https://developer.apple.com/videos/play/wwdc2020/10097/?time=141
UICollectionView를 구성하는 API는 3개의 카테고리로 구분된다
- Data: "what"
- Layout: "where"
- Presentation
무엇이(어떤 데이터가)
어디에(어떤 레이아웃에)
보여지는지(어떻게 렌더링되는지)
위의 구분이 UICollectionView를 유동적으로 만드는 데 핵심적인 역할을 한다
Advances
iOS 6

- Data는 indexPath-based protocol인 UICollectionViewDataSource로 관리된다
- Layout에 대해서는 abstract class인 UIColelctionViewLayout이 있고,
주로 구체적인 subclass인 UICollectionViewFlowLayout을 사용했다
- Presentation에는 2개의 view type이 있다 (그림 참조)
iOS 13

- Data와 Layout에 대해 new components를 소개한다
- These are APIs to use for building apps with UICollectionView today.
iOS 14
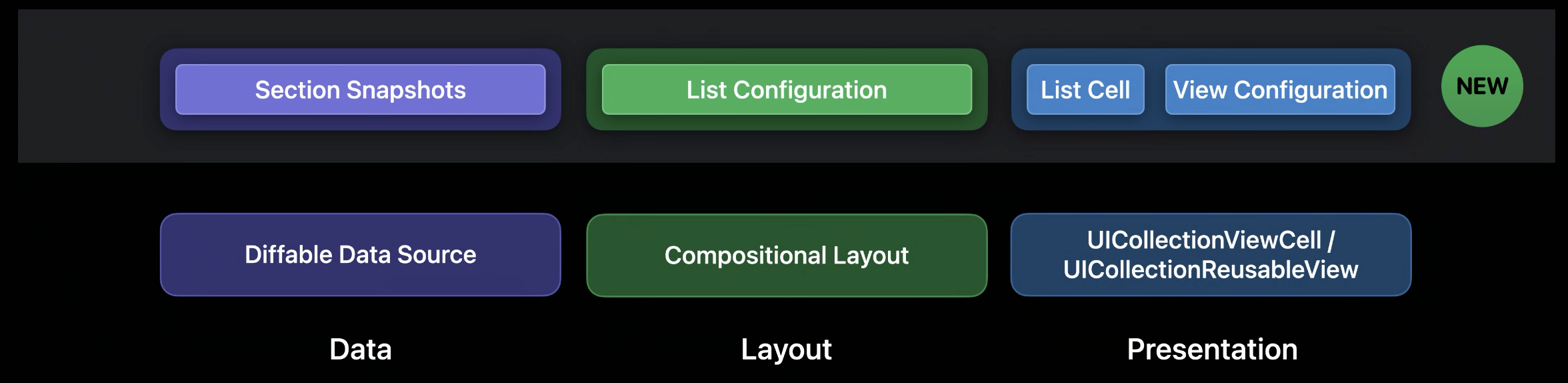
- 위의 modern APIs를 기반으로 new features를 소개한다
Data
Diffable Data Source
- new snapshot data type의 추가로, UI State의 관리를 간단하게 만든다
- snapshot은 unique section and item identifiers의 사용을 통해
