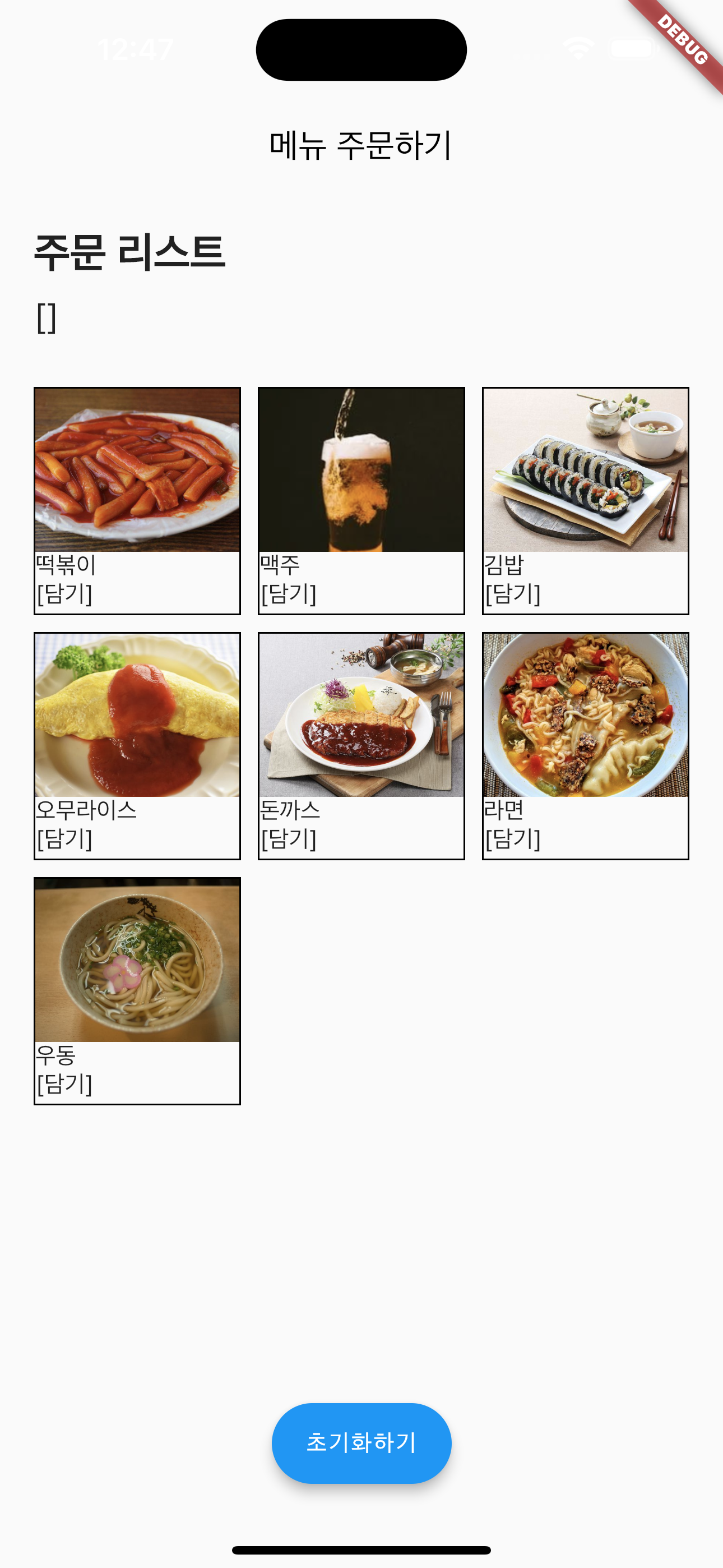
import 'package:flutter/material.dart';
import 'package:kiosk_app/gridViewTile.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatefulWidget {
const MyApp({super.key});
@override
State<MyApp> createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
List<String> list = [];
void _onTabMeun(String name) {
list.add(name);
setState(() {});
}
void _onTabReset() {
list.clear();
setState(() {});
}
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: const Text(
"메뉴 주문하기",
style: TextStyle(color: Colors.black),
),
elevation: 0,
backgroundColor: Colors.transparent,
),
body: Padding(
padding: const EdgeInsets.all(20),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
const Text(
"주문 리스트",
style: TextStyle(
fontWeight: FontWeight.bold,
fontSize: 25,
),
),
const SizedBox(
height: 10,
),
Text(
"$list",
style: const TextStyle(
fontSize: 20,
),
),
const SizedBox(
height: 30,
),
Expanded(
child: GridView(
gridDelegate: const SliverGridDelegateWithFixedCrossAxisCount(
crossAxisCount: 3,
crossAxisSpacing: 10,
mainAxisSpacing: 10,
childAspectRatio: 5 / 5.5,
),
children: [
GridViewTile(
name: "떡볶이",
imgUrl: "assets/option_bokki.png",
onTap: _onTabMeun,
),
GridViewTile(
name: "맥주",
imgUrl: "assets/option_beer.png",
onTap: _onTabMeun,
),
GridViewTile(
name: "김밥",
imgUrl: "assets/option_kimbap.png",
onTap: _onTabMeun,
),
GridViewTile(
name: "오무라이스",
imgUrl: "assets/option_omurice.png",
onTap: _onTabMeun,
),
GridViewTile(
name: "돈까스",
imgUrl: "assets/option_pork_cutlets.png",
onTap: _onTabMeun,
),
GridViewTile(
name: "라면",
imgUrl: "assets/option_ramen.png",
onTap: _onTabMeun,
),
GridViewTile(
name: "우동",
imgUrl: "assets/option_udon.png",
onTap: _onTabMeun,
),
],
),
)
],
),
),
floatingActionButtonLocation: FloatingActionButtonLocation.centerFloat,
floatingActionButton: FloatingActionButton.extended(
onPressed: _onTabReset,
label: const Text("초기화하기"),
),
),
);
}
}