BackGround Image
<style>
div.a{
background-image: url("../flower_ani/s1.JPG");
}
- background-image는 url을 통해 이미지를 배경에 도배
- default 조건 시 해당 이미지가 배경을 가득 채울 때까지 반복
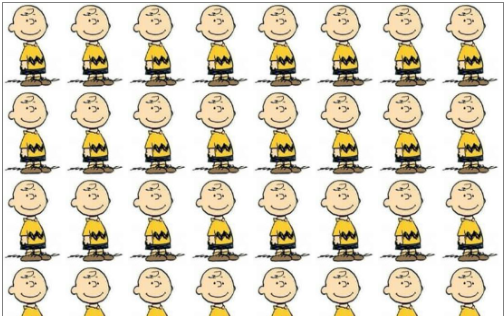
div.b{
background-image: url("../flower_ani/s2.JPG");
background-repeat: repeat-x;
}
div.c{
background-image: url("../flower_ani/s3.JPG");
background-repeat: repeat-y;
}
div.d{
background-image: url("../flower_ani/s4.JPG");
background-repeat: no-repeat;
background-size: 100px;
background-position: right bottom;
}
- background-repeat 조건을 통해 이미지 반복을 설정 가능
- repeat-x : 수평 반복(1회) / repeat-y : 수직 반복(1회) / no-repeat : 단 하나 출력 (모든 시작점은 좌상단)
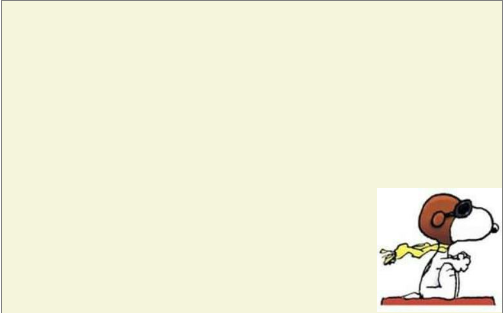
body{
background-image: url("../flower_ani/1.jpg");
background-repeat: no-repeat;
background-position: 800px 200px; /*x,y*/
}
</style>
<body>
<div class="a"></div>
<div class="b"></div>
<div class="c bg"></div>
<div class="d bg"></div>
</body>
Mouse Hovering
Style Tag Way
<style>
#myimg1:hover{
background-image: url("../flower_ani/1.jpg");
}
#myimg1:active{
background-image: url("../flower_ani/11.jpg");
}
</style>
- myimg id를 부여하여 hover와 active 조건 설정(조건은 : (colon)으로 연결)
- hover : 마우스 커서가 이미지에 올라가면 코드 실행
- active : 커서가 이미지를 클릭하면 코드 실행
Attributes Way
<body>
<img src="../Food/f1.png" title="꽃"
onmouseover="this.src='../flower_ani/12.jpg'"
onmouseout="src='../Food/f1.png'">
<img src="../html_img/1.png"
onmouseenter="this.src='../html_img/2.png'"
onmouseleave="this.src='../html_img/1.png'"> /*this.는 img 자체의미*/
</body>
- onmouseover과 onmouseset은 한 쌍이며 커서를 올리면 변경되는 조건과 빠져나오면 변경되는 조건
- onmouseenter과 onmouseleave는 한 쌍이며 위와 같은 기능
Tables
Style
<style>
table{
border: 1px solid gray;
border-collapse: collapse;
}
tr,th,td{
border: 1px solid purple;
}
</style>
- table style은 표 전체의 외곽 선 조건
- border-collapse: collapse; 조건을 통해 표 전체의 이중 선들이 겹쳐짐
Tables Elements
<body>
<table>
<tr>
<th>이름</th>
<th>학교</th>
<th>학과</th>
<th>학년</th>
</tr>
<tr>
<td>이성신</td>
<td>쌍용대</td>
<td>자바</td>
<td>2학년</td>
</tr>
</table>
</body>
- <tr>은 하나의 행을 작성, <th>는 제목 행(text bold, align center), <td>는 데이터 행
RowSpan & ColumnSpan
<table class="tb2">
<caption><b>[표 문제_span]</b></caption>
<tr>
<td rowspan="2" align="center">1</td>
<td colspan="2">2</td>
<td align="center">3</td>
</tr>
<tr>
<td colspan="3">4</td>
</tr>
<tr align="center">
<td>5</td>
<td>6</td>
<td>7</td>
<td>8</td>