전체적인 UI State를 캡슐화(encapsulate)한다
- UICollectionView를 업데이트할 때,
- 새로운 snapshot을 만들고, (create a new snapshot)
- 현재의 UI State으로 그걸 모으고, (populate it)
- 그걸 data source에 적용한다 (apply it)
Section Snapshots
- iOS 14에서 새로운 snapshot type을 추가했다
- UICollectionView의 single section에 대한 데이터를 캡슐화한다
- this enhancement에 대한 2가지 이유가 있다
- data source가 section-sized로 데이터를 쉽게 구성하기 위함
- hierarchical dat에 대한 modeling을 하기 위함
- outline-style UI rendering 지원이 필요하다
- iOS 14에서 전반적으로 볼 수 있는 common visual design이다
Example
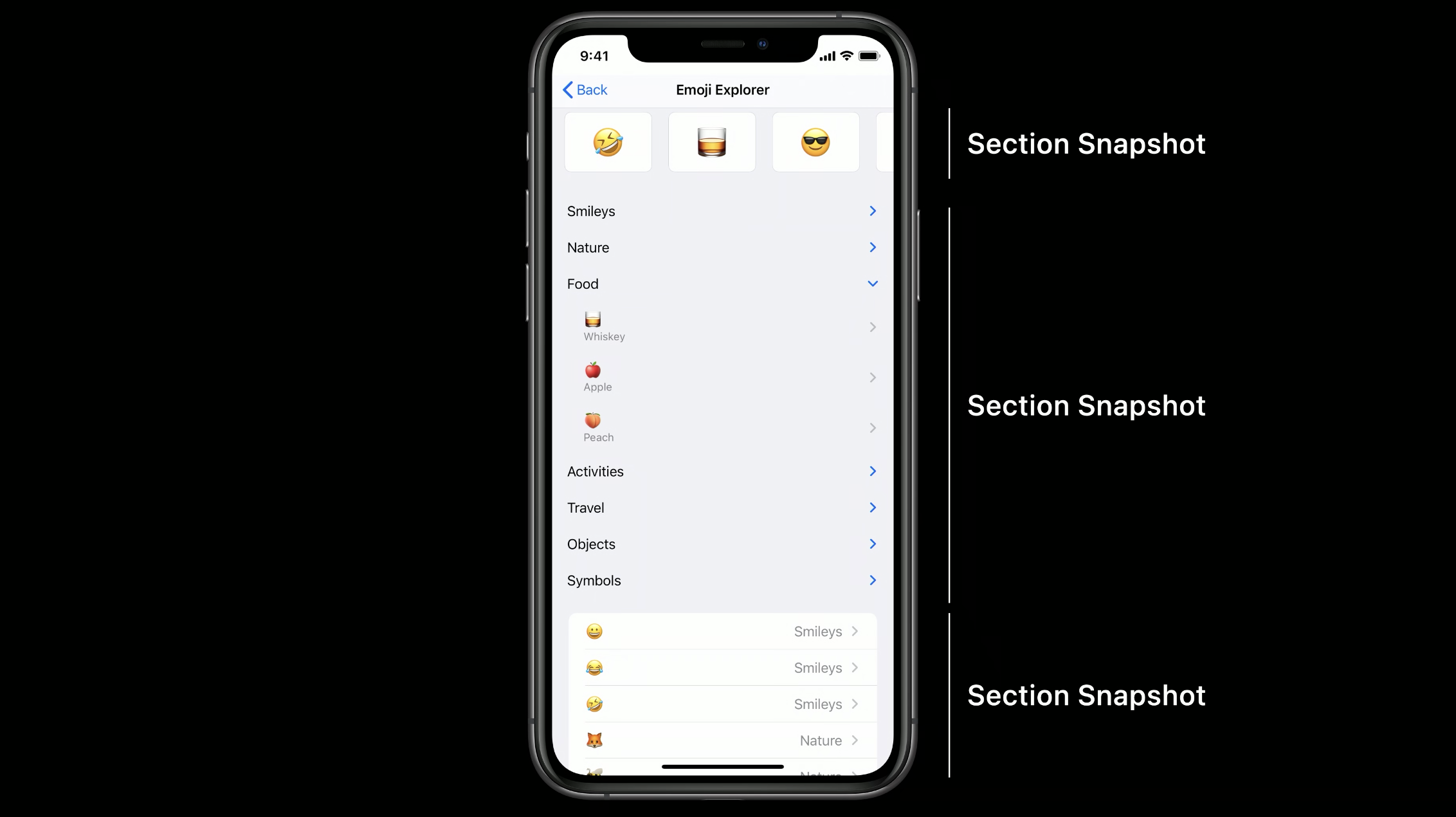
- 첫 번째 section에서는,
using a single section snapshot to completely model the content found here
- 두 번째 section에서는,
expandable collapsible outline style을 확인할 수 있고,
이러한 hierarchical data를 modeling 한다
- 세 번째 section에서는,
third section snapshot으로 content를 다시 modeling한다
- 위 예시는 iOS 14에 추가된 new section snapshot에 대한 overview이다.
