📌 url()
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
#wrap{
width:500px; height:500px;
margin:0 auto; border: 1px solid black;
}
#content{
width:80%; height:100%;
margin:0 auto;
background-image: url("https://picsum.photos/200/300");
color:white; font-size: 2em; font-weight: bold; text-align: center;
}
</style>
</head>
<body>
<div id="wrap">
<div id="content">
해당 이미지는 저작권 보호를 받습니다.
</div>
</div>
</body>
</html>
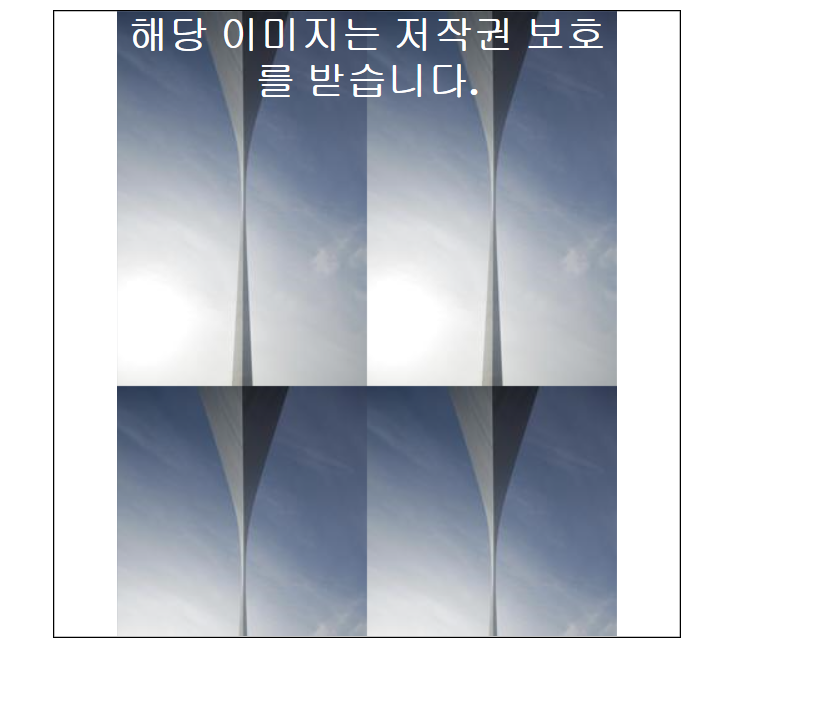
📌 display 속성
✅ inline / block / none
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
body div:nth-child(1), body div:nth-child(2), body div:nth-child(3), body div:nth-child(4){
width:100px; height:100px;
background-color: goldenrod; color:white; font-weight: bold; text-align: center;
}
body div:nth-child(5), body div:nth-child(7){
width:100px; height:100px;
background-color: grey; color:white; font-weight: bold;
text-align:center;
display:inline;
margin:10px 10px 10px 10px;
}
body div:nth-child(6){
width:100px; height:100px;
background-color: grey; color:white; font-weight: bold;
text-align:center;
display:none;
}
body div:nth-child(8){
width:100px; height:100px;
background-color: tomato; color:white; font-weight: bold;
text-align: center;
display:inline-block;
}
</style>
</head>
<body>
<div>
content1
</div>
<div>
content2
</div>
<div>
content3
</div>
<div>
content4
</div>
<div>
content5
</div>
<div>
content6
</div>
<div>
content7
</div>
<div>
content8
</div>
</body>
</html>
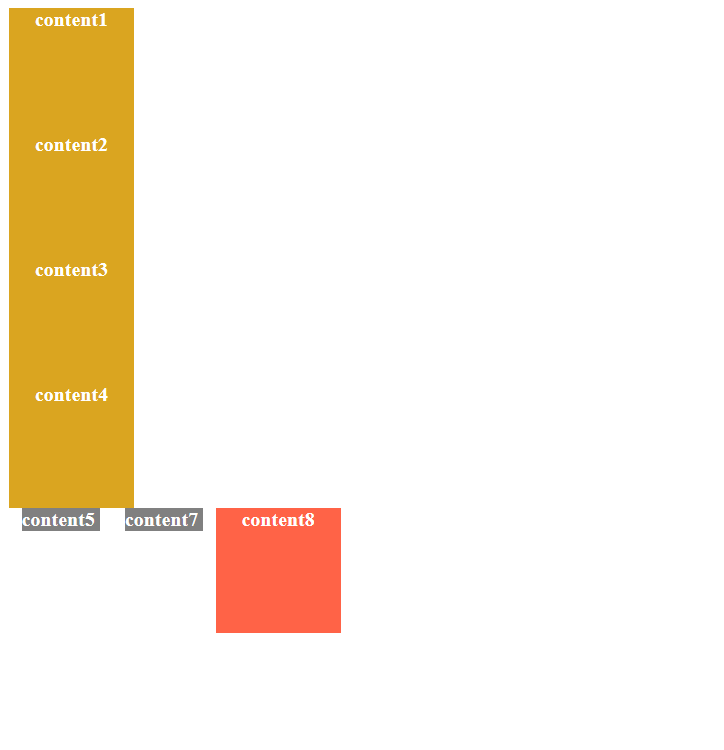
📌 visibility 속성
