1. FriendProfile.js에 상단 UI 작성
import React from 'react';
import {
View,
Text,
TouchableOpacity,
ScrollView,
Image,
SafeAreaView,
} from 'react-native';
import Ionic from 'react-native-vector-icons/Ionicons';
import Feather from 'react-native-vector-icons/Feather';
const FriendProfile = ({route, navigation}) => {
const {name, profileImage, post, followers, following} = route.params;
return (
<SafeAreaView
style={{
width: '100%',
backgroundColor: 'white',
}}>
<View style={{padding: 10}}>
<View style={{flexDirection: 'row', alignItems: 'center'}}>
<TouchableOpacity onPress={() => navigation.goBack()}>
<Ionic name="arrow-back" style={{fontSize: 20, color: 'black'}} />
</TouchableOpacity>
<View
style={{
flexDirection: 'row',
justifyContent: 'space-between',
alignItems: 'center',
width: '92%',
}}>
<Text style={{fontSize: 15, marginLeft: 10, fontWeight: 'bold'}}>
{name}
</Text>
<Feather
name="more-vertical"
style={{fontSize: 20, color: 'black'}}
/>
</View>
</View>
</View>
</SafeAreaView>
);
};
export default FriendProfile;
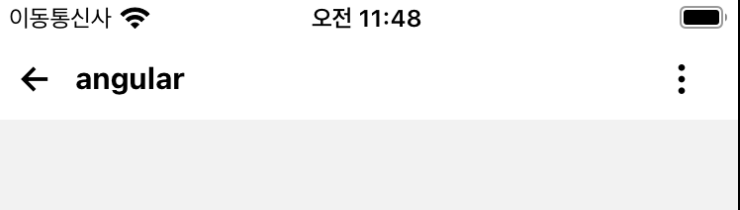
2. ProfileBody.js 컴포넌트 작성
ProfileBody.js 컴포넌트 만들기

FriendProfile.js에 ProfileBody.js import
<ProfileBody
name={name}
profileImage={profileImage}
post={post}
followers={followers}
following={following}
/>
</View>
</SafeAreaView>
);
};
코드 작성
import React from 'react';
import {View, Text, Image} from 'react-native';
import Feather from 'react-native-vector-icons/Feather';
const ProfileBody = ({
name,
accountName,
profileImage,
post,
followers,
following,
}) => {
return (
<View>
{accountName ? (
<View
style={{
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'space-between',
}}>
<View
style={{
flexDirection: 'row',
alignItems: 'center',
}}>
<Text
style={{
fontSize: 18,
fontWeight: 'bold',
}}>
{accountName}
</Text>
<Feather
name="chevron-down"
style={{
fontSize: 20,
color: 'black',
paddingHorizontal: 5,
opacity: 0.5,
}}
/>
</View>
<View style={{flexDirection: 'row', alignItems: 'center'}}>
<Feather
name="plus-square"
style={{
fontSize: 25,
color: 'black',
paddingHorizontal: 15,
}}
/>
<Feather
name="menu"
style={{
fontSize: 25,
}}
/>
</View>
</View>
) : null}
<View
style={{
flexDirection: 'row',
alignItems: 'center',
justifyContent: 'space-around',
paddingVertical: 20,
}}>
<View
style={{
alignItems: 'center',
}}>
<Image
source={profileImage}
style={{
resizeMode: 'cover',
width: 80,
height: 80,
borderRadius: 100,
}}
/>
<Text
style={{
paddingVertical: 5,
fontWeight: 'bold',
}}>
{name}
</Text>
</View>
<View style={{alignItems: 'center'}}>
<Text style={{fontWeight: 'bold', fontSize: 18}}>{post}</Text>
<Text>게시물</Text>
</View>
<View style={{alignItems: 'center'}}>
<Text style={{fontWeight: 'bold', fontSize: 18}}>{followers}</Text>
<Text>팔로워</Text>
</View>
<View style={{alignItems: 'center'}}>
<Text style={{fontWeight: 'bold', fontSize: 18}}>{following}</Text>
<Text>팔로잉</Text>
</View>
</View>
</View>
);
};
export default ProfileBody;
<ProfileBody
name={name}
profileImage={profileImage}
post={post}
followers={followers}
following={following}
/>
<ProfileButton id={1} />
import {StyleSheet, Text, View, TouchableOpacity} from 'react-native';
import React, {useState} from 'react';