Layout
Compositional Layout
- it allows to build rich, complex layouts by composing
smaller, easy-to-reason bits of layout together
(작은 레이아웃들 구성해서 복잡하고 풍성한 레이아웃 만들 수 있게 한다?)
- layout이 어떻게 일해야 하는지가 아닌, 어떻게 생겨야 하는지를 describe하면 되기 때문에 빠르다
- 또한 보다 sophisticated한 UI를 만드는 것을 도와주기 위해
section-specific layout을 만들 수 있게 한다
- 또또한 orthogonal scrolling sections에 대한 지원도 있다
List Configuration
- Compositional Layout 기반으로 만들어졌고, 컬렉션뷰를 테이블뷰처럼 사용할 수 있게 한다. 섹션별로 다른 레이아웃 설정 가능하고, 스와이프 등의 기능 구현도 가능하다
- UICollectionLayoutListConfiguration
- UICollectionViewCompositionalLayout과 NSCollectionLayoutSection을 기반으로 구성되어 있다
- 따라서 유연한 레이아웃 대응이 가능하고, 특히 self-sizing 기능을 통해 셀 높이를 수동으로 계산할 필요가 없다
- iOS 14에서, list라는 새로운 feature가 생겻다
- Lists는 UICollectionView에서 UITableView-like section을 만들 수 있게 한다
- 요러한 lists는 UITableView에서 기대하는 특징들을 많이 가진다
- swipe action이나 many common cell layouts
-
list-style layout을 만드는 건 간단하다
-
List는 Compositional Layout 기반으로 build되므로,
List들과 other kinds of layout을 on a per-section basis로
mix and match 할 수 있다
func layout() -> UICollectionViewLayout {
let configuration = UICollectionLayoutListConfiguration(appearance: .grouped)
let layout = UICollectionViewCompositionalLayout.list(using: configuration)
return layout
}
- 이 외에도 다양한 appearance를 만들 수 있다
Presentation
Cell Registration
- iOS 14에서, cell registration이라고 불리는 새로운 API를 통해
cell을 configure하는 brand-new way가 생겻다
- cell registration은 cell을 간단하고, 재사용 가능하게 만든다. from a view model
- cell registration eliminate the extra step
of registering a cell class or nib
to associate it with a reuse identifier
- 대신, view model로부터 cell을 만들기 위해
configuration closure를 포함하는
generic registration type을 이용한다
-
Example
let reg = UICollectionView.CellRegistration<MyCell, ViewModel> { cell, indexPath, model in
}
let dataSource = UICollectionViewDiffableDataSource<S,I>(collectionView: collectionView) {
collectionView, indexPath, item -> UICollectionViewCell in
return collectionView.dequeueConfiguredReusableCell(using: reg, for: indexPath, item: item)
}
- First, cell registration을 만든다. cell class에 대해 generic 타입을 갖는다 (Mycell)
- 새로운 cell이 만들어질 때, closure가 호출된다.
- 요기서 we configure the cell's content from ViewModel
- cell registration과 함께라면 더이상 register를 부를 필요가 없다
Cell Content Configuration
- cell content configuration은 cell에 대한 standardized layout을 제공한다.
- similar to what is seen in UITableView standard cell types
-
이러한 configuration은 any cell에나 사용될 수 있다.
심지어는 일반적인 UIView에도 사용이 가능하다.
var contentConfiguration = UIListContentConfiguration.cell()
contentConfiguration.image = UIImage(systemName:"hammer")
contentConfiguration.text = "Ready. Set. Code"
cell.contentConfiguration = contentConfiguration
var contentConfiguration = UIListContentConfiguration.valueCell()
contentConfiguration.image = UIImage(systemName:"hammer")
contentConfiguration.text = "Ready. Set. Code."
contentConfiguration.secondaryText = "#WWDC20"
cell.contentConfiguration = contentConfiguration
var contentConfiguration = UIListContentConfiguration.subtitleCell()
contentConfiguration.image = UIImage(systemName:"hammer")
contentConfiguration.text = "Ready. Set. Code."