</tr>
</table>
- rowspan은 여러 row를 하나로 묶어줌(상하)
- colspan은 여러 column을 하나로 묶어줌(좌우)
- rowspan은 span이 시작하는 가장 윗 행에 설정하면 설정한 행까지 행 생성된 것으로 인식
- colspan은 span이 시작하는 가장 좌측 열에 설정하면 설정한 열까지 열 생성된 것으로 인식
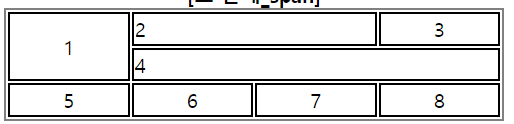
Using BootStrap
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0/dist/css/bootstrap.min.css" rel="stylesheet">
<title>Document</title>
</head>
- 부트스트랩으로부터 필요한 링크를 link 태그에 삽입
<body>
<table class="table table-striped" style="width: 500px;">
<tr>
<th>학번</th>
<th>이름</th>
<th>학과</th>
<th>학년</th>
</tr>
<tr>
<td>204801</td>
<td>홍성경</td>
<td>법학과</td>
<td>2</td>
</tr>
<tr>
<td colspan="4" align="center">
<button type="button" class="btn btn-outline-info">전송</button>
<button type="button" class="btn btn-outline-warning">취소</button>
</td>
</tr>
</table>
</body>
- 부트스트랩으로부터 table의 양식과 button 양식을 받아옴
- 부트스트랩에서 지정한 형식 그대로 입력해야 양식 받아오기 가능
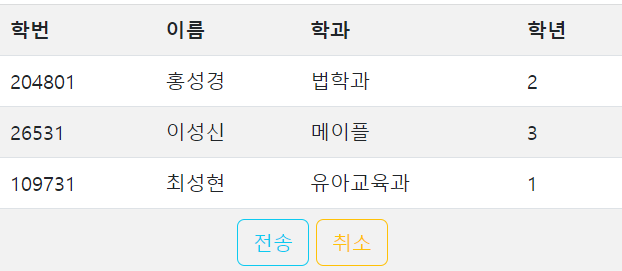
Link
<style>
a:link{
color: black;
text-decoration: none;
}
a:visited{
color: blueviolet;
text-decoration: none;
}
a:hover{
color: red;
text-decoration: underline;
}
</style>
- link 조건은 <a>태그의 default 조건과 거의 동일
- visited 조건은 링크를 방문했을 때 실행되는 조건
- hover 조건은 커서를 링크에 올렸을 때 실행되는 조건
List Usages
<body>
<div>
<ul>
<li class="main">
<a href="#">7월12일</a>
<ul class="submenu">
<li>
<a href="../day0712/ex1_htmlTag.html">1번예제</a>
</li>
<li>
<a href="../day0712/ex2_htmlTag.html">2번예제</a>
</li>
<li>
<a href="../day0712/quiz.html">퀴즈</a>
</li>
</ul>
</li>
</ul>
<ul>
<li class="main">
<a href="#">7월13일</a>
<ul class="submenu">
<li>
<a href="../day0713/ex01_htmlTag.html">1번예제</a>
</li>
<li>
<a href="../day0713/ex02_olul.html">2번예제</a>
</li>
<li>
<a href="../day0713/ex03_textStyle.html">3번예제</a>
</li>
</ul>
</li>
</ul>
</body>
- <a>태그의 참조를 “#”이라 지정하면 하이퍼링크가 생성되지만 이동하는 링크가 없음을 의미
- 리스트 내의 각 요소들 내부에 또 리스트 작성 가능
<style>
a {
text-decoration: none;
}
ul li{
list-style: none;
}
li.main{
float: left;
}
li.main>a{
color: red;
font-weight: bold;
display: block;
width: 180px;
}
ul.submenu{
font-size: 12pt;
margin-top: 20px;
}
ul.submenu>li>a{
display: block;
width: 180px;
height: 20px;
color: orange;
}
</style>
- 위 <body>태그에 활용되는 <style> 조건들
- text-decoration: none; 조건은 하이퍼링크의 밑줄을 제거
- list-style: none;은 리스트의 순서 표기를 제거
- float: left;를 통해 큰 리스트가 우측으로 정렬
- Selector를 정확히 지정하지 않으면 엉뚱한 태그에 조건이 부여됨
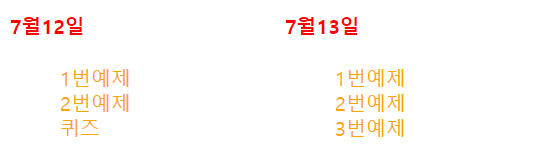