import Feather from 'react-native-vector-icons/Feather';
const ProfileButton = ({id}) => {
const [follow, setFollow] = useState(follow);
return (
<>
{id === 0 ? (
<></>
) : (
<View
style={{
width: '100%',
flexDirection: 'row',
justifyContent: 'space-evenly',
alignItems: 'center',
}}>
<TouchableOpacity
onPress={() => setFollow(!follow)}
style={{width: '42%'}}>
<View
style={{
width: '100%',
height: 35,
borderRadius: 5,
backgroundColor: follow ? null : '#3493D9',
borderWidth: follow ? 1 : 0,
borderColor: '#DEDEDE',
justifyContent: 'center',
alignItems: 'center',
}}>
<Text style={{color: follow ? 'black' : 'white'}}>
{follow ? '팔로잉' : '팔로우'}
</Text>
</View>
</TouchableOpacity>
<View
style={{
width: '42%',
height: 35,
borderWidth: 1,
borderColor: '#DEDEDE',
justifyContent: 'center',
alignItems: 'center',
borderRadius: 5,
}}>
<Text>메시지</Text>
</View>
<View
style={{
width: '10%',
height: 35,
borderWidth: 1,
borderColor: '#DEDEDE',
justifyContent: 'center',
alignItems: 'center',
borderRadius: 5,
}}>
<Feather
name="chevron-down"
style={{fontSize: 20, color: 'black'}}
/>
</View>
</View>
)}
</>
);
};
export default ProfileButton;
const styles = StyleSheet.create({});
4. FriendItem.js 코드 작성
FriendItem.js 컴포넌트 생성

FriendProfile.js 코드 추가
import {FriendsProfileData} from '../components/Database';
import FriendItem from '../components/FriendItem';
...
<ProfileButton id={1} />
<Text style={{paddingVertical: 10, fontSize: 15, fontWeight: 'bold'}}>
회원님을 위한 추천
</Text>
<ScrollView
horizontal={true}
showsHorizontalScrollIndicator={false}
style={{paddingTop: 10}}>
{name === FriendsProfileData.name
? null
: FriendsProfileData.map((data, index) => (
<FriendItem key={index} data={data} name={name} />
))}
</ScrollView>
</View>
FriendItem.js 코드 작성
import {View, Text, TouchableOpacity, Image} from 'react-native';
import React, {useState} from 'react';
import AntDesign from 'react-native-vector-icons/AntDesign';
const FriendItem = ({data, name}) => {
const [isFollow, setIsFollow] = useState(false);
const [close, setClose] = useState(false);
return (
<View>
{data.name === name || close ? null : (
<View
style={{
width: 160,
height: 200,
margin: 3,
justifyContent: 'center',
alignItems: 'center',
borderWidth: 0.5,
borderColor: '#DEDEDE',
borderRadius: 2,
position: 'relative',
}}>
<TouchableOpacity
onPress={() => setClose(true)}
style={{
position: 'absolute',
top: 10,
right: 10,
}}>
<AntDesign
name="close"
style={{
fontSize: 20,
color: 'black',
opacity: 0.5,
}}
/>
</TouchableOpacity>
<Image
source={data.profileImage}
style={{
width: 80,
height: 80,
borderRadius: 100,
margin: 10,
}}
/>
<Text style={{fontWeight: 'bold', fontSize: 16}}>{data.name}</Text>
<Text style={{fontSize: 12}}>{data.accountName}</Text>
<TouchableOpacity
onPress={() => setIsFollow(!isFollow)}
style={{width: '80%', paddingVertical: 10}}>
<View
style={{
width: '100%',
height: 30,
borderRadius: 5,
backgroundColor: isFollow ? null : '#3493D9',
borderWidth: isFollow ? 1 : 0,
borderColor: '#DEDEDE',
justifyContent: 'center',
alignItems: 'center',
}}>
<Text style={{color: isFollow ? 'black' : 'white'}}>
{isFollow ? '팔로잉' : '팔로우'}
</Text>
</View>
</TouchableOpacity>
</View>
)}
</View>
);
};
export default FriendItem;