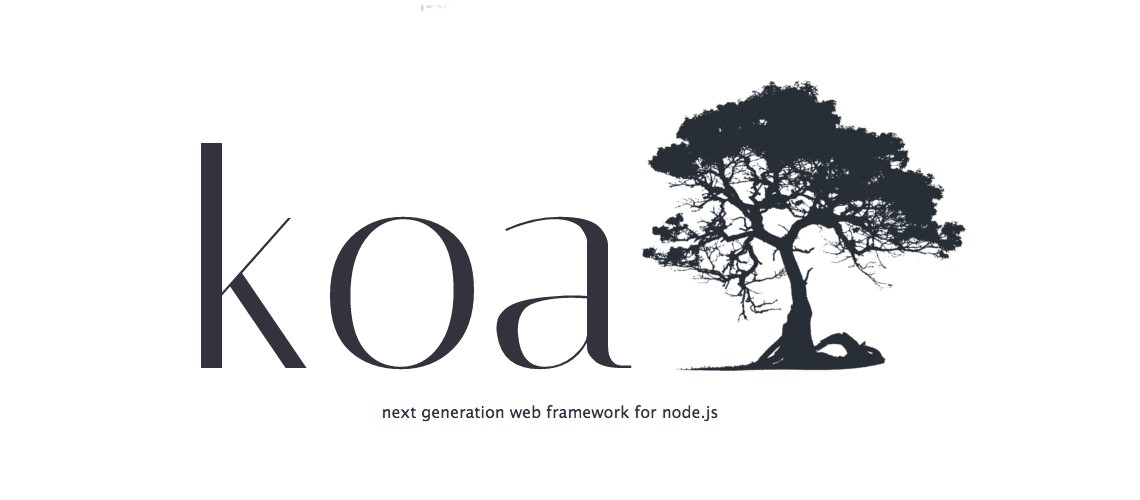
โ๏ธ ํ๋ก์ ํธ ์ ๋ฆฌ
- Koa๋ฅผ ์ฌ์ฉํ์ฌ ๋ฐฑ์๋ ์๋ฒ๋ฅผ ๋ง๋๋ ๊ธฐ๋ณธ ๊ฐ๋
์ ๋ํด์ ๊ณต๋ถํ์ต๋๋ค.
- REST API๋ฅผ ์ดํด๋ณด๊ณ ์ด๋ป๊ฒ ๋์ํ๋์ง ์๋ฐ์คํฌ๋ฆฝํธ๋ฅผ ๋ฐฐ์ด์ ํตํด ๋ฐฐ์๋ณด์์ต๋๋ค.
- koa-router๋ฅผ ์ด์ฉํ์ฌ ๊ฒฝ๋ก์ ๋ฐ๋ผ ๋ค๋ฅธ ์์
์ ํ ์ ์๊ฒ ๋์์ค๋๋ค.
- MongoDB๋ฅผ ์ด์ฉํ์ฌ ๋ฐ์ดํฐ๋ฒ ์ด์ค๋ฅผ ์ฌ์ฉํ๊ณ ๊ด๋ฆฌํ ์์ ์
๋๋ค.
โ๏ธ ์ฝ๋
const Router = require('koa-router');
const postsCtrl = require('./posts.ctrl');
const posts = new Router();
posts.get('/', postsCtrl.list);
posts.post('/', postsCtrl.write);
posts.get('/:id', postsCtrl.read);
posts.delete('/:id', postsCtrl.remove);
posts.put('/:id', postsCtrl.replace);
posts.patch('/:id', postsCtrl.update);
module.exports = posts;
let postId = 1;
const posts = [
{
id: 1,
title: '์ ๋ชฉ',
body: '๋ด์ฉ',
},
];
exports.write = ctx => {
const { title, body } = ctx.request.body;
postId += 1;
const post = { id: postId, title, body };
console.log(post)
posts.push(post);
ctx.body = post;
};
exports.list = ctx => {
ctx.body = posts;
};
exports.read = ctx => {
const { id } = ctx.params;
const post = posts.find(p => p.id.toString() === id);
if (!post) {
ctx.status = 404;
ctx.body = {
message: 'ํฌ์คํธ๊ฐ ์กด์ฌํ์ง ์์ต๋๋ค.',
};
return;
}
ctx.body = post;
};
exports.remove = ctx => {
const { id } = ctx.params;
const index = posts.findIndex(p => p.id.toString() === id);
if (index === -1) {
ctx.status = 404;
ctx.body = {
message: 'ํฌ์คํธ๊ฐ ์กด์ฌํ์ง ์์ต๋๋ค.',
};
return;
}
posts.splice(index, 1);
ctx.status = 204;
};
exports.replace = ctx => {
const { id } = ctx.params;
const index = posts.findIndex(p => p.id.toString() === id);
if (index === -1) {
ctx.status = 404;
ctx.body = {
message: 'ํฌ์คํธ๊ฐ ์กด์ฌํ์ง ์์ต๋๋ค.',
};
return;
}
posts[index] = {
id,
...ctx.request.body,
};
ctx.body = posts[index];
};
exports.update = ctx => {
const { id } = ctx.params;
const index = posts.findIndex(p => p.id.toString() === id);
if (index === -1) {
ctx.status = 404;
ctx.body = {
message: 'ํฌ์คํธ๊ฐ ์กด์ฌํ์ง ์์ต๋๋ค.',
};
return;
}
posts[index] = {
...posts[index],
...ctx.request.body,
};
ctx.body = posts[index];
};