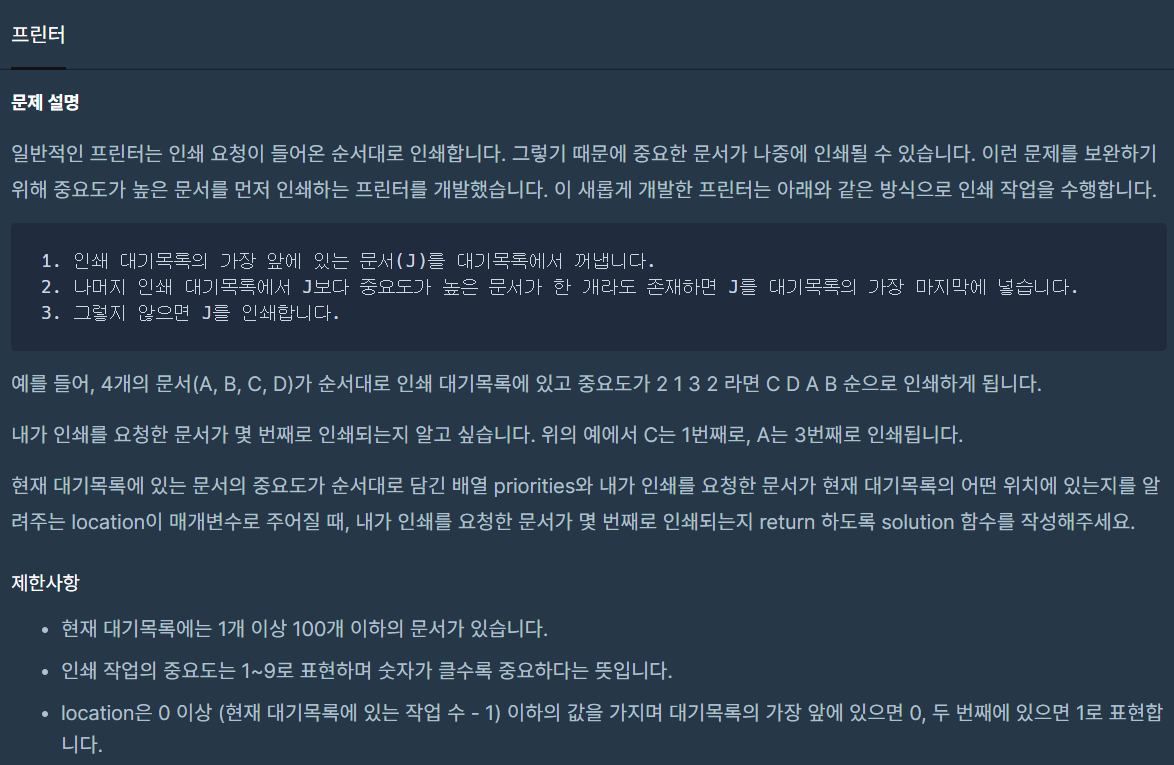
🔑풀이
import java.util.*
class Solution {
fun solution(priorities: IntArray, location: Int): Int {
val targetPriority = priorities[location]
val circularQueue = Array<Pair<Int, Boolean>>(priorities.size){index-> Pair(priorities[index], false)}.toList() as ArrayList<Pair<Int, Boolean>>
circularQueue[location] = circularQueue[location].copy(second = true)
var answer = 0
var headPair : Pair<Int, Boolean>
do {
val moveCount : Int = with(circularQueue){
map{it.first}.indexOf(maxOf { it.first })
}
with (circularQueue) {
Collections.rotate(this, -moveCount)
headPair = first()
removeAt(0)
}
answer++
} while (headPair.first > targetPriority)
circularQueue.add(0, headPair)
answer--
for (index in circularQueue.indices) {
if(circularQueue[index].first == targetPriority) answer++
if(circularQueue[index].second) break
}
return answer
}
}
🔨풀이 리펙토링
import java.util.*
class Solution {
data class WorkPair(val priority: Int, val isTarget: Boolean) : Comparable<WorkPair>{
override fun compareTo(nextWork: WorkPair) = this.priority.compareTo(nextWork.priority)
}
fun solution(priorities: IntArray, location: Int): Int {
var answer = 1
val queue : ArrayList<WorkPair> = Array<WorkPair>(priorities.size){ index-> WorkPair(priorities[index], false)}.also { it[location] = it[location].copy(isTarget = true)}.toList() as ArrayList<WorkPair>
while(true) {
with(queue) {
Collections.rotate(this, -indexOf(maxOf{it}))
}
if (!queue.first().isTarget) {
queue.removeAt(0)
answer++
} else {
break
}
}
return answer
}
}