✅ display:none / visibility:hidden
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
#display_none{
margin-bottom: 10px;
}
#display_none div:nth-child(1), #display_none div:nth-child(3){
width:100px; height:100px;
background-color:tomato;
color:white; text-align: center;
font-weight: bold;
}
#display_none div:nth-child(2){
width:100px; height:100px;
background-color:tomato;
color:white; text-align: center;
font-weight: bold;
display:none;
}
#visibility_hidden div:nth-child(1), #visibility_hidden div:nth-child(3){
width:100px; height:100px;
background-color:darkblue;
color:white; text-align: center;
font-weight: bold;
}
#visibility_hidden div:nth-child(2){
width:100px; height:100px;
background-color:tomato;
color:white; text-align: center;
font-weight: bold;
visibility: hidden;
}
</style>
</head>
<body>
<div id="display_none">
<div>content1</div>
<div>content2</div>
<div>content3</div>
</div>
<div id="visibility_hidden">
<div>content1</div>
<div>content2</div>
<div>content3</div>
</div>
</body>
</html>
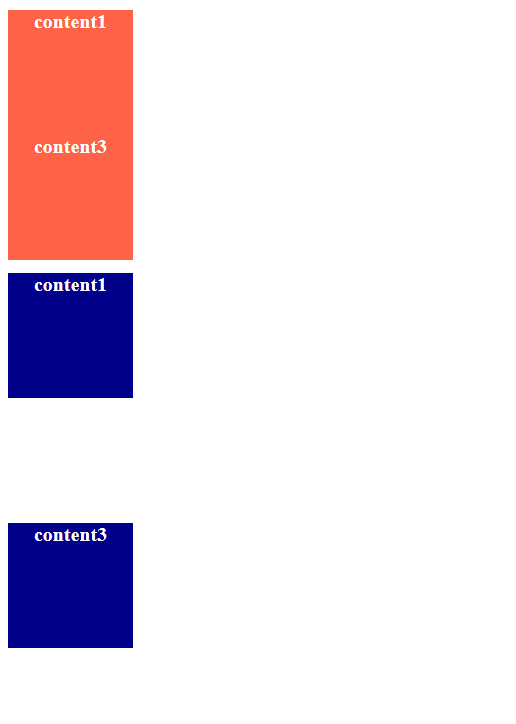
📌 opacity 속성
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
*{ margin:0; padding:0; }
ul li{
display:inline-block;
width:200px; height:100px;
text-align: center;
background-color: grey;
}
ul li:nth-child(2){
background-color: grey;
opacity: 0.7;;
}
ul li:nth-child(3){
background-color: grey;
opacity: 0.4;;
}
</style>
</head>
<body>
<ul>
<li>content1</li>
<li>content2</li>
<li>content3</li>
</ul>
</body>
</html>
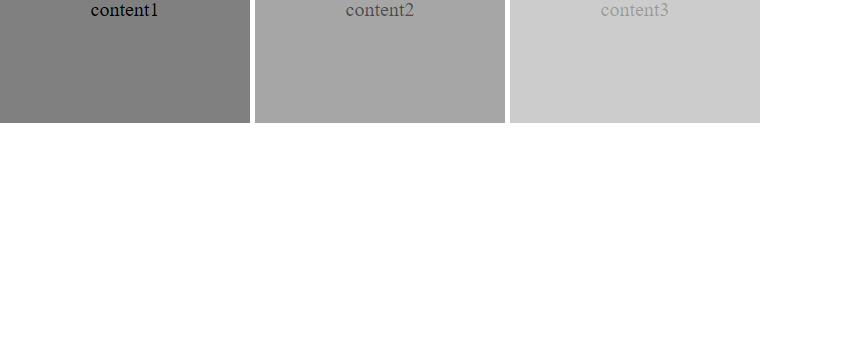