Spring Boot
필요 라이브러리
* MyBatis
xml
annotation
* 메일 처리
jsp - smtp(직접 설정할 수는 있지만 돈 내야한다)
* 파일 업로드
MultipartRequest - cos.jar(사용) - Boot에서 자동 제공이 아님
Apache Common Upload - 이것을 이용하여 사용
package com.example.controller;
import java.io.File;
import java.io.IOException;
import java.util.UUID;
import javax.servlet.http.HttpServletRequest;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.multipart.MultipartFile;
import org.springframework.web.servlet.ModelAndView;
import ch.qos.logback.core.recovery.ResilientSyslogOutputStream;
@RestController
public class UploadController {
@RequestMapping("/form.do")
public ModelAndView mail(HttpServletRequest request) {
// 타임스탬프 확인용
//System.out.println(System.currentTimeMillis());
// 나노단위로하면 중복이 더 확실히 제거
//System.out.println(System.nanoTime());
// uuid
//System.out.println(UUID.randomUUID().toString());
// session id - 장바구니같은거 만들때 사용
ModelAndView modelAndView = new ModelAndView();
modelAndView.setViewName("form");
return modelAndView;
}
@RequestMapping("/form_ok.do")
public ModelAndView form_ok(MultipartFile upload) {
try {
System.out.println(upload.getOriginalFilename());
System.out.println(upload.getSize());
// 중복파일 제거 흐름
// 파일명_타임스탬프(1970년부터... ~). 확장자
// 타임스탬프 : System / Date / Calendar
// System.out.println(System.currentTimeMillis());
// 파일명과 확장자 분리 - xxx.jpg / xx.xx.xx.jpg 형식도 있어서 split으로 하기 힘들수도 있음
// +1을 없애주면 .이 포함되서 나옴 / 확장자명
String extension = upload.getOriginalFilename().substring(upload.getOriginalFilename().lastIndexOf(".") );
// 파일명
String filename = upload.getOriginalFilename().substring(0, upload.getOriginalFilename().lastIndexOf("."));
System.out.println(extension);
System.out.println(filename);
long timestamp = System.currentTimeMillis();
// 중복파일을 제거한 흐름 생성
String newfilename = filename + "-" + timestamp + extension;
// transferTo : 메모리에 있는파일을 실제로 저장해달라
upload.transferTo(new File(newfilename));
} catch (IllegalStateException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
ModelAndView modelAndView = new ModelAndView();
modelAndView.setViewName("form_ok");
return modelAndView;
}
@RequestMapping("/form_ok2.do")
public ModelAndView form_ok2(MultipartFile upload, HttpServletRequest request) {
System.out.println(upload.getOriginalFilename());
System.out.println(request.getParameter("data"));
ModelAndView modelAndView = new ModelAndView();
modelAndView.setViewName("form_ok");
return modelAndView;
}
}
파일 업로드시 타임스탬프가 붙으며 중복파일에 대한 구별 / 처리를 해줌
실제로 경로에는 올려주지 않아서 콘솔로 값 나오는지 확인
@RequestMapping("/form_ok2.do")
public ModelAndView form_ok2(MultipartFile upload, HttpServletRequest request) {
System.out.println(upload.getOriginalFilename());
System.out.println(request.getParameter("data"));
ModelAndView modelAndView = new ModelAndView();
modelAndView.setViewName("form_ok");
return modelAndView;
}
<form action="form_ok2.do" method="post" enctype="multipart/form-data" >
파일 <input type="file" name="upload" />
/
데이터 <input type="text" name="data" />
<input type="submit" value="파일 업로드" />
</form>
upload폴더 경로로 변경
spring.servlet.multipart.location=C:/java/boot-workspace/BootUpload/src/main/webapp/upload
@RequestMapping("/form_ok3.do")
// 다중 파일을 받기위해 []로 설정
public ModelAndView form_ok3(MultipartFile[] uploads) {
// 업로드 파일 개수 확인
System.out.println(uploads.length);
// 업로도된 파일 이름 확인
for(MultipartFile upload : uploads) {
System.out.println(upload.getOriginalFilename());
}
ModelAndView modelAndView = new ModelAndView();
modelAndView.setViewName("form_ok");
return modelAndView;
}
여러개의 파일 업로드여서 name 은 uplods로 복수형태로 이름 설정.
multiple="multiple" 추가
<form action="form_ok3.do" method="post" enctype="multipart/form-data" >
파일 <input type="file" name="uploads" multiple="multiple" />
<input type="submit" value="파일 업로드" />
</form>
아직 다른 메서드에는 실제 파일 업로드 기능을 넣어주지 않아서 form_ok에서 실행
create table album_cmt_board2 (
seq int not null primary key auto_increment,
subject varchar(150) not null,
writer varchar(12) not null,
mail varchar(50),
password varchar(12) not null,
content varchar(2000),
cmt int not null,
cmtyes char(1),
hit int not null,
wip varchar(15) not null,
wdate datetime not null
);
create table album_cmt_comment2 (
seq int not null primary key auto_increment,
pseq int not null,
writer varchar(12) not null,
password varchar(12) not null,
content varchar(2000) not null,
wdate datetime not null
);
create table album_cmt_file2 (
seq int not null primary key auto_increment,
pseq int not null,
filename varchar(200) not null,
filesize int not null,
latitude varchar(12),
longitude varchar(12),
wdate datetime not null
);
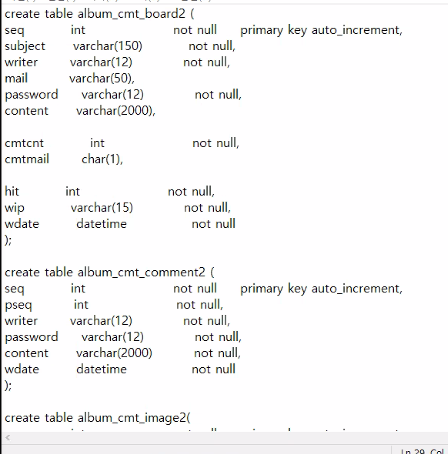