- Jest는 테스트 전 또는 후에 실행되어야 할 작업들을 다룰 수 있는 함수 제공
테스트 마다 반복 실행이 필요한 작업 - beforeEach / afterEach
- beforeEach/afterEach와 동일 레벨 또는 하위 레벨의 테스트가 실행 될 때 마다 반복적으로 실행
- 비동기 함수를 사용하는 경우 일반 테스트 함수와 동일하게 처리 (done 파라미터 사용, promise return)
beforeEach(() => {
return initializeCityDatabase();
});
afterEach(() => {
clearCityDatabase();
});
describe('beforeEach / afterEach Search Vienna', () => {
test('city database has Vienna', () => {
expect(isCity('Vienna')).toBeTruthy();
});
});
describe('beforeEach / afterEach Search San Juan', () => {
test('city database has San Juan', () => {
expect(isCity('San Juan')).toBeTruthy();
});
});
딱 한번 실행이 필요한 작업 - beforeAll / afterAll
- beforeAll/afterAll과 동일 레벨 또는 하위 레벨의 테스트가 실행 될 때 딱 한번만 실행
beforeAll(() => {
return initializeCityDatabase();
});
afterAll(() => {
clearCityDatabase();
});
describe('One-Time Setup - Vienna, Seoul', () => {
test('city database has Vienna', () => {
expect(isCity('Vienna')).toBeTruthy();
});
test('city database has Seoul', () => {
expect(isCity('Seoul')).toBeTruthy();
});
});
describe('One-Time Setup - San Juan', () => {
test('city database has San Juan', () => {
expect(isCity('San Juan')).toBeTruthy();
});
});
범위 지정 (Scoping)
beforeEach(() => {
return initializeCityDatabase();
});
describe('Outer Scope', () => {
test('city database has Vienna', () => {
expect(isCity('Vienna')).toBeTruthy();
});
test('city database has San Juan', () => {
expect(isCity('San Juan')).toBeTruthy();
});
describe('Inner Scope', () => {
beforeEach(() => {
return initializeFoodDatabase();
});
test('Vienna <3 sausage', () => {
expect(isValidCityFoodPair('Vienna', 'Wiener Schnitzel')).toBeTruthy();
});
test('San Juan <3 plantains', () => {
expect(isValidCityFoodPair('San Juan', 'Mofongo')).toBeTruthy();
});
});
});
beforeAll/afterAll, beforeEach/afterEach의 실행 순서
beforeAll(() => console.log('outer - beforeAll'));
afterAll(() => console.log('outer - afterAll'));
beforeEach(() => console.log('outer - beforeEach'));
afterEach(() => console.log('outer - afterEach'));
test('Outer test', () => console.log('outer - test'));
describe('Scoped / Nested block', () => {
beforeAll(() => console.log('inner - beforeAll'));
afterAll(() => console.log('inner - afterAll'));
beforeEach(() => console.log('inner - beforeEach'));
afterEach(() => console.log('inner - afterEach'));
test('Inner test', () => console.log('inner - test'));
});
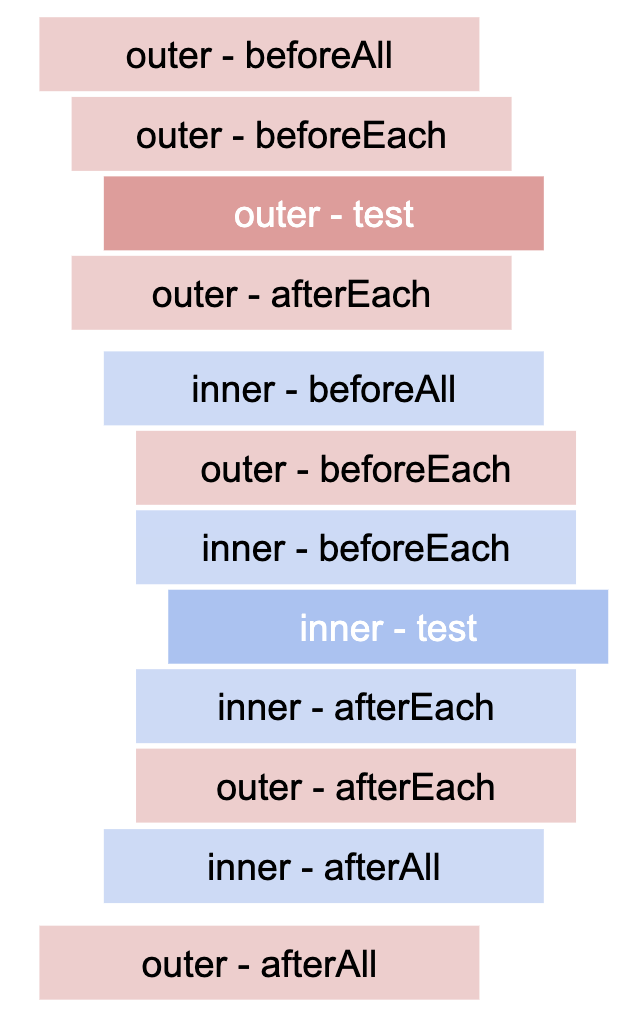
describe, test block의 실행 순서
- 실제 test 코드는 나머지 코드들이 먼저 실행되고 난 후 제일 마지막에 순서대로 실행 됨
describe('outer', () => {
console.log('describe outer-a');
describe('describe inner 1', () => {
console.log('describe inner 1');
test('test for describe inner 1', () => {
console.log('test for describe inner 1');
expect(true).toEqual(true);
});
});
console.log('describe outer-b');
test('test for outer 1', () => {
console.log('test for outer 1');
expect('ok').toEqual('ok');
});
describe('describe inner 2', () => {
console.log('describe inner 2');
test('test for describe inner 2', () => {
console.log('test for describe inner 2');
expect(false).toEqual(false);
});
})
console.log('describe outer-c');
});
테스팅 팁
- 테스트가 실패할 때 가장 먼저 체크해 봐야 될 것은 실패하는 테스트만 개별로 실행 했을 때에도 실패 하는지를 살펴 봐야 됨
- 임시로 해당 테스트 코드만 실행시키는 방법은
only
키워드를 추가해 주는 것
- 상태를 공유할 경우 다른 테스트에 영향을 받아서 해당 테스트가 실패하는 경우가 있음
beforeEach
함수를 통해서 해당 테스트 실행 전의 상태를 확인하는 것도 디버깅 방법 중 하나
test.only('this will be the only test that runs', () => {
expect(true).toBe(false);
});
test('this test will not run', () => {
expect('A').toBe('A');
});