๐Goal
์๋ฃ๊ตฌ์กฐ ๋์ ์๋ฆฌ๋ฅผ ์ดํด, ์ฅ๋จ์ ํ์
์๋ฃ๊ตฌ์กฐ๋?
๋ค์ํ๊ณ ์ ๋ง์ ๋ฐ์ดํฐ๋ค์ ์ด๋ป๊ฒ ํจ์จ์ ์ผ๋ก ๊ด๋ฆฌ ํ ๊ฒ์ธ์ง ํํํ๋ ๊ฒ. ex) ๋์๊ด์์์ ์ฑ
๋ถ๋ฅ
์๋ฃ๊ตฌ์กฐ๋ ํฌ๊ฒ ๋๊ฐ์ง๋ก ๋ถ๋ฅ
์ ํ ๊ตฌ์กฐ(1๋1 ์๋ฃ๊ฐ ๊ด๊ณ) - Array, Stack, Queue, Dequeue, List
๋น์ ํ ๊ตฌ์กฐ(1๋N ๋๋ N๋M์ ์๋ฃ๊ฐ์ ๊ด๊ณ) - Tree, Graph, Binary Tree, Heap
๐Stack(์คํ)
Stack์ ํ์
์ ์ถ(Last In First Out: LIFO)์ ์๋ฃ๊ตฌ์กฐ
๋จผ์ ์
๋ ฅ๋ ๊ฐ์ด ์ ์ผ ๋ง์ง๋ง์ ์ถ๋ ฅ ๊ฐ๋ฅํ ์๋ฃ๊ตฌ์กฐ
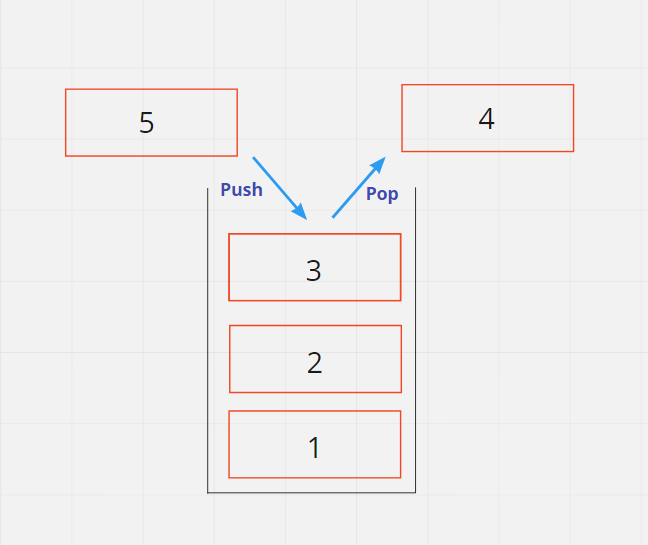
์คํ์ด ์คํ๋ ๋ ์ถ์์ ์ด๋ฏธ์ง
< ๋ง์ฝ Stack์ ๋ชจ๋ ์์๋ฅผ ์ง์ด๋ฃ์๋ค๊ฐ ์ถ๋ ฅํ๋ฉด ์ถ๋ ฅ๋ ๋ฆฌ์คํธ๋ ์ฒ์ ๋ฆฌ์คํธ์ ์ญ์์ด ๋๋ค. >
Stack์ ๊ฒฝ์ฐ Array ๋ฉ์๋์ Push, Pop์ ์๊ฐํ๋ฉด ์ฝ๋ค.
Stack์ ์๋๊ฒ์ Push / Stack์์ ๋นผ๋ด๋ ๊ฒ์ Pop
Stack ๊ตฌํ์ ํ์ ๋ณ์
MAX_SIZE : Stack์ ์ฌ์ด์ฆ, Array : ๋ฐ์ดํฐ๋ฅผ ๋ด์ ๋ฐฐ์ด, top : Stack์ ํฌ์ธํฐ, ์ด๊ธฐ๊ฐ = -1
top์ ํ์ฌ stack์์ ์์ฌ์ง ๋ฐ์ดํฐ ์ค์ ๊ฐ์ฅ ๋ง์ง๋ง ๋ฐ์ดํฐ ์์น๋ฅผ ๋ปํ๋ค. ๋ง์ฝ Stack ์์ ์๋ฌด๊ฒ๋ ์์ ์๋ฅผ ์๊ฐํด์ top์ ์ด๊ธฐ๊ฐ์ -1๋ก ์ค์ ํ๊ณ , ํ๋์ ๋ฐ์ดํฐ๊ฐ Stack์์ ์์ธ๋ค๋ฉด stack์ index = 0 ์ด๋ฏ๋ก top์ ๊ฐ๋ 0์ผ๋ก ์ค์ ๋ ์ ์๋๋ก ํ๋ค.
Stack ์ค๋ฅ
stack overflow / stack underflow
์ฃผ์ด์ง stack๋ฉ๋ชจ๋ฆฌ๋ณด๋ค ๋ฐ์ดํฐ๋ฅผ ๋ ๋ฃ์๊ฑฐ๋, stack์ด ๋ฉ๋ชจ๋ฆฌ๊ฐ ๋น์ด์๋๋ฐ ๊ฑฐ๊ธฐ์ ๋ฐ์ดํฐ๋ฅผ ๊บผ๋ด๋ ค๊ณ ํ ๋ ๋ฐ์ํ๋ ๋ํ์ ์ธ ์๋ฌ ex) ์ฌ๊ทํจ์ ๋ฌดํ ํธ์ถ์
์ฅ์ : ๊ฐ๋จํ๊ฒ ๊ตฌํ ๊ฐ๋ฅ
๋จ์ : ์คํ์ ํฌ๊ธฐ๊ฐ ๋ถํ์ค ํ ๋์๋ ํ์ฅ์ ํ์ฅ์ฐ์ฐ์ด ์ค๋ ๊ฑธ๋ฆฐ๋ค.
๐Stack(Pseudo Code)
var stackArr = [];
var element1 = 'air';
var element2 = 'fire';
stackArr.push(element1);
stackArr.push(element2);
console.log(stackArr);
stackArr.pop();
๐Stack(functional)
const Stack = function() {
const someInstance = {};
const storage = {};
var count = 0;
someInstance.push = function(value) {
storage[count] = value;
count++;
};
someInstance.pop = function() {
if (count > 0) {
var popValue = storage[count - 1];
delete storage[count - 1];
count--;
return popValue;
}
};
someInstance.size = function() {
return count;
};
return someInstance;
};