02. JavaScript Clock
Learning Point
- 현재 시간을 읽어들여 움직이는 시계를 완성시키기
- html만 보고 css를 직접 짜보는 것도 많은 도움이 될 듯하다
- static과 relative의 차이점 학습
CSS Part
* {
font-family: "Spoqa Han Sans Neo", "sans-serif";
}
html {
background-color: #e1dcd9;
text-align: center;
font-size: 10px;
}
body {
margin-top: 50px;
font-size: 2rem;
display: flex;
flex-direction: column;
flex: 1;
}
header {
font-size: 42px;
color: #8f8681;
}
.clock_letter {
color: #666666;
font-size: 90px;
}
.clock {
width: 20rem;
height: 20rem;
border: 20px solid #fdfdfd;
border-radius: 50%;
margin: 0 auto;
margin-top: 60px;
position: relative;
padding: 2rem;
box-shadow: 0 0 0 4px rgba(0, 0, 0, 0.1), inset 0 0 0 3px #efefef,
inset 0 0 10px black, 0 0 10px rgba(0, 0, 0, 0.2);
}
.clock-face {
position: relative;
width: 100%;
height: 100%;
transform: translateY(-3px);
}
.clock_center {
position: relative;
top: 50%;
left: 50%;
width: 15px;
height: 15px;
background: black;
border-radius: 50%;
transform: translate(-50%, -50%);
z-index: 10000;
}
.hand {
background: #666666;
position: absolute;
top: 50%;
transform-origin: 100%;
}
.hour-hand {
margin-left: 10%;
width: 40%;
height: 6px;
background: #8f8681;
}
.min-hand {
margin-left: 5%;
width: 45%;
height: 5px;
}
.second-hand {
width: 50%;
height: 6px;
height: 3px;
}
Javascript Part
const hourHand = document.querySelector(".hour-hand");
const minHand = document.querySelector(".min-hand");
const secondHand = document.querySelector(".second-hand");
const clockLetter = document.querySelector(".clock_letter");
function setClock() {
let today = new Date();
let hours = today.getHours();
let minutes = today.getMinutes();
let seconds = today.getSeconds();
let hourDeg = hours * (360 / 12) + 90;
let minuteDeg = minutes * (360 / 60) + 90;
let secondDeg = seconds * (360 / 60) + 90;
hourHand.style.transform = `rotate(${hourDeg}deg)`;
minHand.style.transform = `rotate(${minuteDeg}deg)`;
secondHand.style.transform = `rotate(${secondDeg}deg)`;
clockLetter.innerHTML = `${formatSetter(hours)}:${formatSetter(
minutes
)}:${formatSetter(seconds)}`;
}
function formatSetter(time) {
if (time < 10) time = "0" + time;
return time;
}
setInterval(setClock, 1000);
정리
Position - Static vs Relative
- static
- default 값으로 설정됨
- top, bottom, left, right의 속성값이 적용되지 않음
- 앞에 설정한 position을 무시할 때도 사용
- relative
- static으로 부터의 위치를 계산
- 즉, 원래 static이었을때 있어야 되는 위치로부터 top, bottom, left , right등의 속성 먹음
Min-height : 100vh
- min-height는 height 속성의 최소 높이를 정의함.
- transform이 일어나는 기준점을 정의한다.
- top, right, bottom, left 값과 %값으로도 설정 할 수 있다.
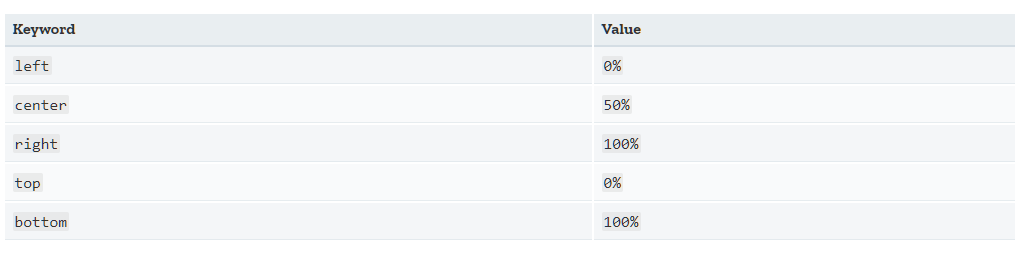
- 이번에는 시계 바늘을 회전시키기 위해서 transform-origin : 100%써 오른쪽 기준으로 회전이 되도록 설정했다.