contentConfiguration.secondaryText = "#WWDC20"
cell.contentConfiguration = contentConfiguration
-
이러한 content configuration type은 매우 lightweight하고,
content가 어떻게 생겨야 하는지 간단하게 describe할 수 있다
-
The framwork takes care of all the layout considerations and automatically optimizes for performances
Background Configuration
- content configuration과 비슷하지만, 모든 cell의 background에 적용되어
색상이나 테두리 스타일 같은 properties를 조절한다
수업 코드 정리
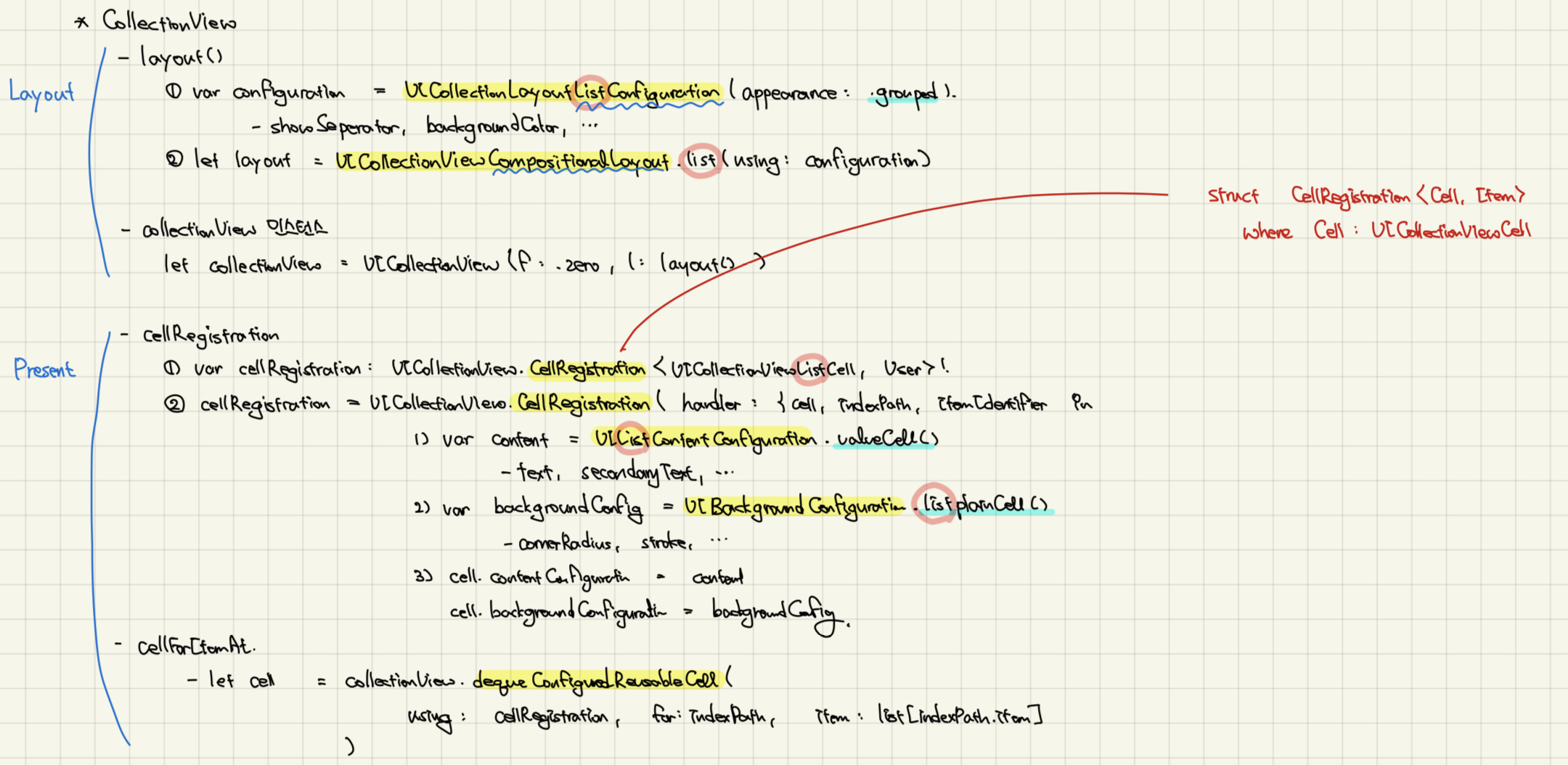
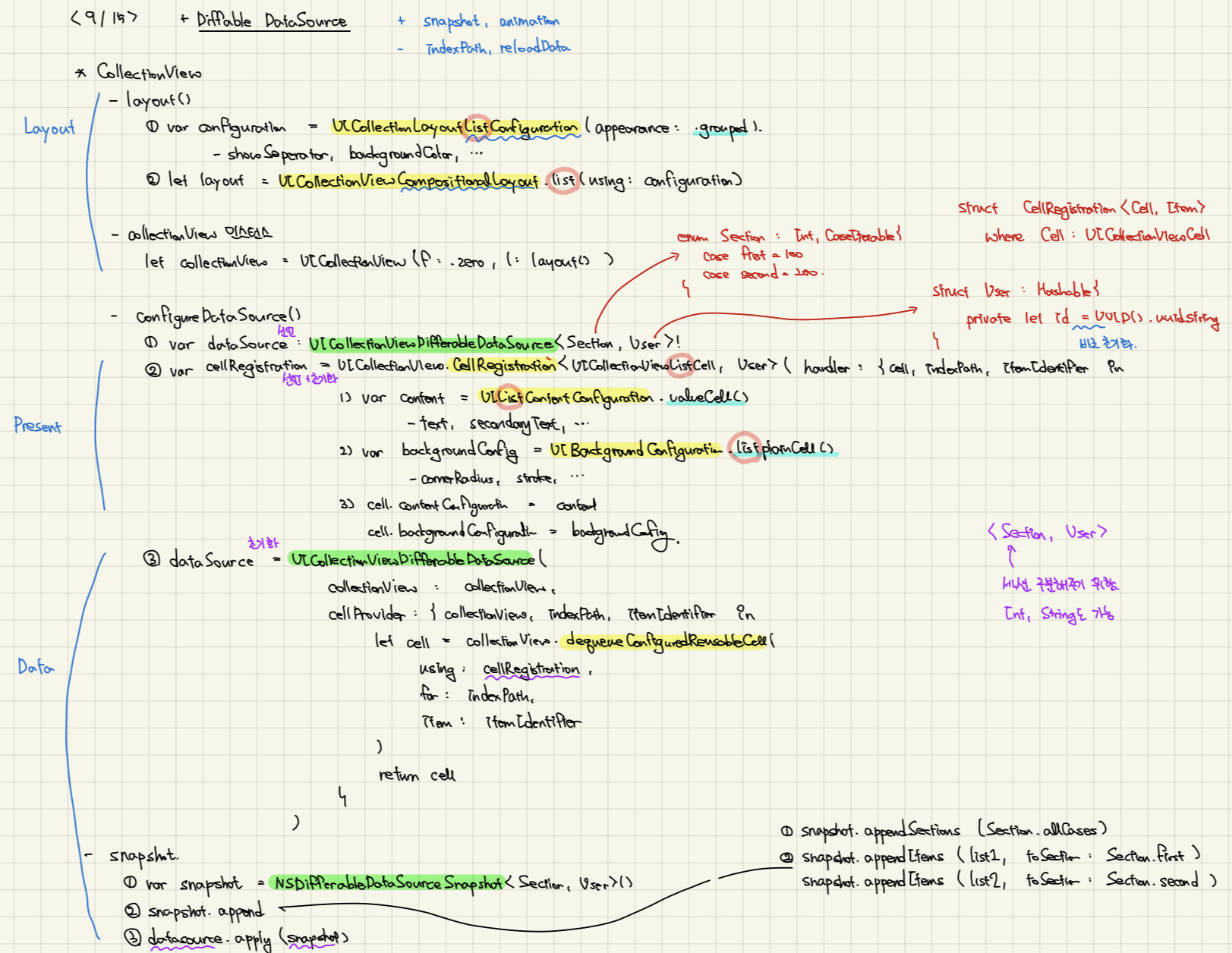
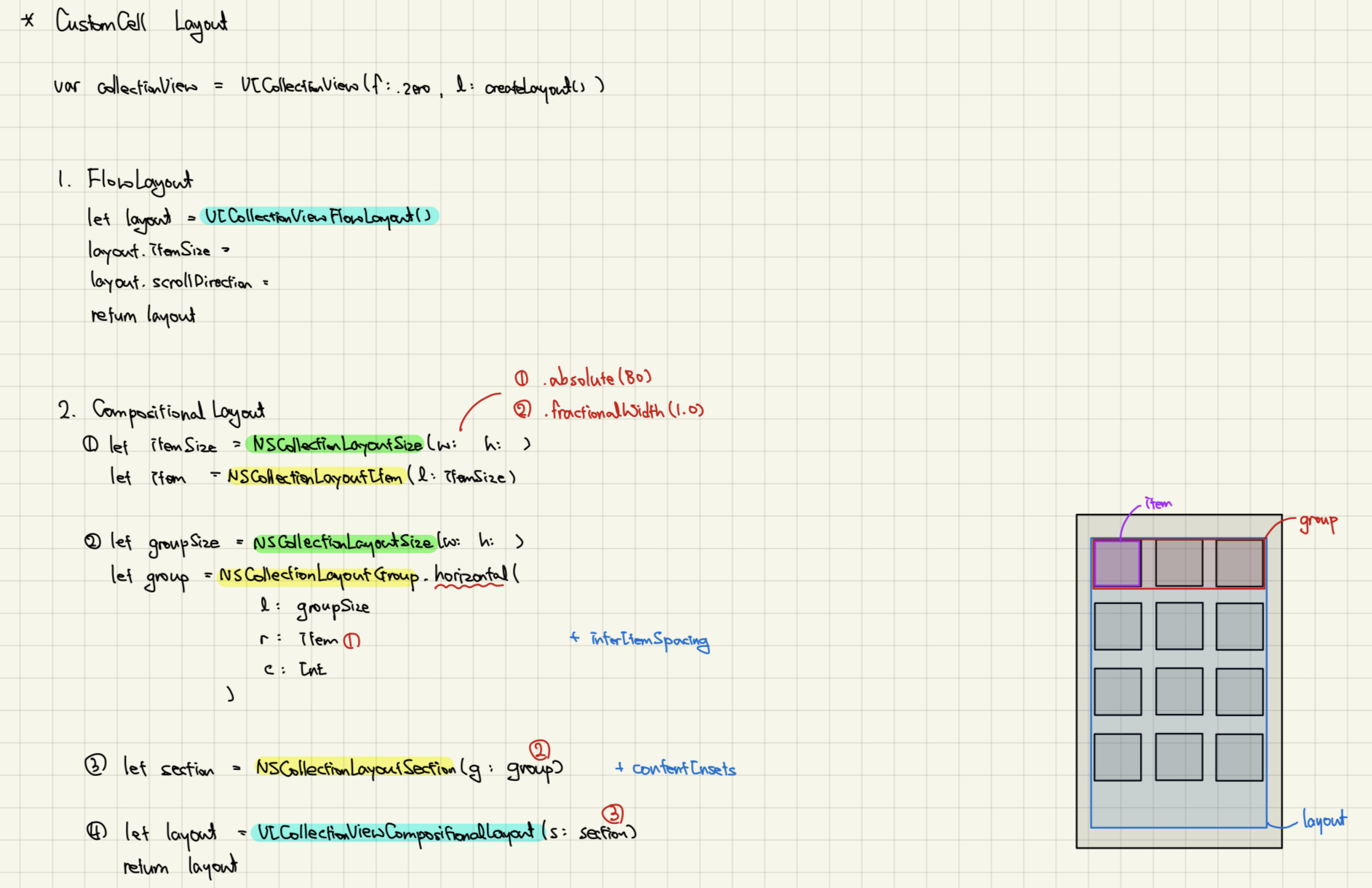